C++ W3 refers to the application of C++ programming language concepts and commands as taught and demonstrated on platforms like W3Schools, emphasizing quick, efficient learning.
Here's a simple code snippet that demonstrates how to output "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Importance
What is C++?
C++ is a powerful general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It builds on the foundation of the C programming language, providing both low-level functionality and high-level abstractions. One of its most notable features is its support for object-oriented programming (OOP), which allows developers to create complex systems in a more manageable way. C++ supports various paradigms, including procedural and functional programming, making it versatile and applicable in a wide range of fields.
Why Learn C++?
Learning C++ is essential for those who aspire to work in fields such as game development, systems programming, and application development. The language is known for its performance and efficiency, as it offers fine-grained control over system resources and memory management. Additionally, many well-known software applications and game engines, such as Unreal Engine, have been written in C++. C++ is also used extensively in competitive programming and algorithm design, providing an excellent platform for sharpening analytical skills.
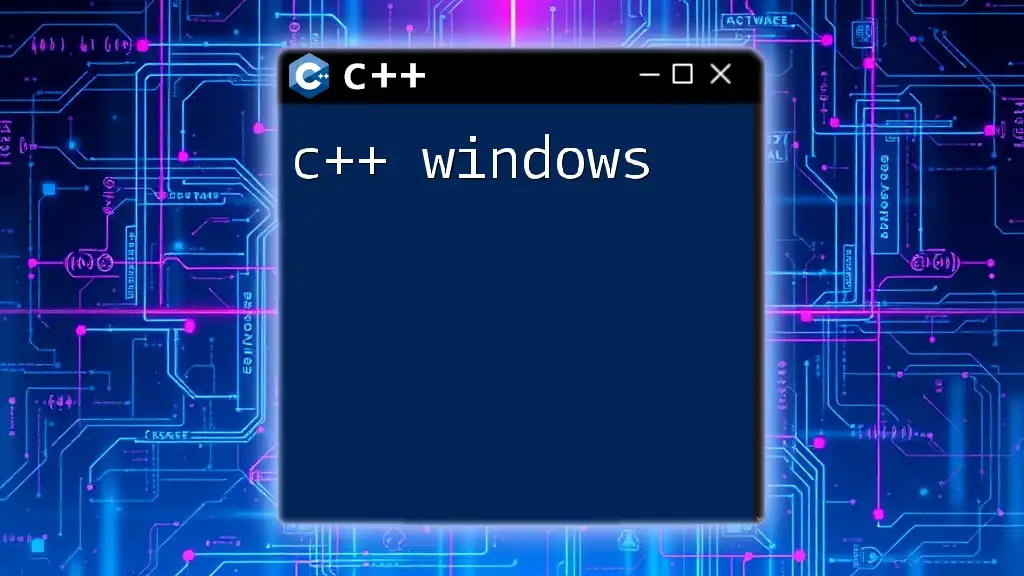
Overview of W3 and Its Role in C++
What is W3?
The World Wide Web Consortium (W3C) is an international community that develops open standards to ensure the long-term growth of the web. Established in 1994, W3C's mission is to make the web accessible to all users, no matter their background or abilities. It plays a critical role in web technologies, setting standards for languages such as HTML, CSS, and JavaScript, which are foundational to web development.
Connection Between C++ and W3
While C++ is primarily known for system-level software, it also plays a role within web development ecosystems. C++ can be used in backend server applications, leveraging its performance benefits. Additionally, some web frameworks support C++, enabling developers to create dynamic web applications. These connections demonstrate that C++ can complement the standards defined by W3, allowing developers to harness the power of C++ while complying with web standards.
