The `#pragma pack` directive in C++ is used to control the alignment of data structures, allowing you to specify the byte alignment for members of structures, which can help reduce memory usage in certain situations.
Here’s a code snippet demonstrating its use:
#pragma pack(push, 1)
struct MyPackedStruct {
char a; // 1 byte
int b; // 4 bytes
short c; // 2 bytes
};
#pragma pack(pop)
int main() {
MyPackedStruct example;
// The total size of example is now 7 bytes instead of the default 12 bytes.
return 0;
}
What is `#pragma pack`?
`#pragma pack` is a preprocessor directive in C++ that controls the alignment of structure members in memory. Its primary purpose is to modify the default alignment behavior of the compiler, allowing developers to pack data structures more tightly. This technique can minimize memory consumption and improve compatibility with various data structures, such as network packets or binary file formats.
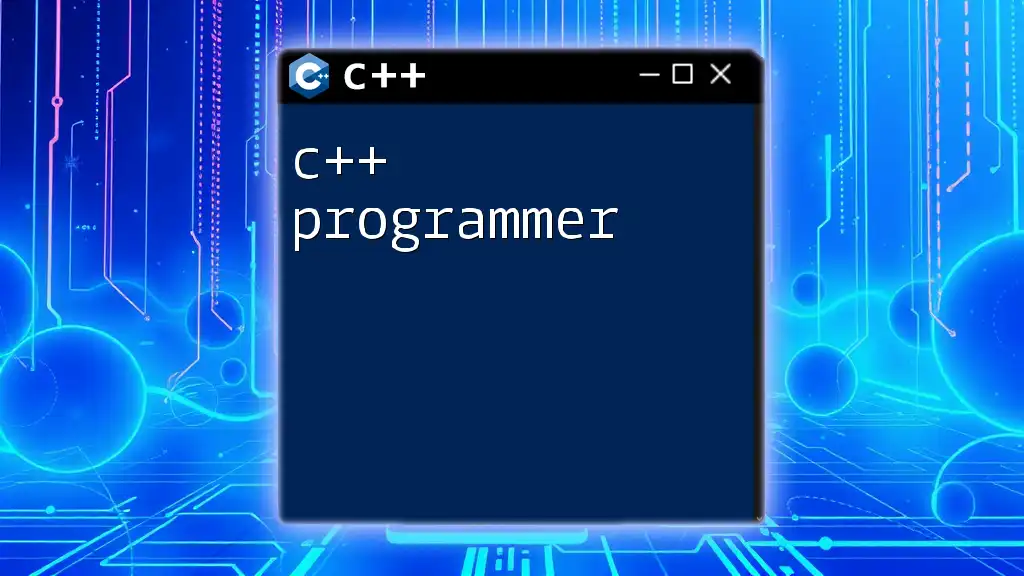
Importance of Alignment in Memory Management
Data alignment is crucial in programming, particularly in C++. It dictates how data is organized in memory and affects how quickly the CPU can access this data. Properly aligned data can lead to improved performance due to reduced CPU cycles spent accessing misaligned data. Thus, understanding how to use `#pragma pack` effectively can enhance both memory usage and performance.
