C++11 introduced several new features, including auto type deduction, range-based for loops, and nullptr, enhancing code simplicity and performance.
Here's a code snippet demonstrating the use of auto and a range-based for loop:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
return 0;
}
Key Features of C++10
Auto Keyword
The auto keyword in C++10 allows for type deduction, simplifying the process of variable declaration. Previously, developers needed to explicitly state the type of a variable, which could lead to verbose and repetitive code. With auto, the compiler can determine the type of a variable based on its initializer.
Benefits: This not only reduces boilerplate code but also enhances readability, making it easier to understand the intent of the code.
Example:
auto x = 5; // x is inferred as int
auto y = 3.14; // y is inferred as double
In the above example, `x` and `y` are clearly defined without needing to state their types explicitly. This feature is especially useful when working with iterators and complex types.
Range-Based For Loop
C++10 introduced the range-based for loop, which provides a more straightforward way to iterate through collections, such as arrays and vectors. This enhancement eliminates the need for manual iterator management, reducing the risk of errors.
Advantages: The syntax is cleaner and easier to read, making the intent of the loop immediately obvious.
Example:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
In this instance, `num` will take on each value within the `numbers` vector, allowing for simple and efficient iteration.
nullptr
With the introduction of nullptr, C++10 provides a clear and type-safe way to represent null pointers. Before C++10, developers used `NULL`, which could lead to ambiguities between integer and pointer types.
The benefits of using `nullptr` are significant as it avoids type conversion issues, thereby increasing the safety of code.
Example:
int* ptr = nullptr; // safer than using NULL
This adds clarity, indicating that `ptr` is intended to point to no valid memory location.
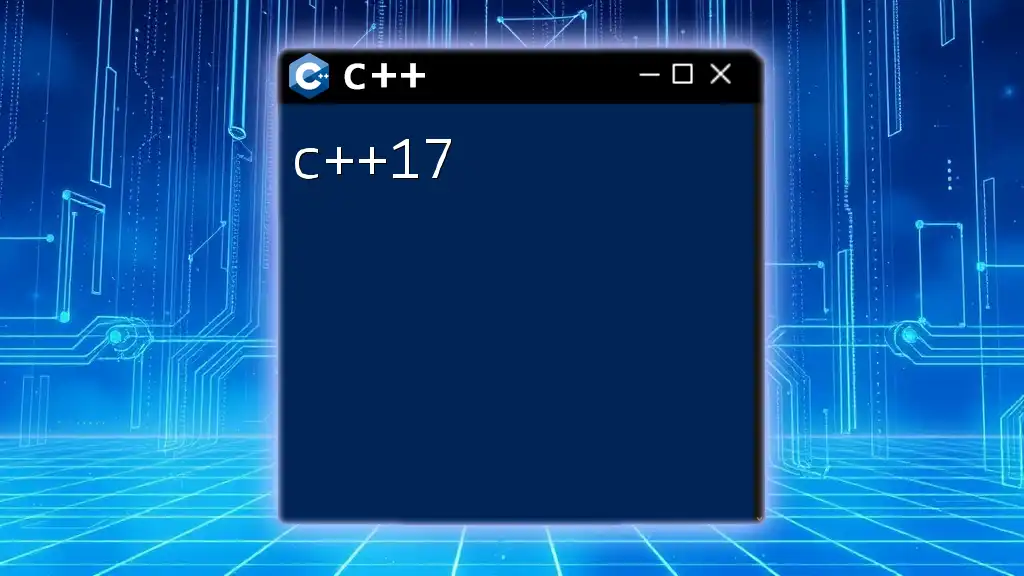
Enhancements in C++10
Type Traits
Type traits are compile-time templates that provide information about types. C++10 introduced these templates, which are instrumental in template programming. They enable developers to write generic code that adapts depending on type characteristics.
Commonly used type traits include `is_integral`, `is_same`, and many others. They enable compile-time checks on types, enhancing safety and allowing for specialization of templates.
Example:
#include <type_traits>
static_assert(std::is_integral<int>::value, "int should be integral");
The `static_assert` statement checks whether `int` is an integral type at compile time. If the assertion fails, the code will not compile, promoting type safety.
Static Assertions
static_assert provides compile-time assertions that allow developers to enforce conditions in their code. This means that if a condition fails, the compilation will fail, effectively preventing erroneous code from executing.
