C++20 introduces the `<bit>` header, which provides convenient functions for manipulating bits, including operations such as counting, shifting, and manipulating individual bits in an efficient manner.
Here's a simple code snippet demonstrating the use of the `std::bitset` class from the `<bit>` header:
#include <iostream>
#include <bitset>
int main() {
std::bitset<8> b("10101010"); // Create a bitset with binary value 10101010
std::cout << "Number of set bits: " << b.count() << std::endl; // Count set bits
std::cout << "Bitset in decimal: " << b.to_ulong() << std::endl; // Convert to decimal
return 0;
}
Key Concepts in Bit Manipulation
Importance of Bit Manipulation
Bit manipulation is a fundamental skill for programmers, especially in systems programming, graphics, and performance-critical applications. By understanding how to manipulate individual bits, developers can achieve more efficient algorithms and gain greater control over memory and data representation. Bit manipulation allows you to perform fast operations, such as checking or setting flags, encoding and decoding information, or optimizing data storage. Real-world scenarios include graphics rendering, cryptography, networking, and aspects of game development, where performance is paramount.
Understanding Bitwise Operators
C++ provides several bitwise operators that enable you to interact directly with the bits of integer types:
- AND (`&`): Produces a bit set only if both bits are set.
- OR (`|`): Produces a bit set if at least one of the bits is set.
- NOT (`~`): Inverts the bits.
- XOR (`^`): Produces a bit set if the bits are different.
- Left Shift (`<<`): Shifts bits to the left, adding zeros from the right.
- Right Shift (`>>`): Shifts bits to the right, discarding bits from the right.
Here’s an example that showcases these operators:
#include <iostream>
int main() {
unsigned int a = 5; // Binary: 0101
unsigned int b = 3; // Binary: 0011
std::cout << "a & b: " << (a & b) << std::endl; // Output: 1 (0001)
std::cout << "a | b: " << (a | b) << std::endl; // Output: 7 (0111)
std::cout << "a ^ b: " << (a ^ b) << std::endl; // Output: 6 (0110)
std::cout << "~a: " << (~a) << std::endl; // Output: -6 (inverted bits)
std::cout << "a << 1: " << (a << 1) << std::endl; // Output: 10 (1010)
std::cout << "a >> 1: " << (a >> 1) << std::endl; // Output: 2 (0010)
return 0;
}

New Features in C++20 Related to Bit Manipulation
std::bitset
C++20 introduces `std::bitset`, allowing developers to manage a sequence of bits efficiently. This data structure is fixed in size and provides member functions for bit manipulation, making it easy to conduct operations on individual bits or sets of bits.
To declare a bitset, you specify the size:
#include <bitset>
#include <iostream>
int main() {
std::bitset<8> bits("10101101");
std::cout << bits << std::endl; // Output: 10101101
// Checking specific bits
std::cout << "Bit 1: " << bits[1] << std::endl; // Output: 1
std::cout << "Bit 4: " << bits[4] << std::endl; // Output: 0
// Setting Unsetting bits
bits.set(2); // Set bit 2
bits.reset(0); // Reset bit 0
std::cout << bits << std::endl; // Output: 10111101
return 0;
}
std::to_integer and std::from_integer
C++20 also introduces `std::to_integer` and `std::from_integer`, which simplify the conversion between `std::bitset` and integral types. This is especially useful for scenarios where bit patterns need to be stored or transmitted as integers.
Here’s how you can convert a bitset to an integer:
#include <bitset>
#include <iostream>
int main() {
std::bitset<8> bits("11010101");
unsigned int number = bits.to_ulong();
std::cout << number << std::endl; // Output: 213
// Converting back to bitset
std::bitset<8> new_bits = std::bitset<8>(number);
std::cout << new_bits << std::endl; // Output: 11010101
return 0;
}
Bit Manipulation Functions
std::popcount
The `std::popcount` function is a handy new feature for counting the number of set bits in a number. This can help optimize operations where you need to know how many bits are active.
Here’s a simple demonstration:
#include <iostream>
#include <bit>
int main() {
unsigned int number = 29; // Binary: 11101
std::cout << std::popcount(number) << std::endl; // Output: 4
return 0;
}
std::bit_serialize and std::bit_deserialize
Serialization and deserialization of bit patterns are made easier with `std::bit_serialize` and `std::bit_deserialize`. These functions facilitate converting the internal representation of objects into a format that can be easily stored or transmitted. While more complex implementation details can be context-dependent, using them can streamline your work with bit patterns.
std::bit_cast
C++20 adds `std::bit_cast`, allowing safe, compile-time type-casting without altering bit patterns. This feature is especially useful for reading data structures across different types safely.