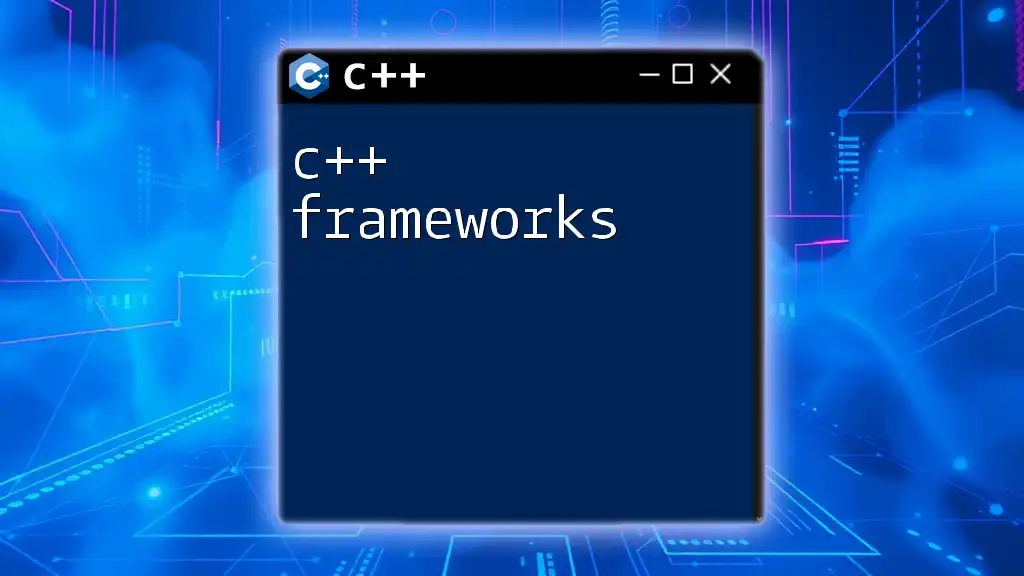
Understanding Data Alignment
What is Data Alignment?
Data alignment refers to the arrangement of data in memory according to specific byte boundaries. Most architectures prefer accessing data that is aligned to its size (e.g., 4-byte integers should start at addresses that are multiples of 4).
Memory Padding
To meet these alignment requirements, compilers often insert padding—extra bytes between data members to ensure they adhere to alignment rules. For instance, in a structure with an `int` (4 bytes) followed by a `char` (1 byte), the compiler may insert an extra 3 bytes of padding after the `char`, ensuring that the subsequent `int` starts at a properly aligned address.
The Impact of Misalignment
Misalignment can lead to performance degradation since accessing misaligned data often requires multiple memory accesses. Consequently, understanding alignment, padding, and how to manipulate these settings using `#pragma pack` is vital for optimal application performance.
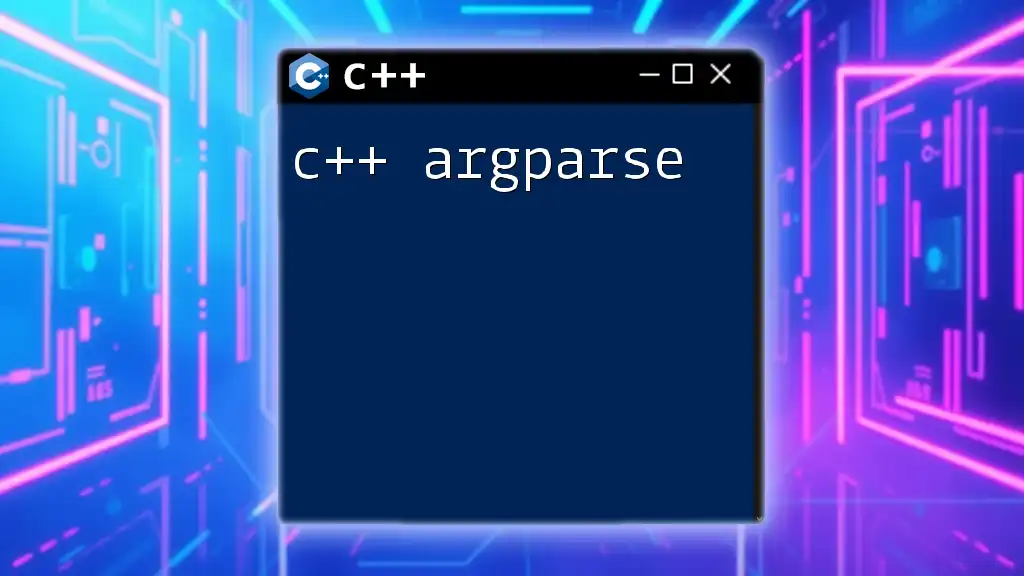
Using `#pragma pack`
What Does `#pragma pack` Do?
The `#pragma pack` directive allows developers to specify the alignment of structure members. When used, it overrides the compiler's default packing and can result in more tightly packed data structures, reducing their overall memory footprint.