Use Cases: This feature is particularly useful in template programming where you want to validate template parameters.
Example:
template<typename T>
void check() {
static_assert(sizeof(T) == 4, "T must be 4 bytes");
}
In this example, if the size of `T` does not equal 4 bytes, the code will not compile, ensuring that only allowed types can be used.
Lambda Expressions
C++10 brought significant usability improvements with lambda expressions. These allow for the creation of anonymous functions that can be defined inline, resulting in cleaner and more concise code.
Advantages: Lambdas can capture variables from their surrounding context, making them very powerful while minimizing the surrounding boilerplate code. They are highly useful for operations like sorting and transforming data.
Example:
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Outputs 8
In this case, a simple addition function is defined using a lambda, which can be directly used without the need for a separate function definition.
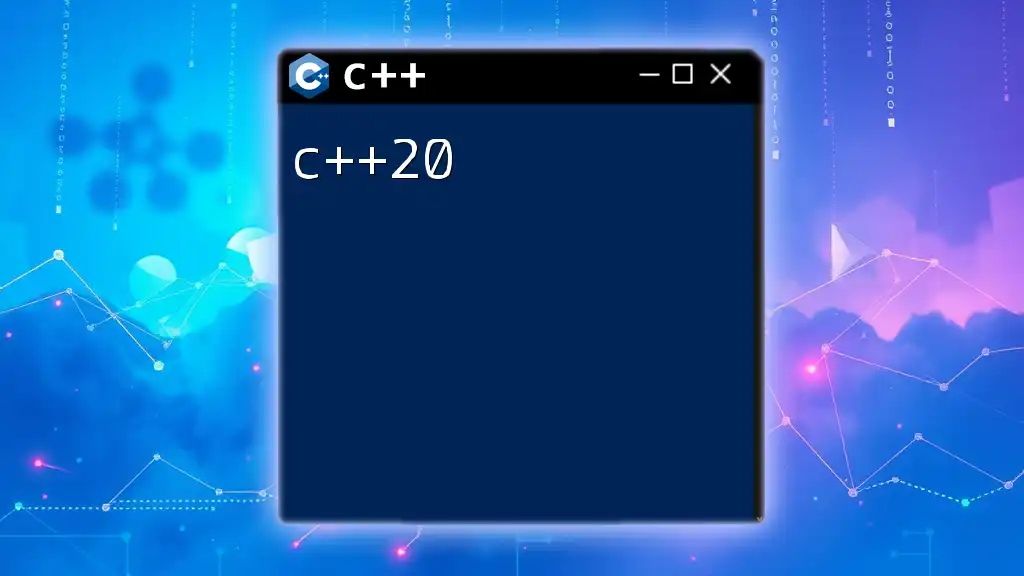
New Standard Library Features
Concurrency Support
C++10 improved concurrency support by introducing std::thread, allowing developers to create and manage threads more easily. This is a pivotal enhancement for writing efficient multi-threaded programs.
Developers can spawn threads to perform operations concurrently, leading to better resource utilization and faster execution of programs.
Example:
void threadFunction() {
std::cout << "Thread is running\n";
}
std::thread t(threadFunction);
t.join();
In this example, a new thread is created to execute the `threadFunction`, demonstrating the ease with which threads can be managed in C++10.
Other Library Additions
C++10 also introduced several new library features, such as `std::array`, `std::forward`, and various type traits. These additions serve to enhance code efficiency, making data structures and algorithms easier to implement and manage.
Example:
std::array<int, 5> arr = {1, 2, 3, 4, 5};
The `std::array` provides a container that encapsulates fixed-size arrays. This allows for more robust functionality while maintaining the easy syntax that developers expect from modern C++.
