A `map` in the C++ Standard Template Library (STL) is an associative container that stores elements in key-value pairs, allowing for fast retrieval based on unique keys.
Here’s a simple example of using a `map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
return 0;
}
What is STL?
The Standard Template Library (STL) is an essential part of the C++ programming language. It provides a collection of template classes and functions to facilitate generic programming. By utilizing STL, developers can save time and effort as it offers pre-defined data structures and algorithms.
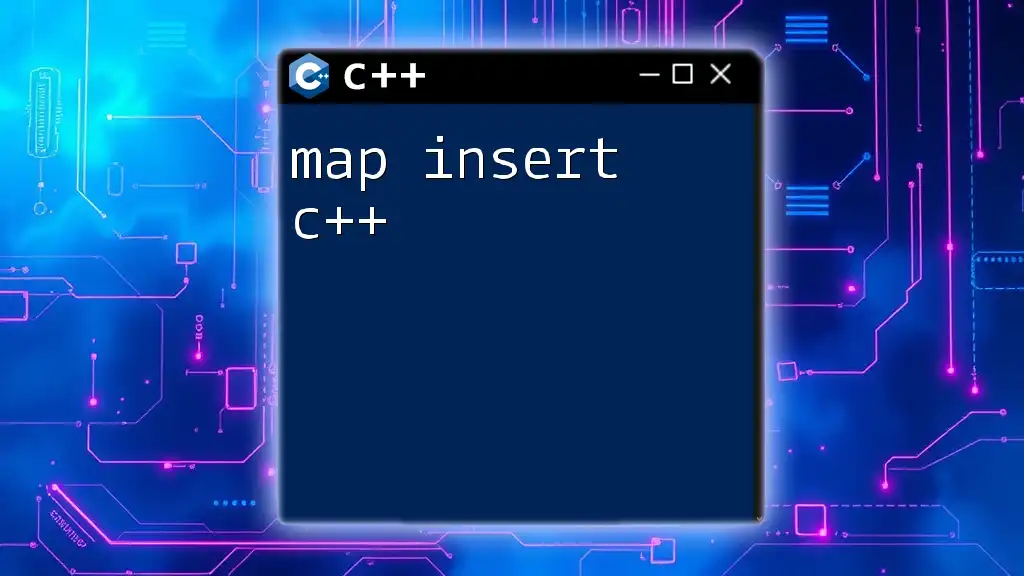
Overview of Map
In C++, a map is a type of associative container that stores elements in key-value pairs. Each key is unique, and it can be associated with a corresponding value. The main characteristics of a map include:
- Associative Nature: It allows for fast retrieval of values based on keys.
- Ordered Key Sequence: Maps are sorted based on keys, with the smallest key present at the beginning and the largest at the end.
- Dynamic Size: The size of a map can change dynamically as elements are added or removed.
Understanding the differences between a `map` and other associative containers such as `set` is crucial. While a `set` stores only unique elements without associated values, a `map` includes both the key and its associated value.
