C++26 refers to the upcoming version of the C++ programming language standard, which is expected to introduce new features and enhancements, further optimizing the language for modern software development.
Here's a simple example of a new feature expected in C++26:
#include <iostream>
#include <concepts>
template<typename T>
concept Incrementable = requires(T a) { ++a; };
template<Incrementable T>
void increment(T& value) {
++value;
}
int main() {
int number = 5;
increment(number);
std::cout << "Incremented number: " << number << std::endl; // Outputs: Incremented number: 6
}
New Features in C++26
Core Language Enhancements
Modules and Their Usage
Modules provide a powerful alternative to include directives, enhancing code organization and compilation speed. By encapsulating data and providing clear interfaces, modules streamline interactions between different parts of a program. In C++26, using modules reduces compilation time significantly, especially in large projects.
Code Example: Here's how you can create and use a module:
// my_module.cppm
export module my_module; // Declare a module
export void hello() { // Exported function
std::cout << "Hello from my_module!" << std::endl;
}
To use this module:
// main.cpp
import my_module; // Import the module
int main() {
hello(); // Call the function from the module
return 0;
}
Expanded Concepts
Concepts in C++26 bring more intuitive and clear constraints to template programming, ensuring a better function and template matching process.
Code Example: Implementing concepts can simplify code and prevent errors:
template<typename T>
concept Integral = std::is_integral_v<T>; // Define a concept
template<Integral T>
void process(T value) {
// Process integral values
}
Standard Library Improvements
Enhanced Data Structures
C++26 introduces new data structures, including enhanced maps and sets that optimize lookup and insertion times.
Code Snippet: Here’s a quick example of utilizing a new map feature:
#include <map>
std::map<int, std::string> myMap;
myMap.insert({1, "one"});
myMap.insert({2, "two"});
String Handling Improvements
C++26 enhances string operations, making manipulations more efficient and intuitive.
Code Example: With new string handling features, you can easily reverse a string:
#include <string>
#include <algorithm>
std::string reverseString(const std::string& str) {
std::string reversed = str;
std::reverse(reversed.begin(), reversed.end());
return reversed;
}
Coroutines and Asynchronous Programming
Improvements in Coroutines
With advancements in C++26, coroutines are now more powerful, supporting better resource management and performance optimizations.
Code Example: A simple coroutine example showcasing asynchronous operations:
#include <coroutine>
struct generator {
struct promise_type {
generator get_return_object() { return {}; }
std::suspend_always yield_value(int value) { return {}; }
std::suspend_never return_void() {}
void unhandled_exception() {}
};
// Coroutine functions can be defined here
};
Future and Promise Enhancements
New features in futures and promises enable more robust asynchronous programming, making it easier to write code that waits for asynchronous results.
Code Snippet: An example of enhanced futures:
#include <future>
auto asyncTask() {
return std::async(std::launch::async, [] { return 42; });
}
int main() {
auto result = asyncTask().get(); // Await result
std::cout << "Result: " << result << std::endl;
return 0;
}
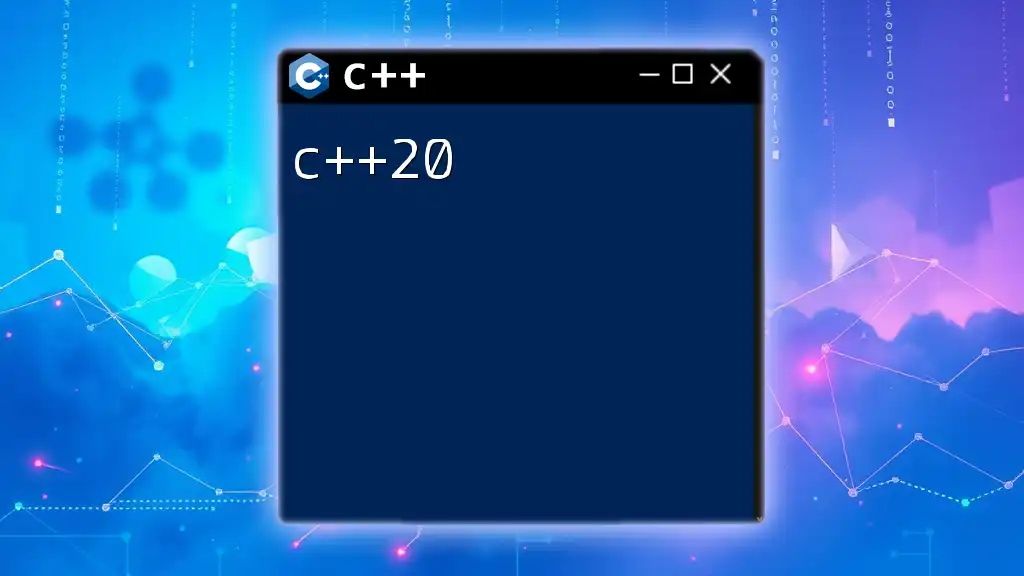
C++26 Language Features Explained
Template Enhancements
Improved Template Syntax
C++26 provides a revamped syntax for templates, allowing for clearer and more efficient template programming.
