In C++, `int main()` is the standard entry point for execution, but `void main()` is a non-standard usage that is discouraged; here’s how they differ in code:
#include <iostream>
void main() { // Non-standard usage
std::cout << "Hello, World!" << std::endl;
}
int main() { // Standard usage
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the `main` Function
What is the `main` Function?
The `main` function is a crucial component of any C++ program. It serves as the entry point where execution begins. In essence, when you run a C++ program, the execution starts at the `main` function, making it an indispensable part of the program's structure.
Anatomy of the `main` Function
The typical syntax of the `main` function is straightforward, embodying a structured format:
int main() {
// Code goes here
return 0;
}
In this example:
- Return Type: The function returns an `int`, which indicates the exit status of the application.
- Parameters: The `main` function can also take parameters, allowing the program to receive command line arguments, enhancing its flexibility.
Example of a Basic `main` Function
Here’s a simple example showcasing the fundamental structure of the `main` function:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` imports the input-output stream library.
- `std::cout` is used to output text to the console.
- The statement `return 0;` signifies that the program has executed successfully.
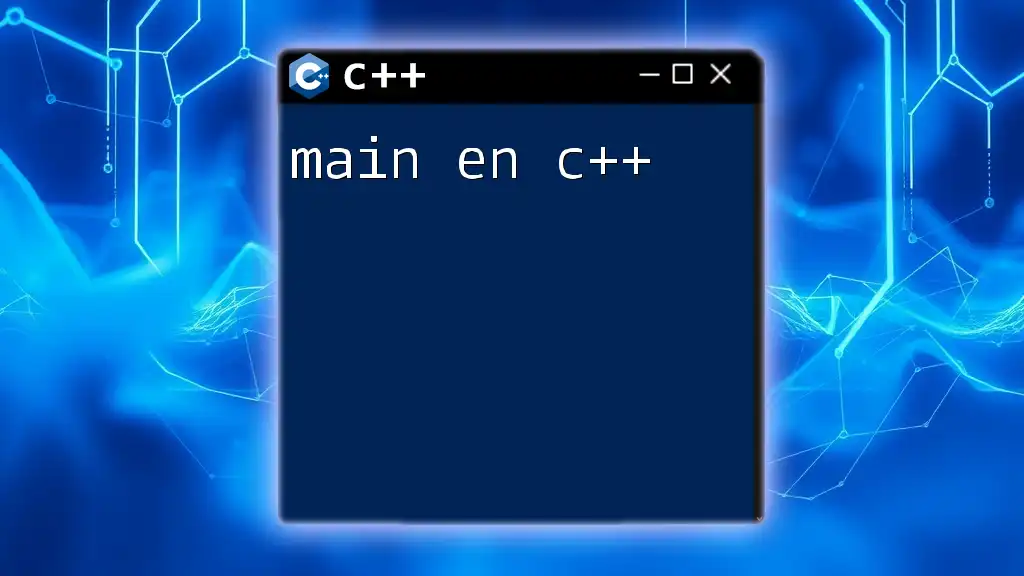
The Return Value of `main`
Why is the Return Value Important?
The return value of the `main` function is significant in C++. It communicates the program's status upon termination. A return value of 0 typically signifies successful execution, whereas a non-zero value indicates an error or abnormal termination. This convention allows other programs or operating systems to determine the result of a program's execution.
Example of Return Values
#include <iostream>
int main() {
// Successful execution
return 0;
}
This example clearly illustrates how a return value is provided. If a program encounters an error during execution, returning a non-zero value can signal this condition, enabling error management by calling processes or user scripts.
