C++14 is a version of the C++ programming language that introduced several enhancements and new features to improve code usability and performance, such as generic lambdas and the `std::make_unique` function.
#include <memory> // Required for std::make_unique
#include <vector>
int main() {
// Using std::make_unique to create a unique_ptr for a vector of integers
auto vec = std::make_unique<std::vector<int>>(10); // Initializes a vector with 10 elements
return 0;
}
What is C++14?
C++14 is an important iteration of the C++ programming language, introduced after C++11. It brings a mix of new features along with enhancements to existing functionality, making it a valuable update for programmers. By refining and building upon C++11’s groundwork, C++14 improves efficiency, usability, and overall performance.
C++14 primarily stands out due to its subtle but effective improvements over C++11 rather than introducing a major overhaul. This makes it a significant upgrade for developers looking to familiarize themselves with the language's evolution.
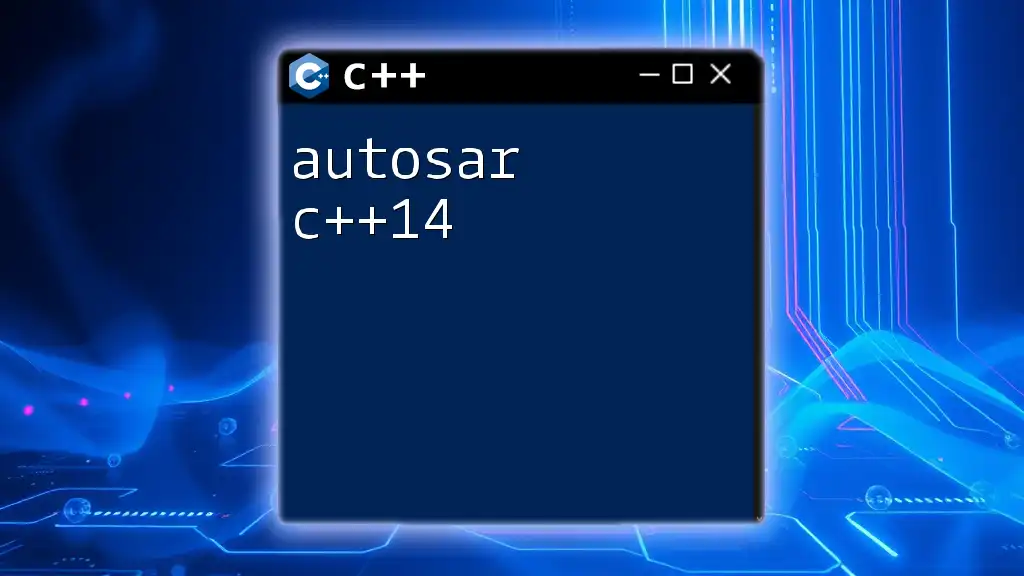
New Features in C++14
More Relaxed Constraints on Variable Templates
Variable templates allow you to define a template that is not just a function or class, but a variable. C++14 improves upon C++11 by relaxing constraints, making templated variables more flexible and usable.
For example, you can define a constant value like this:
template<typename T>
constexpr T pi = T(3.1415926535897932385);
This segment illustrates how you can create a templated constant for different types, enhancing code reusability and clarity.
Generic Lambdas
C++14 introduces generic lambdas, allowing lambdas to take parameters of any type without explicitly specifying the type in the lambda definition. This adds more versatility in how lambdas can be used in your code.
Here’s an example of a generic lambda:
auto f = [](auto x) { return x + 1; };
In this case, `f` can accept any type that supports the addition operator. This flexibility makes your code more concise and adaptable.
Return Type Deduction
With C++14, you can now leverage auto keywords to deduce the return type of functions implicitly. This reduces verbosity while still ensuring type safety.
Here’s how you can implement return type deduction:
auto add(int a, int b) { return a + b; }
In this snippet, the type of the return value is automatically deduced from the operation performed. This feature is particularly useful in situations where the return type might be complex or cumbersome to explicitly state.
Enhanced `std::make_unique`
Smart pointers have become an essential aspect of modern C++ programming. C++14 improved support for them with the introduction of `std::make_unique`, which simplifies the creation of unique pointers while ensuring exception safety.
