C++17 is a version of the C++ programming language that introduced several enhancements and features, such as structured bindings, if constexpr, and parallel algorithms, to improve code efficiency and clarity.
Here's a code snippet demonstrating structured bindings in C++17:
#include <iostream>
#include <tuple>
std::tuple<int, double, std::string> getValues() {
return {42, 3.14, "Hello, C++17!"};
}
int main() {
auto [intVal, doubleVal, strVal] = getValues();
std::cout << "Integer: " << intVal << ", Double: " << doubleVal << ", String: " << strVal << std::endl;
return 0;
}
Overview of C++17
C++17 is a significant update to the C++ programming language, aiming to improve usability and performance while adding new features that enhance expressiveness. Building upon the foundations laid by C++11 and C++14, C++17 emphasizes convenience and increased efficiency. With these enhancements, developers can write cleaner, more efficient code that remains highly maintainable.
Important Features of C++17
C++17 introduces several key enhancements, including but not limited to structured bindings, inline variables, and a more robust standard library. These changes not only help streamline everyday programming tasks but also improve performance and safety in C++ applications.
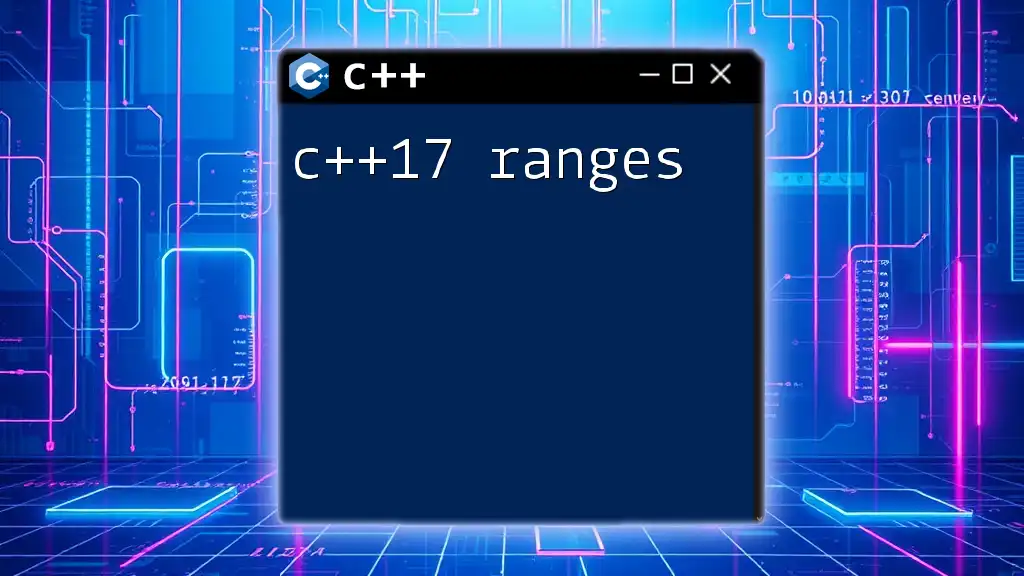
Core Language Features
Inline Variables
One of the standout features in C++17 is the introduction of inline variables. This feature allows variables to be declared inline, facilitating their use in header files without violating the One Definition Rule (ODR).
Use Cases: Inline variables prove especially beneficial in header files because they avoid the need for a separate definition in a source file. This reduces boilerplate code and potential linking issues.
Example:
inline int count = 0;
Structured Bindings
Structured bindings enable developers to unpack tuples, pairs, and arrays easily and intuitively. This feature simplifies code that previously required verbose handling of multiple variables.
Benefits: With structured bindings, code becomes cleaner and more readable. This leads to less error-prone code when dealing with aggregate types.
Example:
auto [x, y] = std::make_tuple(1, 2);
If with Initializer
C++17 allows for an if statement with an initializer, enhancing the control flow of code. This feature helps limit the scope of variables used within a conditional statement.
