The C++ `mt19937` is a pseudorandom number generator from the Mersenne Twister family, which is widely used for generating high-quality random numbers.
#include <iostream>
#include <random>
int main() {
std::mt19937 generator(std::random_device{}()); // Initialize generator with a random seed
std::uniform_int_distribution<int> distribution(1, 100); // Define the range
int randomNumber = distribution(generator); // Generate a random number
std::cout << "Random Number: " << randomNumber << std::endl;
return 0;
}
What is mt19937?
mt19937 stands for "Mersenne Twister 19937" and is an efficient, widely-used algorithm for generating pseudo-random numbers. Developed by Makoto Matsumoto and Takuji Nishimura in 1997, it is known for its long period (2^19937-1) and the high-quality randomness it produces. Unlike simpler random number generators, like linear congruential generators, mt19937 is designed to avoid patterns and provides a better uniform distribution of values. This makes it an excellent choice for applications that require random sampling, simulations, and games.
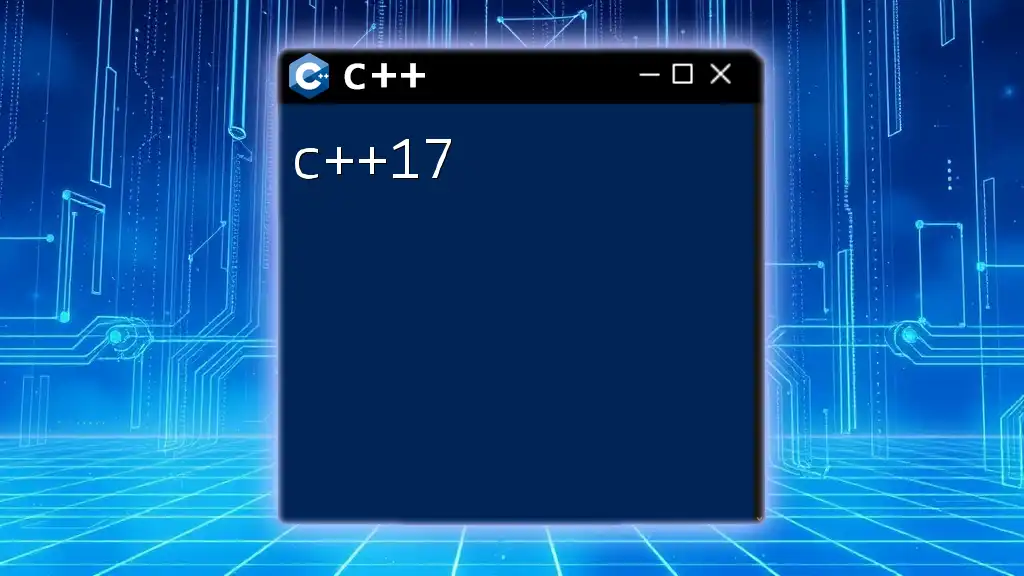
Setting Up mt19937 in Your C++ Environment
Including the Necessary Header
To use mt19937, you need to include the `<random>` header, which provides tools for random number generation. This ensures that you have access to the necessary classes and functions.
#include <random>
Creating an mt19937 Generator Instance
After including the header, you can create an instance of the mt19937 generator. By default, the generator uses a specific initial state, but it's crucial to note that the quality of randomness can significantly improve with an appropriate seed.
std::mt19937 rng; // default constructor
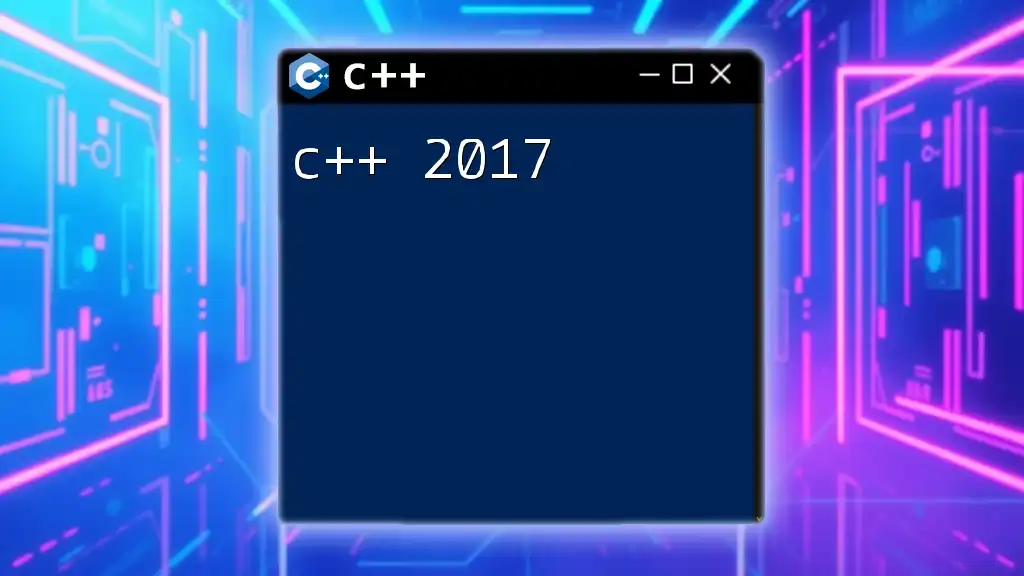
How to Generate Random Numbers with mt19937
Seeding the Generator
Seeding the generator properly is essential to produce a different sequence of random numbers each time the program runs. Failing to seed the generator or using the same seed can lead to repeating sequences of numbers, which may not be desirable in many applications.