An example of `std::make_unique` in action looks like this:
auto ptr = std::make_unique<MyClass>(args);
This approach simplifies memory management and reduces the risk of memory leaks, making your code cleaner and safer.
Deprecated Features
C++14 also identifies certain features as deprecated, signaling to developers that future versions may remove them. It’s crucial for programmers to stay aware of these deprecated features to ensure code longevity and compatibility with upcoming C++ standards.
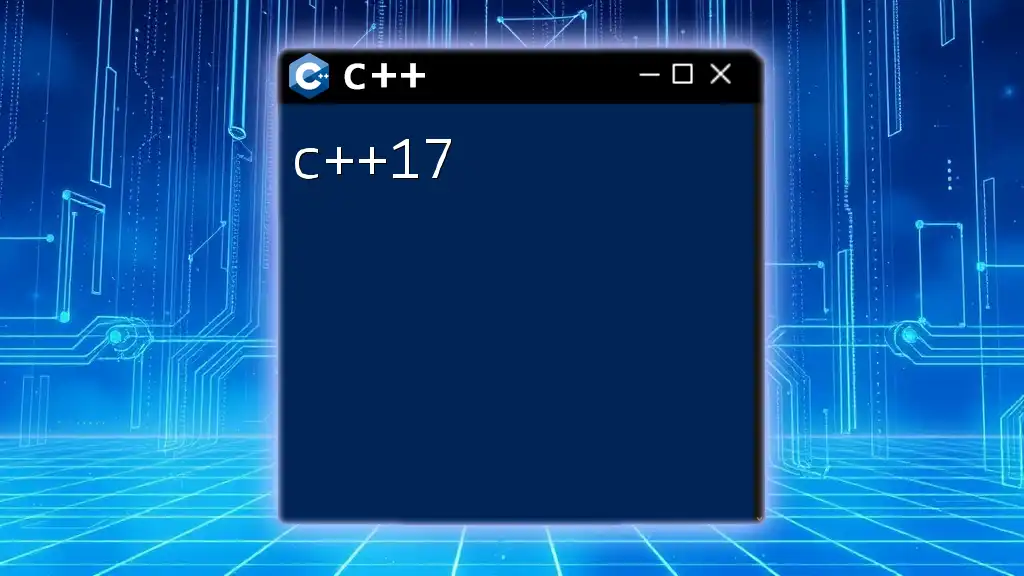
Important Library Additions in C++14
New Standard Library Features
C++14 extends the capabilities of the standard library by introducing new features and functionality. One notable example is the `std::shared_timed_mutex`, which allows for more precise control of thread access in multithreaded environments.
Here’s how you might use `std::shared_timed_mutex`:
#include <shared_mutex>
std::shared_timed_mutex mtx;
This enhancement allows for shared access among threads, improving concurrency in your applications.
Improved Support for Multithreading
C++14 builds upon the multithreading capabilities introduced in C++11, offering enhanced performance and usability. For instance, the library expansions and refinements make threading more intuitive.
A basic illustration of threading in C++14 could look like this:
std::thread t([] { /* thread work */ });
With C++14, developers can implement complex multithreaded applications more easily, making it an attractive choice for performance-critical systems.
