In C++, `.h` files are used for declaring class interfaces and function prototypes, while `.cpp` files contain the implementation of those functions and methods.
Here's a simple example:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
class Example {
public:
void sayHello();
};
#endif // EXAMPLE_H
// example.cpp
#include <iostream>
#include "example.h"
void Example::sayHello() {
std::cout << "Hello, World!" << std::endl;
}
Understanding Header Files (.h)
What is a Header File?
A header file in C++ is a file that typically includes declarations for functions and classes. Header files often have a .h extension and serve as a way to inform different parts of a program about the definitions and relationships in your code. Essentially, header files tell the compiler what to expect when it comes to functionalities that may be implemented in other files.
Purpose of Header Files
One of the primary roles of header files is code declaration. By declaring functions, classes, and variables within a header file, you allow other source files to use these definitions without needing to understand the implementation details.
Facilitating Reusability is another crucial function of header files. By encapsulating declarations, you can include a single header file across multiple .cpp files, enabling code reuse and ensuring consistency in your program.
Structure of a Header File
A well-structured header file generally starts with include guards, follows with necessary includes, and contains declarations. Here’s an example of a simple header file:
// my_header.h
#ifndef MY_HEADER_H
#define MY_HEADER_H
void myFunction();
class MyClass {
public:
void myMethod();
};
#endif // MY_HEADER_H
In the example above, `#ifndef`, `#define`, and `#endif` are used as include guards to prevent multiple inclusions of the same header file, which can lead to compilation errors.
Include Guards
Include guards play a vital role in maintaining the integrity of your code. When multiple files include the same header without guards, the compiler encounters redefinitions, leading to confusion and errors. By employing include guards, you ensure that your header file is only processed once by the compiler, safeguarding against potential issues.
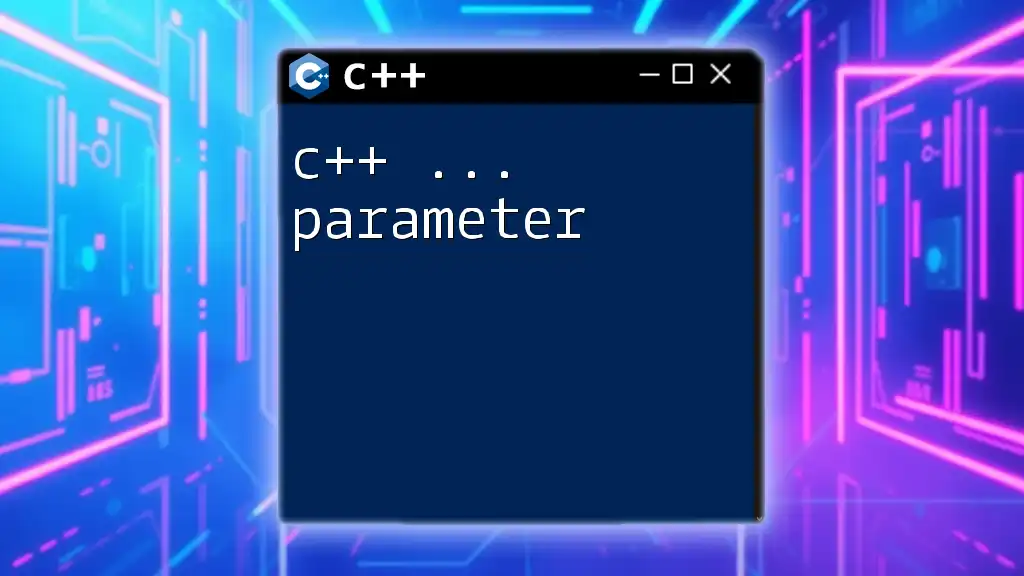
Understanding Source Files (.cpp)
What is a Source File?
A source file in C++ is where the actual implementation of declared functions occurs. These files have a .cpp extension and contain the logic that executes when a program runs. A source file connects to one or more header files, allowing for a full picture of the functionality your code provides.
Purpose of Source Files
The primary purpose of source files is to contain implementation details. In a well-organized program, each .cpp file generally implements the functionality for one or more related classes or functions declared in associated header files.
