The C++ Pimpl (Pointer to Implementation) idiom is a design pattern that helps to hide implementation details from the header file, reducing compilation dependencies and improving compile times.
Here’s a simple example of the Pimpl idiom:
// Header File: MyClass.h
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClassImpl; // Forward declaration
class MyClass {
public:
MyClass(); // Constructor
~MyClass(); // Destructor
void doSomething();
private:
MyClassImpl* pImpl; // Pointer to implementation
};
#endif // MYCLASS_H
// Implementation File: MyClass.cpp
#include "MyClass.h"
#include <iostream>
class MyClassImpl {
public:
void doSomethingImpl() {
std::cout << "Doing something!" << std::endl;
}
};
MyClass::MyClass() : pImpl(new MyClassImpl()) {}
MyClass::~MyClass() {
delete pImpl; // Clean up
}
void MyClass::doSomething() {
pImpl->doSomethingImpl();
}
What is the Pimpl Pattern?
The Pimpl pattern, short for “Pointer to Implementation,” is a widely used idiom in C++ programming designed to encapsulate the implementation details of a class, keeping them hidden from the class user. This separation is crucial for minimizing compile-time dependencies and enhancing program modularity.
By shielding the implementation of a class from the end user, the Pimpl pattern allows developers to change the internal workings without impacting the user interface. This leads to cleaner code management and greater flexibility when managing libraries.
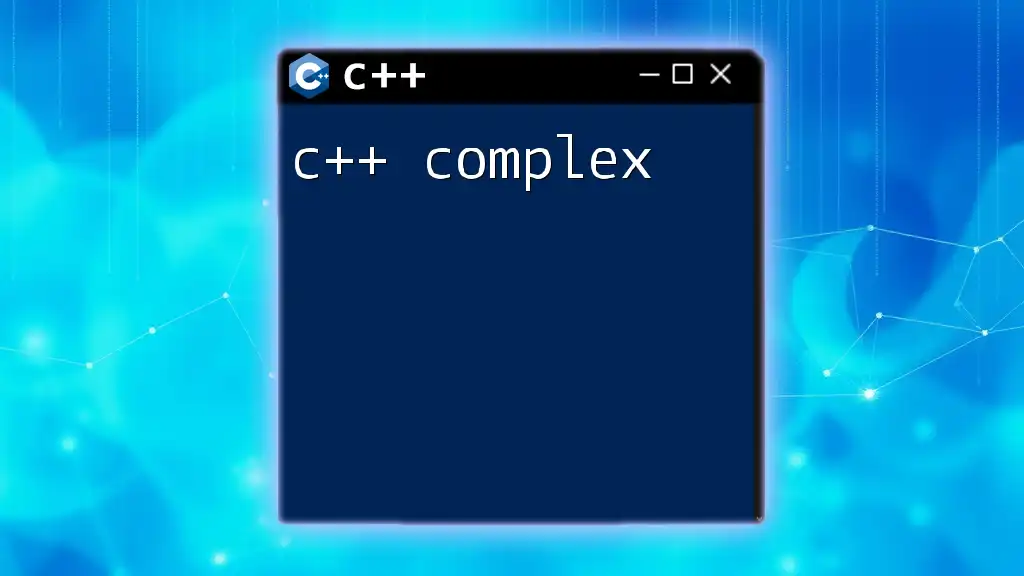
Why Use the Pimpl Pattern?
Utilizing the Pimpl pattern in C++ provides several significant advantages:
-
Reducing compilation dependencies: When implementation details are hidden in a separate source file, changes to these details do not require recompilation of the header file, which is beneficial when the project grows in size.
-
Encapsulating implementation details: By exposing only the public interface in the header file, developers prevent users from relying on the internal workings, allowing for easier adjustments.
-
Enhancing binary compatibility: Modifications in the underlying implementation do not necessitate recompilation of code that uses the class, as long as the interface remains unchanged. This is particularly useful in shared libraries.