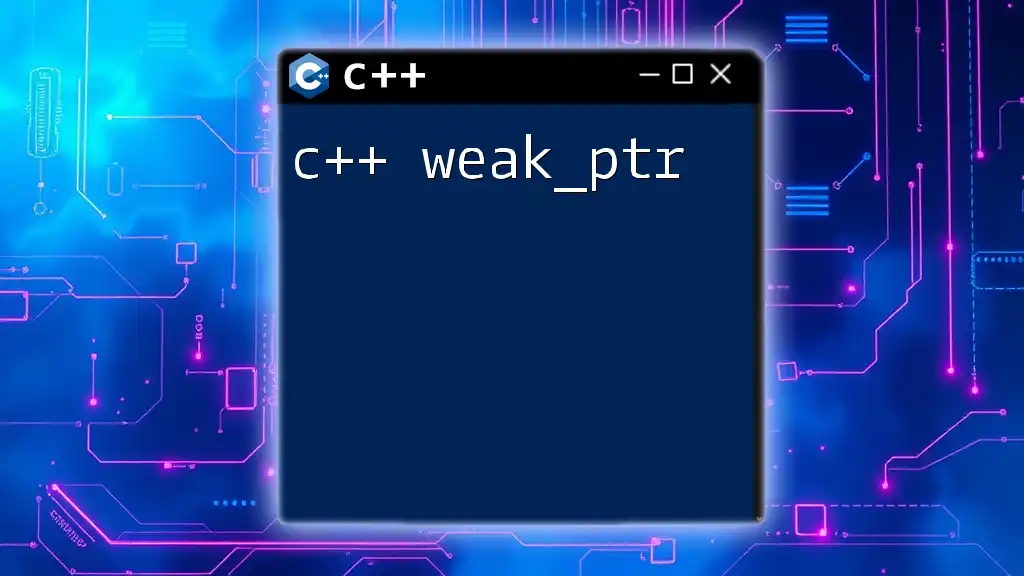
C++ for Web Development: Key Concepts
C++ Environment Setup
Installation Guide To start your journey with C++ and web development, you need to set up your development environment. Recommended IDEs include:
- Visual Studio: A comprehensive IDE ideal for Windows users.
- Code::Blocks: A free, cross-platform IDE suitable for beginners.
- CLion: A paid, yet powerful IDE by JetBrains, particularly useful for professional projects.
You may also need to install specific libraries that facilitate web development, such as POCO and CPPCMS.
Hello World Example Here’s how to write a simple C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
This example showcases the basic structure of a C++ program, including the main function, which serves as the entry point.
Basics of C++ Syntax
Variables and Data Types C++ provides several built-in data types, including:
- int: Represents integer values.
- float: For single-precision floating-point numbers.
- char: For characters.
An example of declaring and using variables is as follows:
int number = 5;
float pi = 3.14;
char letter = 'A';
Control Statements Control statements govern the flow of execution in a program. C++ supports various types, including:
-
If Statements: Allow conditional execution of code blocks.
if (number > 0) { std::cout << "Positive Number"; }
-
Switch Statements: Offer a cleaner way to execute multiple conditions.
switch (number) { case 1: std::cout << "One"; break; // other cases }
-
Loops: Include "for", "while", and "do-while" for repeated execution of code.
for (int i = 0; i < 5; i++) {
std::cout << i;
}
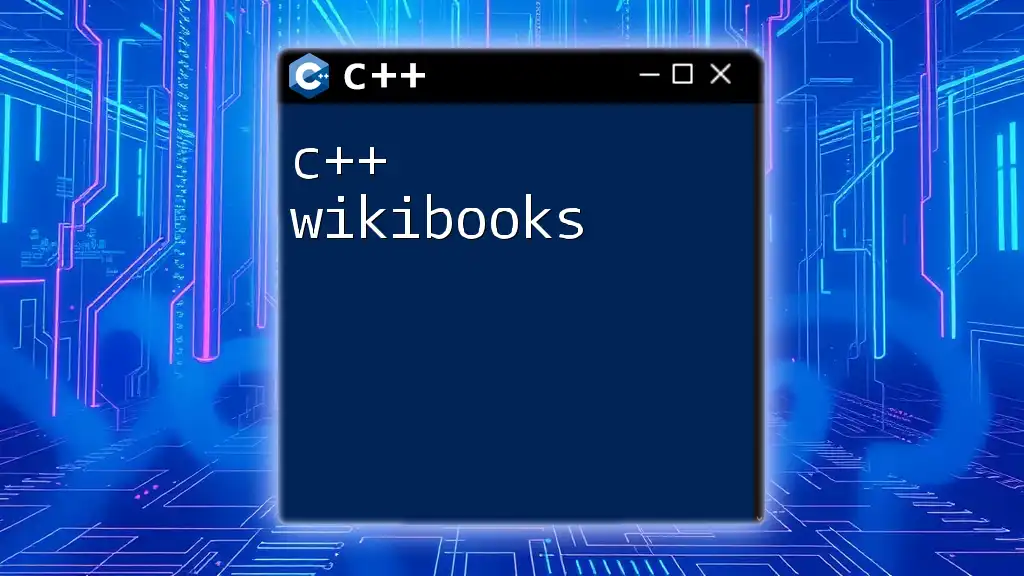
C++ Libraries and Frameworks for Web Development
Introduction to C++ Libraries
Libraries are critical in C++ development, offering pre-written code that saves time and enhances functionality. Some popular C++ libraries relevant to web development include:
- Boost: A widely-used library that adds numerous utility functions and components.