Separation of concerns is a key advantage of dividing declarations (in .h files) and implementations (in .cpp files). This separation enhances code maintainability, making it easier for multiple developers to work simultaneously without overlapping conflicts in declarations and implementations.
Structure of a Source File
The structure of a .cpp file is straightforward, starting with the inclusion of its corresponding header file, followed by the function and class implementations. Here’s an example of a simple source file:
// my_source.cpp
#include "my_header.h"
void myFunction() {
// function implementation
}
void MyClass::myMethod() {
// method implementation
}
Notice how the function `myFunction` and method `myMethod` directly implement the functionalities outlined in `my_header.h`.
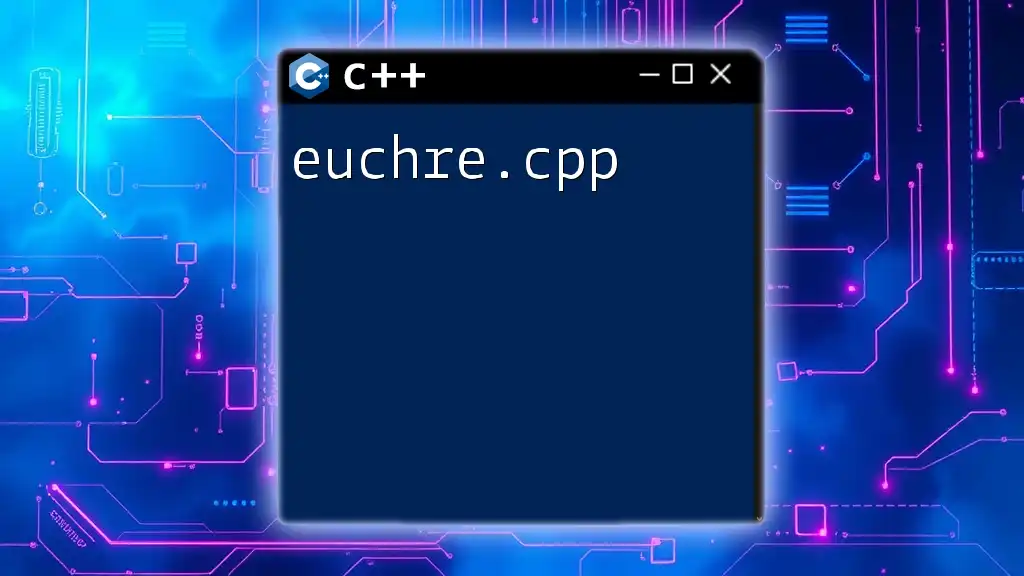
The Relationship Between .h and .cpp Files
Linking Header and Source Files
The relationship between .h and .cpp files is crucial in establishing a clear contract for the compiler. When a .cpp file includes a .h file (using `#include "my_header.h"`), it is assured of the existence of the corresponding declarations. This linkage is vital for compiling large applications where many pieces of code interrelate.
Compilation Process
Understanding the compilation process can help demystify how C++ processes .h and .cpp files. When you compile a C++ program, the compiler processes header files before implementing the source files. This allows the compiler to build a full picture of all declarations before delving into the details of their implementations.
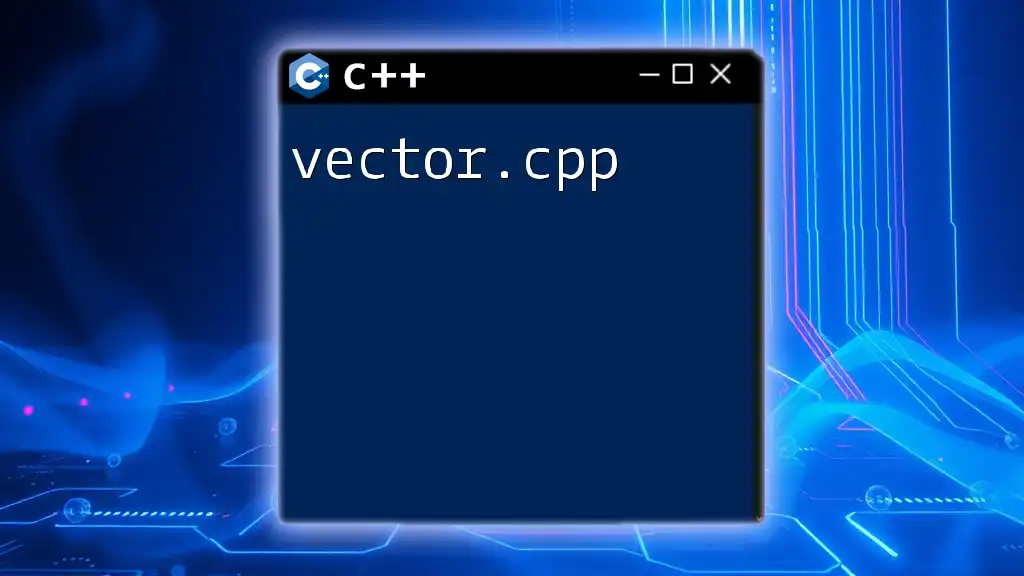
Best Practices for Using .h and .cpp Files
Organizing Your Code
Organizing your code effectively can significantly enhance clarity and maintainability, especially as projects grow. A good practice is to separate your header and source files into distinct folders like `/include` for headers and `/src` for source files.