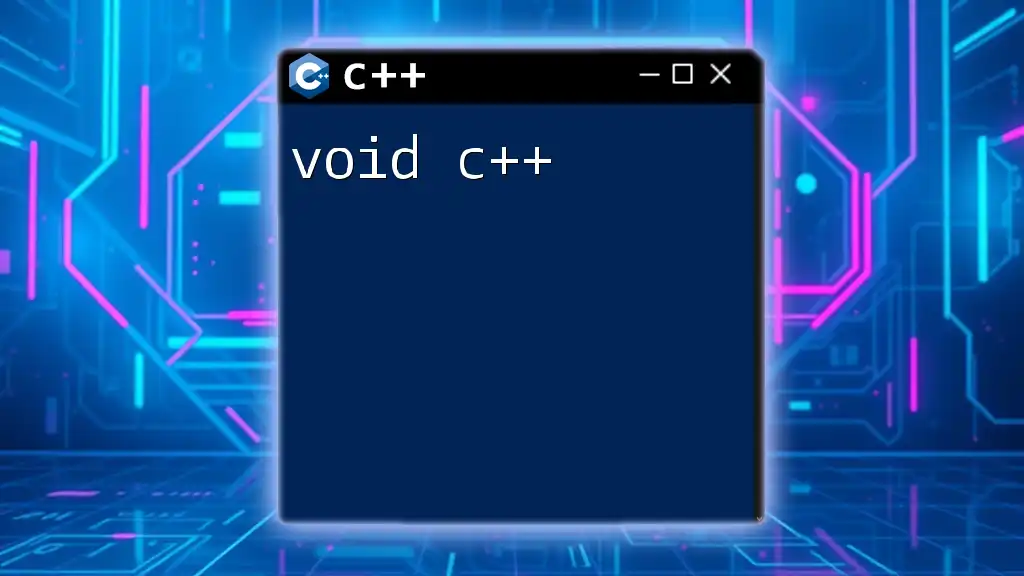
Parameters of the `main` Function
Command Line Arguments
The `main` function can be enhanced to accept command line arguments, which allows the program to take input at runtime. The parameters `argc` and `argv` serve this purpose:
- `argc`: Represents the count of command line arguments.
- `argv`: An array of C-style strings representing the actual arguments passed.
Example of Using Command Line Arguments
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
This function verifies the number of arguments received. The loop iterates through each argument, providing insight into the inputs supplied by the user, demonstrating flexibility and usability in program execution.
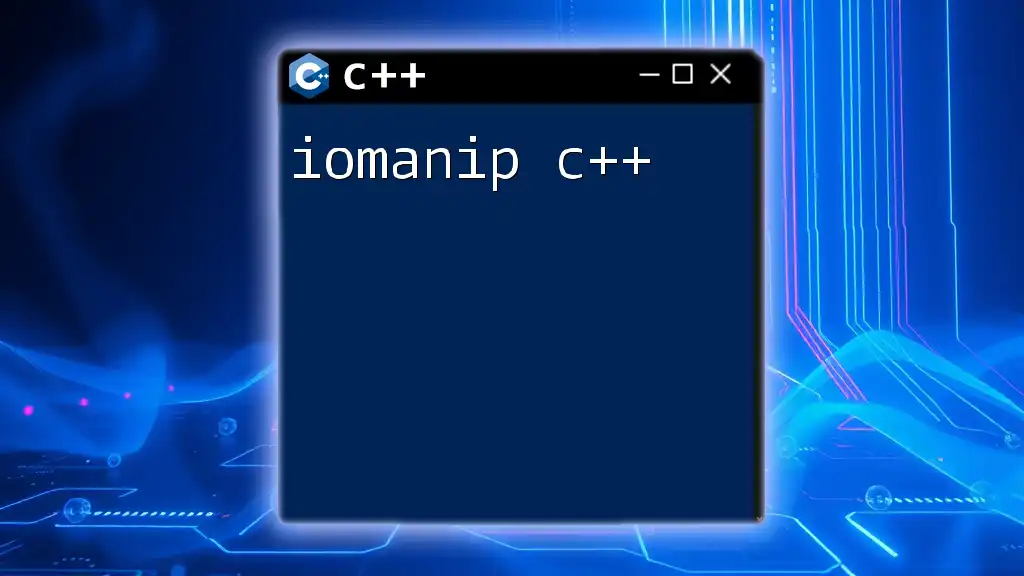
Variants of the `main` Function
Acceptable Variations
While `int main()` and `int main(int argc, char* argv[])` are the most common forms, C++ also allows minor variations:
- Using `const char*` instead of `char*` for `argv` can enhance const-correctness in your program, preventing accidental modification.
Performance and Design Considerations
Choosing between these variations generally depends on the program's requirements. If a program does not require command line input, `int main()` is sufficient and often leads to cleaner code. Conversely, when input from the command line is essential, using parameters becomes necessary.
