C++ can be challenging due to its complex syntax, extensive feature set, and the need for manual memory management, which can lead to subtle bugs and undefined behavior if not handled correctly.
Here is a simple code snippet demonstrating memory management that can lead to issues if not used properly:
#include <iostream>
int main() {
int* ptr = new int(10); // Dynamically allocated memory
std::cout << *ptr << std::endl; // Accessing the value
delete ptr; // Deleting the allocated memory
// Forgetting to delete can cause memory leaks
return 0;
}
Understanding C++ Complexity
The Dual Paradigm of Programming
C++ embodies a dual programming paradigm: procedural programming and object-oriented programming (OOP). This multifaceted nature is both a strength and a source of confusion for newcomers.
Procedural programming focuses on writing procedures or functions that operate on data, promoting a linear approach to problem-solving. On the other hand, object-oriented programming centers around objects that combine data and functions, allowing for more complex and flexible designs.
While both paradigms have their merits, navigating between them can pose challenges. For instance, consider the following code snippet that demonstrates a simple method of a class in OOP:
class Rectangle {
public:
int width, height;
int area() {
return width * height;
}
};
In contrast, a procedural approach would look like this:
int area(int width, int height) {
return width * height;
}
From the simplicity of procedure calls to the encapsulation of data in classes, the effort required to grasp such complexity can leave many learners feeling overwhelmed.
The Rich Feature Set
C++ boasts an extensive Standard Library (STL) that offers a plethora of pre-built functions and data structures, including vectors, lists, and algorithms. While this vast library can enhance productivity, it can also be daunting. With so many options available, beginners may feel lost trying to identify which tools they need for their projects.
Additionally, C++ features many advanced concepts like templates, exceptions, and operator overloads. Each of these adds significant power but increases the learning curve dramatically. For example, consider using templates:
template <typename T>
T maximum(T a, T b) {
return (a > b) ? a : b;
}
Here, the templated function offers flexibility, but new learners might struggle to grasp how templates actually operate.
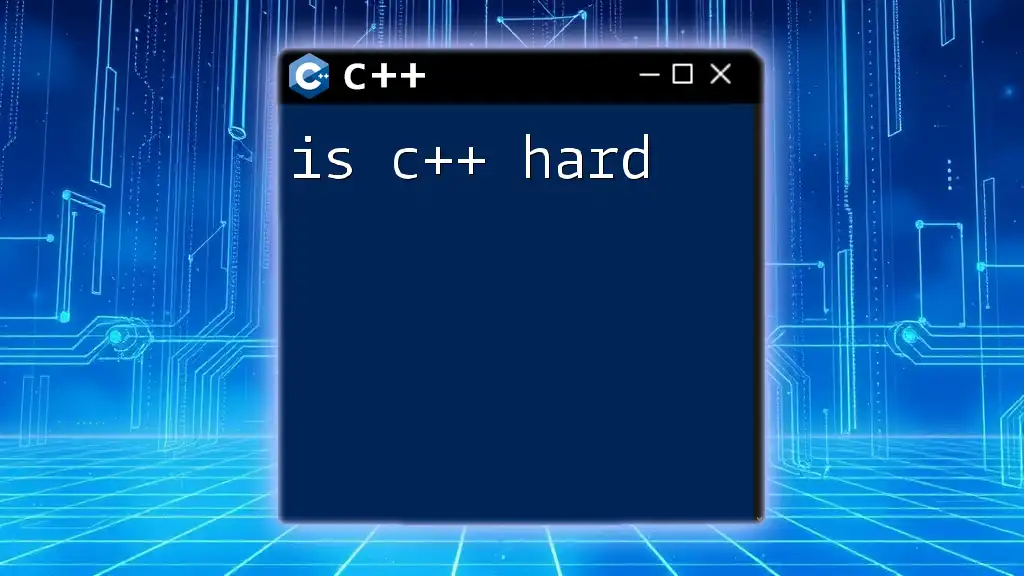
C++ Syntax and Semantics
Language Complexity
C++ syntax can be seen as verbose compared to other languages, such as Python. For example, variable declarations in C++ require explicit types:
int number = 5;
In contrast, Python allows for dynamic typing:
number = 5
This difference not only makes C++ more complex but also may contribute to frustrations among learners accustomed to more straightforward syntax.
Memory Management
One of the defining features that make C++ hard is manual memory management. Unlike languages with automatic garbage collection, C++ requires developers to manage memory manually, leading to possible memory leaks and dangling pointers.
Here's an example of dynamic memory allocation in C++:
int* arr = new int[10]; // Allocating memory
//... (use arr)
delete[] arr; // Freeing memory
While this gives programmers greater control over memory usage, it can lead to major pitfalls if not handled correctly. For instance, failing to call `delete` eventually results in memory leaks, leading to wasted resources and potential application slowdowns.