A common approach to seed the generator is by using the current system time:
rng.seed(std::chrono::system_clock::now().time_since_epoch().count());
Generating Random Integers
To generate random integers, you can use `std::uniform_int_distribution`, which allows you to specify a range. This distribution ensures that every integer within the specified range has an equal chance of being selected.
Here’s how you can create a random integer between 1 and 100:
std::uniform_int_distribution<int> dist(1, 100);
int random_number = dist(rng);
In this code snippet, `dist(rng)` produces a random integer every time it is called.
Generating Random Floating-Point Numbers
Similar to generating integers, you can also generate random floating-point numbers using `std::uniform_real_distribution`. This allows you to specify a range for the floating-point numbers. For instance, to generate a random number between 0.0 and 1.0:
std::uniform_real_distribution<double> dist(0.0, 1.0);
double random_double = dist(rng);
This will produce a floating-point number each time you call `dist(rng)`, with a uniform distribution across the specified range.
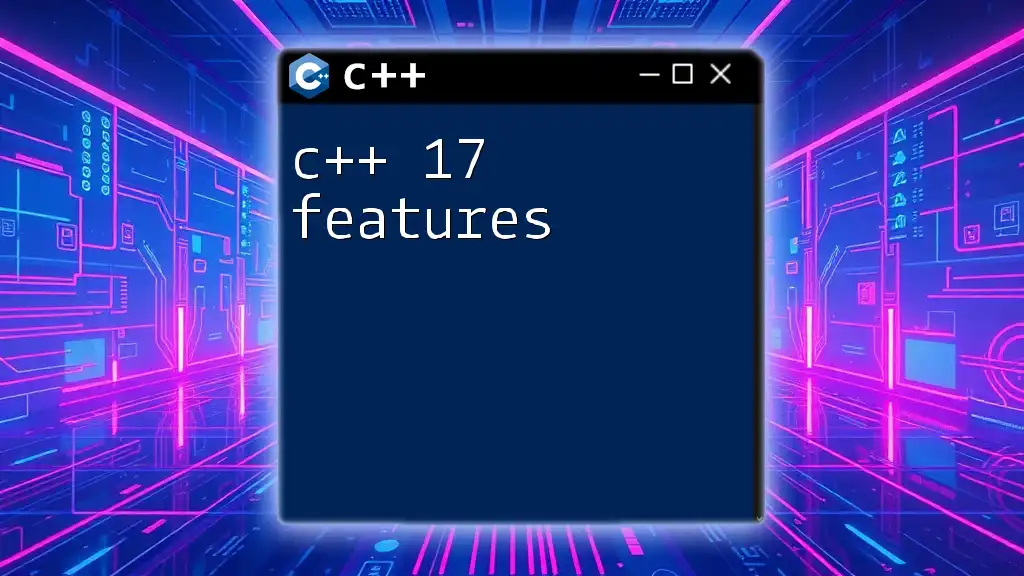
Using mt19937 for Different Applications
Simulating Games
mt19937 is particularly useful in game development, where random outcomes are essential. For example, simulating a dice roll can be easily accomplished:
for(int i = 0; i < 10; ++i) {
int dice_roll = dist(rng);
std::cout << "Dice Roll: " << dice_roll << std::endl;
}
In this loop, it rolls a dice ten times, generating random numbers between 1 and 100. You can easily adjust the distribution to match typical dice values (1-6, for instance).
Statistical Sampling
The mt19937 algorithm is also invaluable in statistical analyses where randomness is required to create samples from larger datasets. By generating random indices or selections, you can draw meaningful samples for further analysis.
Here is a simple code example to select random samples from a population:
const int population_size = 50;
std::vector<int> population(population_size);
std::iota(population.begin(), population.end(), 1); // Fill with 1 to 50
std::uniform_int_distribution<int> sample_dist(0, population_size - 1);
for(int i = 0; i < 10; ++i) {
int sample_index = sample_dist(rng);
std::cout << "Sampled Value: " << population[sample_index] << std::endl;
}
In this snippet, we fill a vector with numbers from 1 to 50 and sample ten values at random.
