In C++, the notation `0.0f` represents a floating-point literal that is specifically of type `float`, indicating that the number is a floating-point number with a precision of 32 bits.
Here’s an example of how you might use `0.0f` in code:
#include <iostream>
int main() {
float myNumber = 0.0f; // Initializes a float variable to 0.0
std::cout << "The value is: " << myNumber << std::endl;
return 0;
}
Understanding Floating-Point Numbers in C++
What are Floating-Point Numbers?
In C++, floating-point numbers are used to represent real numbers, which can have decimal places. This representation allows for both very large and very small numbers to be approximated with a certain level of precision. Floating-point representation is crucial in scenarios needing fractional values, such as calculations in scientific computations, graphics, and finance.
The 'float' Data Type
The `float` data type in C++ is designed to store floating-point numbers. It typically occupies 4 bytes of memory, which results in a precision of about 7 decimal digits. It is important to note that `float` numbers consume less memory compared to `double` (which allocates 8 bytes and provides approximately 15 decimal digits of precision). Therefore, using `float` can enhance performance when numerous floating-point operations occur, especially in resource-constrained environments such as embedded systems or in large-scale simulations.
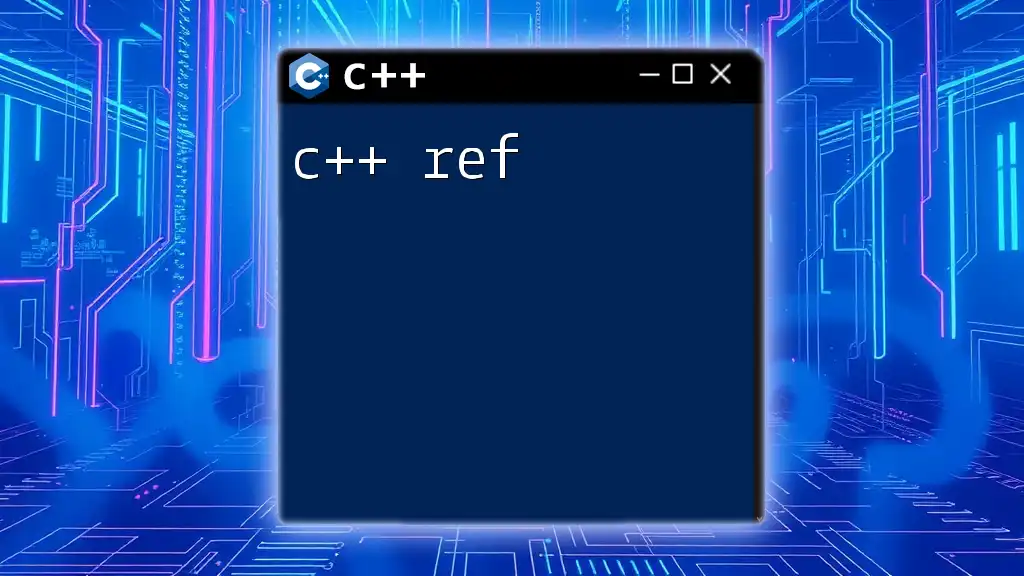
The Significance of 0.0f
What Does 0.0f Represent?
The literal `0.0f` explicitly indicates a floating-point value of zero. The suffix `f` denotes that this value should be treated as a `float` data type, rather than the default `double`. This distinction is critical, as using the proper type can save memory and prevent implicitly converting between types, which can lead to unintended results or performance overhead.
0.0f vs 0.0 vs 0
When programming in C++, it's essential to understand the difference between `0.0f`, `0.0`, and `0`:
- 0 is an integer literal with no decimal component.
- 0.0 is a double literal, which has higher precision than floats.
- 0.0f is explicitly a float literal, ensuring the compiler treats it as a float.
Using `0.0f` instead of `0.0` or `0` can help prevent potential bugs in floating-point arithmetic and enhance the clarity of your code.
