The `argv` parameter in C++ is an array of C-style strings (character pointers) that represents the command-line arguments passed to a program, allowing developers to access and utilize user inputs at runtime.
Here's a simple example demonstrating its usage:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
What are `argc` and `argv`?
Definition of `argc`
In C++, `argc` (argument count) represents the number of command-line arguments that the program receives upon execution. It is an integer value that counts the arguments passed into the program, including the program name itself.
For example, when you run a program using the command line like this:
./myProgram arg1 arg2
In this case, `argc` would be 3. Here’s a foundational C++ example to illustrate the usage:
#include <iostream>
int main(int argc, char *argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
return 0;
}
In this snippet, you'll see that `argc` provides an easy and reliable way to access how many arguments the user has input.
Definition of `argv`
`argv` (argument vector) is an array of C-style strings (character pointers) that contains the actual parameters passed to the application. Each element in `argv` corresponds to a command-line argument, and the first element (i.e., `argv[0]`) usually contains the name of the program itself.
To access the arguments, you can reference `argv` with an index. Here's how you can print the first argument:
#include <iostream>
int main(int argc, char *argv[]) {
std::cout << "First argument: " << argv[0] << std::endl; // Program name
return 0;
}
This simple logic allows you to obtain and manipulate the input given by users when they execute your program.
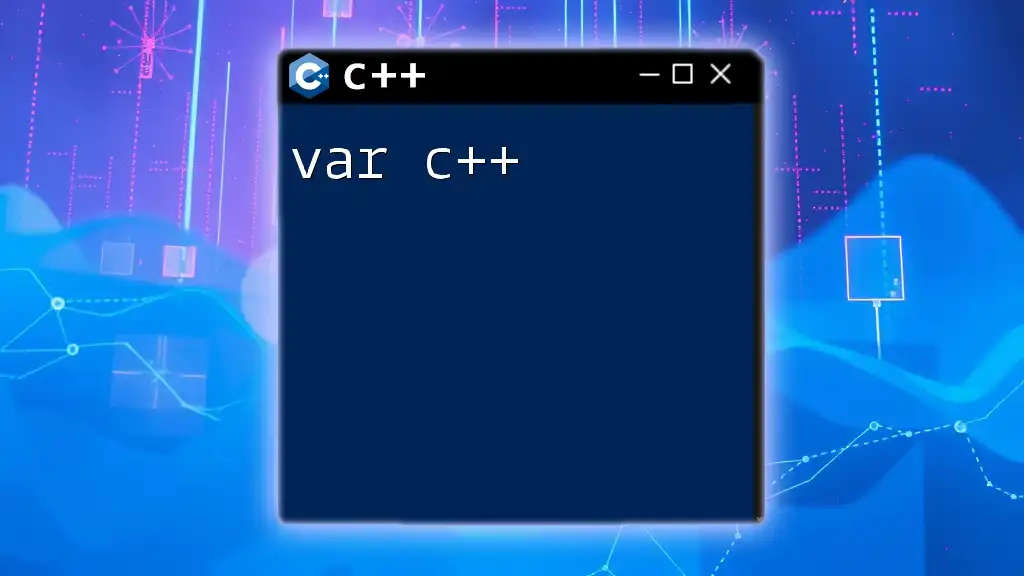
How `argc` and `argv` Work Together
The Role of `argc` in the Command Line
The value of `argc` directly informs you how many arguments are available to work with in your program. Understanding this is crucial for ensuring that your program behaves correctly, especially when expecting specific inputs. For example, a well-structured program will use `argc` to control input flow, preventing crashes from accessing elements outside the `argv` bounds.
Accessing Command-Line Arguments with `argv`
By using a loop to iterate through `argv`, you can access every command-line argument provided. Here's a straightforward example that shows how to do this:
#include <iostream>
int main(int argc, char *argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
This snippet iterates through all the arguments, displaying each one alongside its index. It provides a clear understanding of how `argv` and `argc` function in tandem.