- POCO: Focused on providing platform- and language-independent libraries.
C++ Frameworks for Web Development
Overview of Popular Frameworks Two of the notable C++ frameworks designed for web development are:
-
CPPCMS: A web development framework aimed at high-performance web applications, allowing developers to build scalable systems efficiently.
-
Wt (Witty): A web toolkit for developing interactive web applications, which brings the programming style of desktop applications to the web.
Examples and Use Cases Here's a basic example of creating a web application using CPPCMS:
#include <cppcms/application.h>
#include <cppcms/service.h>
class HelloWorld : public cppcms::application {
public:
HelloWorld(cppcms::service &srv) : cppcms::application(srv) { }
void main(std::string /*url*/) {
response().out() << "Hello World from CPPCMS!";
}
};
This code illustrates how to set up a simple web application that responds with "Hello World" when accessed via a web browser.
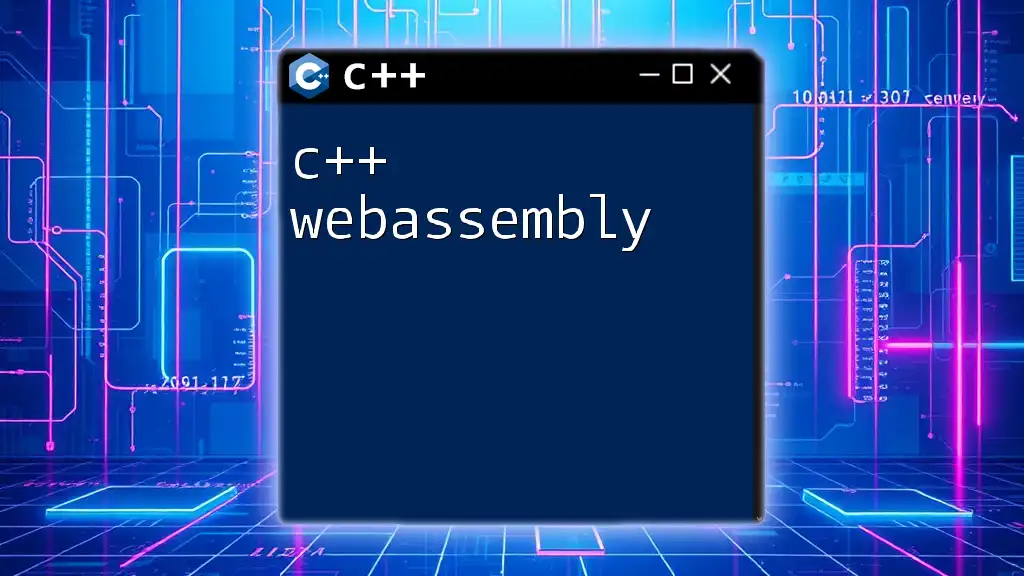
Advanced C++ Concepts Relevant to W3
Object-Oriented Programming in C++
Object-oriented programming (OOP) is a significant feature of C++ that allows developers to create modular, reusable code. Core principles of OOP include:
- Encapsulation: Bundling data alongside the methods that operate on it.