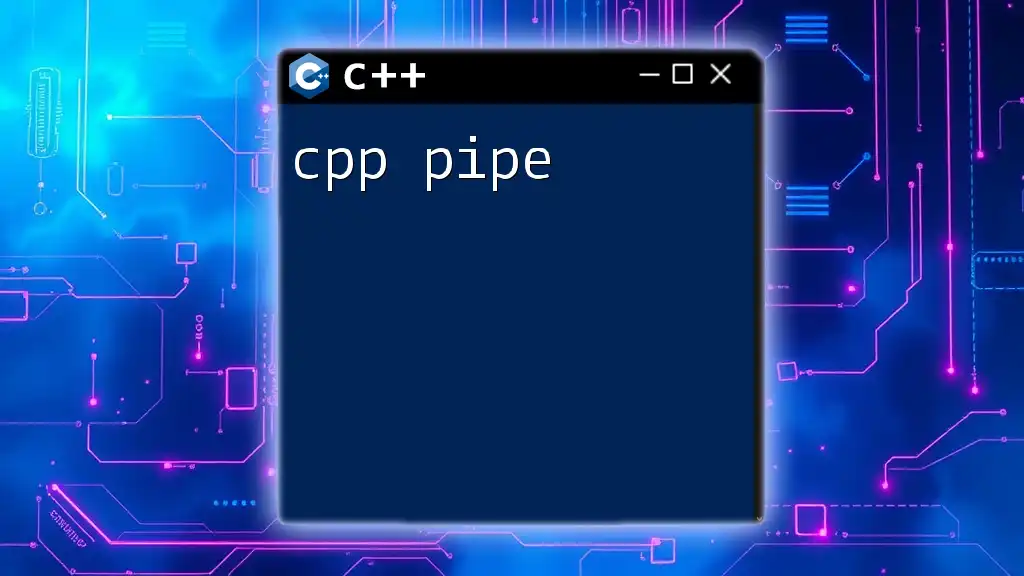
Understanding the Pimpl Pattern
Basic Concept of Pimpl
The essence of the Pimpl pattern revolves around the use of an internal implementation structure that is accessed via a pointer. Essentially, you have a public class with a private struct that contains all data and method implementations. This abstraction allows you to hide what’s occurring inside the class while keeping the interface clean.
When to Use the Pimpl Pattern
The best scenarios to implement the Pimpl pattern include:
-
Heavy header dependencies: If a class relies on many other headers or libraries, changes in any of those may cause a lengthy compilation process. Using Pimpl helps limit those dependencies.
-
Evolving interfaces: When developing libraries or APIs, it is common to change implementations without altering the exposed interface. Pimpl makes it easier to do so by decoupling implementation from the interface.
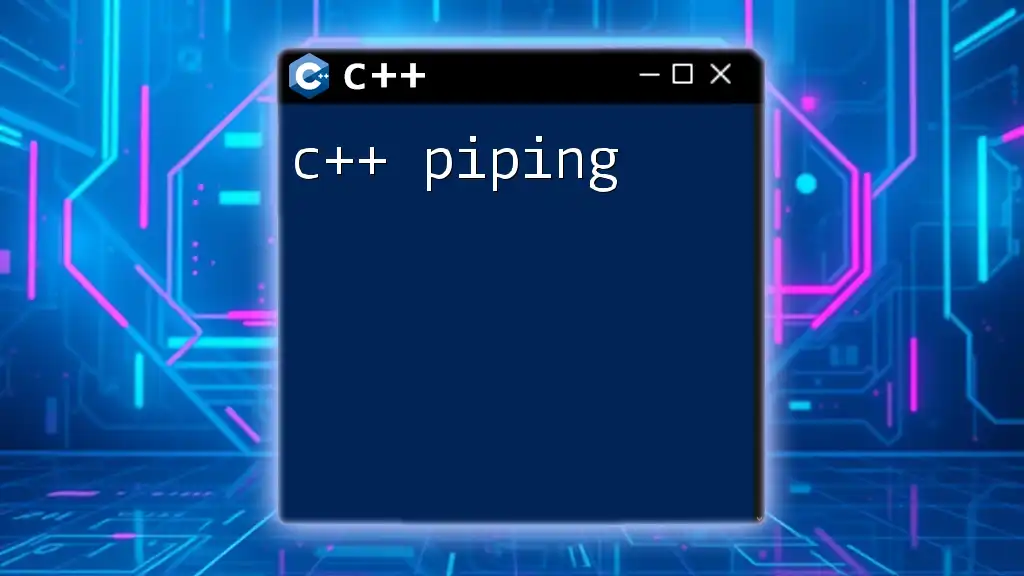
Implementing the Pimpl Pattern
Step-by-Step Guide to Implementing Pimpl
To implement the Pimpl pattern efficiently, you’ll want to follow a strategic approach:
First, create a public class header with a private implementation pointer. Here's an example:
class Widget {
public:
Widget();
~Widget();
void doSomething();
private:
struct Impl;
Impl* pImpl; // Pointer to the implementation
};
Creating the Implementation Class
Next, you define the actual implementation inside the structure that you've declared in the class. This is where the functionalities reside, away from the public interface:
struct Widget::Impl {
void doSomething() {
// Implementation details here
}
};
Constructor and Destructor Handling
In managing the lifecycle of the Pimpl pattern, proper handling of constructors and destructors is paramount. The constructor should allocate the implementation, and the destructor should clean it up.