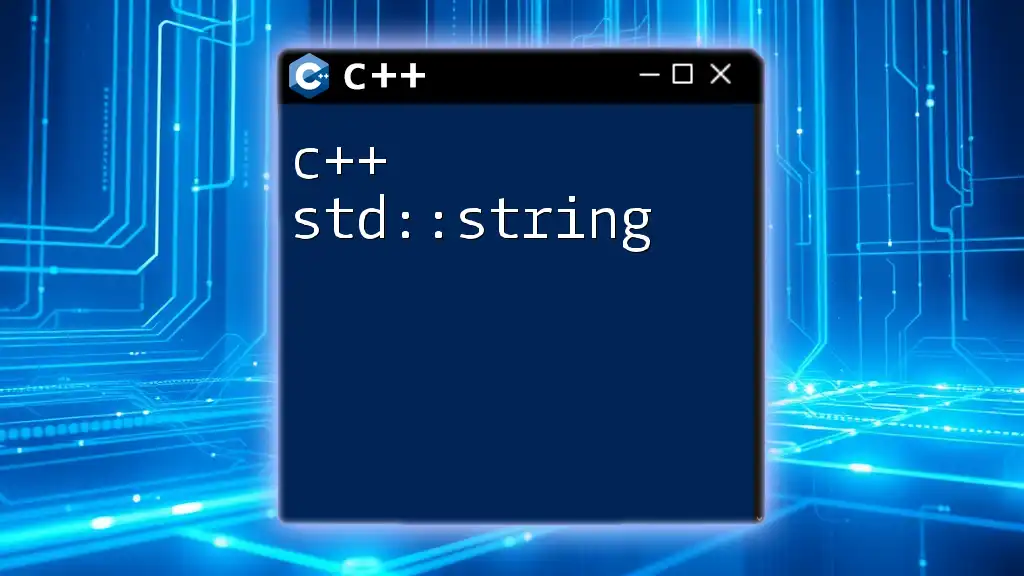
Best Practices for Using mt19937
Choosing the Right Seed
Choosing a seed is crucial. For most applications, you want the seed to be dynamic, such as based on time or hardware details. A constant seed will yield the same sequence of numbers across executions, which defeats the purpose of randomness.
Avoiding Patterns in Randomness
While mt19937 is designed to minimize patterns, programmers should always test their random number generation to ensure the output meets randomness requirements. Various statistical tests are available to validate the quality of randomness.
Thread Safety
When working in multi-threaded environments, take care with instances of mt19937. Each thread should ideally create its own instance of the generator to avoid contention and ensure that randomness remains unpredictable.
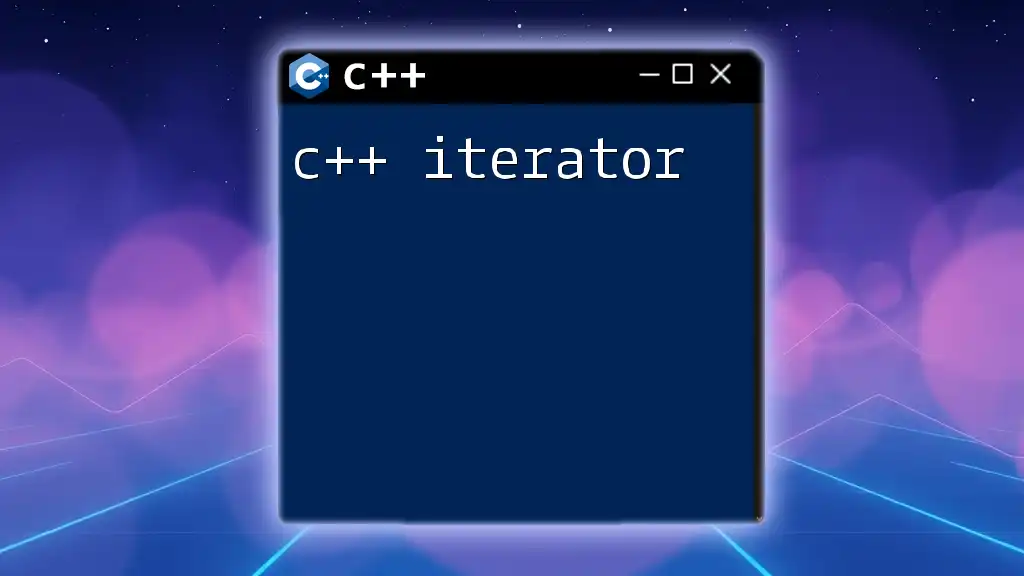
Debugging Common Issues with mt19937
Common pitfalls with mt19937 include:
- Not Seeding: Forgetting to seed your generator may yield the same output across multiple runs.
