Join our "CPP Boot Camp" to master essential C++ commands quickly and efficiently, empowering you to write clean and effective code in no time!
Here's a simple example to get you started with a "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What to Expect from a C++ Bootcamp
Structure of a Typical C++ Bootcamp
When embarking on a C++ Bootcamp, it’s important to understand the structure that will guide you through your learning journey. Typically, these bootcamps feature a combination of classroom sessions and hands-on coding practice. Most bootcamps follow a rigorous daily schedule that allows participants to immerse themselves in the learning process.
Participants can expect a variety of formats, including lectures, pair programming, and group discussions.
- Classroom Sessions: These focus on theoretical aspects, where instructors explain critical concepts like data structures, algorithms, and design patterns.
- Hands-on Coding Practice: Daily coding challenges and projects solidify understanding by applying learned concepts in real-time.
Duration varies from several weeks to a few months, providing options for full-time or part-time commitments based on individual needs. This flexibility allows learners to take ownership of their time and progress.
Outcomes and Skills Acquired
Upon completing a C++ Bootcamp, participants will acquire a broad range of programming competencies:
- Core Programming Competencies:
Understanding the basic syntax and semantics of C++, participants will learn about:
- Data Types and Variables: Grasping the fundamentals allows for effective resource management.
- Advanced Concepts: Bootcamps often cover crucial areas such as:
- Object-Oriented Programming (OOP): This principle is vital for structuring complex software and systems.
- Memory Management with Pointers: Understanding how to utilize pointers effectively is critical for performance optimization.

Getting Started with C++
Setting Up Your Development Environment
To dive into C++, you'll need to set up a suitable development environment. There are several options for IDEs and text editors. Visual Studio, Code::Blocks, and Eclipse IDE are among the most popular choices. For those preferring online solutions, Replit or JDoodle can be used to practice without installing software.
Installation Guide:
- Download the IDE of your choice.
- Follow the installation prompts for your operating system (Windows, macOS, or Linux).
- Set up your compiler, ensuring it’s compatible with the IDE.
Your First C++ Program: Hello World
Creating your first program is an essential milestone. Here’s a classic example of the "Hello, World!" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code snippet, `#include <iostream>` is crucial as it allows the program to utilize input and output streams. The directive `using namespace std;` simplifies the syntax by allowing access to the standard library without needing a prefix. The `main` function serves as the entry point, where the program execution begins, and `cout` outputs the text to the console.
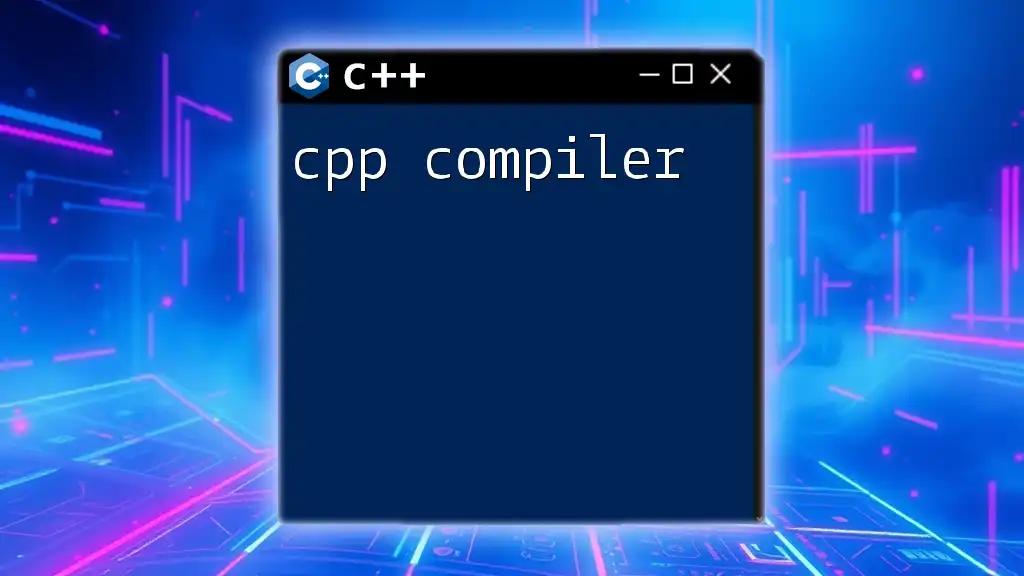
Key Topics Covered in C++ Bootcamp
Basics of C++ Syntax
For anyone new to programming, understanding the basics of C++ syntax is foundational.
