In C++, the `push_back` function is used to add an element to the end of a `std::vector`, dynamically increasing its size.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(5);
numbers.push_back(10);
for (int num : numbers) {
std::cout << num << " ";
} // Output: 5 10
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a dynamic array that can grow and shrink in size, making it one of the most versatile data structures provided by the C++ Standard Template Library (STL). Unlike traditional arrays, vectors can change their size automatically, which is particularly useful when the number of elements is not known at compile time. Vectors also offer powerful features like random access, iteration, and memory management.
Importance of Vectors in C++
Vectors play a crucial role in C++ programming due to their dynamic memory allocation capabilities. They allow developers to manage collections of data without explicitly handling memory allocation and deallocation. This flexibility makes vectors ideal for a wide range of applications, from storing lists of items to managing dynamic datasets.
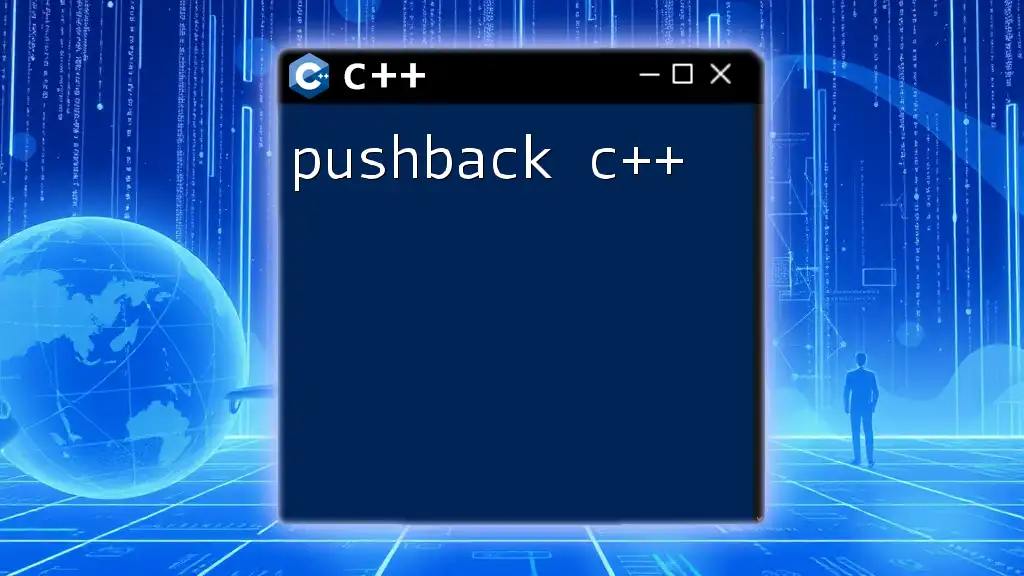
The `push_back` Method
What is `push_back`?
The `push_back` method is a member function of the C++ vector class, designed to add an element to the end of a vector. This command effectively increases the size of the vector by one, enabling you to append values dynamically as your program runs.
Syntax of `push_back`
The syntax for using `push_back` is straightforward:
vector_name.push_back(value);
In this command, `vector_name` is the name of your vector, and `value` is the element you want to add.
Functionality of `push_back`
When you invoke `push_back`, the vector's internal array may need to be resized if its current capacity is exceeded. This reallocation involves allocating a new larger array, copying existing elements to this new array, and then deleting the old array. This mechanism ensures that vectors can manage their elements efficiently while still allowing for rapid additions.