Syntax of `#pragma pack`
The basic syntax of `#pragma pack` is straightforward. Here’s an example of a simple usage:
#pragma pack(push, 1)
struct MyStruct {
char a; // 1 byte
int b; // 4 bytes
};
#pragma pack(pop)
In this example, `#pragma pack(push, 1)` stores the current packing alignment and sets it to 1 byte, effectively eliminating any padding between `char a` and `int b`. The `#pragma pack(pop)` directive restores the previous alignment setting.
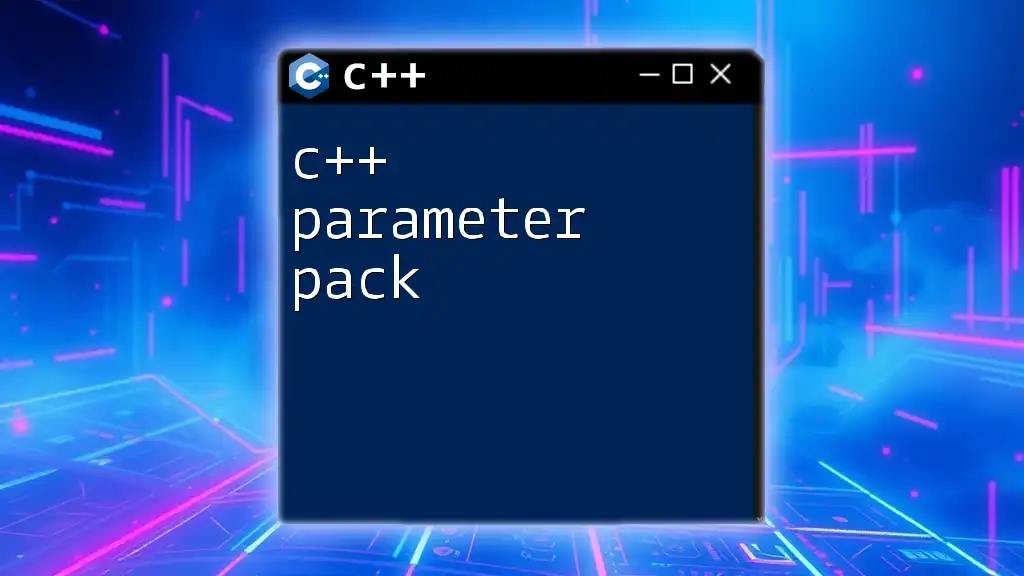
Customizing Structure Packing
Understanding the Pack Value
The value provided to `#pragma pack` (in this case, 1) determines the alignment boundary. A value of 1 means no padding, while values like 2 or 4 would enforce alignment on 2-byte or 4-byte boundaries, respectively.
Here’s a more detailed example:
#pragma pack(2) // Align structure members to 2-byte boundaries
struct AnotherStruct {
char x; // 1 byte
short y; // 2 bytes
int z; // 4 bytes
};
In this case, `AnotherStruct` is aligned to 2 bytes, meaning that there will be no padding after `char x`. However, an `int` typically has an alignment requirement of 4 bytes, potentially introducing padding before it.