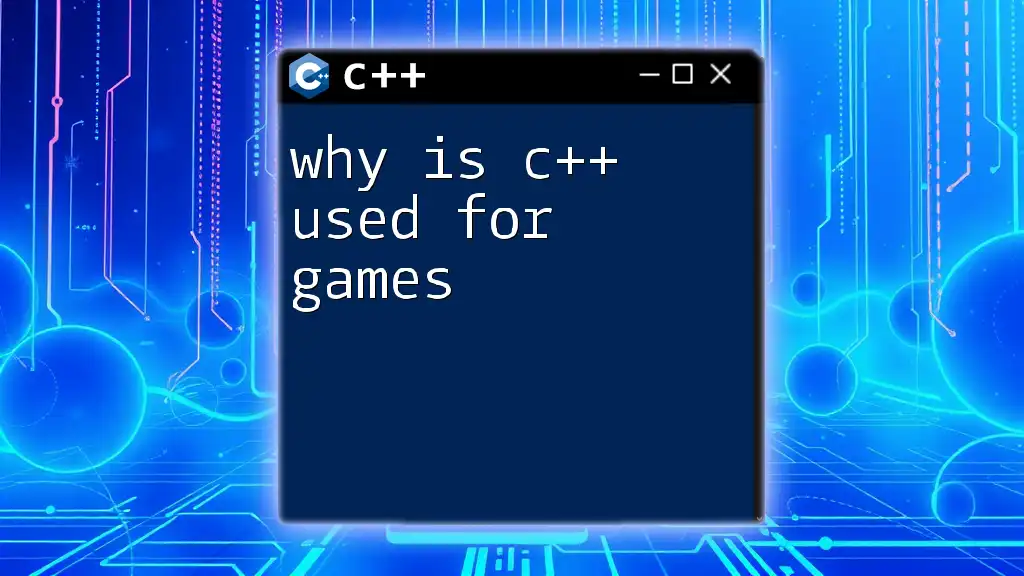
Advanced Concepts
Templates and Meta-programming
Templates allow for the creation of generic and reusable code, enabling developers to write functions and classes that work with any data type. However, for learners, understanding templates and meta-programming can be particularly challenging, as they require a deep understanding of both C++ syntax and the underlying logic.
Consider a templated function:
template <typename T>
void printArray(T arr[], int size) {
for (int i = 0; i < size; i++)
std::cout << arr[i] << " ";
}
While this code works for any data type, the concept of templates and specialization may lead beginners to feel overwhelmed.
Multi-paradigm Features
C++ caters to various programming styles, allowing developers to employ functional programming along with object-oriented techniques. This versatility enhances C++, but it also introduces complexity. For instance, switching between paradigms can cause confusion if one isn't completely comfortable with either style.
The Learning Curve
Steeper Learning Path
A unique aspect of C++ is its compilation and linking model, which can pose significant hurdles for newcomers. Understanding the difference between compilation and linking is crucial; improper management of headers and source files can lead to frustrating error messages.
Moreover, C++ compiles separately from other languages, introducing concepts like Makefiles and build systems that can befuddle learners.
Error Handling
Compiling errors in C++ can often be cryptic and challenging to decipher for beginners. For example, consider this simple code that results in a compilation error due to a missing semicolon:
int main() {
std::cout << "Hello World!" // Missing semicolon
return 0;
}
The compiler error may not immediately indicate what's wrong, leaving learners confused and unsure how to resolve the issue.
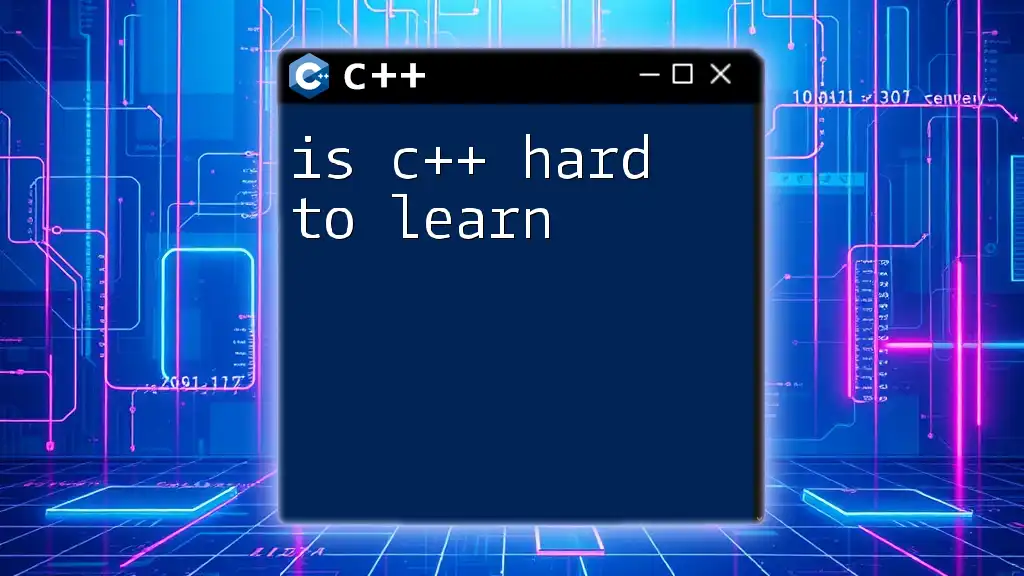
Community and Resources
Lack of Unified Learning Resources
One of the significant barriers many learners face when trying to master C++ is the fragmentation of available learning resources. With a plethora of tutorials, documentation, and courses spread across different platforms, identifying trustworthy and coherent resources can be a daunting task.