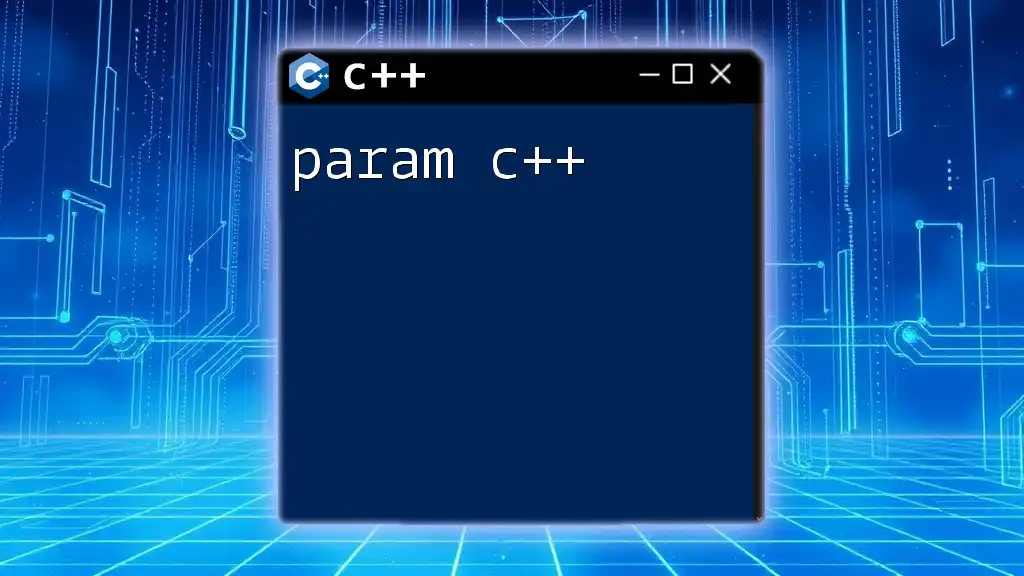
Practical Examples of Using `argc` and `argv` in C++
Setting Up Your C++ Environment
Before diving into examples, ensure that you have a C++ compiler set up. You can compile your C++ files using commands like `g++` on the terminal:
g++ myProgram.cpp -o myProgram
Then, you can run it in the terminal with command-line arguments:
./myProgram arg1 arg2
Example 1: Simple Argument Printing
To demonstrate the basic use of `argc` and `argv`, here's a program that prints each command-line argument:
#include <iostream>
int main(int argc, char *argv[]) {
std::cout << "You entered " << argc << " arguments:" << std::endl;
for (int i = 0; i < argc; i++) {
std::cout << argv[i] << std::endl;
}
return 0;
}
When executed, it provides a straightforward list of the arguments input by the user, reinforcing their collective functionality.
Example 2: Summing Integer Arguments
Next, let’s implement a program to sum integer arguments passed from the command line. This will utilize `argv` to convert string inputs into integers:
#include <iostream>
#include <cstdlib>
int main(int argc, char *argv[]) {
int sum = 0;
for (int i = 1; i < argc; ++i) { // Start from 1 to skip program name
sum += std::atoi(argv[i]);
}
std::cout << "Sum of arguments: " << sum << std::endl;
return 0;
}
In this example, the program ignores the first argument (the program’s name) and calculates the sum of the integers passed as input, showcasing a practical application of `argc` and `argv`.
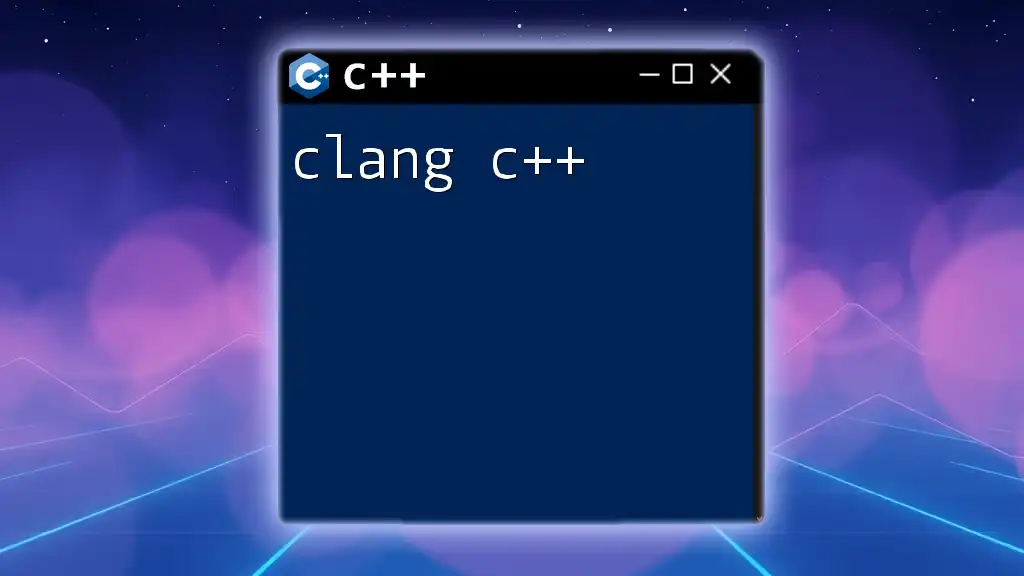
Common Mistakes with `argc` and `argv`
Forgetting to Handle Edge Cases
C++ may not always gracefully handle unexpected command-line inputs. Always check the value of `argc` before accessing elements of `argv`. For instance, if your program expects at least one argument but runs with none, accessing `argv[1]` will lead to undefined behavior.