Code Example: The following illustrates updated syntax:
template<typename T>
struct MyType {
T data;
};
// Using the structure
MyType<int> myInstance;
Variadic Templates Optimization
C++26 optimizes variadic templates, making them more efficient and easier to manage.
Code Snippet: Demonstrating efficient variadic template use:
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << std::endl; // Fold expression
}
Lambdas and Functional Programming
Lambdas with Template Parameters
C++26 introduces powerful new features for lambdas, including the ability to use template parameters within lambda expressions.
Code Example: Here’s how you can define a lambda with a template parameter:
auto add = [](auto a, auto b) { return a + b; };
std::cout << "Sum: " << add(3, 4) << std::endl; // Works with different types
Function Objects Enhancements
Enhancements to function objects in C++26 allow for greater flexibility and functionality.
Code Snippet: Here's an example showing advanced function object usage:
struct Adder {
int operator()(int a, int b) const {
return a + b;
}
};
Adder add;
std::cout << "Add: " << add(10, 20) << std::endl;
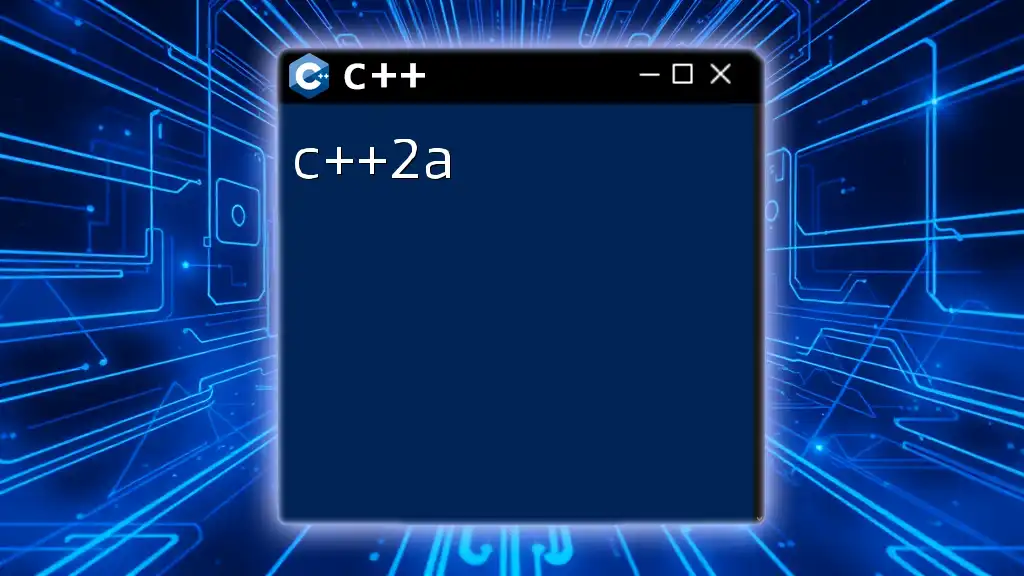
Interoperability and Compilation Improvements
Language Interoperability
Integration with Other Languages (e.g., Python, Rust)
C++26 enhances the ability to interface with other languages, allowing developers to utilize libraries and frameworks more seamlessly.
Code Example: A basic interoperability example could be calling a Python function from C++:
// Imagine using a Python API
PyObject* pModule = PyImport_ImportModule("my_python_module");
Foreign Function Interface (FFI)
FFI provides a systematic way to call functions written in other programming languages directly from C++26 code.