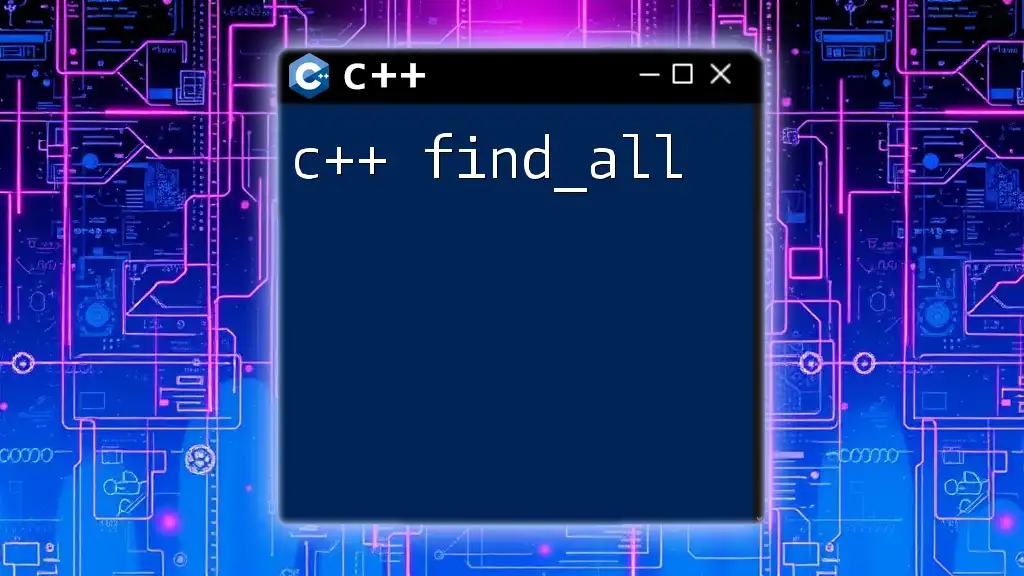
Practical Applications of 0.0f
Initialization of Floating-Point Variables
Initializing variables with `0.0f` is a best practice in C++. It signifies that the variable is meant to hold a floating-point value. For instance, a float variable can be initialized as follows:
float value = 0.0f;
This initialization makes it clear to anyone reading the code that `value` is a float.
Use in Mathematical Operations
When performing calculations, using `0.0f` can often simplify your expressions and enhance readability. For example, consider the following addition of `0.0f`:
float a = 5.0f;
float result = a + 0.0f; // result is 5.0f
Here, `0.0f` does not alter the value of `a`, reinforcing the concept of identity in addition.
Conditional Statements and 0.0f
In conditions, comparing values to `0.0f` is common, but it requires careful handling due to floating-point precision issues. The following IF statement checks whether `value` equals zero:
if (value == 0.0f) {
// Code to execute if value is zero
}
However, directly comparing floating-point numbers may lead to unexpected behavior, especially due to precision errors.
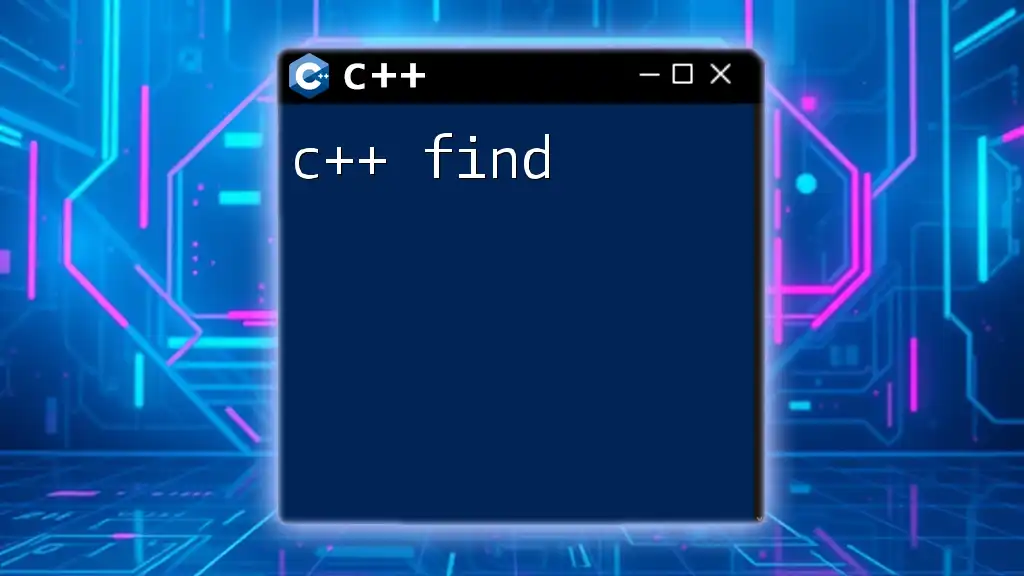
Common Mistakes with 0.0f
Implicit Conversions
C++ automatically converts `float` values to `double` in mixed-type expressions. For example:
double result = 0.0f; // Implicit conversion from float to double
While this conversion is often harmless, relying on it can lead to code that is less portable and understandable. Always use the correct type where possible to avoid ambiguity.