Misunderstanding Argument Types
A common pitfall is the assumption that all command-line input types are automatically managed. Command-line arguments are inherently strings, so you will frequently need to convert them to the appropriate type using functions like `std::atoi` or `std::stof`.
For example:
int number = std::atoi(argv[1]); // Convert string to integer
Be cautious—failure to validate these inputs can lead to runtime errors.
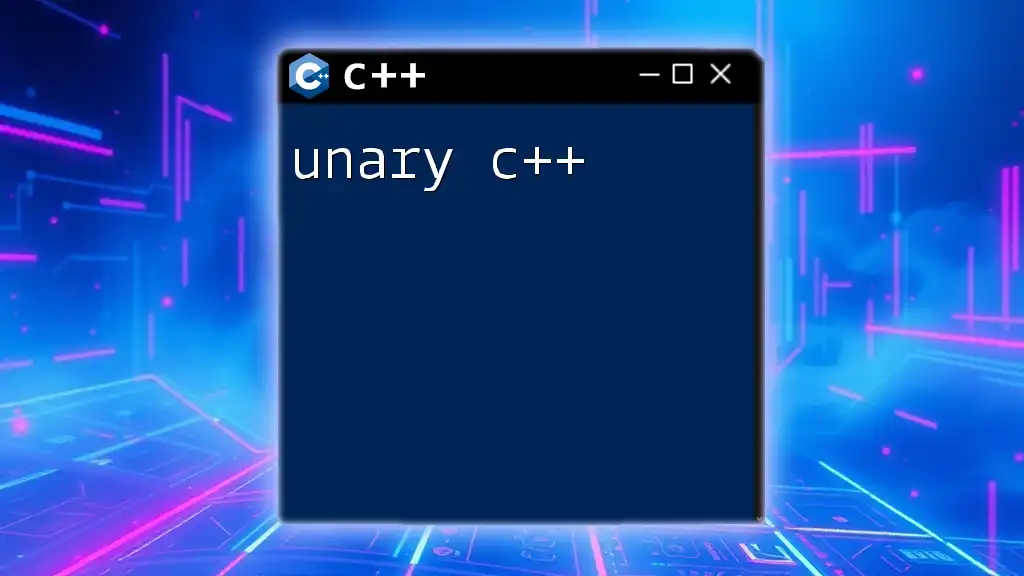
Best Practices for Using `argc` and `argv`
Validating Input Arguments
Implementing robust validation of command-line arguments is essential. Checking that the requisite number of arguments is supplied can avert potential errors.
Here's an example of validating input arguments:
if (argc != 2) {
std::cout << "Usage: " << argv[0] << " <number>" << std::endl;
return 1;
}
This code ensures there's a proper format and that users know how to run your program correctly.
Modularizing Code with Functions
For maintainability, consider separating functionality into functions. It can make your program cleaner and easier to manage. For example, you could create a function that processes the command-line arguments:
void processArguments(int argc, char *argv[]) {
// Logic to process arguments
}
This separation allows you to focus on individual tasks without cluttering the main function.
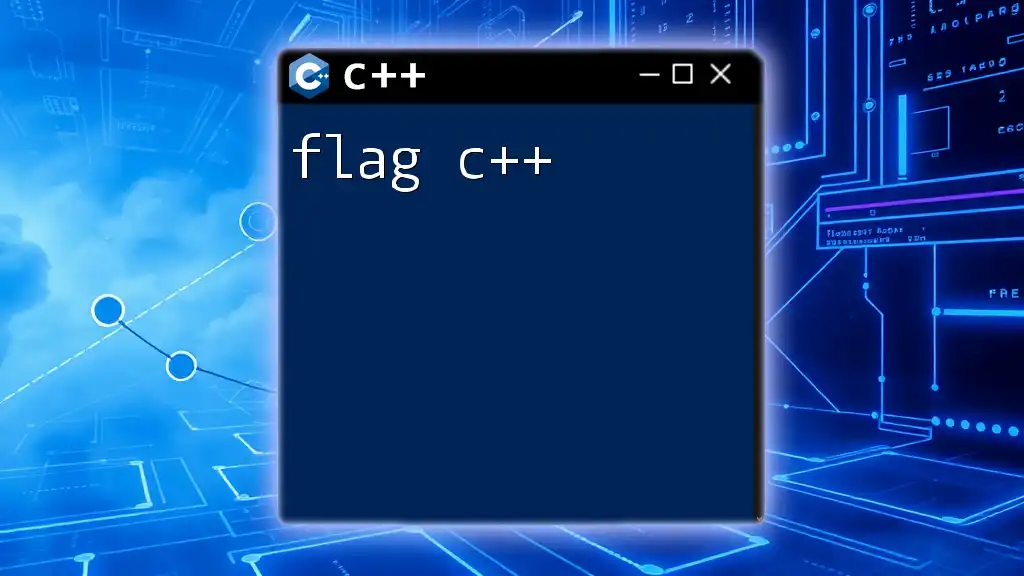
Conclusion
Understanding `argc` and `argv` is fundamental for working with command-line arguments in C++. The examples provided in this guide show how you can leverage these concepts effectively in your programs. By recognizing the importance of validation, modularization, and handling potential pitfalls, you can enhance the robustness and utility of your C++ applications.