Code Snippet: Implementing FFI with an external library might look like this:
extern "C" {
int some_function(int a, int b);
}
int main() {
int result = some_function(5, 3);
std::cout << "Result from C function: " << result << std::endl;
}
Compilation Speed and Performance
Compiler Optimizations
Optimizations in compilers for C++26 focus on reducing compile times and improving overall performance, enabling developers to write more efficient code.
Examples: Before and after comparisons can demonstrate these improvements, showcasing the dramatic reductions in compilation times.
Compile-time Programming
Compile-time programming in C++26 is an essential feature that allows developers to run computations during compilation, providing performance boosts at runtime.
Code Example: A simple compile-time computation:
constexpr int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
static_assert(factorial(5) == 120); // Compile-time validation
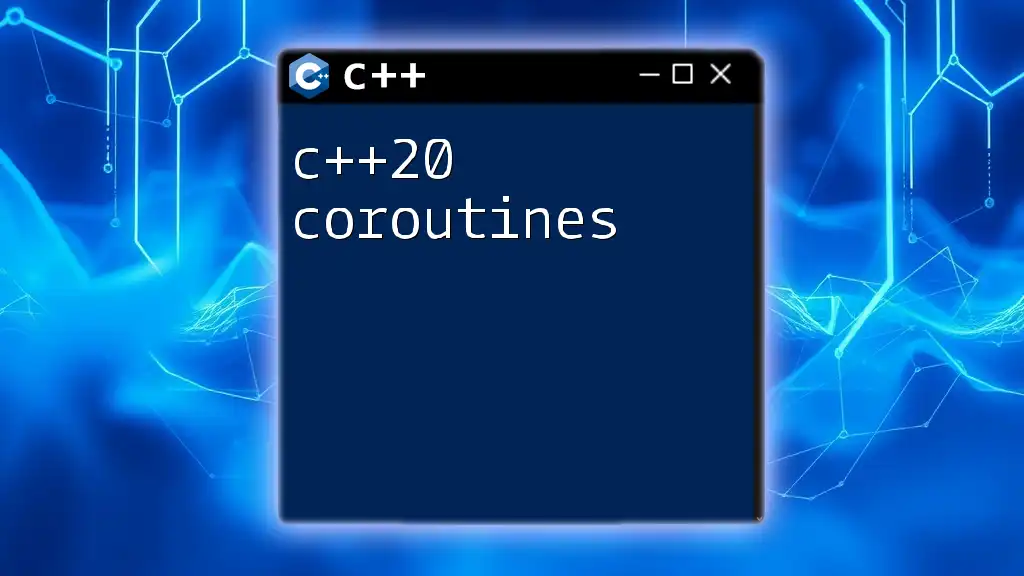
Community and Ecosystem Updates
C++26 Community Contributions
Open Source Projects
Throughout the development of C++26, numerous open-source projects have played a vital role in enhancing its capabilities and showcasing its features.
Case Studies: Highlighting successful projects that leverage C++26 innovations can inspire developers and show practical applications.
Collaborative Tools
C++26’s ecosystem sees improved collaborative tools that enhance development, making it easier for developers to work together on projects.
Examples: Tools such as GitHub repositories offering C++26 templates and shared libraries.
Educational Resources
Courses and Workshops
There are now an abundance of online courses and workshops that focus on teaching the new features and intricacies of C++26.
List: Platforms like Coursera and Udemy provide in-depth courses tailored to beginners and experienced programmers alike.
Books and Reference Materials
For a more profound understanding and mastery, numerous informative books detail the intricacies of C++26.
List: Consider reading titles such as "C++26: The Complete Guide" or "Modern C++ Programming".