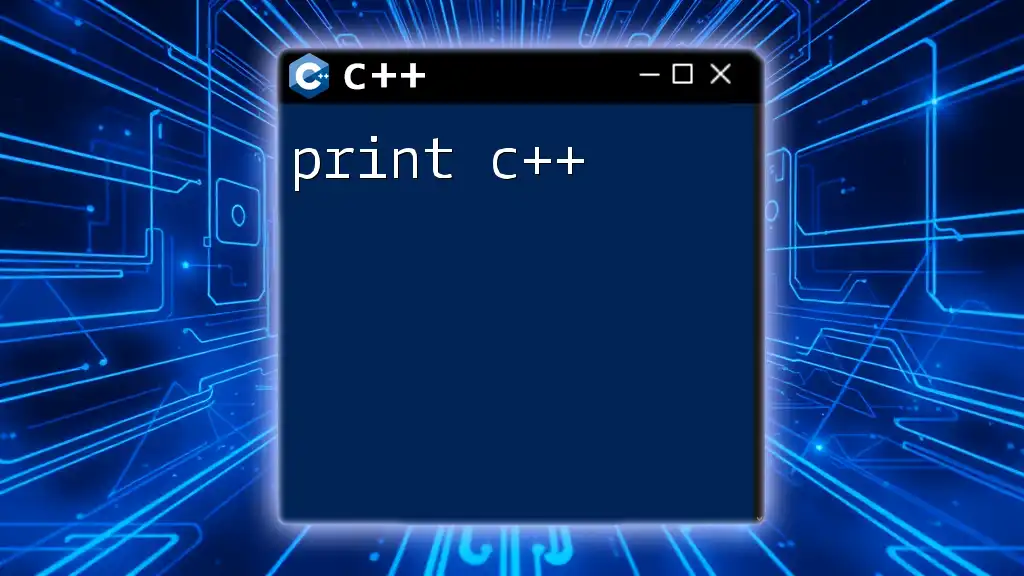
Understanding the Structure of a Map
Key-Value Pairs
A map is essentially a collection of key-value pairs. Each key in the map is unique and is used to access its corresponding value. For example, if you have a map storing the population of various cities, the city names can be the keys, and their populations can be the respective values.
Underlying Implementation
Internally, maps are typically implemented using balanced binary trees (like Red-Black Trees). This structure ensures that operations such as insertion, deletion, and search can be performed in logarithmic time, \(O(\log n)\). This balanced approach maintains the order of elements while allowing for efficient access.
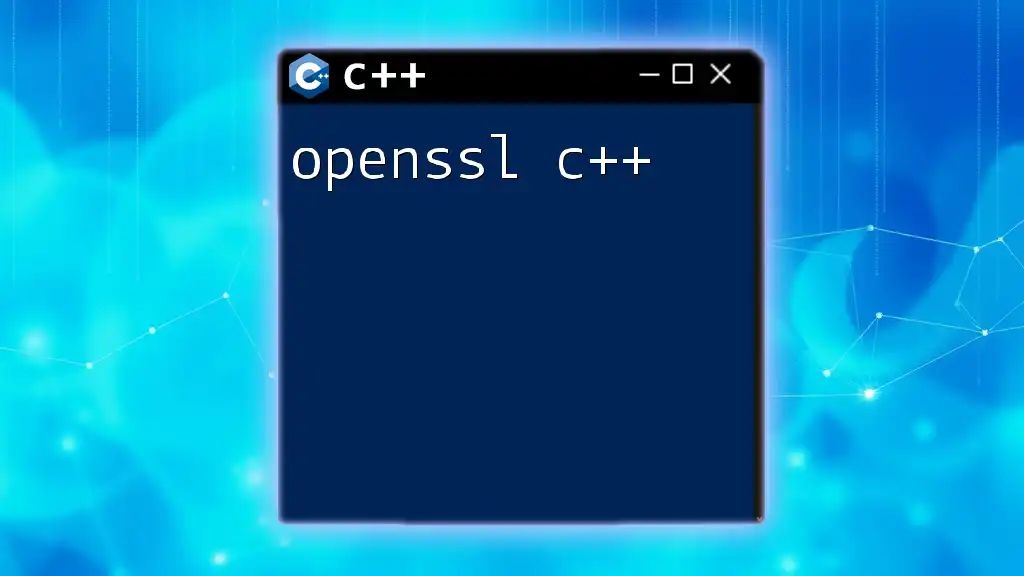
Creating and Initializing Maps
Basic Syntax
To declare a map in C++, the general syntax is:
std::map<keyType, valueType> mapName;
Here `keyType` is the type of the key, and `valueType` is the type of the value.