You can see how `std::bit_cast` works in the following example:
#include <bit>
#include <iostream>
struct Source { int x; };
struct Destination { int y; };
int main() {
Source s = { 42 };
Destination d = std::bit_cast<Destination>(s);
std::cout << d.y << std::endl; // Output: 42
return 0;
}
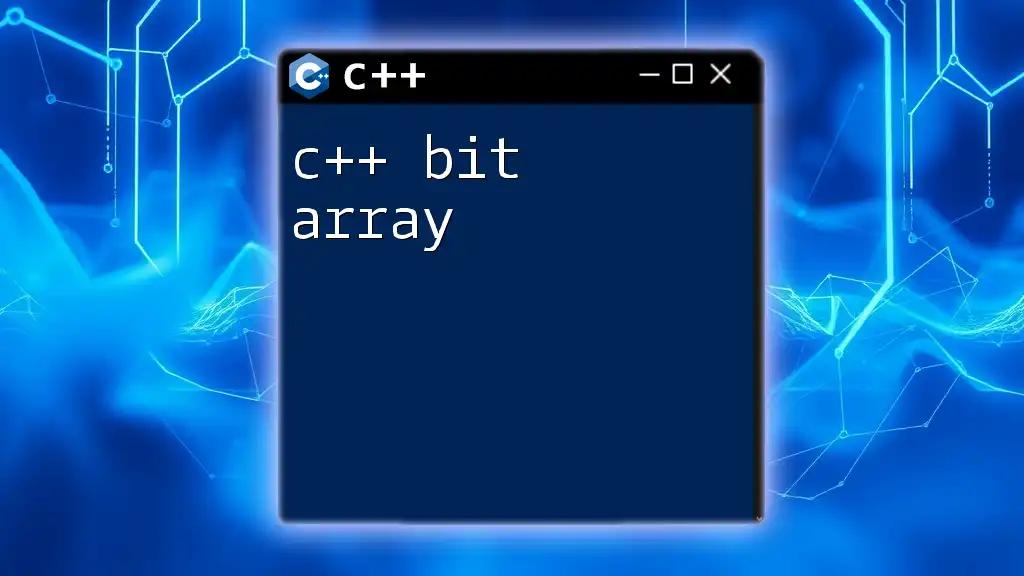
Practical Applications of C++20 Bit Features
Performance Optimization
Mastering bit manipulation can lead to significant performance enhancements in your applications. For instance, using bitwise operations is often more efficient than arithmetic ones, especially in embedded systems where resources are limited. By optimizing algorithms to leverage bit manipulation techniques, developers can reduce execution times and improve the overall efficiency of their applications.
Cryptography and Security
Within the realm of cryptography, bit manipulation plays a critical role in algorithms that encrypt and decrypt data. The performance benefits become apparent when you're working with large volumes of data, where manipulating bits directly can minimize overhead.
Here’s a simple example demonstrating how bitwise operations can be used in a basic encryption method (XOR cipher):
#include <iostream>
#include <string>
std::string encrypt(const std::string &plainText, char key) {
std::string encrypted = plainText;
for (size_t i = 0; i < plainText.length(); ++i) {
encrypted[i] = plainText[i] ^ key; // XOR operation
}
return encrypted;
}
int main() {
std::string text = "Hello World";
char key = 'K';
std::string encryptedText = encrypt(text, key);
std::cout << "Encrypted: ";
for (char c : encryptedText) {
std::cout << c;
}
std::cout << std::endl;
return 0;
}
Data Structures
Bit manipulation enables developers to create compact and efficient data structures. For instance, a Bloom filter uses bit arrays to test whether an element is a member of a set, significantly reducing memory usage compared to traditional data structures while allowing for a small probability of false positives.

Best Practices for Using C++20 Bit Features
When utilizing the new C++20 bit features, consider several best practices:
- Keep your code readable: Overusing bitwise operations can lead to confusion. Strike a balance between performance and clarity.
- Document your intentions: Whenever you manipulate bits, provide comments to clarify why. This will help others (and yourself at a later time) understand your logic.
- Avoid common pitfalls: Always be aware of the size of your integers and potential overflows when performing bit manipulations, especially with shifts and masks.

