C++20, often referred to as C++2a during its development, introduces several new features and enhancements to the C++ programming language, including concepts, ranges, and coroutines.
Here's a simple example demonstrating the use of a range-based for loop with new standard library features in C++20:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> numbers{1, 2, 3, 4, 5};
for (auto num : numbers | std::views::filter([](int n) { return n % 2 == 0; })) {
std::cout << num << " ";
}
return 0;
}
What is C++20?
C++20, often referred to as C++2a, represents a significant leap in the evolution of the C++ programming language. It introduces new features and improvements that enhance both the performance and usability of the language. Building upon the foundations laid by C++17, C++20 aims to make programming more intuitive and powerful.
The transition from C++17 to C++20 is marked by a focus on creating expressive and maintainable code. As a modern programmer, understanding C++20 is essential for developing high-quality software that meets contemporary requirements.

Why Learn C++20?
Learning C++2a provides several advantages:
- Updated Language Features: Staying abreast of new standards helps in leveraging the latest enhancements and best practices.
- Enhanced Performance: Many new features are optimized for speed, stability, and maintainability.
- Broader Job Opportunities: Proficiency in C++20 can expand your career prospects, as many companies seek developers skilled in modern programming techniques.

Key Features of C++20
Concepts
Concepts are a powerful feature that introduces a new level of type constraints to templates. They allow developers to specify the requirements that template arguments must meet. This leads to cleaner code and improved error messages, making it easier for developers to understand what went wrong when templates are misused.
Here's a straightforward example demonstrating concepts:
template<typename T>
concept Integral = std::is_integral_v<T>;
template<Integral T>
T add(T a, T b) {
return a + b;
}
In this example, the concept `Integral` ensures that the `add` function can only accept integral types. This serves as a form of type safety, reducing the chances of runtime errors and improving code readability.
Ranges
The introduction of the ranges library significantly redefines how developers interact with collections of data. It provides a more expressive way to manipulate sequences of elements using an intuitive pipeline of operations.
Take a look at this example that filters even numbers from a vector:
#include <ranges>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec{1, 2, 3, 4, 5};
auto even_numbers = vec | std::views::filter([](int i) { return i % 2 == 0; });
for (int n : even_numbers) {
std::cout << n << " "; // Outputs: 2 4
}
}
In this snippet, the use of `std::views::filter` provides a lazy way to access elements that satisfy a condition. This leads to potentially improved performance and better separation of logic, making the code easier to understand.
Coroutines
Coroutines introduce a new way of handling asynchronous programming, enabling operations that can be suspended and resumed. This feature adds flexibility and efficiency to tasks such as I/O operations and concurrency.
Consider the following simple coroutine example:
#include <iostream>
#include <coroutine>
struct Generator {
struct promise_type {
int current_value;
auto get_return_object() { return Generator{std::coroutine_handle<promise_type>::from_promise(*this)}; }
auto yield_value(int value) { current_value = value; return std::suspend_always(); }
void return_void() {}
};
std::coroutine_handle<promise_type> handle;
~Generator() { if (handle) handle.destroy(); }
int next() { handle.resume(); return handle.promise().current_value; }
};
Generator numbers() {
for (int i = 0; i < 5; i++) {
co_yield i;
}
}
int main() {
auto gen = numbers();
for (int i = 0; i < 5; i++) {
std::cout << gen.next() << " "; // Outputs: 0 1 2 3 4