Initialization Techniques
You can initialize a map in several ways:
-
Using Initializer Lists:
std::map<std::string, int> cityPopulation = {{"New York", 8419600}, {"Los Angeles", 3980400}, {"Chicago", 2716000}};
-
Using the `insert` Function:
std::map<std::string, int> fruitCount; fruitCount.insert(std::make_pair("Apples", 20)); fruitCount.insert(std::pair<std::string, int>("Oranges", 15));
Both methods effectively allow you to populate your map upon creation.
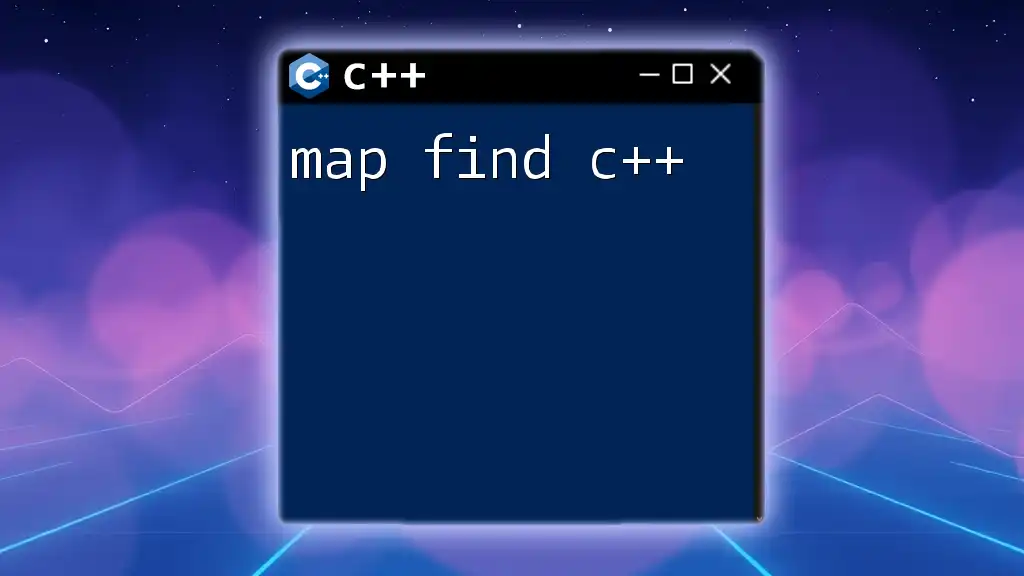
Common Operations on Maps
Inserting Elements
Inserting elements into a map can be done using two main methods:
-
Using the `insert` method:
std::map<std::string, int> studentGrades; studentGrades.insert(std::make_pair("Alice", 90));
-
Using the `operator[]`:
studentGrades["Bob"] = 85; // Automatically inserts 'Bob' with a value of 85
Accessing Elements
To retrieve values associated with keys, you can access them directly using either the `operator[]` or the `find` method. Remember that `operator[]` will insert a new entry with a default value if the key is not found, while `find` will simply return an iterator pointing to the desired element:
int aliceGrade = studentGrades["Alice"];
auto it = studentGrades.find("Bob");
if (it != studentGrades.end()) {
int bobGrade = it->second;
}
Modifying Elements
You can modify existing values by simply accessing them with the key:
studentGrades["Alice"] = 95; // Changes Alice's grade to 95
Deleting Elements
To erase elements from a map, you can use the `erase` method:
studentGrades.erase("Bob"); // Removes Bob from the map
To clear all entries, utilize the `clear` method:
studentGrades.clear(); // Clears the entire map
Iterating Through a Map
