In C++, the "not in" operation can be simulated using the "std::find" algorithm to check if an element is absent from a container, as shown in the following code snippet:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int value = 6;
if (std::find(numbers.begin(), numbers.end(), value) == numbers.end()) {
std::cout << value << " is not in the vector." << std::endl;
} else {
std::cout << value << " is in the vector." << std::endl;
}
return 0;
}
Understanding the Basics
Definition of "not in"
The term "not in" refers to a logical condition that checks if a particular element does not exist within a collection, such as an array, vector, or set. In C++, the most common way to implement "not in" logic is through the use of the not operator `!` combined with other comparison techniques. This operator negates a boolean value, effectively checking if a condition is false.
Common Use Cases
The "not in" functionality is crucial in various scenarios, including:
- Validation Checks: Ensuring user input does not match certain criteria.
- Search Operations: Verifying if an item is absent from a list before performing actions.
- Conditional Logic: Implementing business rules where certain conditions must not be met.
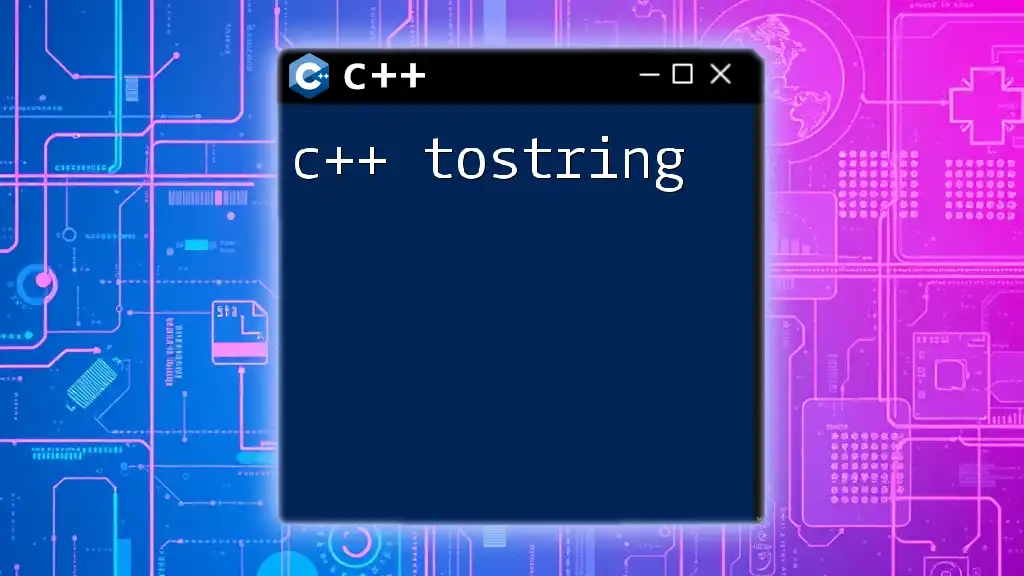
Implementing "not in" in C++
Using the Not Operator (!)
The most straightforward way to check if an element is not present is to use the not operator `!`. This operator can be applied to conditions, effectively flipping their truth values.
Code Snippet: Basic Example
#include <iostream>
int main() {
bool condition = false;
if (!condition) {
std::cout << "Condition is NOT true." << std::endl;
}
return 0;
}
In this simple example, the `condition` is set to false. When checked within the `if` statement, `!condition` evaluates to true, resulting in the output "Condition is NOT true." This demonstrates the core working of the not operator.
Combining "not in" with Conditionals
You can use "not in" logic effectively within conditional statements, allowing for more sophisticated checks.
Code Snippet: if-else Example
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int test_number = 6;
if (std::find(numbers.begin(), numbers.end(), test_number) == numbers.end()) {
std::cout << test_number << " is NOT in the vector." << std::endl;
} else {
std::cout << test_number << " is in the vector." << std::endl;
}
return 0;
}
In this code, we utilize `std::find`, a STL function that returns an iterator to the element if found, or `end()` if not. By checking if the result of `std::find` equals `numbers.end()`, we determine that `test_number` (6) is indeed not in the vector, leading to the output "6 is NOT in the vector."
