C++ 2015, commonly referred to as C++11 with further enhancements in the C++14 standard, introduced features like auto type deduction and lambda expressions to simplify programming and increase code efficiency.
Here's an example showcasing the usage of `auto` and a lambda function:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto sum = 0;
std::for_each(numbers.begin(), numbers.end(), [&sum](int n) { sum += n; });
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Key Features of C++ 2015
Lambda Expressions Enhancements
Lambda expressions are anonymous functions that allow you to define inline function behavior. They greatly simplify the syntax for writing function objects, helping your code become more concise and easier to read. In C++ 2015, lambda expressions received enhancements that add new capabilities.
Capture Expressions
You can capture variables from the surrounding scope into your lambda expression. This can be done by value or by reference. Capturing by value creates a copy of the variable, while capturing by reference allows you to access the original variable directly.
Example Code Snippet:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers {1, 2, 3, 4, 5};
int factor = 2;
std::for_each(numbers.begin(), numbers.end(), [&factor](int &n) {
n *= factor; // Capture factor by reference
});
for (const auto& n : numbers) {
std::cout << n << " ";
} // Output: 2 4 6 8 10
return 0;
}
Generalized Attribute Annotations
C++ 2015 introduced a new syntax for attribute annotations, making it easier for developers to convey additional information about their code to both compilers and programmers.
Usage of [[ ... ]] Syntax
The new syntax involves wrapping attributes in double square brackets. These attributes can be assertions, optimizations, and other instructions that provide hints to the compiler.
Example Code Snippet:
[[nodiscard]] int compute() {
return 42; // This indicates the return value should not be discarded
}
This `[[nodiscard]]` attribute signals to users of the function that ignoring the return value may lead to errors or unintended behavior.
std::make_unique
Memory management is vital in C++. With the introduction of `std::make_unique`, developers can now create `std::unique_ptr` in a safer manner, preventing potential memory leaks.
Benefits of Using make_unique
Using `std::make_unique` eliminates the risk of forgetting to release memory, as it ensures proper management of dynamically allocated memory.
Example Code Snippet:
#include <memory>
class MyClass {
public:
MyClass() { /* Constructor logic */ }
~MyClass() { /* Destructor logic */ }
};
int main() {
auto ptr = std::make_unique<MyClass>(); // Automatically managed
// No need to manually delete, safety ensured
return 0;
}
constexpr Enhancements
`constexpr` allows functions and variables to be evaluated at compile-time, leading to performance improvements.
Extended Capabilities
C++ 2015 extended the capabilities of `constexpr`, enabling more complex functions to be declared as `constexpr`, which allows for better optimization.
Example Code Snippet:
constexpr int square(int x) {
return x * x; // Can be evaluated at compile-time
}
int main() {
constexpr int squareOfFive = square(5); // Evaluated at compile-time
return 0;
}
This feature is particularly useful for defining constants that can be computed at compile-time rather than at runtime.
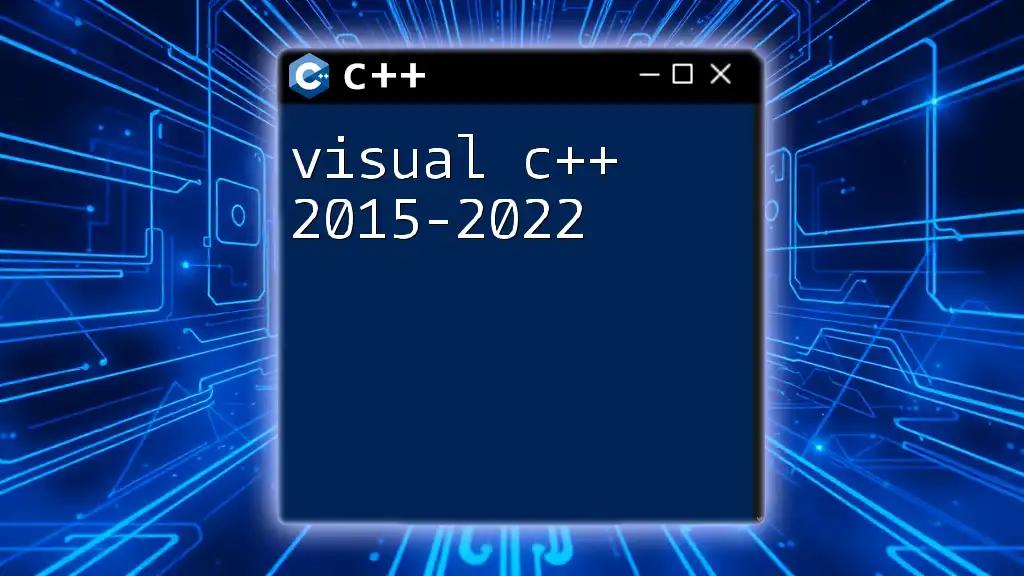
Library Features in C++ 2015
std::optional
`std::optional` represents an object that may or may not hold a value. This is valuable for indicating "no value" states without using special values like `nullptr` or sentinel objects.
Use Cases and Examples
`std::optional` can simplify function return values and improve code clarity. Instead of using pointers or exceptions, returning an `std::optional` makes it clear whether a function's call has yielded a valid result.
Example Code Snippet:
#include <optional>
#include <iostream>
std::optional<int> findValue(bool found) {
if (found) {
return 42;
} else {
return std::nullopt; // No value present
}
}
int main() {
auto value = findValue(true);
if (value) {
std::cout << "Found value: " << *value << std::endl;
} else {
std::cout << "Value not found." << std::endl;
}
return 0;
}
std::any
`std::any` is a type-safe container for single values of any type, allowing type erasure. This provides flexibility in design without losing type safety.
Practical Examples of std::any
By using `std::any`, developers can hold any type of object without explicit type conversions, thus making it easy to create generic containers.
Example Code Snippet:
#include <any>
#include <iostream>
int main() {
std::any a = 42;
std::cout << std::any_cast<int>(a) << std::endl; // Outputs: 42
a = std::string("Hello");
std::cout << std::any_cast<std::string>(a) << std::endl; // Outputs: Hello
return 0;
}
std::variant
`std::variant` is a type-safe union that can hold one of several predefined types, making it a great option for scenarios where a variable may be one of multiple types.
How to Use std::variant
When using `std::variant`, developers can switch easily between types while ensuring type safety.
Example Code Snippet:
#include <variant>
#include <iostream>
std::variant<int, float> var;
int main() {
var = 10; // Holds int
std::cout << std::get<int>(var) << std::endl; // Outputs: 10
var = 5.5f; // Now holds float
std::cout << std::get<float>(var) << std::endl; // Outputs: 5.5
return 0;
}