Floating-Point Precision Issues
When working with floating-point numbers, be wary of precision issues, particularly when performing arithmetic operations. For instance:
float a = 0.1f + 0.2f; // Usually does not equal 0.3f exactly
Due to how floating-point numbers are represented in binary, the result of `a` may not be precisely `0.3f`, which can lead to errors in calculations.
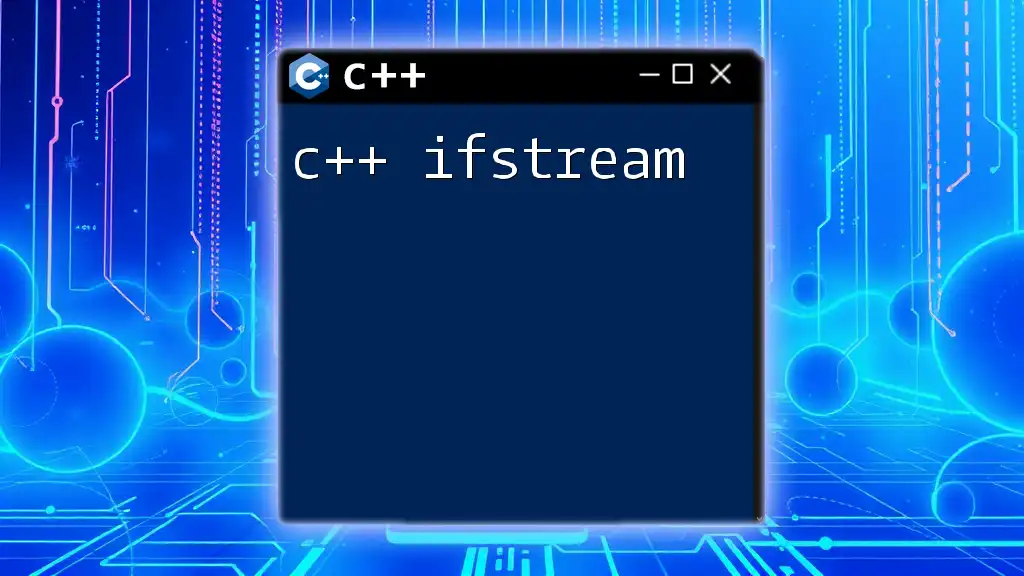
Best Practices with 0.0f
When to Use `0.0f`
Using `0.0f` is highly recommended in contexts where floating-point precision is necessary. For instance, initializing weights or threshold values in algorithms that rely on floating-point calculations. Having the proper type can prevent unforeseen issues related to memory conversion.
Handling Floating-Point Errors
When comparing floating-point values, it is best practice to avoid checking equality directly. Instead, use an epsilon value, which provides a small tolerance for errors in calculations:
const float epsilon = 0.0001f;
if (abs(a - 0.0f) < epsilon) {
// Treat as equal to zero
}
This approach significantly minimizes issues stemming from precision errors, making your code more robust and reliable.