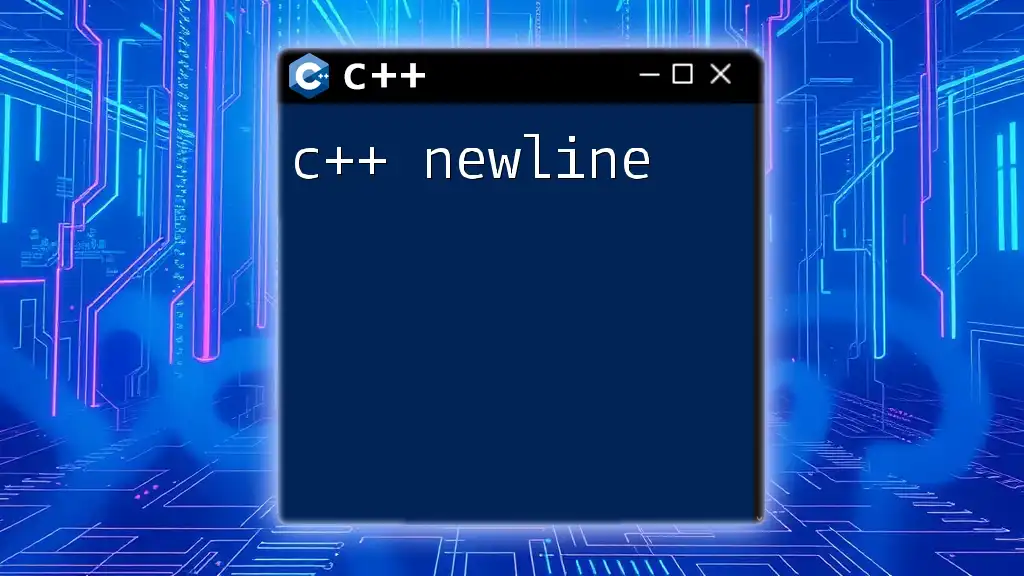
Advanced Techniques
Using STL Functions for "not in" Logic
The Standard Template Library (STL) provides a wealth of functions that simplify checking for absence, enhancing code efficiency and readability.
Code Snippet: Using std::find
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<char> charList = {'a', 'b', 'c', 'd'};
char ch = 'e';
if (std::find(charList.begin(), charList.end(), ch) == charList.end()) {
std::cout << ch << " is NOT in the list." << std::endl;
}
return 0;
}
This example validates whether the character `ch` is absent from `charList`. Using `std::find` streamlines the process, showcasing the utility of STL in managing collections effectively.
Lambda Functions with "not in"
Lambda expressions in C++ introduce a powerful method for concise operations. They enable us to create anonymous functions that can encapsulate custom logic for checking absence.
Code Snippet: Lambda Example
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> values = {10, 20, 30, 40};
int check_value = 25;
auto not_in = [&](int value) {
return std::find(values.begin(), values.end(), value) == values.end();
};
if (not_in(check_value)) {
std::cout << check_value << " is NOT in the values." << std::endl;
}
return 0;
}
In this scenario, the `not_in` lambda function encapsulates the logic for check absence in the `values` vector. It improves code readability and allows for flexible checks without rewriting the logic multiple times.
