The `extern "C"` linkage specification in C++ is used to indicate that the included code should use C linkage, allowing for interoperability with C code and avoiding name mangling.
extern "C" {
void myCFunction(int a);
}
Understanding `extern "C"`
What is `extern`?
The keyword `extern` in C++ is used to declare a variable or a function and indicates that the entity is defined in another file. This is essential for maintaining visibility between different files and ensuring proper linkage during the program compilation process. In simpler terms, when you declare something as `extern`, you tell the compiler, "This is defined elsewhere, so don't focus on its details here."
What is `"C"`?
When we use `"C"` in the `extern` declaration, we're signaling that we want to use C linkage conventions instead of C++ linkage. In C++, when you define a function, the compiler typically applies name mangling, which modifies the function's name to include information about its parameters. This is why a C function would not be callable directly from C++ without proper declaration. Using `extern "C"` prevents name mangling, allowing C++ code to link and call C functions directly.

When to Use `extern "C"`
Mixing C and C++ Code
Interoperability between C and C++ is a common scenario in software development. Developers often have existing C libraries that they want to use in their C++ projects. Without `extern "C"`, linking errors will occur due to the differences in how functions and variables are named in the two languages.
Use Cases
- Invoking C libraries in C++ code: If you're using a popular C library—as many C libraries are well-optimized and widely used—you will need to declare the C functions with `extern "C"`.
- Writing C++ code that can be called from C: If you want to expose some of your C++ functions to be callable from C, you use `extern "C"` in their declarations.
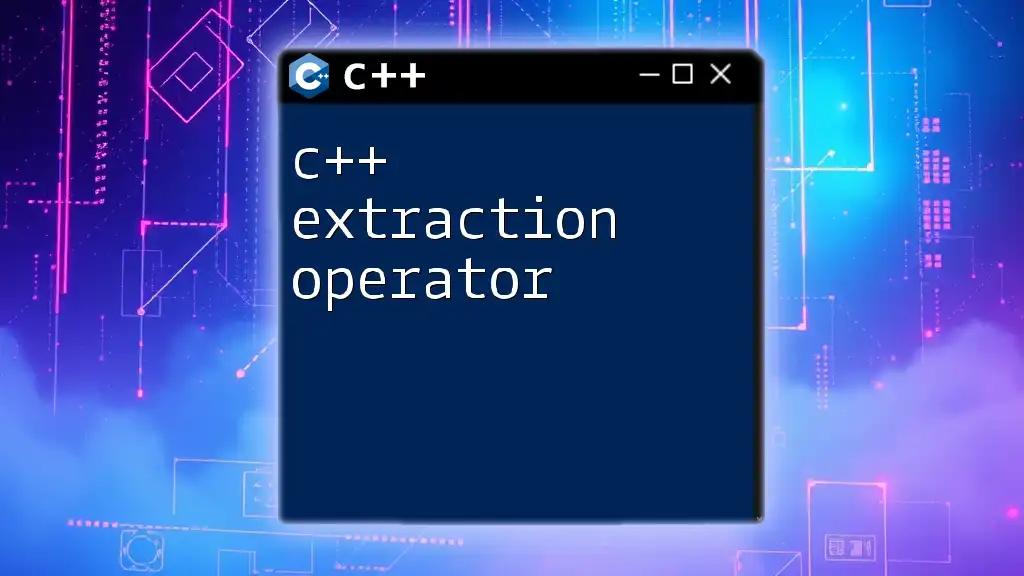
How to Use `extern "C"`
Basic Syntax
The syntax for declaring functions with `extern "C"` is straightforward. Here’s an example code snippet:
extern "C" {
void exampleFunction();
}
This code informs the compiler that `exampleFunction` has C linkage, allowing for proper linking with programs or libraries written in C.
Wrapping C Functions
To wrap a C function, you can encapsulate it within `extern "C"` in your C++ code. Consider the following example:
extern "C" {
void myCFunction(int x) {
// Implementation
// Perform some operation with x
}
}
In this example, `myCFunction` is defined in C++ but can be called from C as it follows the C linkage rules. This is particularly important when you're dealing with legacy code or libraries not originally designed with C++ in mind.

Compilation and Linking
Name Mangling in C++
Name mangling is a process by which the compiler encodes additional information into function names, such as parameter types, making them unique. For instance, a function like `void myFunction(int)` will be mangled into something like `_Z12myFunctioni`, depending on the compiler.
Using `extern "C"` prevents this mangling, enabling seamless linking with C functions. Here’s a simple demonstration of how a function may appear with mangled names in C++:
// C++ Code
void myFunction(); // Mangled name will be different
Compiling C and C++ Together
To compile a project that involves both C and C++ files, it's crucial to have the correct compilation commands. Here is an example of how to compile both types of files using `g++` and `gcc`:
g++ -o myProgram myProgram.cpp myLibrary.c
This command tells the compiler to produce an executable named `myProgram`, utilizing both the C++ source file `myProgram.cpp` and the C source file `myLibrary.c`.