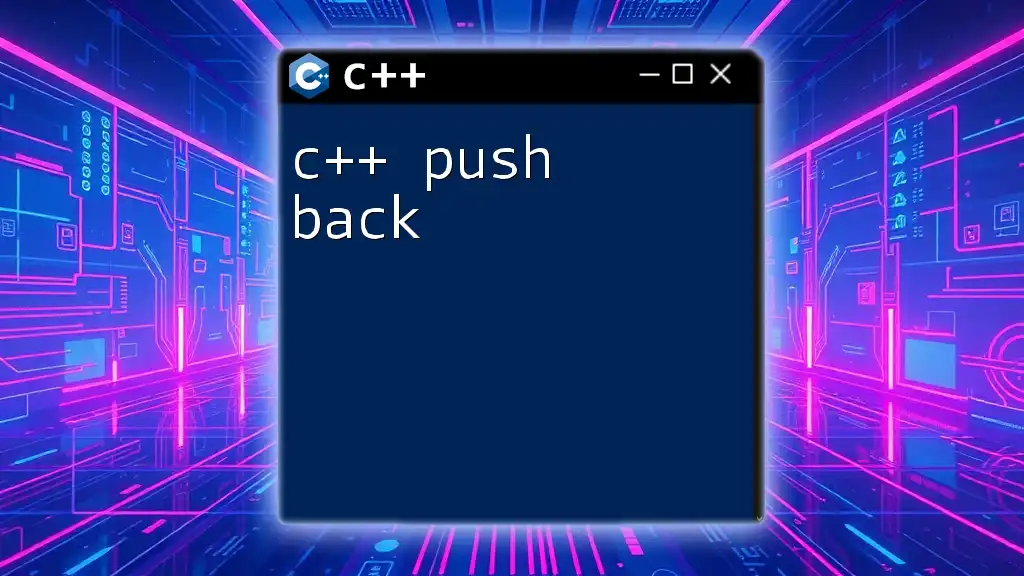
Stack and Heap Considerations
Implications on Stack Memory
When packing data structures, especially on the stack, developers should be aware that using `#pragma pack` may lead to misalignment in some cases, depending on the CPU architecture. This could lead to inefficient access and potentially raised exceptions in strict aligned environments.
Impact on Heap Allocations
Conversely, for heap-allocated structures, using `#pragma pack` generally offers more control over memory layout, enabling optimizations when serializing data or interfacing with hardware-oriented programming.
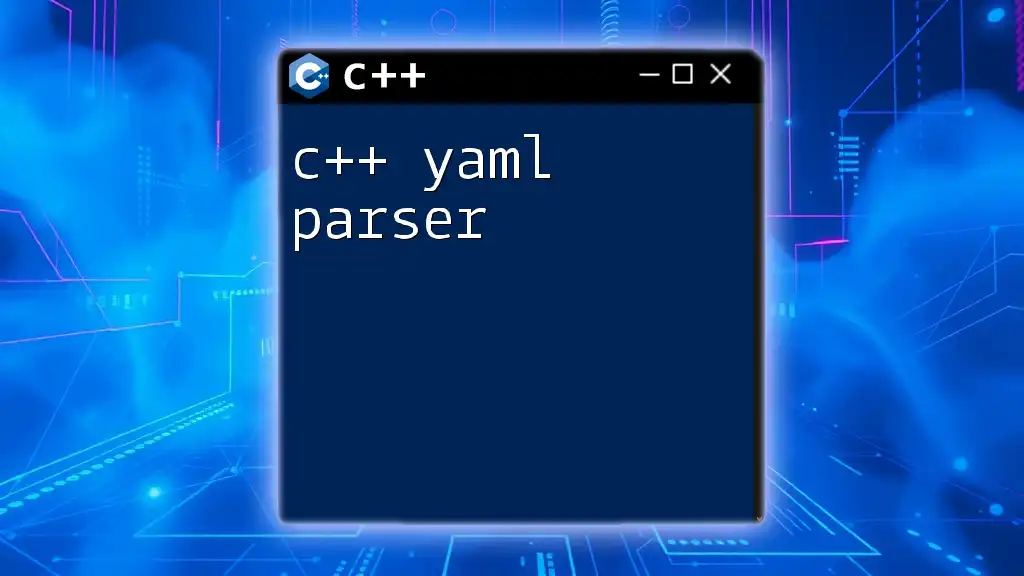
Advantages of Using `#pragma pack`
Memory Efficiency
One of the most significant advantages of using `#pragma pack` is its ability to reduce memory usage. This is especially vital in memory-constrained environments such as embedded systems or during heavy data transfers across networks.
Data Communication Protocols
Tightly packed structures are essential when working with certain communication protocols. For instance, when sending data over a network, aligning data perfectly with protocol standards helps avoid unexpected behavior or data corruption.