Example:
if (auto ptr = find_pointer(); ptr) {
// Use ptr
}
In this case, `ptr` only exists within the scope of the if statement, promoting better resource management.
Fold Expressions
Fold expressions are another powerful addition to C++17, simplifying the process of working with variadic templates. This feature allows developers to apply binary operators to parameter packs without creating complex recursion or iteration logic.
Code snippet illustrating fold expressions:
template<typename... Args>
auto sum(Args... args) {
return (... + args); // Fold expression: sums all args
}
This makes it simpler to perform operations on multiple values while maintaining concise syntax.
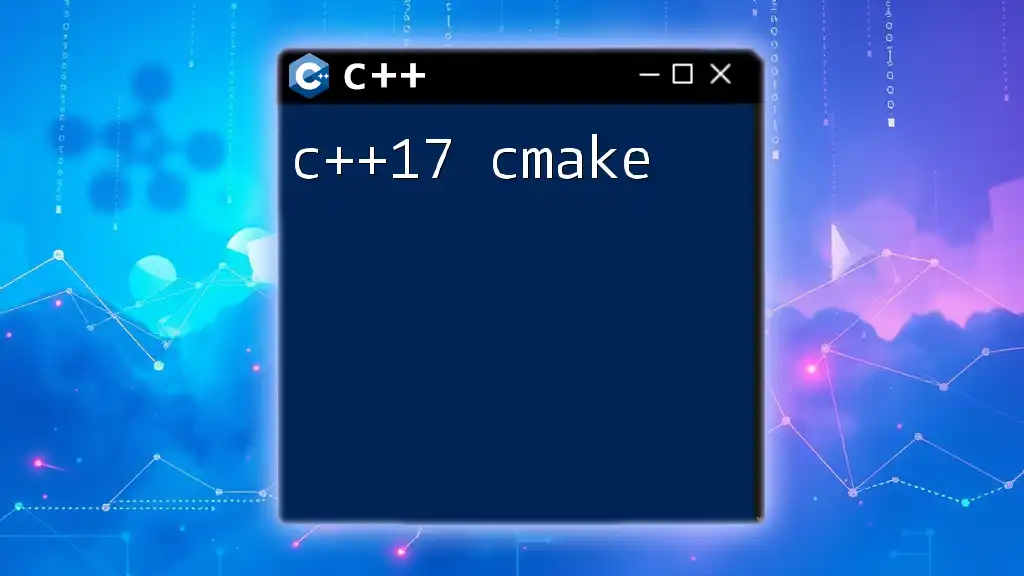
Standard Library Enhancements
std::optional
The introduction of std::optional allows developers to express the absence of a value more clearly. This represents a shift towards safer code, eliminating many issues associated with null pointers.
Use Case: Using std::optional encourages safer coding practices. By explicitly indicating when the absence of a value is possible, it reduces the risk of dereferencing null or uninitialized pointers.
Example:
std::optional<int> maybe_get_value() {
return {}; // No value
}
std::variant
std::variant provides a type-safe union data structure that can hold one of several specified types. This feature enhances flexibility while ensuring type safety, diverging from traditional unions that lack safety and clarity.
Example:
std::variant<int, std::string> v;
// v can now hold either an integer or a string
This allows developers to handle multiple data types without the overhead of type-checking at runtime.
std::any
With std::any, developers gain the ability to store any type of object, offering remarkable flexibility. This is particularly useful when the specific type is unknown at compile time, facilitating generic programming.
Example of storing various types in std::any:
std::any value = 42; // Store int
value = std::string("Hello, World!"); // Now store a string
This allows for greater adaptability in applications that require type erasure.
String View (std::string_view)
std::string_view is a lightweight alternative to std::string that provides performance benefits by avoiding unnecessary string copies. It refers to an existing string without ownership, enabling quick and efficient manipulations.