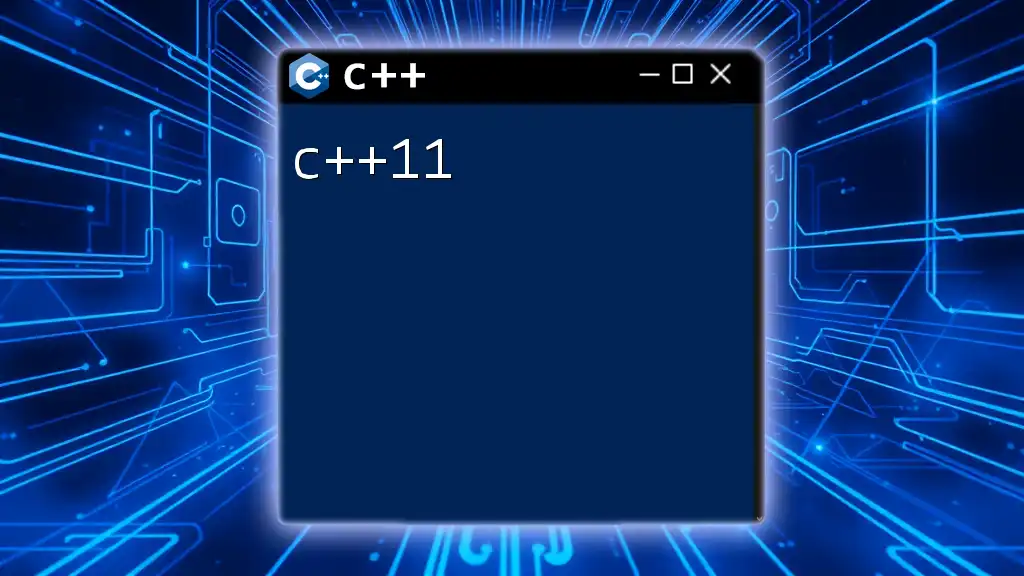
Compiler Support for C++14
Several popular compilers have adopted C++14 support, including:
- GCC: 4.9 and later versions
- Clang: 3.4 and later versions
- MSVC: Visual Studio 2015 and newer
To enable C++14 features in various Integrated Development Environments (IDEs), you typically need to adjust the build settings or use command-line flags, like `-std=c++14` for GCC and Clang.
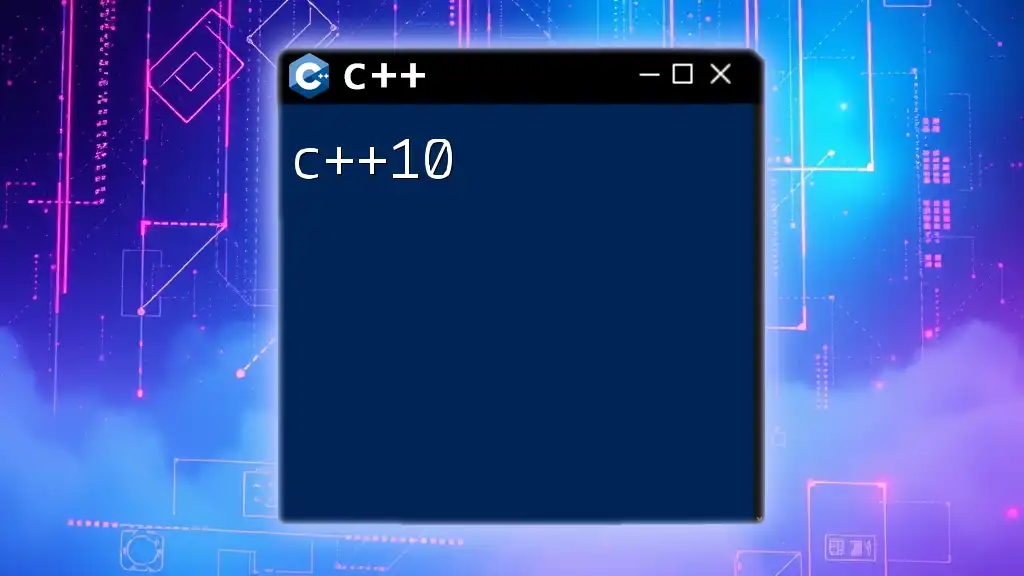
Real-World Applications of C++14
C++14 finds its applications across various industries, such as game development, systems programming, and embedded systems. The blend of performance improvements and advanced libraries has made it a go-to choice for developers seeking to create high-performance applications.
One notable example includes a gaming engine utilizing C++14 features, allowing developers to achieve intricate graphics and responsive gameplay mechanics through optimized threading and resource management.