Widget::Widget() : pImpl(new Impl()) { }
Widget::~Widget() {
delete pImpl;
}
Implementation of Member Functions
When a member function is called, it should delegate the operation to the implementation object. This essentially means forwarding calls to the internals residing within `Impl`.
void Widget::doSomething() {
pImpl->doSomething();
}
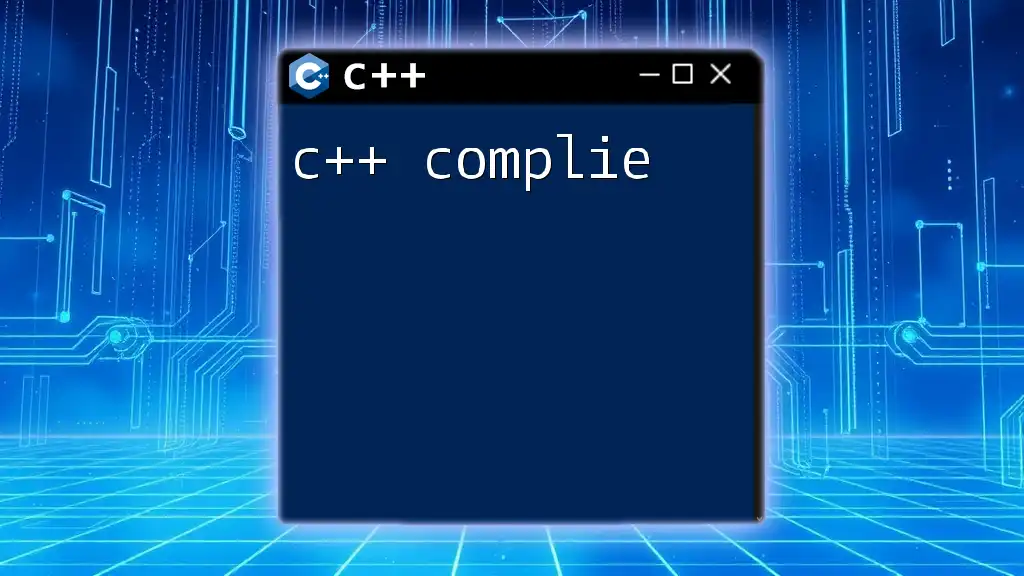
Detailed Examples of Pimpl in Action
Example 1: Simple Pimpl Implementation
Let’s look closely at a simple code example showcasing a basic Widget class using the Pimpl pattern:
class Widget {
public:
Widget();
~Widget();
void doSomething();
private:
struct Impl;
Impl* pImpl; // Pointer to the implementation
};
struct Widget::Impl {
void doSomething() {
// Implementation details here
}
};
Widget::Widget() : pImpl(new Impl()) {}
Widget::~Widget() { delete pImpl; }
void Widget::doSomething() { pImpl->doSomething(); }
This simple example illustrates how the implementation is entirely separate from the interface. Users of the `Widget` class interact with it through its public API without needing to know its inner workings.
Example 2: Complex Pimpl Implementation
In a more complex scenario, you might include additional data members and several functionalities. Here’s a more detailed example:
class AdvancedWidget {
public:
AdvancedWidget(int initialValue);
~AdvancedWidget();
void performAction();
private:
struct Impl;
Impl* pImpl;
};
struct AdvancedWidget::Impl {
int data;
void performAction() {
data *= 2; // Example implementation
// Implement further functionalities
}
};
AdvancedWidget::AdvancedWidget(int initialValue) : pImpl(new Impl()) {
pImpl->data = initialValue;
}
AdvancedWidget::~AdvancedWidget() {
delete pImpl;
}
void AdvancedWidget::performAction() {
pImpl->performAction();
}
In this example, `AdvancedWidget` maintains an integer value and doubles it upon calling `performAction()`. Again, the intricacies are well encapsulated away from the user.