You should also consider using namespaces and avoiding global variables, which can create conflicts and make debugging more complex.
When to Use Forward Declarations
Utilizing forward declarations can streamline your code, especially in situations where two classes reference each other. A forward declaration allows you to declare a class without fully defining it, which can help prevent circular dependencies. Here’s an example:
class MyClass; // Forward declaration
void myFunction(MyClass* obj); // Declares function using forward declaration
In this case, `MyClass` is declared so that `myFunction` can utilize pointers or references to it, while the full definition can be implemented later.
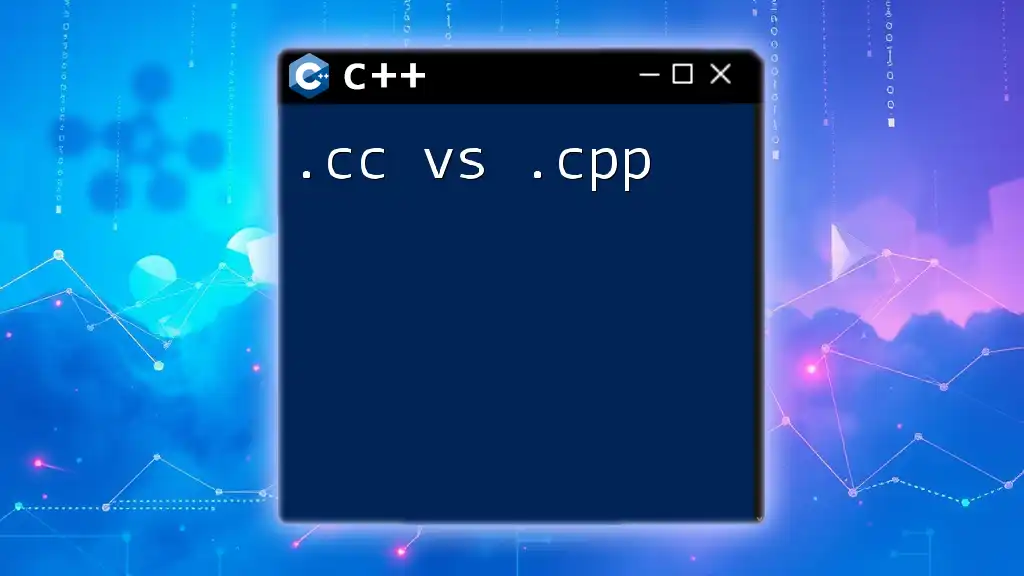
Common Mistakes and How to Avoid Them
Circular Dependencies
A common pitfall in C++ programming is the creation of circular dependencies. This can occur when two or more header files reference each other directly, leading to compilation errors. To avoid this, make use of forward declarations where necessary and carefully structure your includes.
Not Using Include Guards
Neglecting to implement include guards in your header files can create significant issues. Without them, you risk compiling the same declarations multiple times, resulting in redefinition errors. Always remember to include guards in every header file you write!