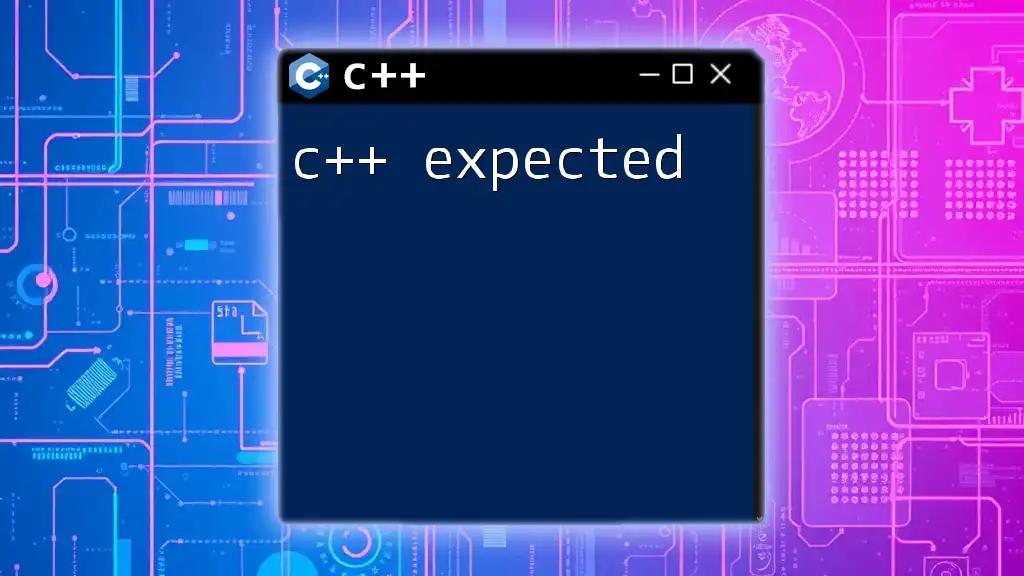
Best Practices
Encapsulating C Headers with `extern "C"`
To effectively manage C headers that will be used in C++, it's a good practice to encapsulate the C function declarations with `extern "C"`. This allows you to prevent compilation errors due to naming conflicts or linkage issues. Here's an example using conditional compilation:
#ifdef __cplusplus
extern "C" {
#endif
void myCFunction();
#ifdef __cplusplus
}
#endif
This pattern ensures that your header files remain compatible with both C and C++ compilers.
Understanding Linkage Specifications
When working with `extern "C"`, it’s important to know when and where to apply it. Applying `extern "C"` directly on function declarations or definitions within a header file grants clarity and maintains modularity in your codebase. It is a best practice to use it consistently for any functions interfacing with C libraries.
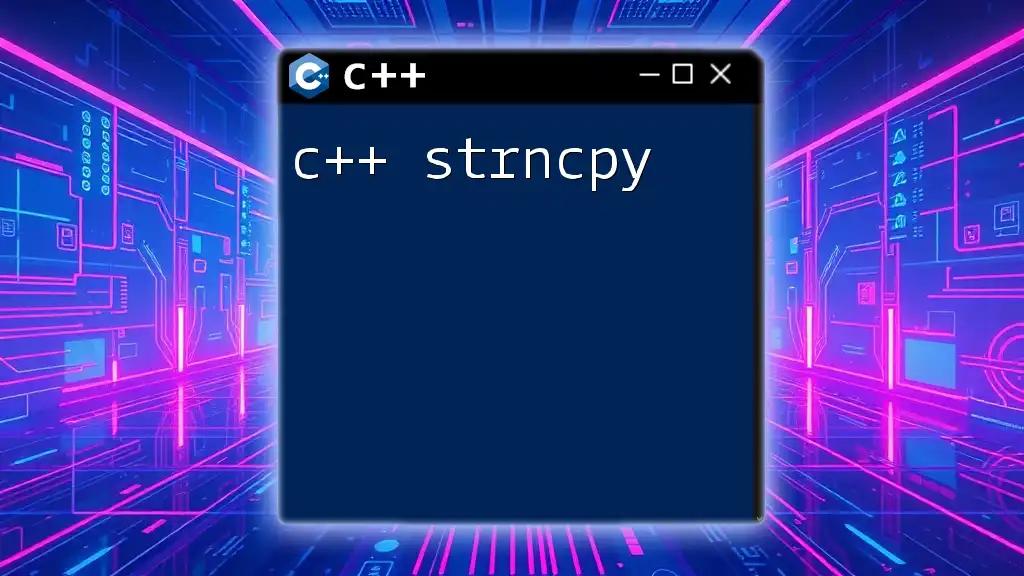
Common Pitfalls and Errors
Mistakes with `extern "C"`
One of the most common mistakes is misunderstanding where to place `extern "C"`. If omitted, it can lead to linker errors, such as unresolved symbols, when trying to combine C++ and C modules.