- Using Global Instances: Global instances may give rise to unintended behavior, especially in concurrent scenarios.
Ensure to troubleshoot these issues by checking your seeding methodology and limiting the scope of generator instances within specific functions or threads.
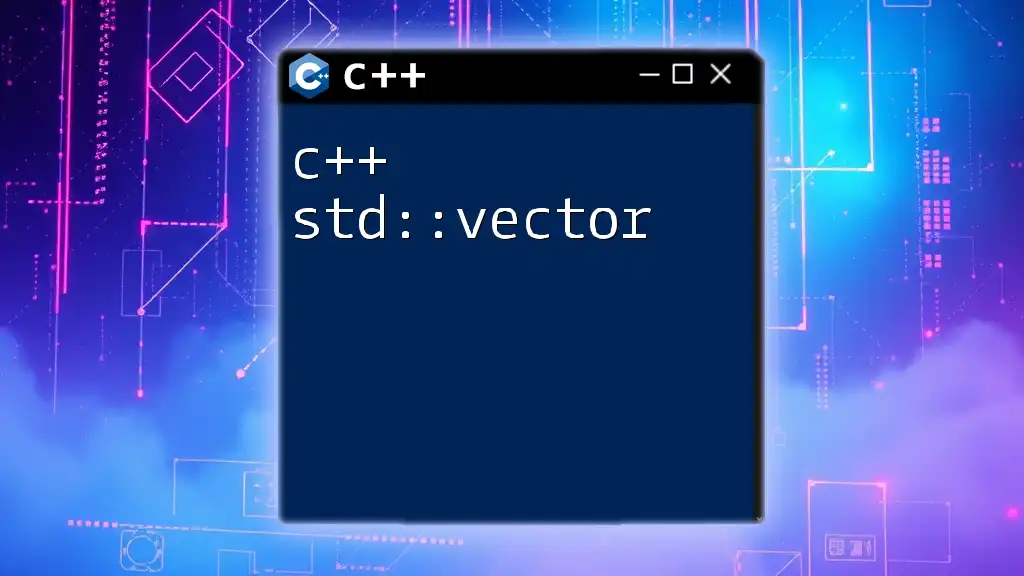
Conclusion
In summary, mt19937 is a powerful tool for generating high-quality random numbers in C++. It is essential for simulations, games, statistical samples, and many other applications. By understanding its proper setup, usage, and best practices, you can leverage its functionalities to enhance the quality and efficiency of your projects. Don't hesitate to experiment and integrate mt19937 into your future developments to take advantage of its effective random number generation capabilities.
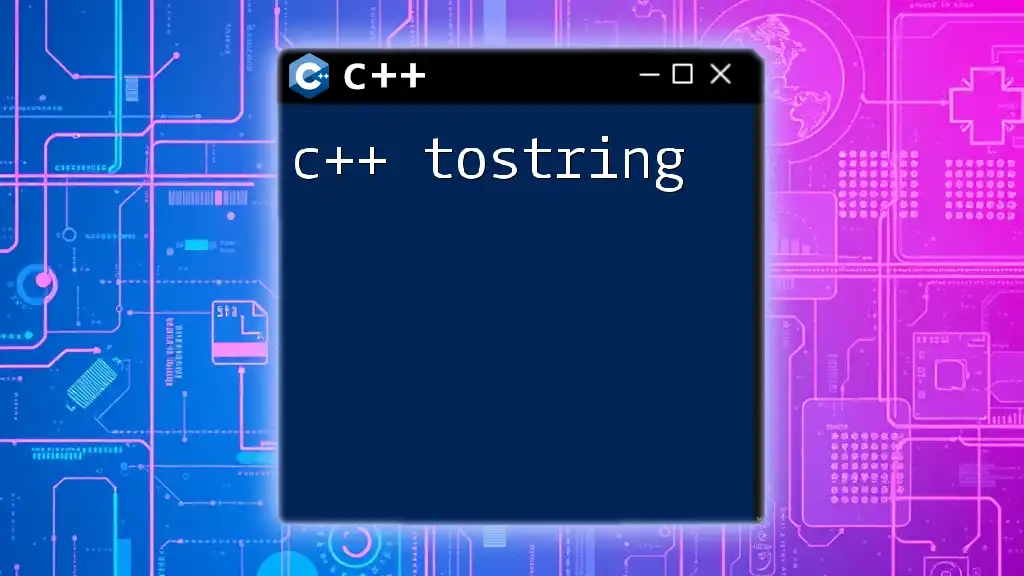
Additional Resources
For deeper knowledge on c++ mt19937, consider consulting the official `<random>` library documentation, exploring books dedicated to C++ programming, or joining online platforms and forums to connect with the programming community for further insights and support.