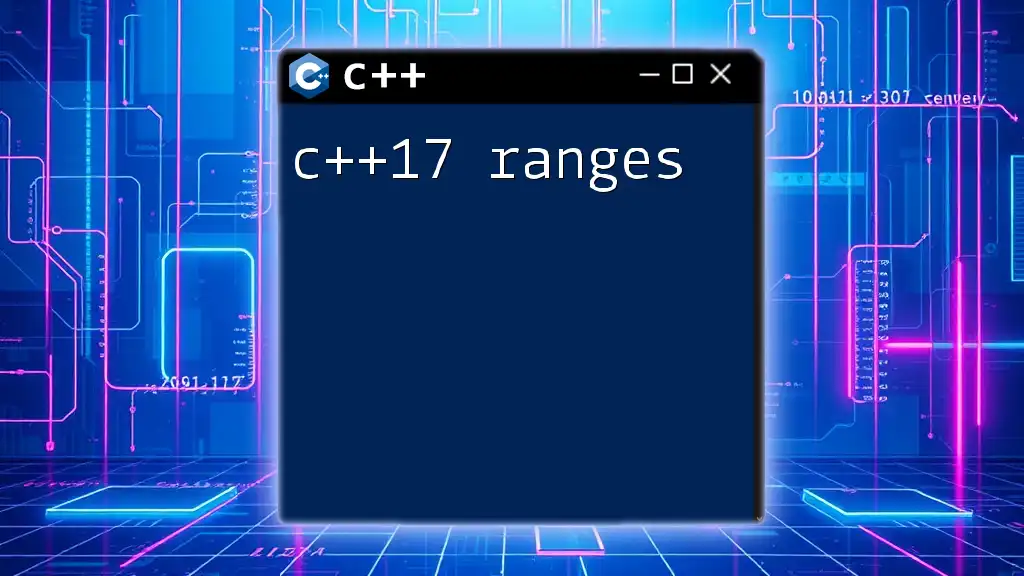
Best Practices for Using C++14
Writing Clean Code
When working with C++14, adhering to principles of clean code is paramount. This includes using meaningful variable names, making judicious use of lambdas and templates to avoid redundancy, and structuring your code for readability.
Optimizing Performance
To capitalize on C++14's capabilities, developers should focus on optimizing their applications. This can include minimizing unnecessary copies, profiling code to identify bottlenecks, and using the new features like `std::make_unique` for better memory management.
An example of performance tuning could look like refactoring a function to leverage move semantics, improving efficiency without sacrificing clarity.
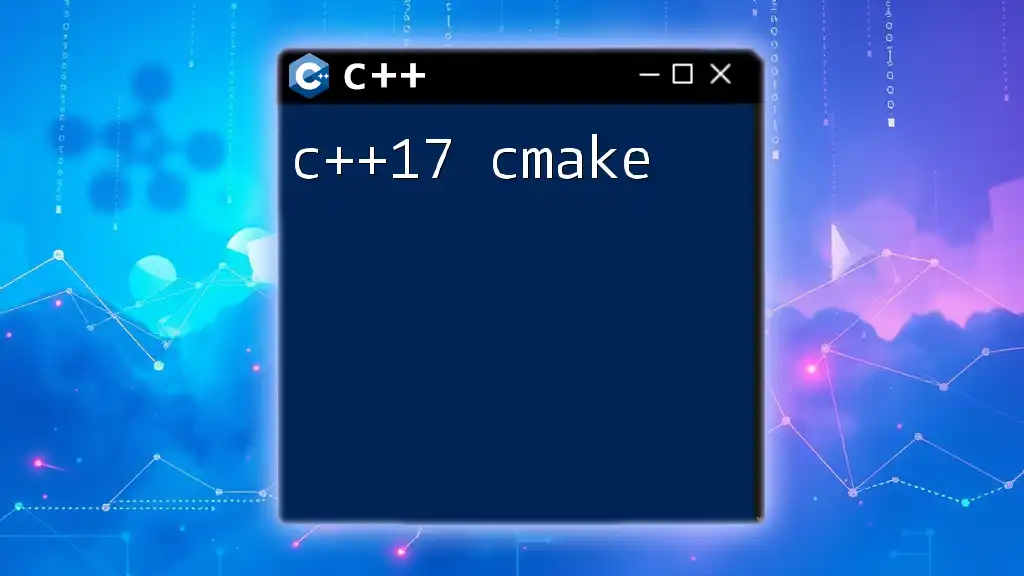
Conclusion
C++14 had a profound impact on the programming landscape, with its blend of new features and enhancements allowing programmers to write cleaner, more efficient code. Its ability to cater to modern development practices while ensuring backward compatibility makes it essential for developers aiming to take advantage of the latest improvements in the C++ language.
By exploring the features and best practices associated with C++14, you can enhance your programming toolkit and leverage this powerful language update in your own projects.