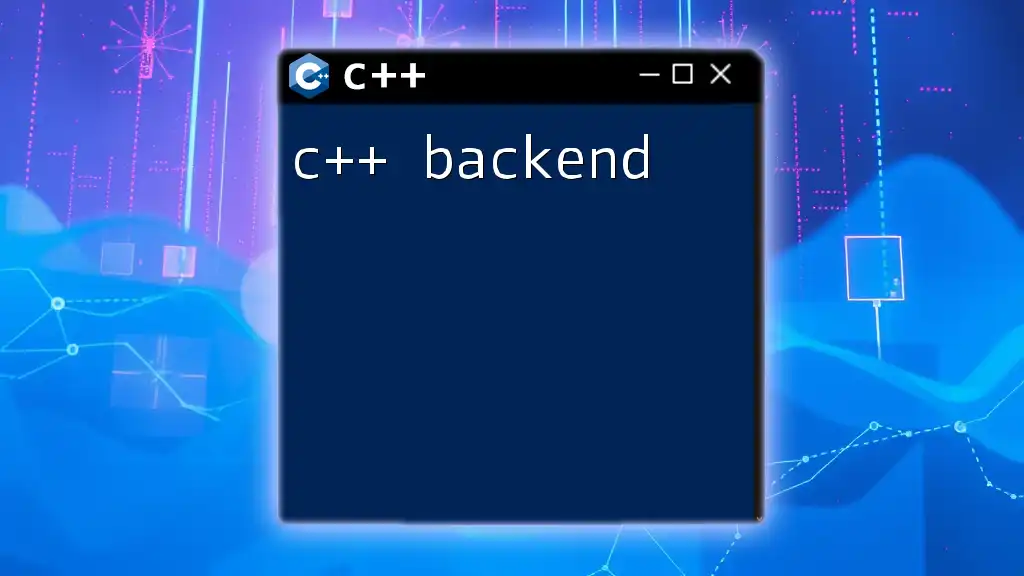
Detailed Usage of `push_back`
Adding Elements to a Vector
To demonstrate how to use the `push_back` method, consider the following example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10);
numbers.push_back(20);
std::cout << "Vector contents: ";
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, we initialize a vector called `numbers`. By using `push_back(10)` and `push_back(20)`, we add two integers to the vector. The loop then prints the contents of the vector—outputting `10` and `20`.
Pushing Back Different Data Types
You can also use `push_back` to add different data types to vectors. Consider this example with strings:
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> fruits;
fruits.push_back("Apple");
fruits.push_back("Banana");
fruits.push_back("Cherry");
std::cout << "Fruits vector contains: ";
for (const auto& fruit : fruits) {
std::cout << fruit << " ";
}
return 0;
}
Here, we demonstrate that `push_back` can effectively handle different data types, like `std::string`. After adding three fruit names, the program prints the contents of the vector, showcasing its versatility.
Capacity and Size Management
Understanding Vector Capacity
When managing vectors, it is essential to recognize the difference between size and capacity. The size refers to the number of elements currently stored in the vector, while the capacity is the amount of space allocated to the vector at any given time. When you call `push_back`, if the current size equals the capacity, the vector reallocates memory to accommodate the new element.
Example of Capacity Changes
The following example demonstrates capacity changes as elements are added:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
std::cout << "Initial capacity: " << numbers.capacity() << std::endl;
for (int i = 0; i < 100; i++) {
numbers.push_back(i);
std::cout << "Capacity after push_back: " << numbers.capacity() << std::endl;
}
return 0;
}
As you run this code, you'll observe that the capacity changes as elements are added. Initially, the capacity is 0, but it increases dynamically to accommodate additional integer values. This showcases the power of the `push_back` method in C++ vectors.
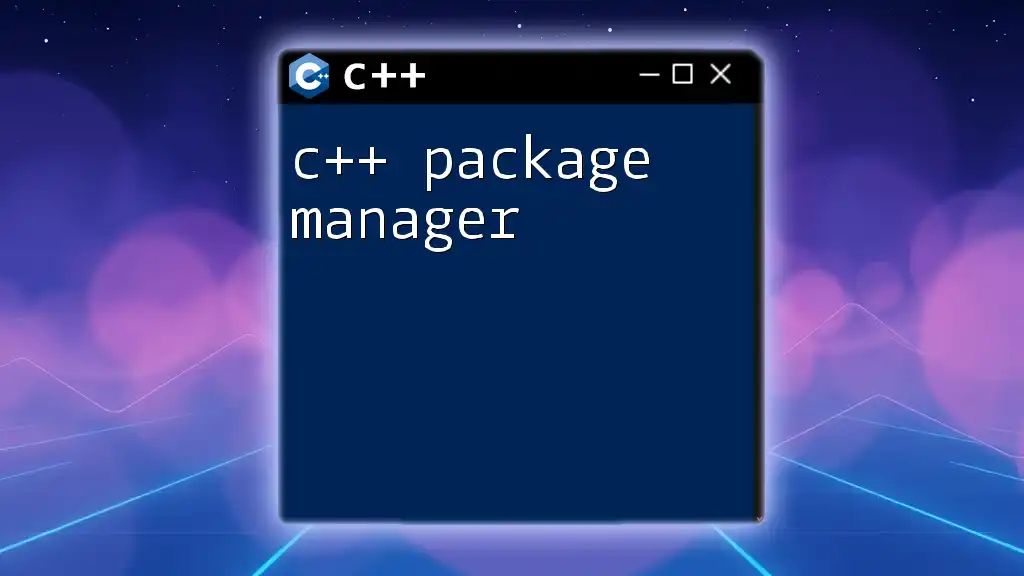
Common Mistakes and Best Practices
Common Mistakes with `push_back`
One common mistake developers make when using `push_back` is forgetting to include the necessary headers. Always ensure you have `#include <vector>` at the top of your code or your program will fail to compile. Another mistake is trying to push back elements into an uninitialized vector, which can lead to unexpected behavior.