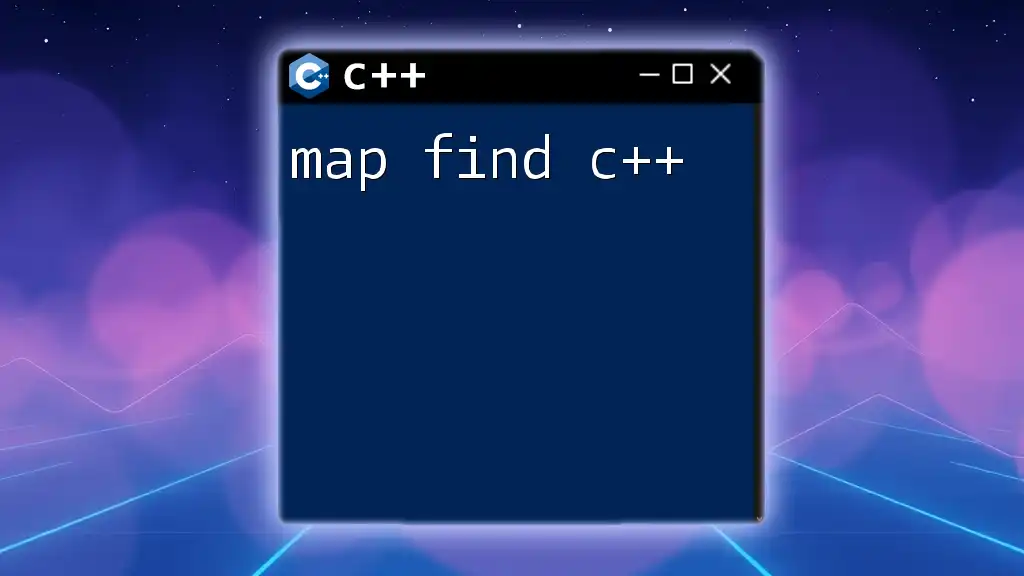
Common Mistakes with the `main` Function
Forgetting the Return Statement
One frequent error is omitting the return statement in the `main` function. Failing to return a value can lead to undefined behavior:
#include <iostream>
int main() {
std::cout << "No return statement." << std::endl;
// Missing return statement
}
In many cases, compilers may not enforce a return statement for `main`, but it is best practice to include one.
Using Incorrect Types
Another common pitfall is mismatching the return types and parameters. For instance, declaring `main` as `void main()` is technically incorrect in standard C++, which requires `int` as the return type. Many compilers will produce warnings or errors.
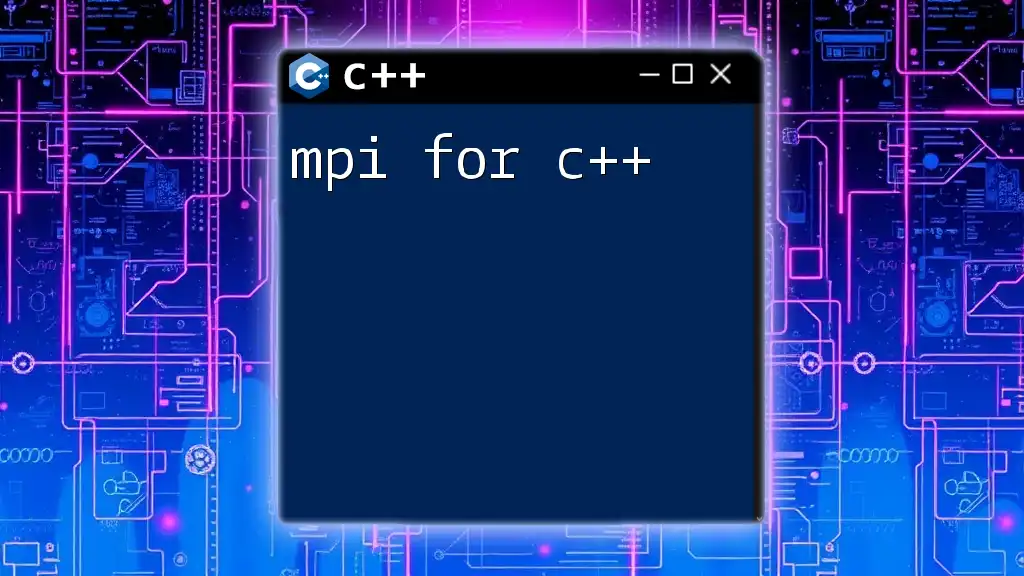
Best Practices for Writing the `main` Function
Code Clarity and Organization
Keep the `main` function succinct, focusing primarily on initializing the program and invoking other functions. This promotes readability and maintainability. The heavier logic should ideally reside in separate functions.
Error Handling
Robust error handling within the `main` function is essential. For instance, validating command line arguments before processing them can prevent runtime errors and improve the user experience.
Example Demonstrating Best Practices
#include <iostream>
#include <stdexcept>
void processArguments(int argc, char* argv[]) {
if (argc < 2) {
throw std::invalid_argument("Not enough arguments.");
}
// Process arguments here
}
int main(int argc, char* argv[]) {
try {
processArguments(argc, argv);
std::cout << "Program executed successfully." << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
In this example, we define a `processArguments` function to handle command line inputs effectively. Additionally, we utilize exception handling within `main`, allowing for clean error reporting. This results in a clear separation of concerns, improving code structure.