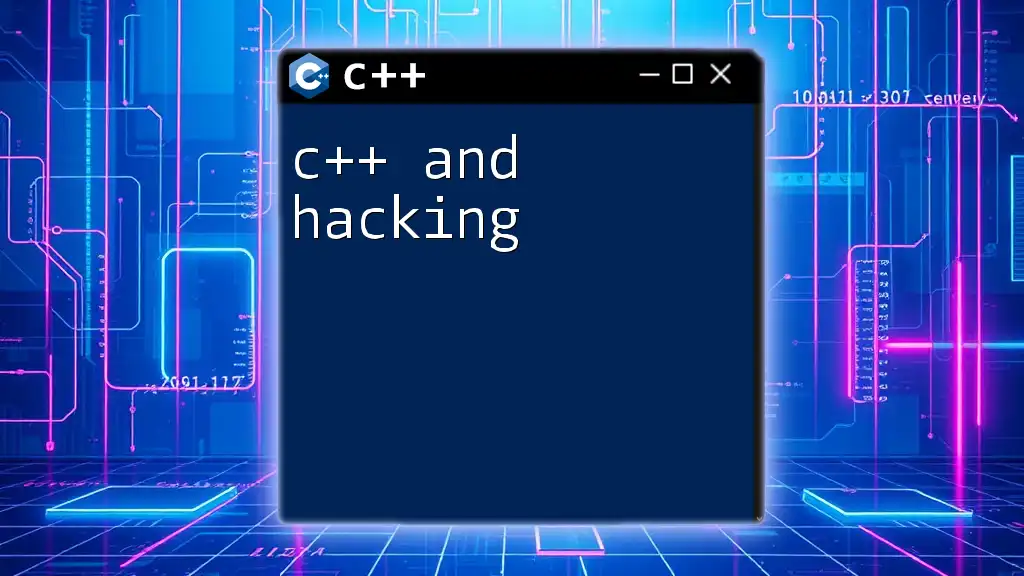
Potential Drawbacks
Performance Trade-offs
While packing structures can reduce memory consumption, it can also lead to performance trade-offs. Accessing packed data often requires additional CPU cycles. For example, a 4-byte integer that is packed tightly and misaligned may necessitate multiple memory accesses, slowing down performance.
Portability Issues
Additionally, compiler-dependent behavior can introduce portability issues. Different compilers may have different defaults for structure packing and alignment. Therefore, the use of `#pragma pack` should be documented carefully to ensure consistency across various platforms.
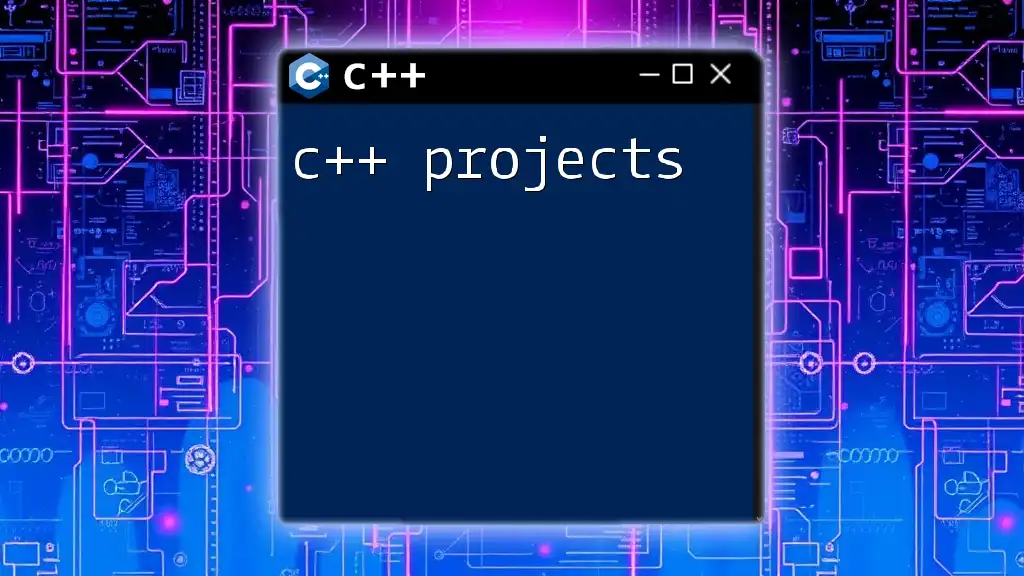
Best Practices for Using `#pragma pack`
Guidelines for Packing Structures
When implementing `#pragma pack`, follow these best practices:
- Use it judiciously, primarily when memory layout is critical (e.g., for file formats and network communications).