- Variables and Data Types define how data is stored, such as integers, floats, and characters. Learning these ensures you know how to work appropriately with data in C++.
- Operators and Expressions are equally important. You will familiarize yourself with arithmetic, relational, and logical operators.
Control Structures
Control structures dictate the flow of your program.
- Conditionals: These allow programs to make decisions based on certain conditions. Here’s a simple example:
int age = 20;
if (age < 18) {
cout << "Minor" << endl;
} else {
cout << "Adult" << endl;
}
- Loops: Essential for executing blocks of code multiple times. You will learn about the different types of loops, including for, while, and do-while loops.
Functions in C++
Functions serve as reusable blocks of code that perform a specific task. Here’s a straightforward example of a function that adds two numbers:
int add(int a, int b) {
return a + b;
}
Understanding how to define, declare, and call functions is pivotal for efficient programming. You’ll also explore function overloading—a feature that improves code readability and reusability—along with default parameters that simplify function calls.
Object-Oriented Programming (OOP) in C++
OOP is a transformative paradigm for structuring code. Core concepts include:
- Classes and Objects: You will learn how to create blueprints (classes) from which objects are instantiated. This encapsulation allows data and functions to be bundled together logically.
- Inheritance and Polymorphism: These concepts enhance code efficiency and extensibility, enabling you to create a hierarchy of classes while allowing for dynamic method resolution.
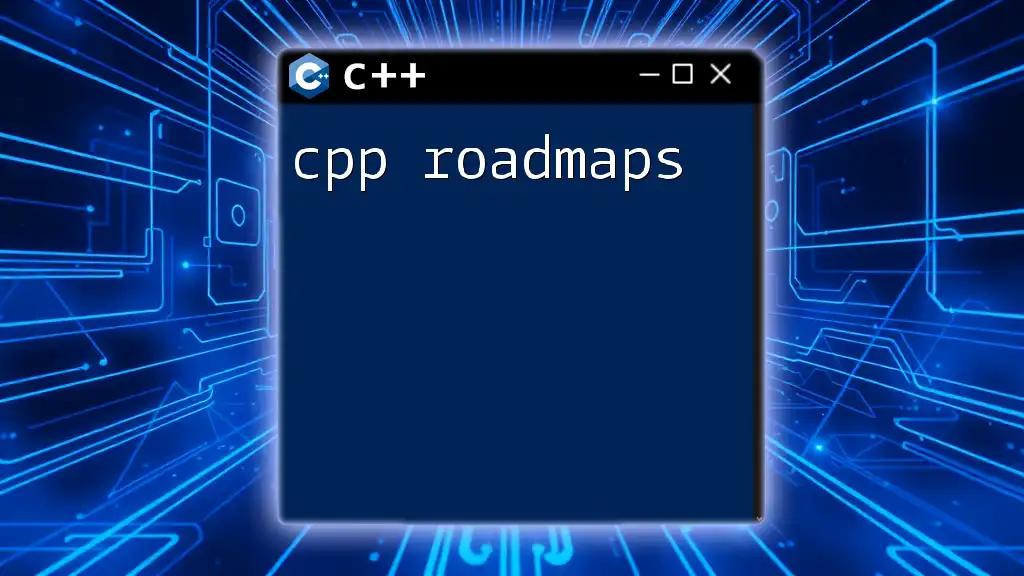
Advanced Topics and Best Practices
Memory Management in C++
One of the many powerful features of C++ is its capability to manage memory explicitly.
- Pointers are variables that store memory addresses, allowing for dynamic memory allocation using `new` and `delete` keywords. Here's how it's done:
int* ptr = new int; // Allocating memory
*ptr = 5; // Assign value
delete ptr; // Deallocating memory
Understanding memory management is essential for optimizing performance and preventing memory leaks, which can cause programs to run out of memory during execution.
Debugging and Testing Your Code
Debugging is an integral part of the programming process. You’ll learn techniques to identify and fix errors in your code, utilizing tools such as:
- Breakpoints and Watches: These allow you to pause execution and inspect variable states during runtime.
- Testing Strategies: You will explore various methodologies to ensure your code is functioning as intended through unit testing and integration testing.