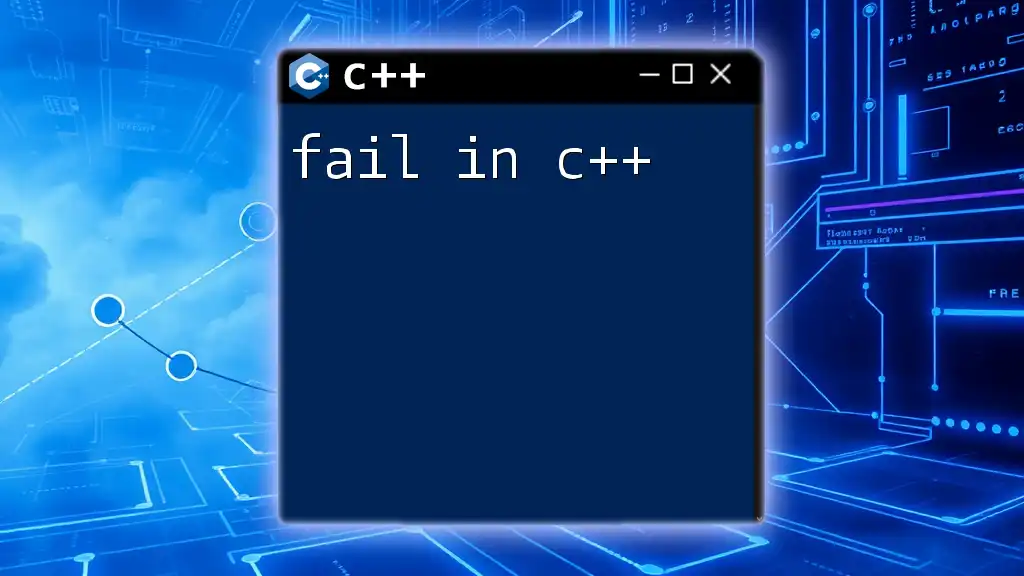
Conclusion
Recap of Key Points
We have covered the primary aspects of the `main` function in C++:
- Its role as the entry point and its significance in program execution.
- The importance of return values that indicate success or failure.
- Acceptable parameter variations and how they enhance the functionality of the program.
- Common pitfalls to avoid, ensuring robust and maintainable code.
Encouragement to Explore Further
Understanding the `main` function is merely the starting point of mastering C++. Take the initiative to explore more complex programming concepts, data structures, and C++ Standard Library features. The journey of becoming proficient in C++ is both challenging and rewarding, and every line of code contributes to your growing skill set.
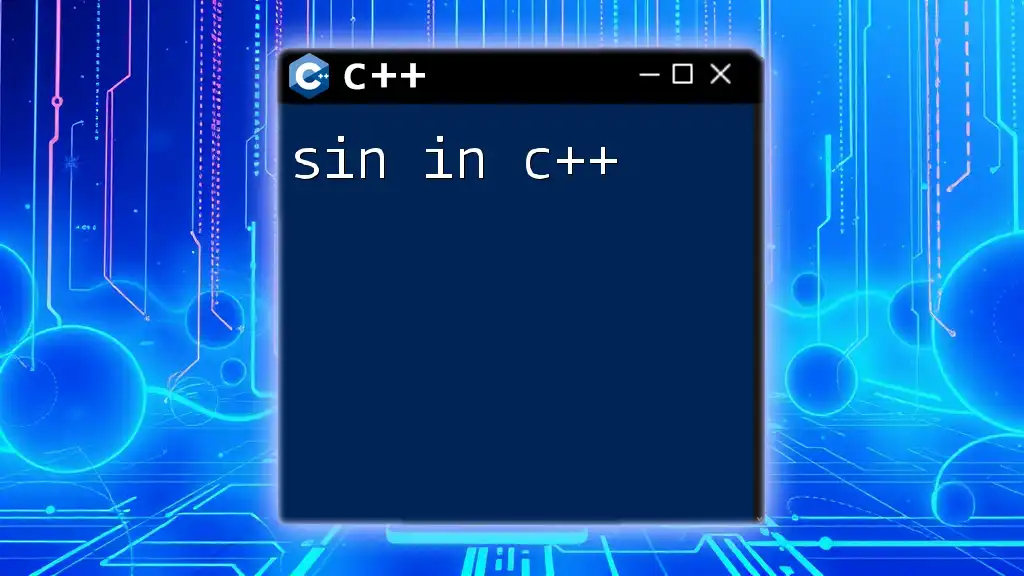
Additional Resources
For further exploration, consider checking the official C++ documentation, practical textbooks, and online courses that provide in-depth knowledge about both the `main` function and the broader C++ language. Engaging with community forums and discussions can also bolster your understanding and inspire new projects.