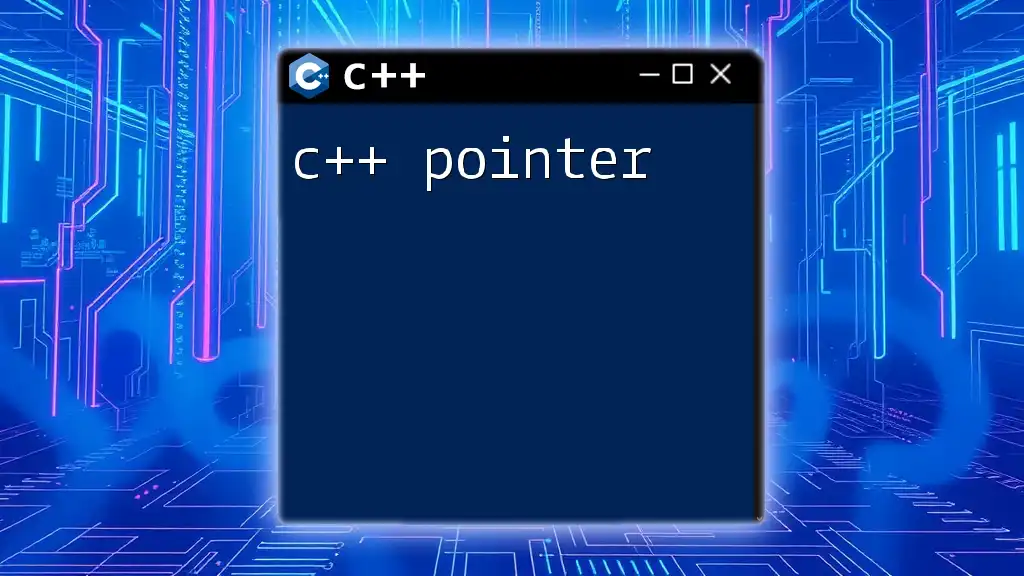
Common Mistakes to Avoid
Logical Errors with "not in"
A frequent mistake programmers make is misinterpreting the logical condition. Ensure that the `not in` check accurately reflects your intent, especially with composite conditions or nested checks. Always validate your logic through debugging.
Performance Considerations
When operating on large data sets, using STL functions such as `std::find` repeatedly can lead to performance bottlenecks, as its time complexity is linear (O(n)). In performance-critical applications, consider using alternative data structures, like sets or maps, which can offer average-case constant time complexity for existence checks.
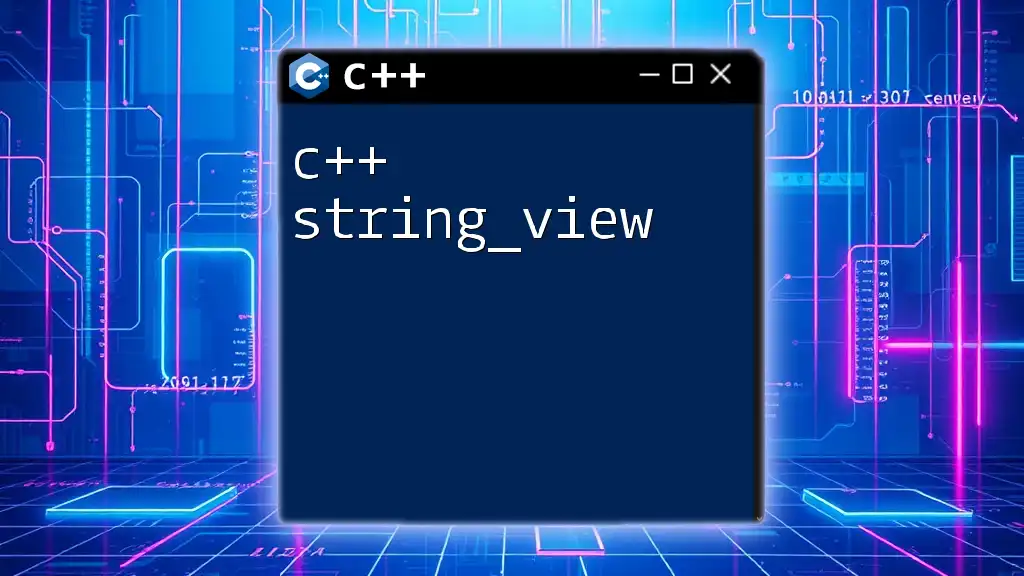
Conclusion
Mastering the c++ not in concept is fundamental for efficient programming, enabling you to write cleaner, more efficient code. By leveraging the not operator, STL functions, and modern C++ features like lambda expressions, you can effectively implement absence checks in various applications. Practice these techniques through coding exercises to solidify your understanding and enhance your C++ skill set.