Tooling Improvements in C++ 2015
C++ 2015 also brought significant improvement in tooling, making it easier for developers to write and debug their applications.
Better Compiler Support
Most modern compilers have adopted better support for C++ 2015 features. This implies that developers can work with cutting-edge features easily, enhancing their productivity.
Static Analysis and Debugging Tools
Using tools such as `clang-tidy` or `cppcheck` can help identify potential issues in C++ codebases. These tools improve code quality by analyzing your code before execution.

Best Practices for C++ 2015
Modern C++ Principles
When developing in C++ 2015, it's essential to emphasize code safety and efficiency. Practice using smart pointers generously; they help with resource management and mitigating memory leaks.
Staying Up-To-Date
The C++ landscape is constantly evolving. Engaging with a variety of resources—such as books, online tutorials, and forums—ensures your skills remain sharp.
Continuous Learning Resources
There are numerous books and online courses available that cover C++ 2015 and beyond. Engaging with these resources will deepen your understanding and skill set.
Community Engagement
Participating in C++ forums, contributing to open-source projects, and joining local meetups fosters personal growth and provides networking opportunities.

Conclusion
In summary, C++ 2015 introduced a plethora of powerful features that improved both the language itself and the overall efficiency of software development. By leveraging these advancements, programmers can write safer, more efficient, and clearer code. Keeping abreast of future developments and augmenting one's skills through continuous learning will ensure success in the dynamic world of C++.
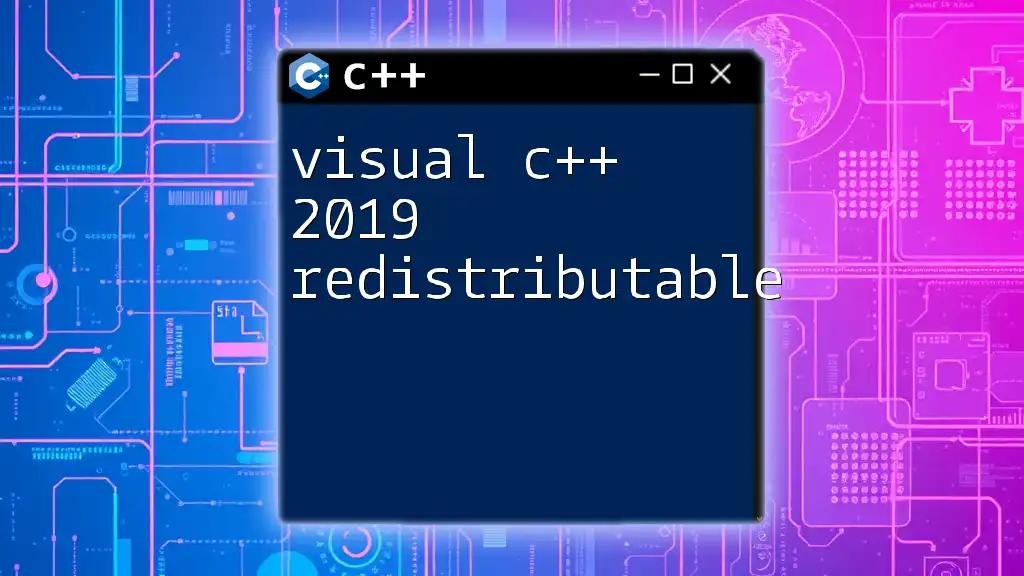
Additional Resources
Engaging with the official C++ documentation and reputable books on modern C++ are great ways to deepen your understanding. Being part of community discussions can also provide valuable insights into best practices and new techniques.