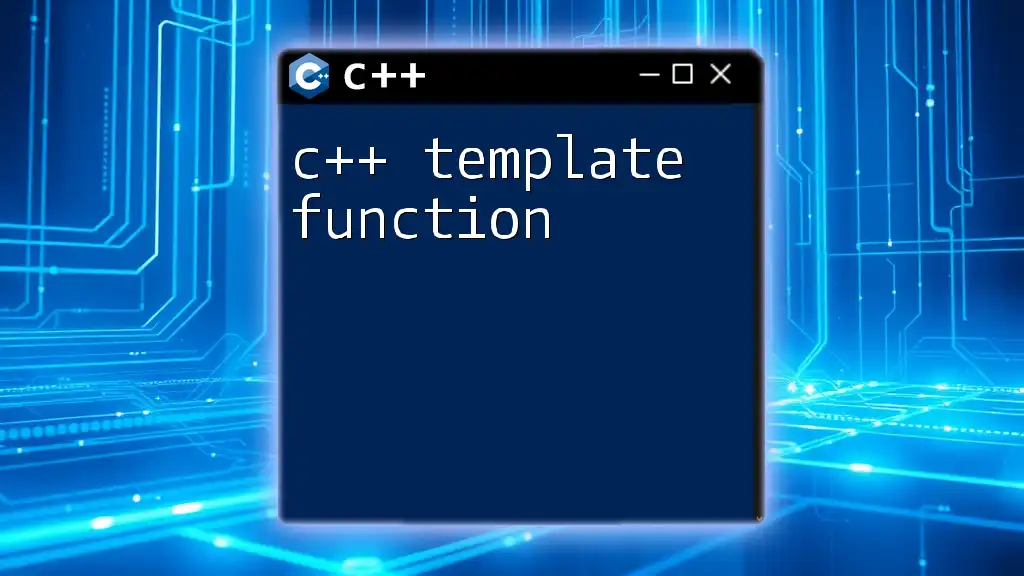
Advantages and Disadvantages of the Pimpl Pattern
Advantages of Pimpl
The Pimpl pattern brings about numerous benefits:
-
Clear separation of interface and implementation: It leads to more comprehensible code and easier maintenance.
-
Ease of modification: Changes in implementation do not propagate errors throughout the dependent code.
-
Reduction in compilation time: Compiling slower parts of the code becomes unnecessary when using Pimpl correctly.
Disadvantages of Pimpl
However, there are some drawbacks to consider:
-
Increased complexity: The code becomes more complicated due to the separation of the interface and implementation.
-
Performance overhead: Using raw pointers may introduce minor performance hits due to dynamic allocations.
-
Possible linker issues: If not managed properly, linking errors may arise if the implementation details change frequently.
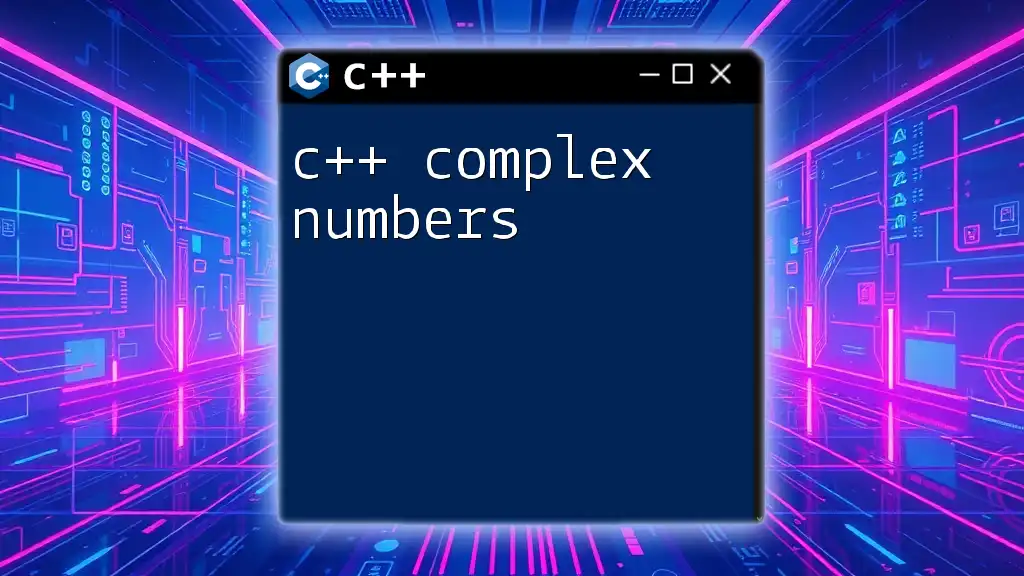
Best Practices for Using Pimpl in C++
Memory Management Considerations
To enhance memory management and reduce pitfalls associated with manual handling, consider using smart pointers as an alternative to raw pointers. This can help mitigate issues like memory leaks.
#include <memory>
class Widget {
public:
Widget();
~Widget();
void doSomething();
private:
struct Impl;
std::unique_ptr<Impl> pImpl; // Using smart pointer
};
Maintaining Header Files
To further optimize your code structure, ensure header files are organized effectively. This aids in improving readability and understanding, streamlining the coding experience.