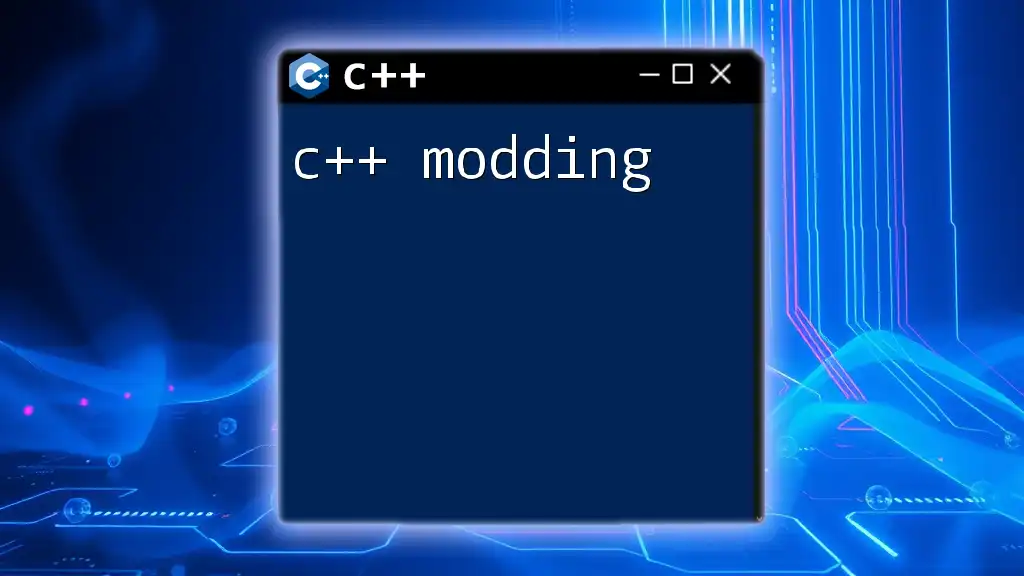
Further Learning Resources
For those looking to expand their knowledge further, consider exploring books on advanced C++, engaging in online courses focused on the Standard Template Library, or participating in community forums and coding challenges that emphasize efficient coding practices.
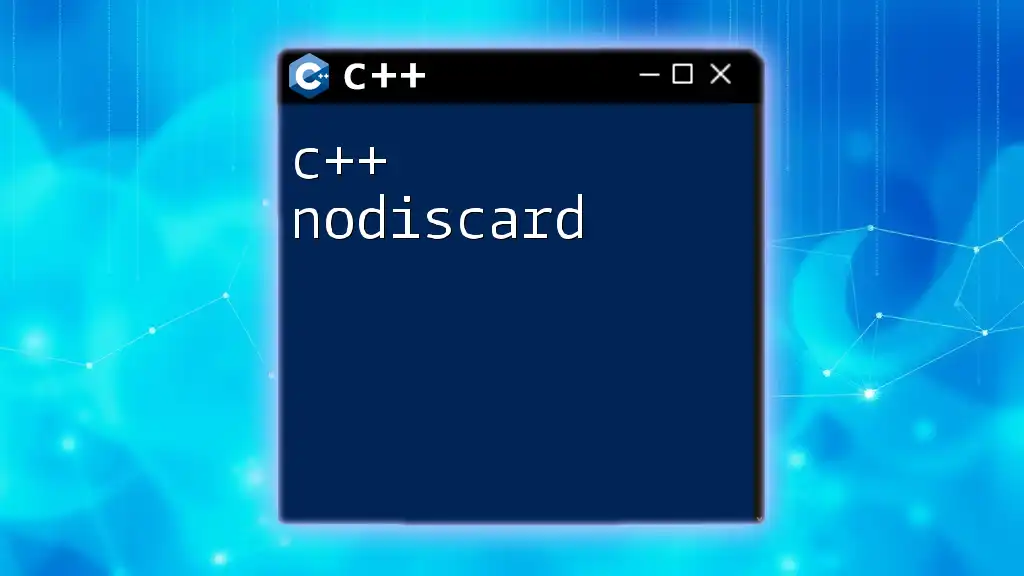
Call to Action
Have you encountered challenges with "not in" logic in C++? Share your experiences with us! Join more conversations about mastering C++ programming by exploring our range of offered resources and guides tailored for aspiring developers.