Example:
void print_length(std::string_view str) {
std::cout << str.length() << '\n';
}
This allows for faster computations and can be especially beneficial in performance-critical scenarios where string manipulations occur frequently.
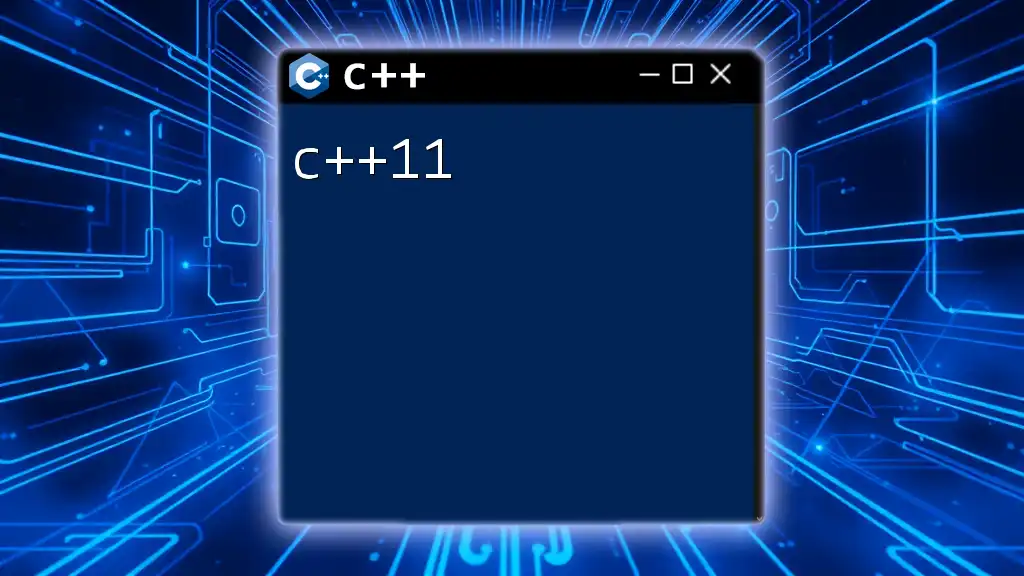
Parallel Algorithms
Introduction to Parallelism in C++17
C++17 introduces support for parallel algorithms, allowing developers to take advantage of multi-core architectures with ease. This represents a significant shift towards concurrent programming within the C++ standard library.
Example of a Parallel Algorithm
An example of utilizing parallel execution policies in C++17 is shown through the `std::for_each` algorithm, which can be executed in parallel.
Code snippet:
#include <execution>
std::for_each(std::execution::par, vec.begin(), vec.end(), [](const auto& v) {
// Perform operation
});
This approach helps maximize CPU efficiency by distributing operations across available threads.
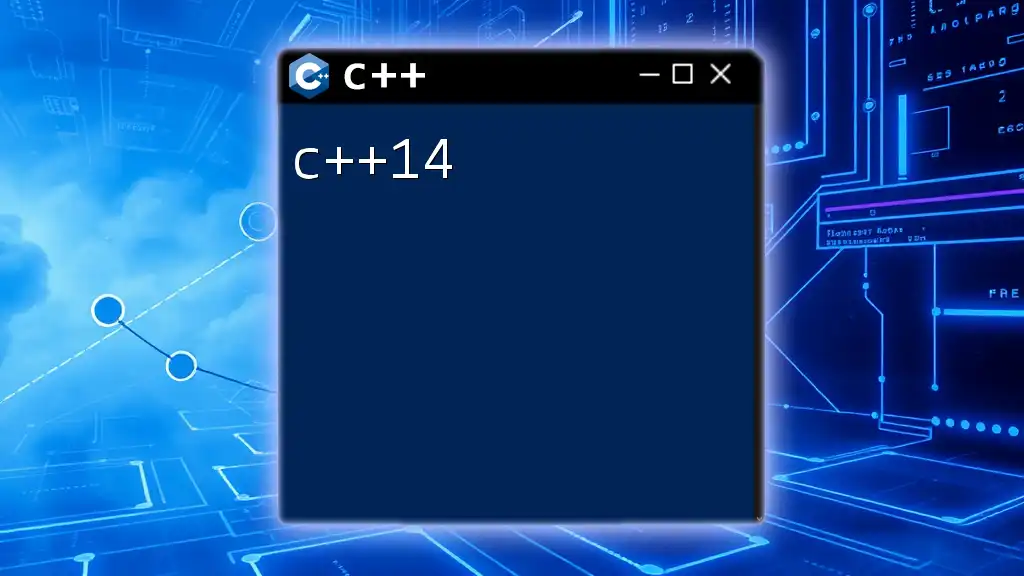
Filesystem Library
Overview of the Filesystem Library
The filesystem library standardizes file and directory manipulation, providing a comprehensive set of functionalities for working with files in a way that is both intuitive and safe.