- Always encapsulate `#pragma pack` directives within structures or modules to limit their scope.
Using `#pragma pack` Responsibly
Avoid overpacking structures where performance is a priority. Instead, explore alternative designs, such as using smaller types or creating well-aligned structures to maximize performance without compromising memory efficiency.
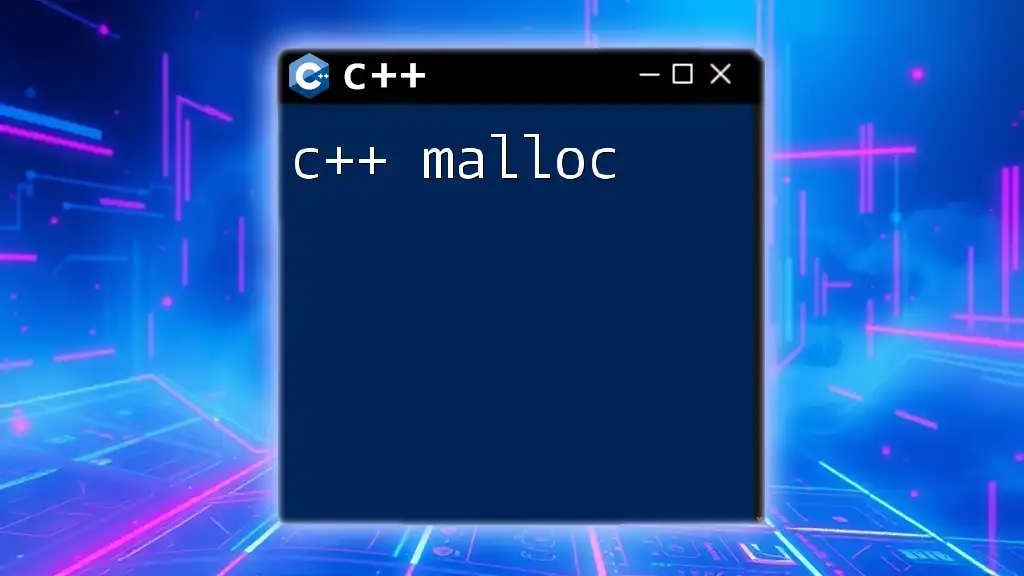
Code Examples from Real Projects
Example 1: Networking Application
In a networking application, packing data is crucial. Here’s a structure representing a network packet:
#pragma pack(push, 1)
struct Packet {
uint16_t header; // 2 bytes
uint32_t data; // 4 bytes
};
#pragma pack(pop)
This example demonstrates how tightly packed structures can be beneficial for defining protocols that require precise memory layout.
Example 2: File Format Handling
For handling file formats, using packed structures can ensure correct data extraction. Here’s an example of a file header structure:
#pragma pack(1)
struct FileHeader {
char signature[4]; // Signature for file identification
uint32_t fileSize; // Size of the file in bytes
};
Using `#pragma pack(1)` in this example guarantees that the header is read from a binary file exactly as expected.