}
}
In this example, the `Generator` struct utilizes coroutines to yield values sequentially. This not only enhances readability but also makes asynchronous code easier to manage compared to traditional callbacks and threading models.
New Standard Library Features
C++20 refines the standard library by introducing several new components, one of the most anticipated being `std::format`. This library simplifies string formatting, making it both safer and more efficient.
Here's how `std::format` streamlines string manipulation:
#include <format>
#include <iostream>
int main() {
int value = 42;
std::string formatted = std::format("The answer is: {}", value);
std::cout << formatted; // Outputs: The answer is: 42
}
With `std::format`, developers can create formatted strings more intuitively compared to older methods such as `printf`, thus enhancing code clarity and reducing the risk of errors.
Modules
Modules represent a significant shift towards modular programming in C++. They allow for better organization of code and improve compile times by exposing only the needed parts of a library rather than recompiling the entire codebase every time. This encapsulation leads to improved dependency management and code clarity.
Benefits of Using Modules
- Faster Compilation: Modules can speed up the build process significantly by minimizing code dependencies.
- Encapsulation: By directly importing only the modules needed, code becomes cleaner and easier to manage.
Improved `constexpr`
The `constexpr` feature has seen substantial enhancements in C++20, allowing for more complex computations at compile-time. Developers can now designate a greater variety of functions as `constexpr`, making it easier to write efficient, evaluable code.
Consider the following example showcasing this improvement:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
constexpr int result = factorial(5); // This can be evaluated at compile time.
In this code snippet, the `factorial` function can now be executed during compile time, resulting in potentially improved performance by reducing runtime calculations.
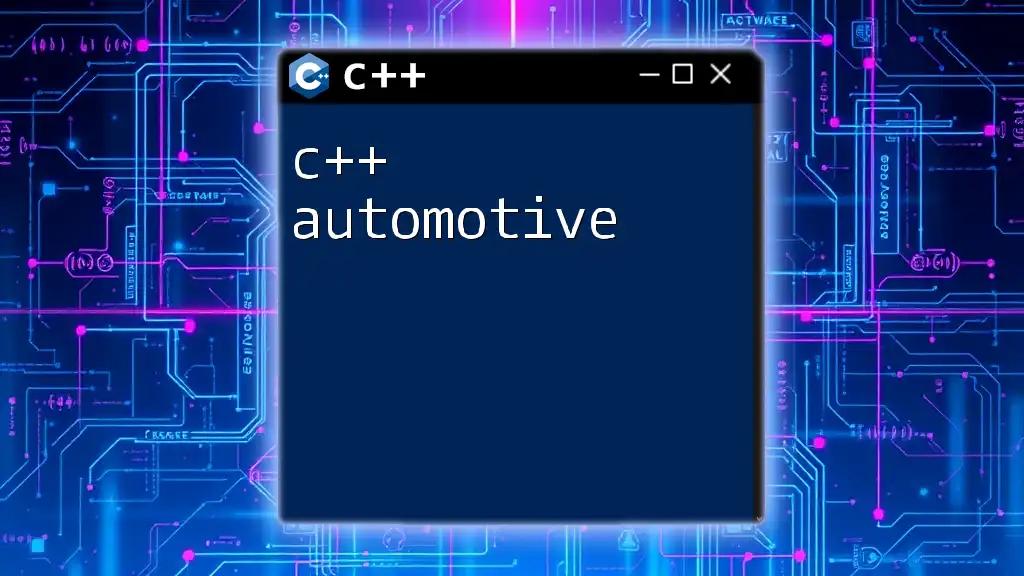
Summary of C++20 Features
C++20 marks a transformative moment in the language’s evolution with its cutting-edge features:
- Concepts elevate type safety and enhance template programming.
- Ranges make data manipulation more streamlined and effective.
- Coroutines simplify asynchronous programming, allowing developers to write cleaner and more controllable code.
- New standard library features like `std::format` improve usability and reduce common pitfalls in string handling.
- Modules enhance compilation processes and offer improved code organization.
- Improved `constexpr` enables complex compile-time evaluations, leading to more efficient programs.
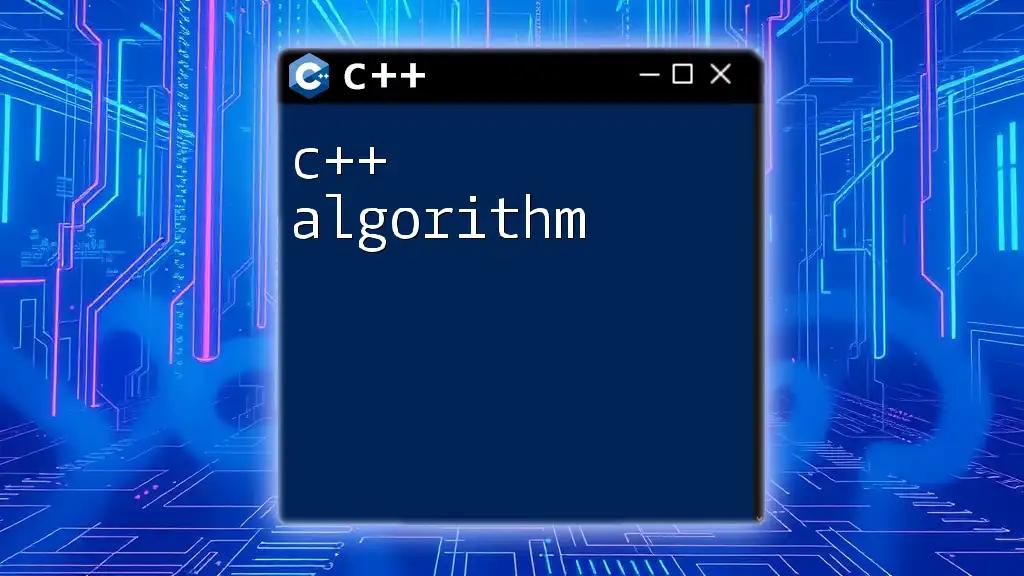
Conclusion
The introduction of C++20 heralds exciting advancements within the C++ ecosystem. By embracing these new features, developers can write more efficient, readable, and maintainable code. As technology continues to progress, it is crucial for programmers to adapt and learn.
Continuing your journey with C++ will not only strengthen your technical skills but will also empower you to tackle complex challenges in modern software development. As we look toward the future, remaining informed about updates and trends in C++ will ensure your growth as a proficient developer.

Additional Resources
To further your understanding of C++20, consider exploring the following:
- Books: Find comprehensive guides on C++20 and its features.
- Online Courses: Enroll in courses that delve into modern C++ programming techniques.
- C++ Communities: Join forums and groups to engage with fellow enthusiasts, share knowledge, and discuss best practices.
Engaging with the community will not only enhance your learning experience but will also keep you motivated to explore the vast potential C++2a has to offer.