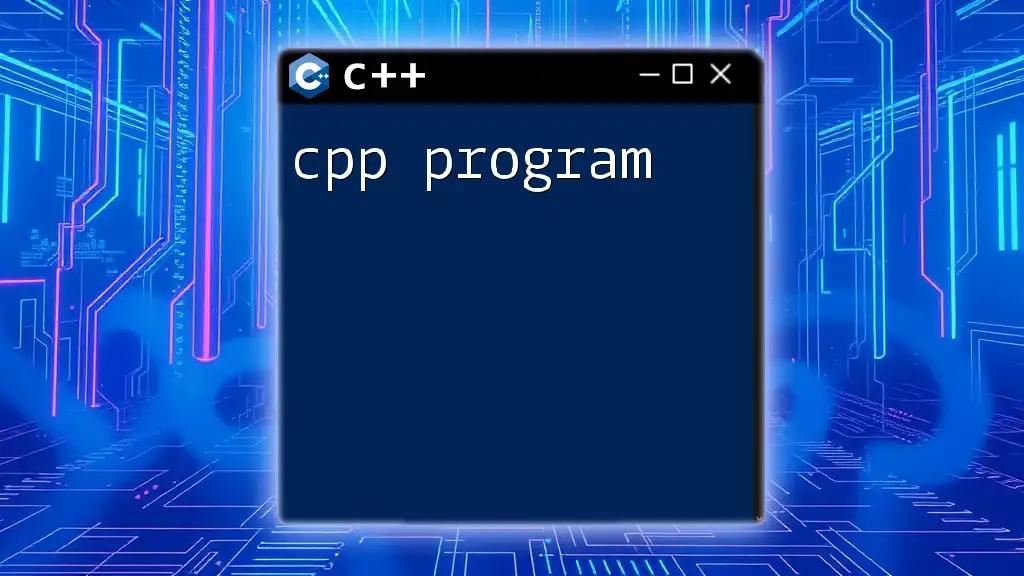
Real-World Applications of C++
C++ in Game Development
The gaming industry heavily relies on C++ for game development, particularly due to its performance. For example, Unreal Engine utilizes C++ to ensure high-frame rates and real-time graphics. By engaging participants in a hands-on project, bootcamps can illustrate how C++ powers the gaming world, providing a thrilling aspect to learning.
C++ in System Programming
Beyond games, C++ plays a crucial role in system programming. This includes tasks like operating systems, device drivers, and other software that interacts directly with hardware. Understanding the real-time application of C++ will prepare participants for various career paths.
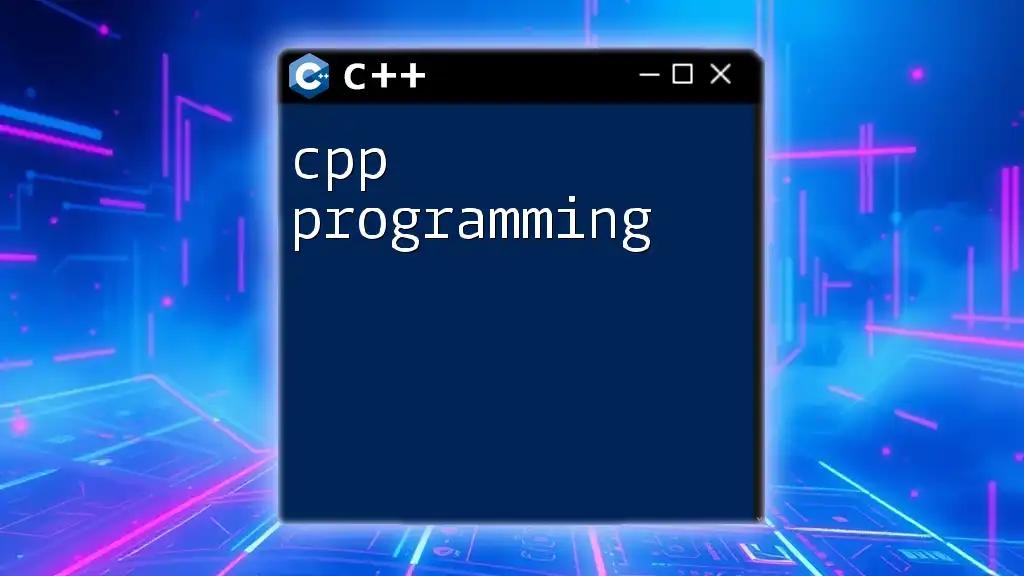
Conclusion
The journey through a C++ Bootcamp is one filled with rigorous training in both theoretical frameworks and hands-on coding experiences. Participants emerge with a robust understanding of C++ principles, ready to tackle real-world programming challenges. The skills acquired are not merely theoretical; they are equipped with practical knowledge that can be applied in various fields.