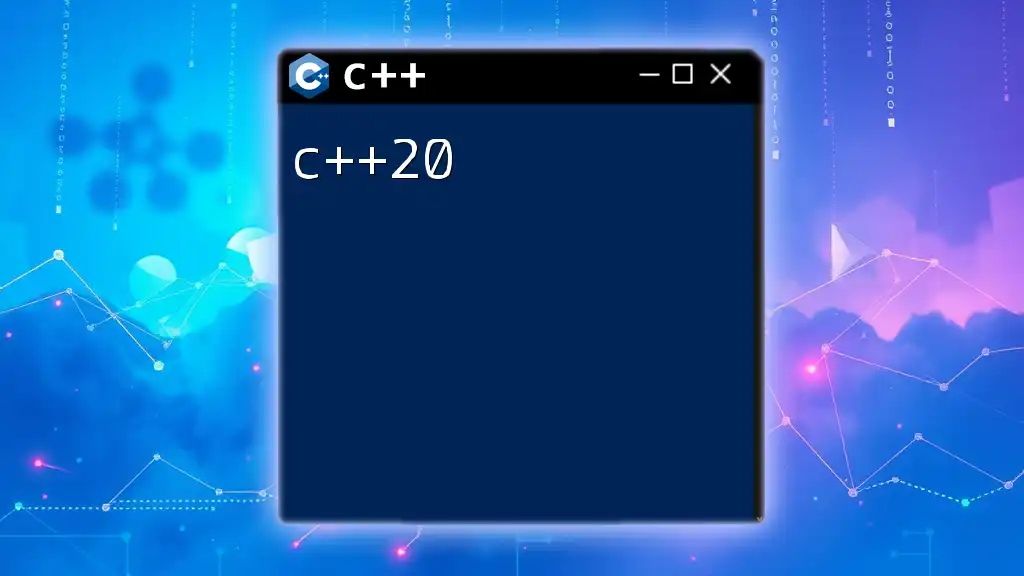
Additional Resources
For further learning, consider exploring recommended books and online courses dedicated to C++14. Many useful online communities exist, offering support and additional insights into leveraging C++14 in your coding projects.
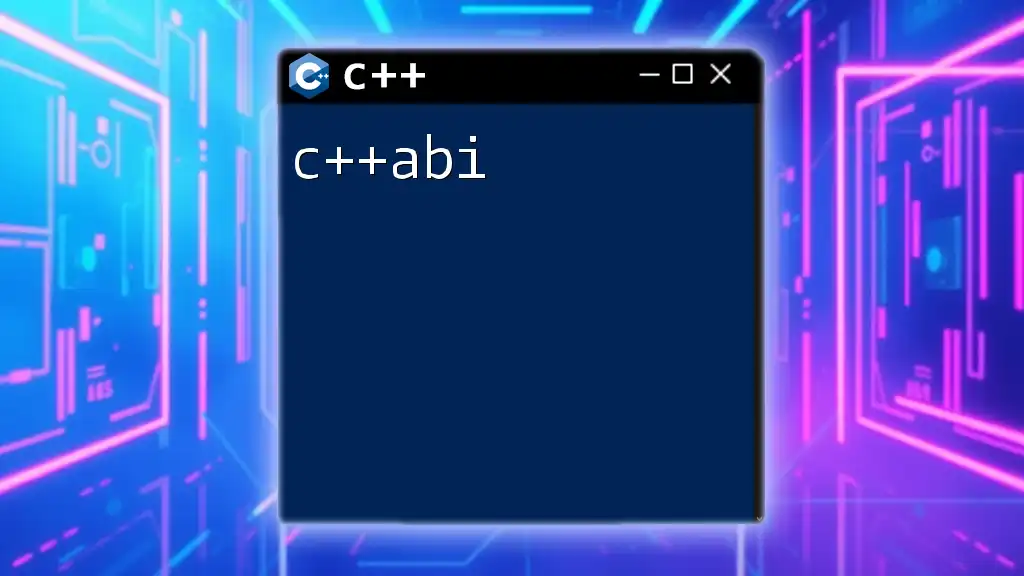
FAQs
What are the most significant advancements in C++14?
C++14 introduces variable templates, generic lambdas, return type deduction, and enhanced smart pointers, all of which contribute significantly to cleaner and more efficient code.
Why should I upgrade to C++14?
Upgrading to C++14 means benefiting from improved language features and standard library enhancements, which can lead to better performance and reduced code complexity.