For instance, attempting to call a C function defined without `extern "C"` from C++ code will yield a linking error, as the names will not match due to mangling.
Debugging Tips
If you encounter linkage errors, you may need to identify whether your function declarations are correctly formatted with `extern "C"`. You can utilize tools like `nm` on Unix-like systems to inspect symbols and determine if name mangling is causing issues.
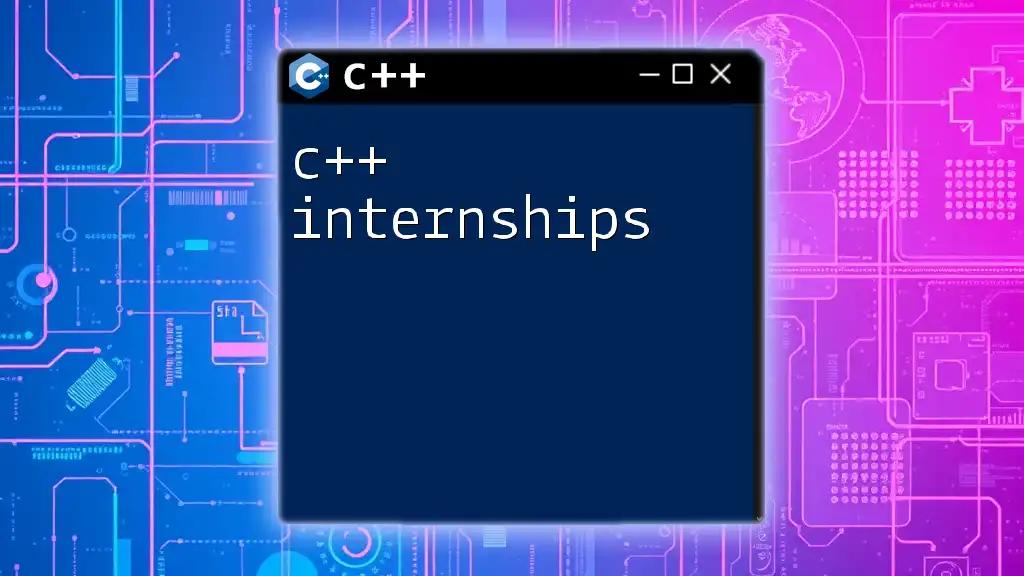
Conclusion
In conclusion, understanding and implementing `extern "C"` in your C++ projects is essential for integrating C libraries and achieving interoperability between C and C++. By following best practices and avoiding common pitfalls, you can leverage the strengths of both languages in your application development. Experimenting with `extern "C"` can open up new possibilities in your coding projects and improve your programming skills significantly.
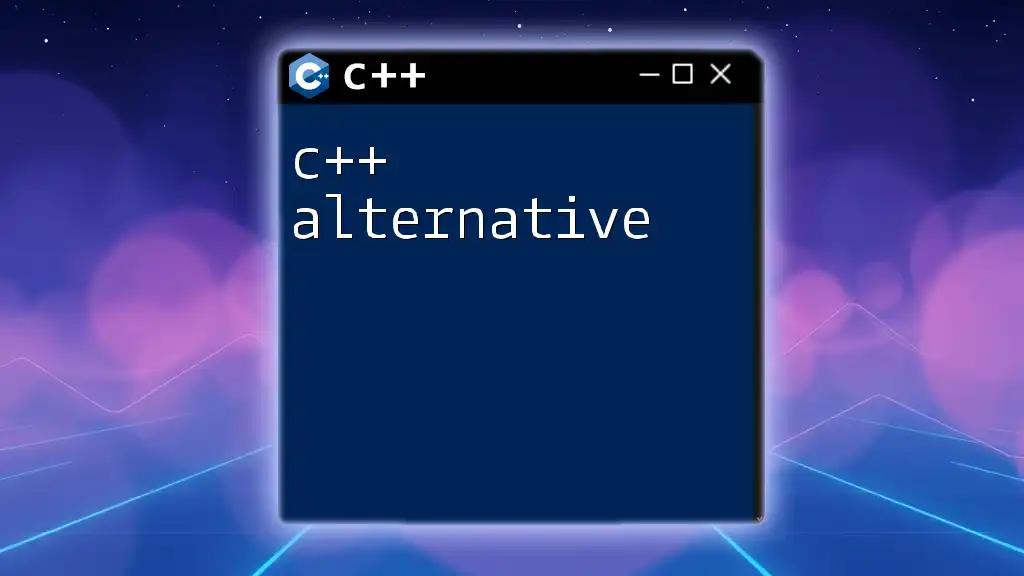
Additional Resources
For further learning, consider diving into blogs, online documentation, and tutorials focused on C++ and C interoperability. Explore tools and libraries that smooth out the development process between these languages, and don’t hesitate to engage with communities for support and shared knowledge. Your journey in mastering `extern "C"` will undoubtedly enhance your coding expertise!