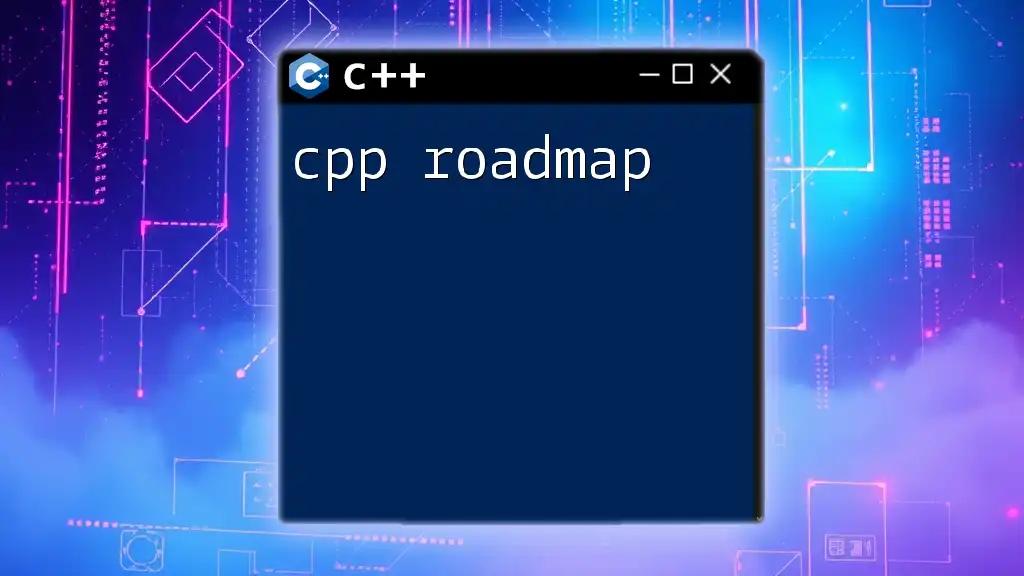
FAQ About C++ Bootcamp
Who is this Bootcamp for?
The C++ Bootcamp is designed for anyone interested in learning programming, whether you are a complete beginner or someone looking to solidify their existing knowledge.
What materials will be provided?
Participants can expect comprehensive course materials, including slides, code examples, and access to an online repository for practice.
Is there a certification?
Yes, successful graduates of the bootcamp receive a certification valued by employers, indicating proficiency in C++ programming.
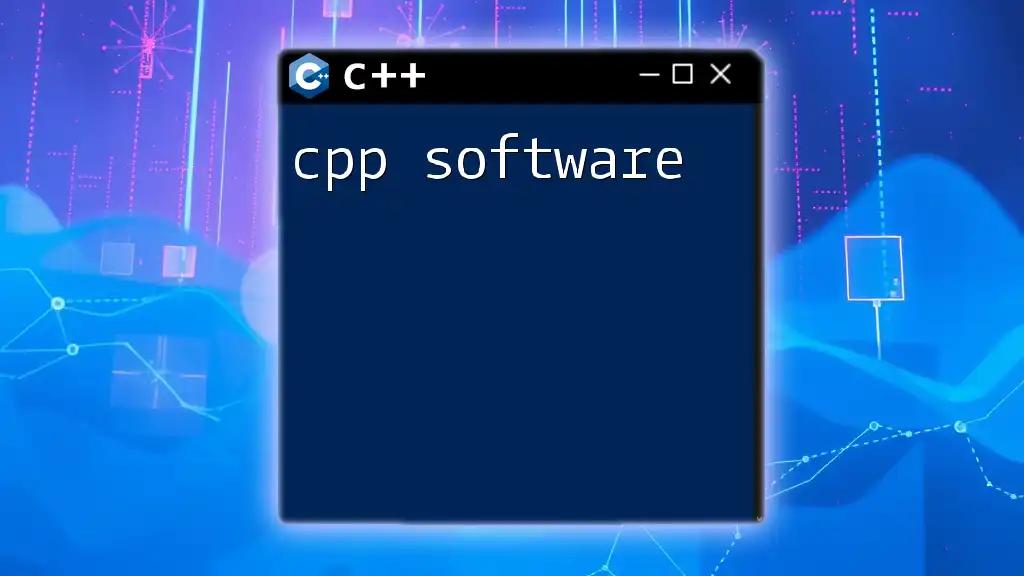
Call to Action
If you’re ready to start your journey into the world of C++ programming, join our C++ Bootcamp today. For inquiries and registration, feel free to contact us or check out our social media pages! Your coding adventure awaits!