Examples of Filesystem Usage
Developers can perform tasks such as creating, iterating, and deleting files and directories seamlessly using the filesystem library.
For instance, to check the existence of a path, the following code can be used:
#include <filesystem>
if (std::filesystem::exists(path)) {
// Path exists
}
This simplifies file handling and ensures that operations are done correctly, enhancing code robustness.
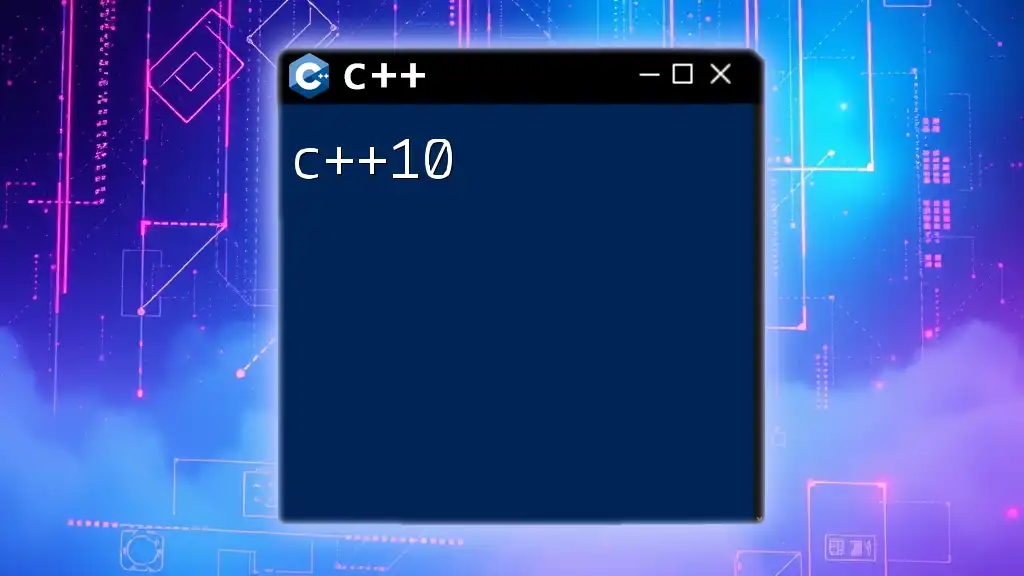
Conclusion
Recap of C++17 Features
C++17 brings forth a plethora of features that improve the language's expressiveness and safety. From inline variables to `std::variant`, each feature is designed to address specific scenarios developers encounter, empowering them to write cleaner and more efficient code.
Future of C++ and Upcoming Standards
As the C++ standard continues to evolve, looking toward C++20 and beyond can provide insights into the future of the language. Developers are encouraged to stay updated with the latest changes, as mastering C++17 will provide a solid foundation for taking on future C++ standards.
Resources for Further Learning
Engaging with community resources, such as online courses, forums, and books dedicated to C++, can further enhance understanding and provide deeper insights into practical applications.