You can iterate through the elements of a map in several ways:
-
Using Iterators:
for (auto it = studentGrades.begin(); it != studentGrades.end(); ++it) { std::cout << it->first << ": " << it->second << std::endl; }
-
Using Range-Based For Loop:
for (const auto &pair : studentGrades) { std::cout << pair.first << ": " << pair.second << std::endl; }
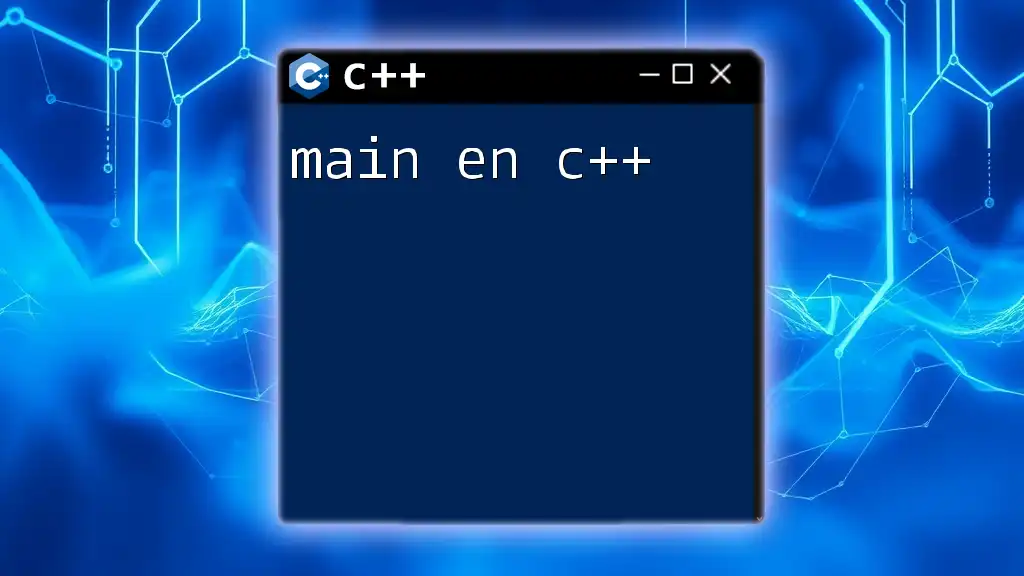
Advanced Features of Maps
Multi-map
A multimap is similar to a map, but it allows for duplicate keys. This feature is useful when you need to associate multiple values with a single key.
std::multimap<char, int> exampleMultimap;
exampleMultimap.insert(std::make_pair('a', 1));
exampleMultimap.insert(std::make_pair('a', 2)); // Allows duplicate key
Sorting and Ordering
Maps are sorted automatically based on the keys in ascending order. If you need custom sorting, you can define your comparator function:
struct customCompare {
bool operator()(const std::string &a, const std::string &b) const {
return a.length() < b.length(); // Sort by string length
}
};
std::map<std::string, int, customCompare> customMap;
Performance Considerations
Choosing when to use a map versus other data structures is crucial. While maps provide efficient access and ordering, keep in mind:
- Overhead: Maps generally consume more memory due to their tree-like structure.
- Best Practices: Utilize maps when you frequently need to access elements based on keys, but assess whether a simpler structure (like an array or vector) might suffice for smaller datasets.
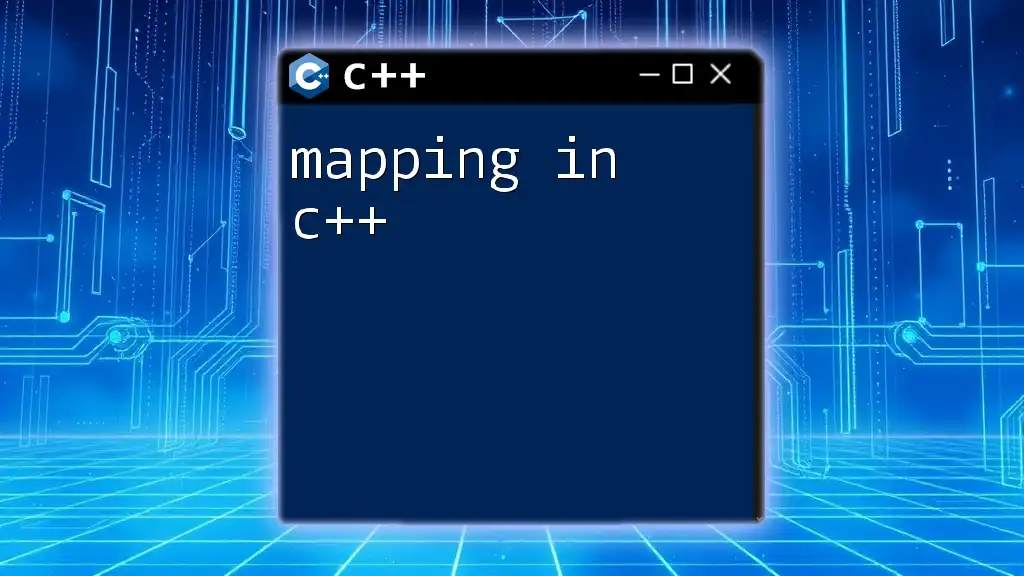
Real-world Applications of Maps
Maps are highly versatile and find numerous applications:
- Frequency Counts: They can count the occurrences of each unique element in a collection.