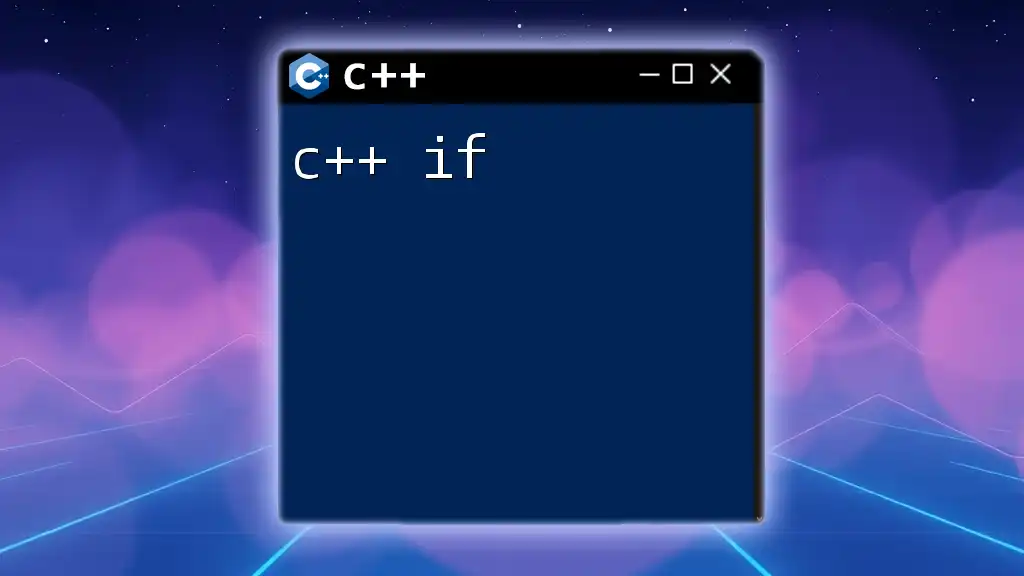
Conclusion
Understanding `c++ 0.0f` and its implications is essential for robust C++ programming. By utilizing float literals appropriately, developers can optimize performance, reduce potential bugs, and create clearer code. Mastering floating-point arithmetic and maintaining precision will further enhance your coding skills and the quality of your software. Embrace these principles, and keep exploring the vast possibilities of C++!
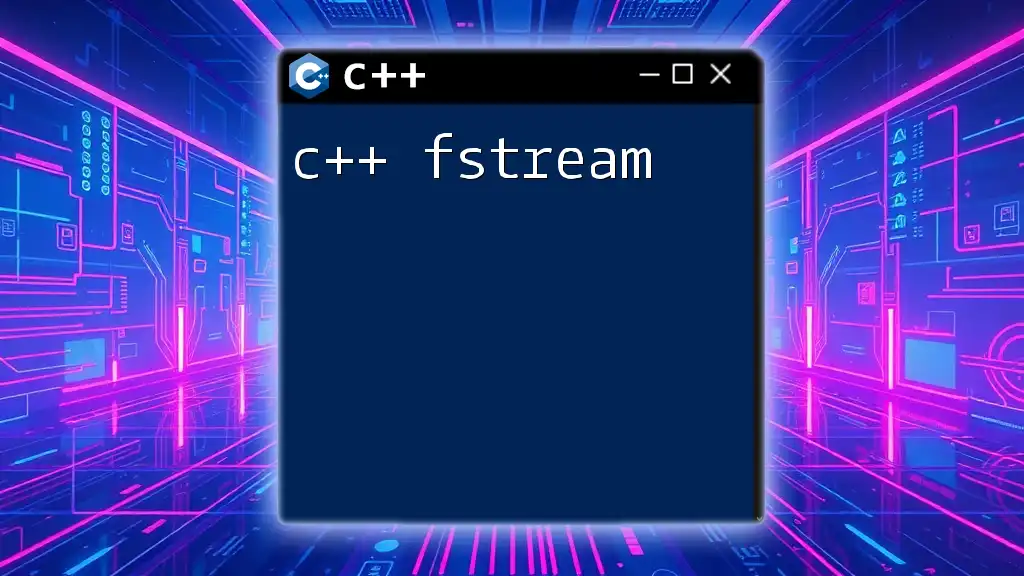
Further Reading
To deepen your knowledge on C++ and floating-point arithmetic, consider exploring trusted resources, online tutorials, and documentation that cover these topics in more detail. Congratulations on taking the first steps toward mastering C++!