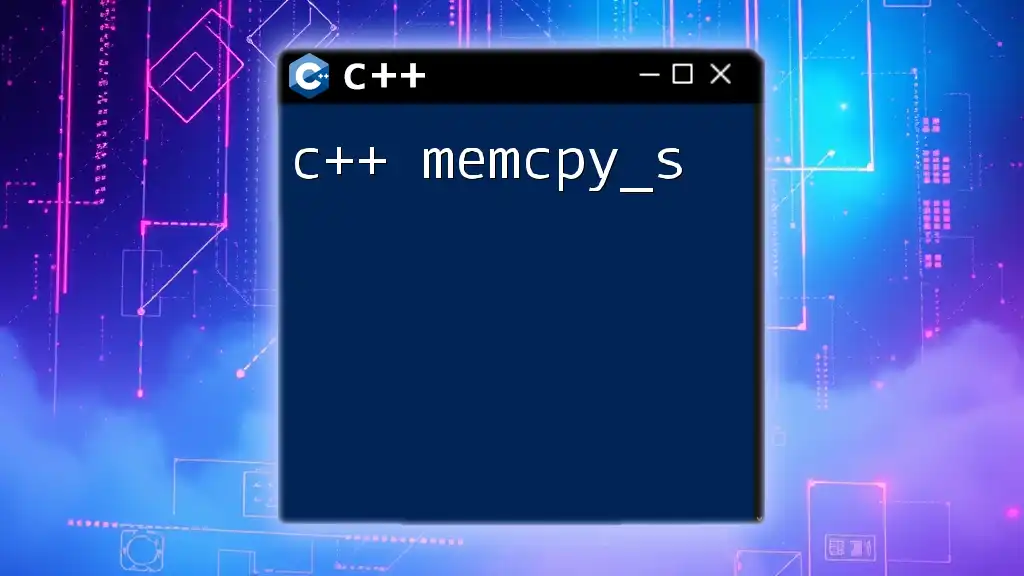
Conclusion
In summary, the use of .h and .cpp files is essential in C++ programming. They not only improve code organization but also enhance efficiency and maintainability. By adhering to best practices and understanding the relationships and purposes of these files, developers can create robust and scalable applications. Implementing these principles will significantly improve your coding journey and project collaboration in C++.
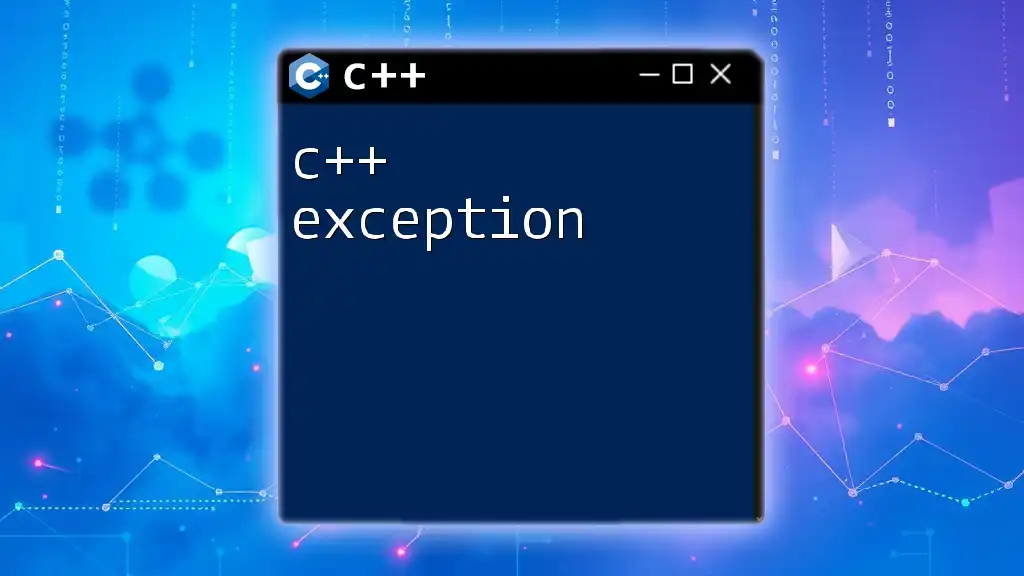
Additional Resources
For further reading and to deepen your understanding of .h and .cpp files, consider exploring tutorials and documentation specific to C++ file organization practices. These resources can provide valuable insights and help refine your coding skills.