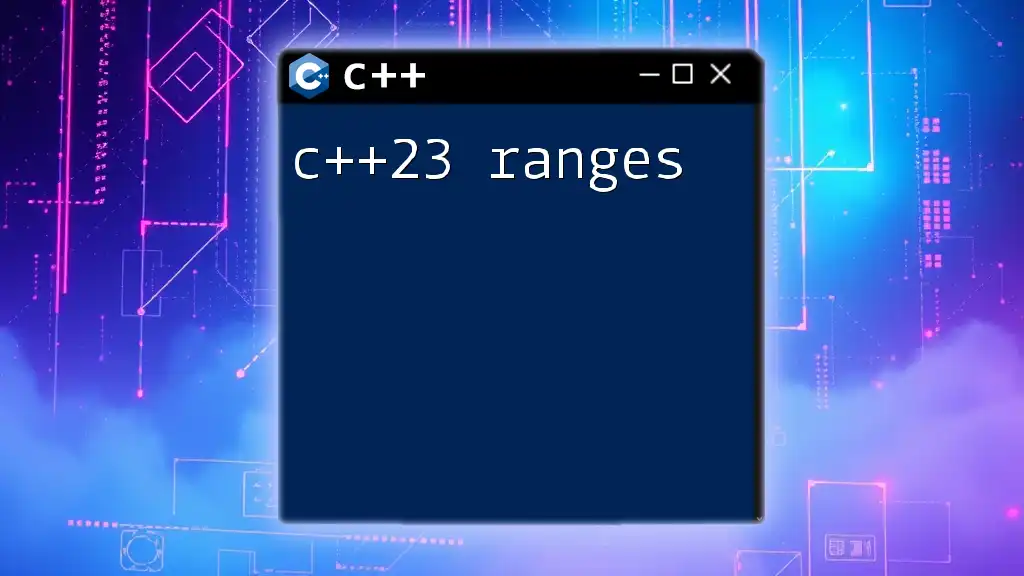
Conclusion
C++26 signifies a pivotal evolution in the C++ programming landscape with its myriad of features enhancing usability, performance, and interoperability. As you grow familiar with the advancements, seize the opportunity to explore and innovate with C++26, ensuring that your skill set remains relevant in a rapidly evolving tech environment.
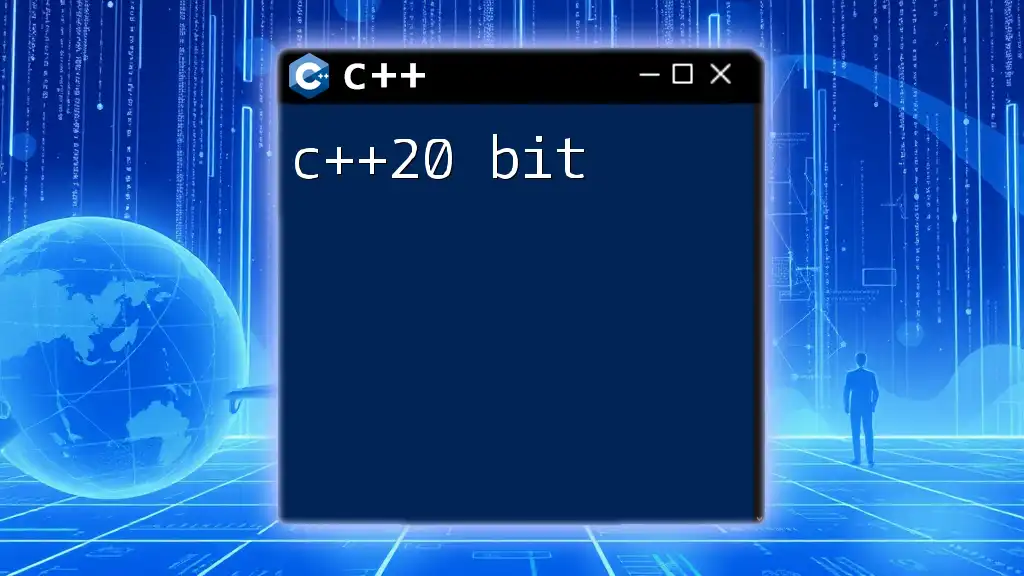
FAQs About C++26
What key features distinguish C++26 from previous versions?
C++26 introduces modules, enhanced templates, and improved coroutines — all aimed at refining the developer experience and code efficiency.
Is C++26 backward compatible?
Yes, C++26 remains compatible with previous versions, ensuring that developers can adopt new features gradually without having to overhaul existing codebases.
Where can I find more resources about C++26?
Explore online learning platforms, open-source project contributions, and dedicated programming forums to expand your knowledge of C++26.