- Inheritance: Creating new classes from existing ones, promoting code reuse.
- Polymorphism: Enabling a single interface to represent different underlying forms (data types).
Real-world examples of OOP in web development could involve designing classes to manage user account information or product inventory in an e-commerce application.
Memory Management
Memory management is crucial in C++ since it allows developers to allocate and deallocate memory manually. Efficient memory management ensures optimal application performance and prevents memory leaks. Techniques include the use of smart pointers, such as:
- std::unique_ptr: Ensures that a single pointer owns a dynamically allocated object.
- std::shared_ptr: Enables shared ownership of an object, useful in many web contexts.
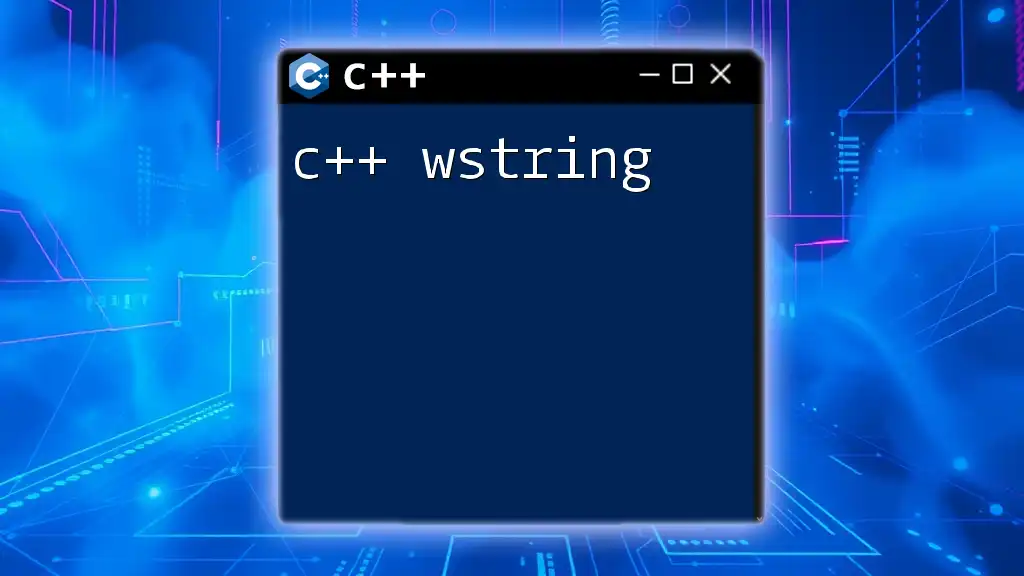
Tools and Resources for Learning C++ for W3
Online Learning Platforms and Courses
Several online platforms offer excellent courses dedicated to C++ programming. Notable mentions are:
- Coursera: Provides courses from accredited universities.
- Udemy: Features a range of beginner to advanced courses at various price points.
- Codecademy: Offers an interactive approach to learning C++ basics.
Communities and Forums
Joining communities and forums can greatly enhance your learning experience. Here are a few valuable resources:
- Stack Overflow: For asking technical questions and getting answers from experts.
- GitHub: A platform for collaborating on projects and exploring open-source code.
- Reddit: Subreddits like r/cpp are great for sharing knowledge and resources.