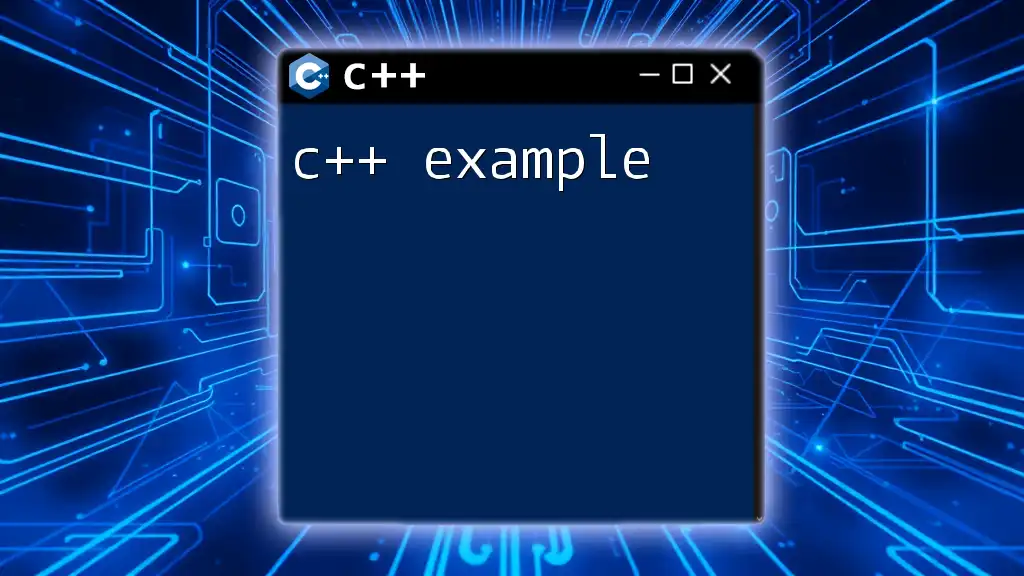
Conclusion
Utilizing `c++ pragma pack` efficiently can significantly enhance memory management and performance in specific scenarios. By understanding its implications on data alignment, padding, and structure packing, developers can create optimized applications suitable for various environments and requirements. Awareness of the associated trade-offs ensures responsible use, maintaining the balance between memory efficiency and performance.
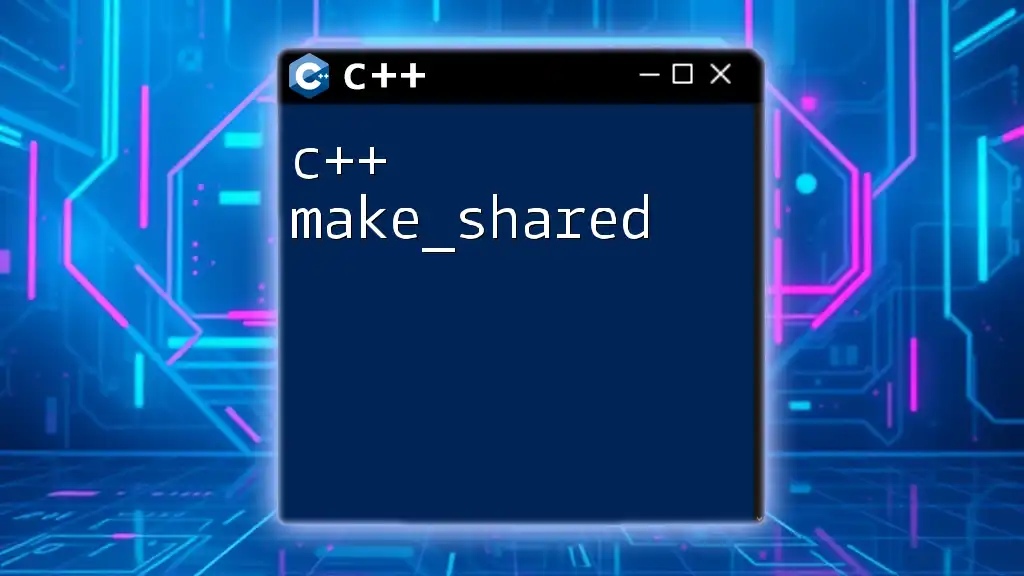
FAQ Section
What happens if I don’t use `#pragma pack`?
If you do not use `#pragma pack`, the compiler will apply its default alignment, which may introduce padding in data structures. This can lead to larger memory consumption and possibly affect the performance of data access, depending on how structures are used in your application.
Can I nest `#pragma pack` directives?
Yes, you can nest `#pragma pack` directives. However, it is essential to manage these properly using `push` and `pop` to avoid unintended consequences on your data structure layouts.
How does `#pragma pack` affect class inheritance?
When using `#pragma pack`, class inheritance can result in changes to the alignment requirements of member variables. It's crucial to test behavior, as the layout of base and derived classes may differ if `#pragma pack` is applied inconsistently.