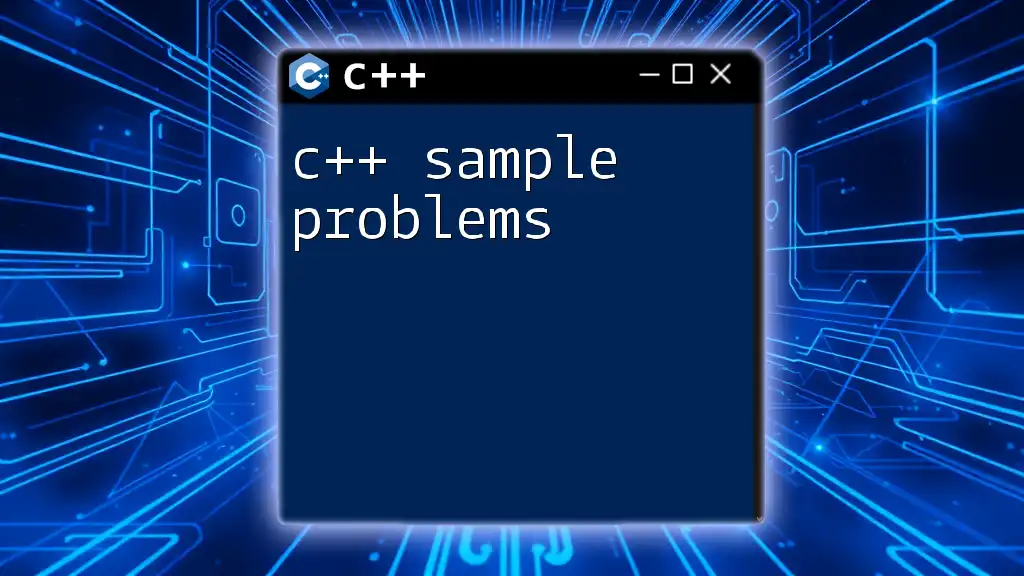
Conclusion
The Pimpl pattern is a powerful technique in C++ that facilitates clear separation between a class’s interface and its implementation. By minimizing dependencies and allowing for greater flexibility, it serves as a valuable strategy for managing complex systems. As C++ evolves with modern practices and tools, integrating Pimpl into your coding repertoire will continue to yield significant benefits for both developers and their users.
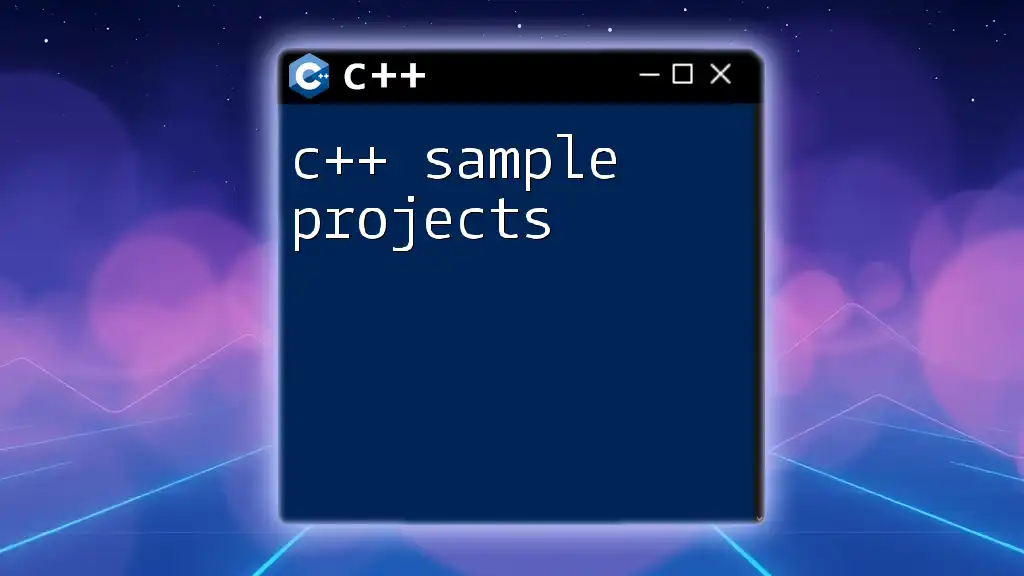
Additional Resources
For ongoing learning, consider exploring books and articles dedicated to advanced C++ programming. Engaging with online forums and communities can also provide additional insights and updates to keep your knowledge fresh and relevant.