Best Practices for Using `push_back`
To optimize vector usage and performance, consider using the `reserve` method to allocate memory ahead of time. By reserving space for your vector, you can reduce reallocations:
numbers.reserve(100);
This line pre-allocates memory for 100 integers, making subsequent `push_back` operations more efficient. Additionally, it's essential to understand when to use `push_back` compared to other methods such as `insert`, which can be useful for adding elements at specific positions.
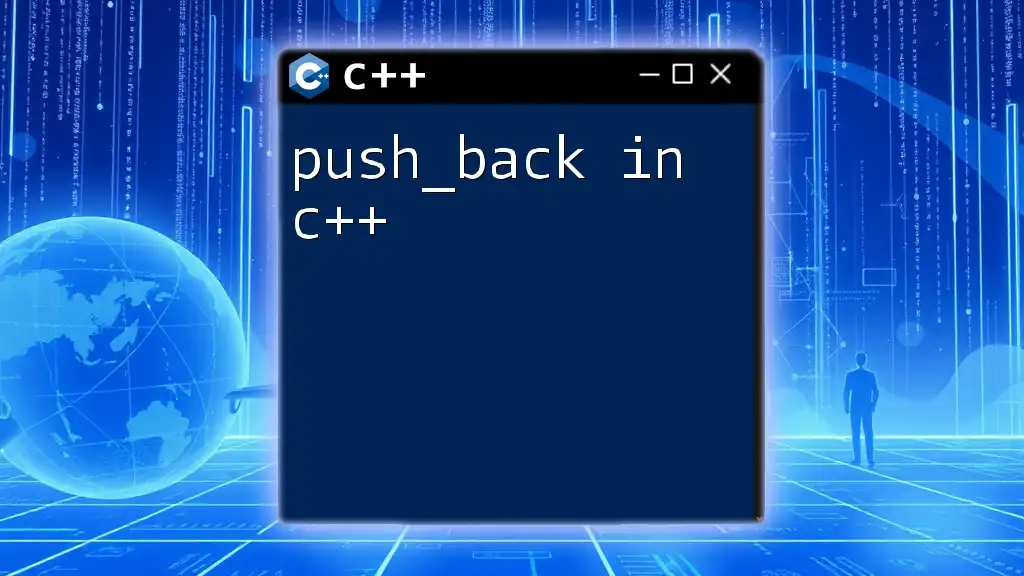
Conclusion
The `push_back` method is a powerful and essential tool for managing data in C++ vectors. By mastering this function, developers can efficiently handle dynamic datasets while leveraging the strengths of the C++ Standard Template Library. Practicing the implementation of `push_back` in various scenarios will solidify your understanding and enhance your programming skills.