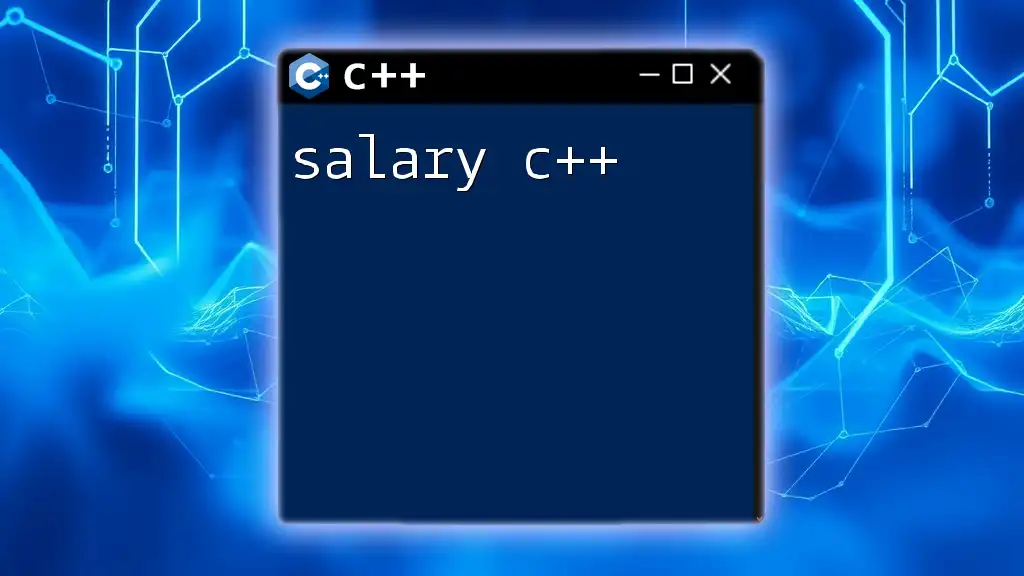
Additional Resources
For those eager to dive deeper, consider checking out:
- C++ reference materials for command-line processing
- Online C++ forums and communities
- Tutorials on data types and input handling
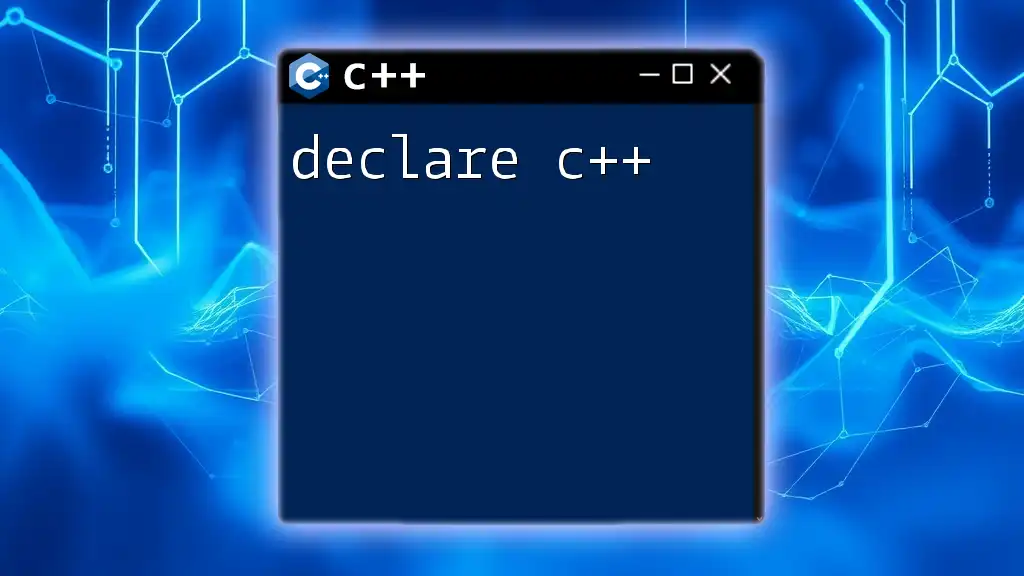
Call to Action
We invite you to share your thoughts or experiences regarding `argc` and `argv` in the comments below! If you found this guide helpful, subscribe or follow for more insightful C++ tips and tricks.