- Implementation of Phone Books: Mapping names to phone numbers provides a practical application for quick lookups.
- Game Development: Keeping track of scores, player attributes, or inventory items in a game environment.
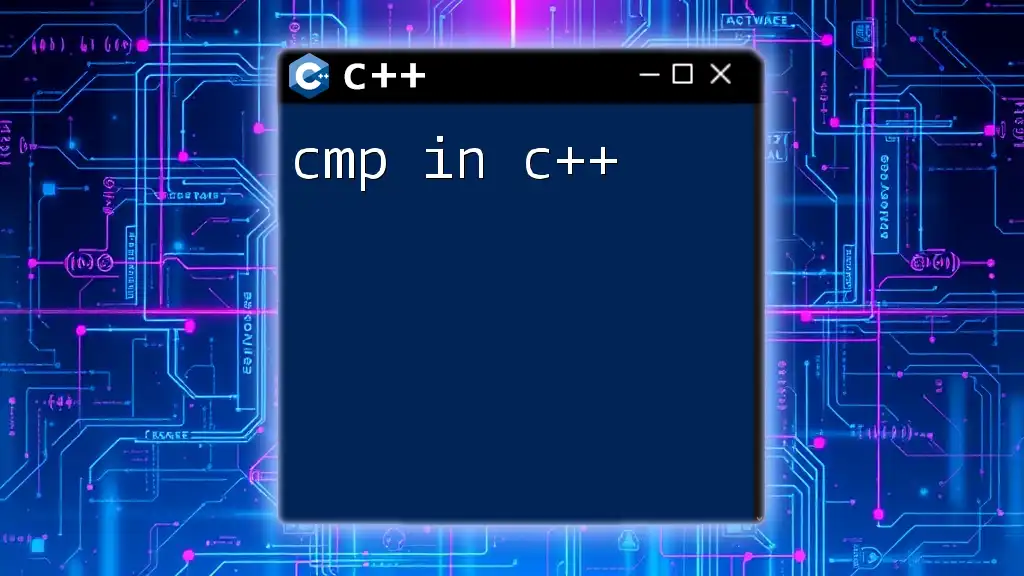
Conclusion
To summarize, understanding maps in STL C++ is critical for efficient data management in various programming scenarios. Their associative nature, combined with dynamic sizing and order maintenance, makes them a powerful tool in any C++ programmer's toolkit.