Learning Approaches
To overcome the hurdles of C++, it’s vital to adopt structured learning pathways. Some effective approaches include:
- Online Courses: Engaging in comprehensive courses that offer guided instruction.
- Coding Bootcamps: Immersive learning strategies that encourage practical application.
- Community Forums: Participating in communities such as Stack Overflow or C++ forums to seek advice and solutions to specific issues.
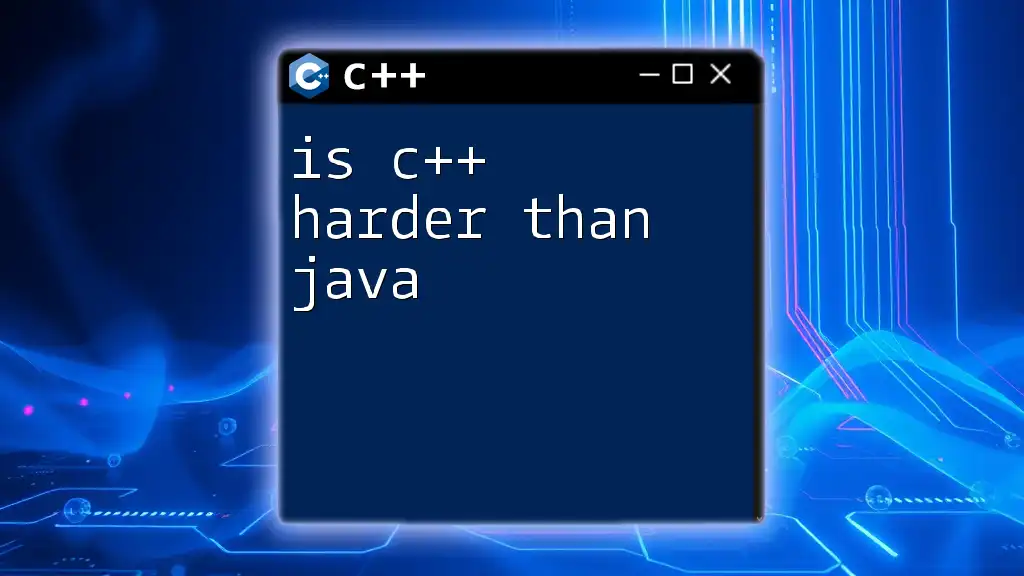
Conclusion
In summary, why is C++ so hard? It incorporates various paradigms, an extensive feature set, complex syntax, and advanced concepts that all contribute to a steep learning curve. Yet, mastering C++ rewards developers with immense power and flexibility in programming.
Encouragement and Advice
Despite these challenges, persistence is essential. The benefits of mastering C++—with its widespread application in industries such as gaming, systems programming, and even artificial intelligence—can offer vast career opportunities. Remember that every expert was once a beginner.
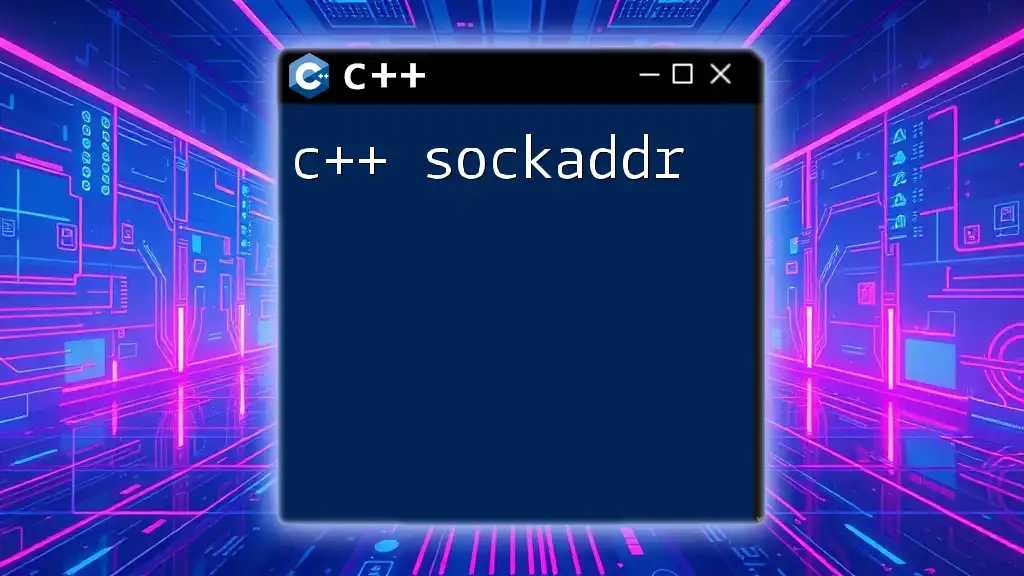
Call to Action
If you're eager to dive deeper into the world of C++, consider engaging with our company for tailored resources and quick guides. Start your journey today with our free resource on fundamental C++ topics and unlock the potential of this powerful language!