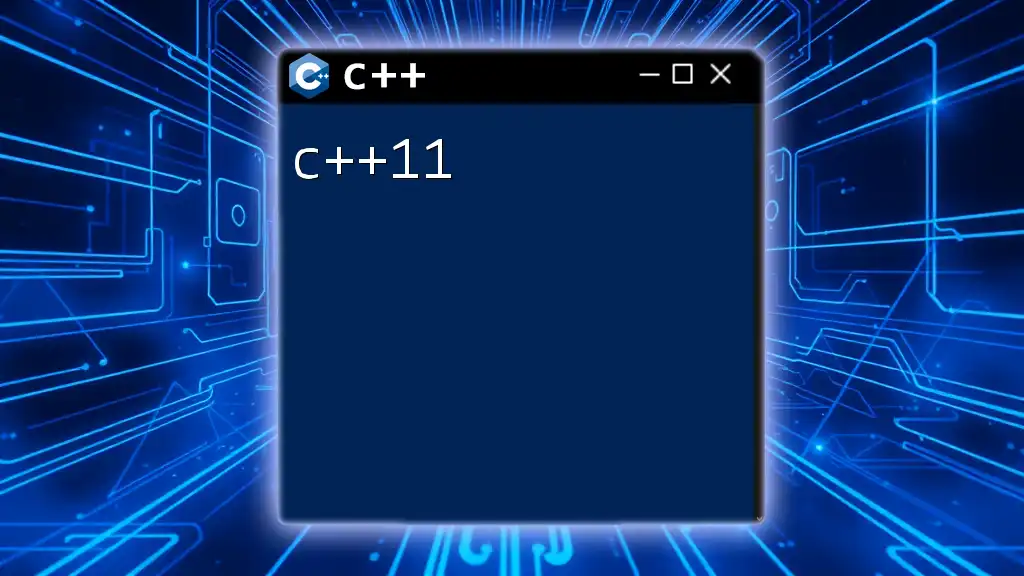
Compiler Support for C++10
Most modern compilers, such as GCC, Clang, and MSVC, provide support for C++10. Setting up a development environment with C++10 capabilities is straightforward, typically requiring just a compiler update or configuration change.
However, industry adherence to the standard can vary. Developers may encounter compatibility issues, particularly with older codebases. Knowing how to check compiler support and ensure that environments are correctly set up is critical for smooth development.
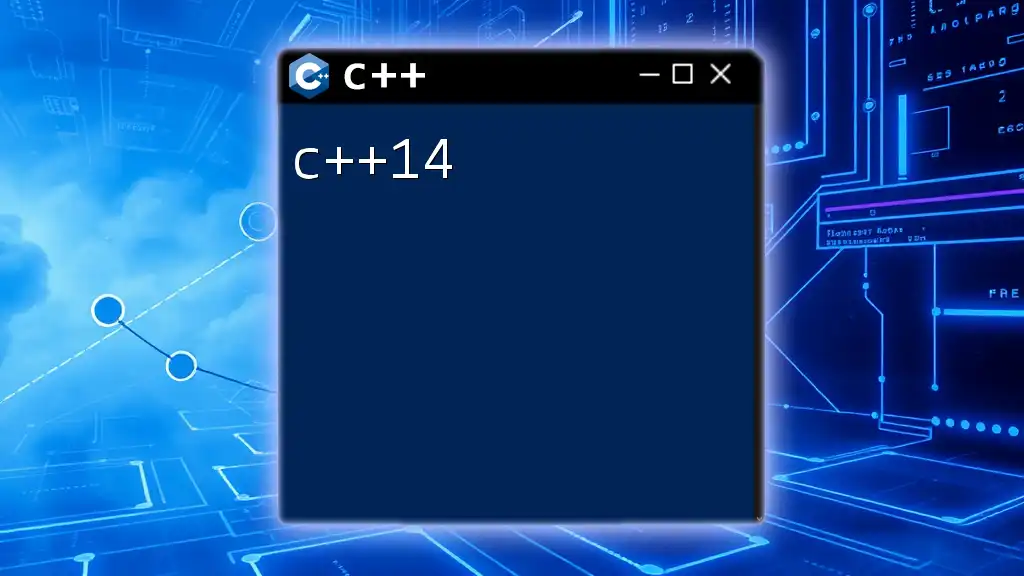
Conclusion
C++10 marked a significant milestone in the evolution of the C++ programming language, introducing features that enhance code clarity, safety, and usability. Features like the auto keyword, range-based for loops, new threading facilities, and lambdas provide programmers with powerful tools to write efficient, clean, and maintainable code.
As developers embrace these innovations, understanding C++10 becomes essential for leveraging its full potential in modern software development. The advancements of C++10 lay the groundwork for further enhancements in future standards, promising even greater capabilities for the C++ community.