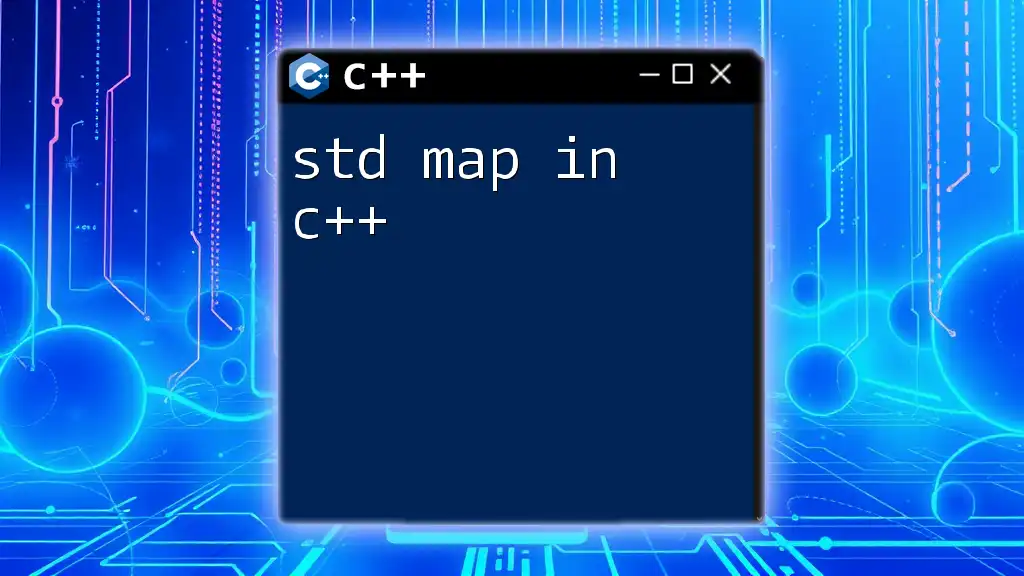
Additional Resources
For further learning and in-depth understanding, consider exploring the following resources:
- C++ Documentation on STL
- Online tutorials specific to C++ maps
- C++ programming community forums and discussion groups
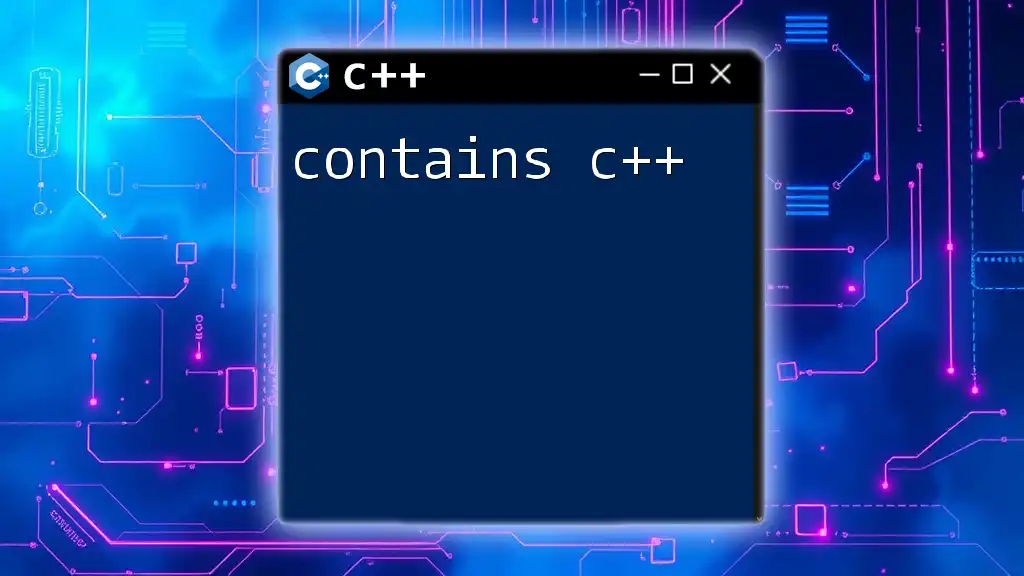
FAQs about Maps in STL C++
- What happens if I use a non-unique key?
- Using a non-unique key in a `map` will overwrite the existing value. Consider using a `multimap` if you require duplicate keys.
- Can I change the key of a map entry once it has been added?
- No, keys in a map are immutable; to change a key, you must remove the old entry and insert a new one with the new key.
This comprehensive guide should empower you to effectively utilize maps in your C++ projects, providing clarity on operations, structure, and practical applications.