Conclusion
Mastering bit manipulation is essential for any C++ developer looking to leverage the new features introduced in C++20. By understanding the core concepts and practical applications, you can optimize your code for performance, create secure applications, and effectively manage data structures. Experimentation and practice are key, so don’t hesitate to dive into these exciting features!
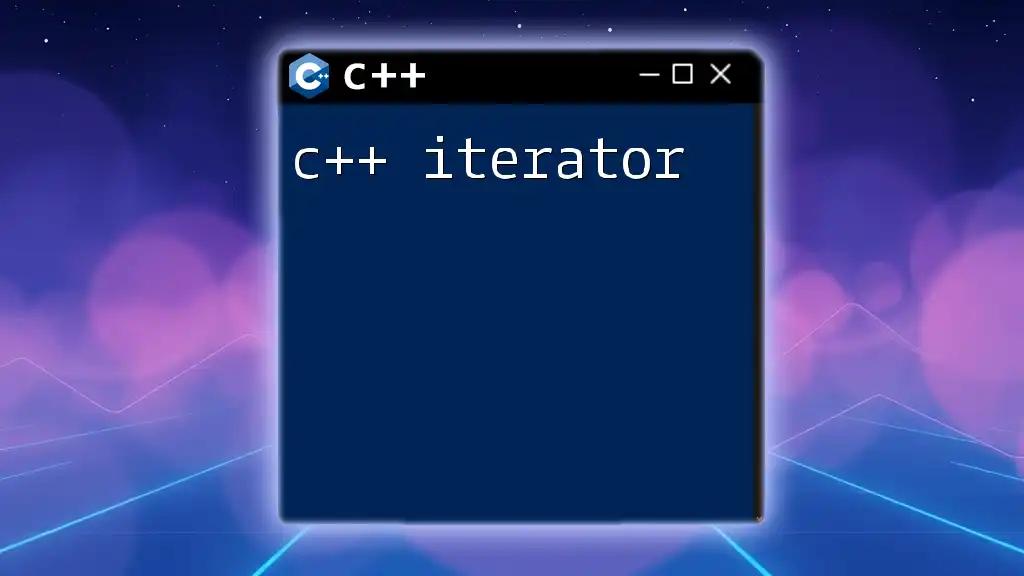
Additional Resources
For more in-depth knowledge, check out the official C++20 documentation and consider exploring books or online courses focused on advanced C++ programming and bit manipulation principles.
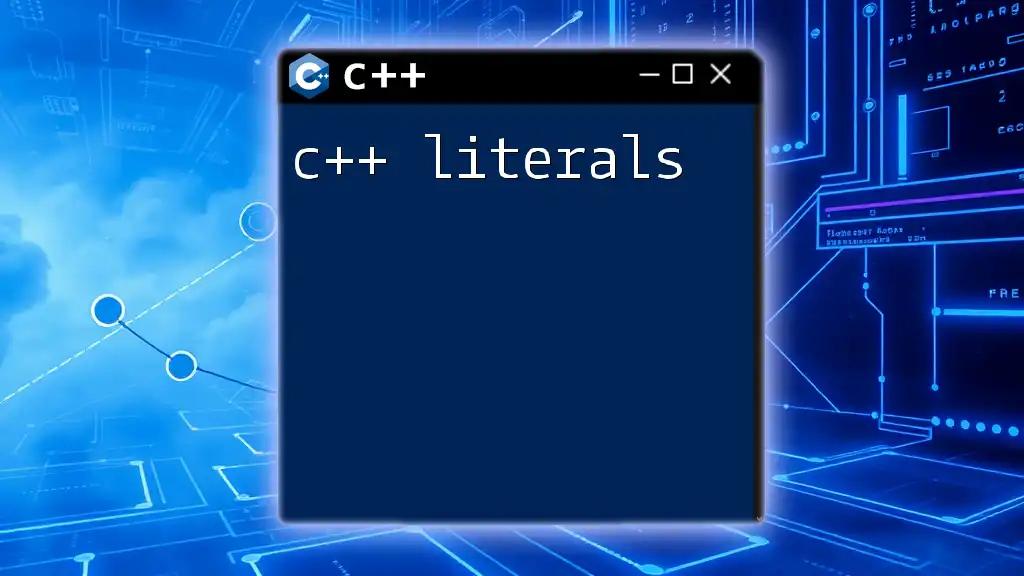
Call to Action
Share your experiences with bit manipulation in C++20! Whether it’s a project you've worked on or an insight you’d like to share, we'd love to hear from you. Remember to explore further with our upcoming courses designed to help you master C++ commands and techniques!