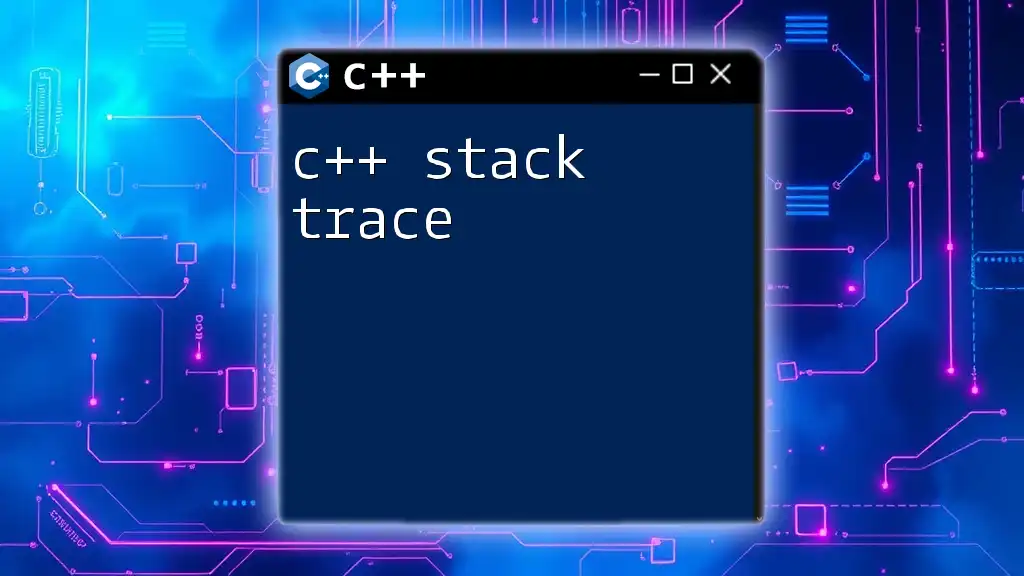
Additional Resources
For further learning, consult the official C++ documentation for more detailed explanations of STL components, or explore additional resources on vectors and other STL containers to deepen your knowledge.
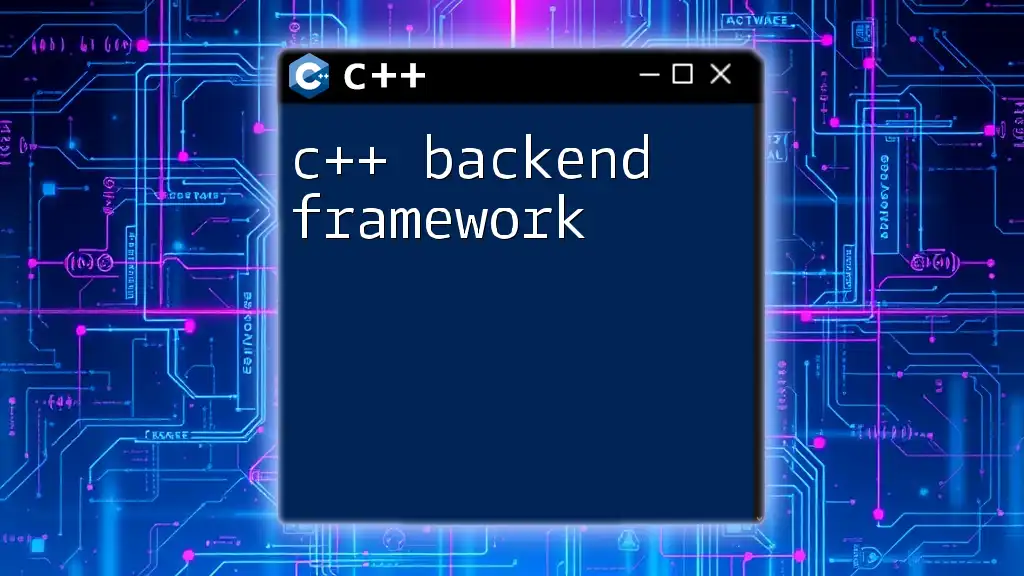
FAQs
What happens if you push back on a full vector?
If you attempt to `push_back` an element when the vector's capacity is full, the vector automatically reallocates memory. This involves creating a new array, copying current elements to it, and disposing of the old array. This process ensures that your vector can always accept new elements.
Can I use `push_back` on other STL containers?
While `push_back` is primarily associated with vectors and deques in C++, other containers like lists and arrays do not use this method. Each STL container has its own methods for adding elements, so it's essential to consult the documentation for specifics of each container.