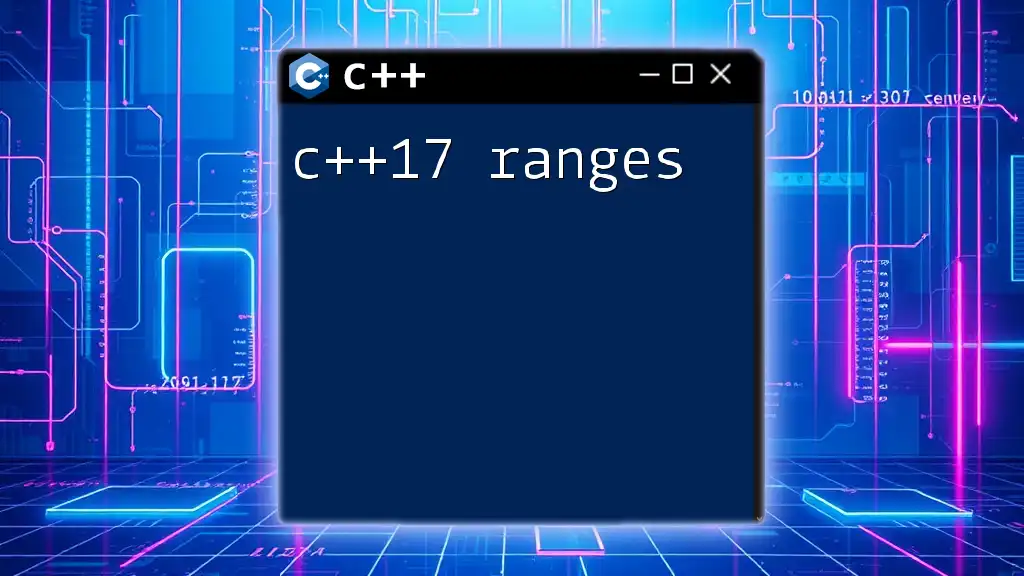
Additional Resources
For those looking to deepen their understanding of C++10, numerous online resources are available, including official documentation, tutorials, and community forums. Consider exploring these materials to further your knowledge and connection with other C++ developers.
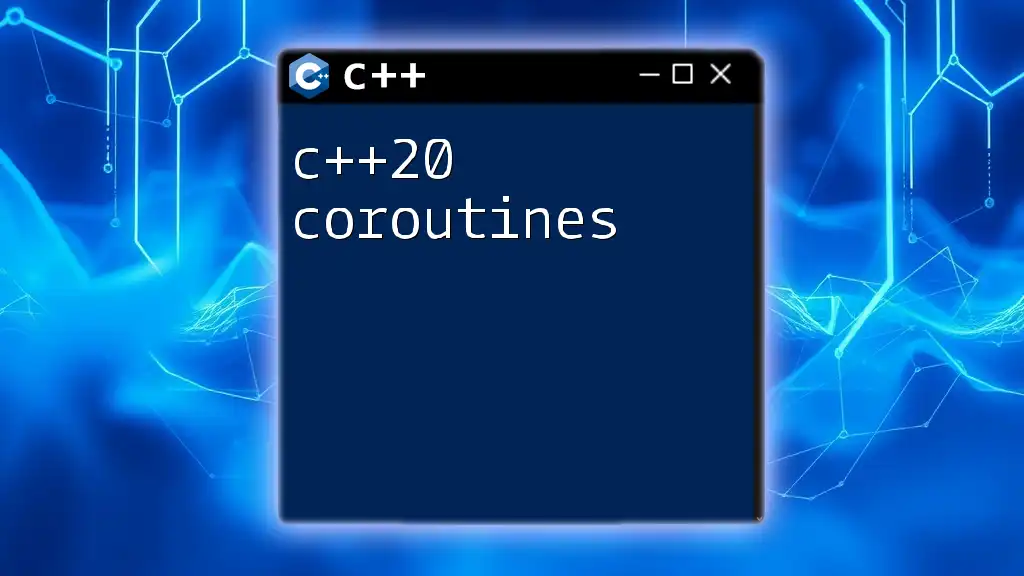
Call to Action
Stay updated with the latest programming insights, tips, and tricks by subscribing to our newsletters or joining our community forums. Share your experiences using C++10 features in the comments below, as we build a vibrant community of C++ enthusiasts together!