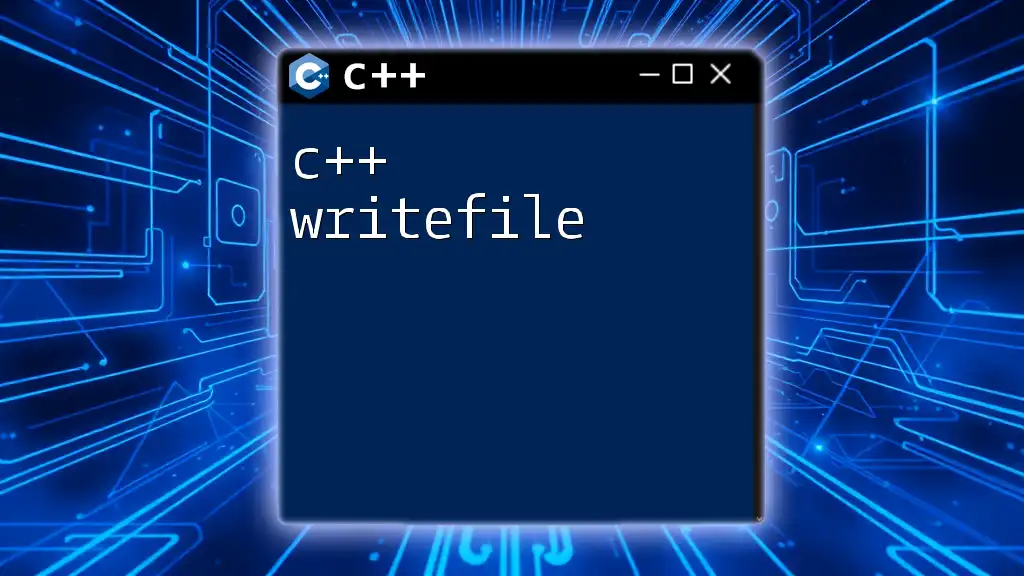
Conclusion
In summary, mastering C++ within the context of W3 opens up numerous opportunities in the web development landscape. C++ brings unique benefits to the table, and its relationship with web standards ensures a robust framework for developing high-performance applications.
If you are eager to explore C++, consider joining our company for structured learning and expert guidance, paving your way towards becoming a proficient C++ developer for web technologies.
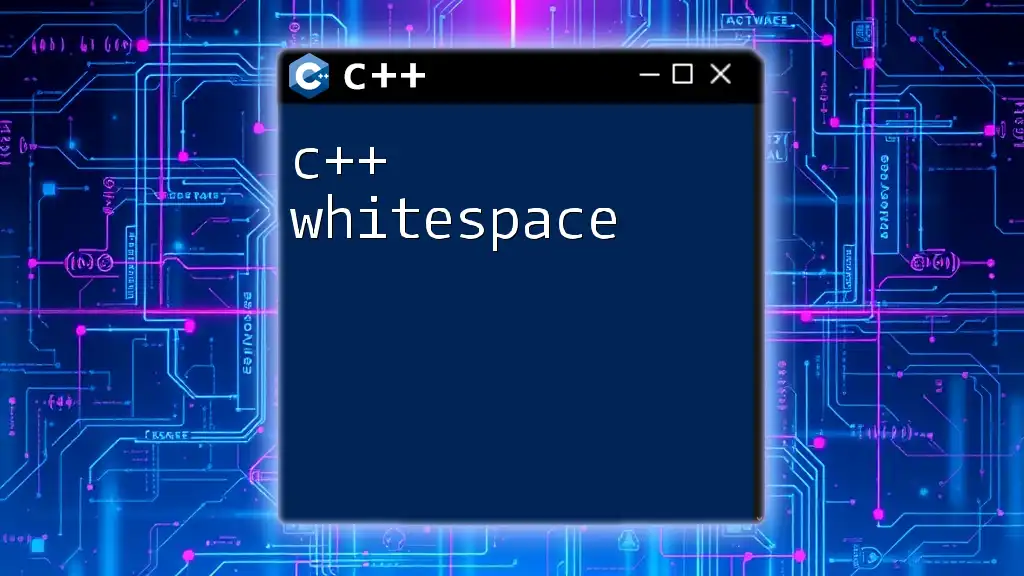
Additional Resources
To further your knowledge, check out:
- Books: "The C++ Programming Language" by Bjarne Stroustrup, "Effective C++" by Scott Meyers.
- Websites: Official C++ documentation, W3C standards.
- Tutorials: Online C++ tutorials on platforms like GeeksforGeeks and CPlusPlus.com.
By leveraging these resources, you can deepen your understanding of C++ and its applications in the ever-evolving web landscape.