The C++ `atof` function converts a string representing a floating-point number into its double representation, making it useful for parsing input data.
#include <iostream>
#include <cstdlib> // for atof
int main() {
const char* str = "3.14";
double value = atof(str);
std::cout << "The converted value is: " << value << std::endl;
return 0;
}
What is atof?
`atof`, which stands for "ASCII to Floating Point," is a function in C++ that is used to convert a C-style string (character array) into a double-precision floating-point number. This makes it especially useful when you need to work with numerical data that is initially represented in a string format, such as user input or data read from a file.
When to Use atof?
You would typically use `atof` in scenarios where you have string representations of floating-point numbers. For example, when programming a calculator or processing user input from a console or file, you might encounter numerical values in string form. While `atoi` is used for converting strings to integers and `strtod` allows for error handling, `atof` provides a straightforward means to obtain a floating-point representation.
Understanding the Syntax of atof
Function Declaration
The function prototype for `atof` is quite simple. It fits seamlessly into the C++ standard library:
double atof(const char* str);
Here, the function takes a single parameter, `str`, which is a pointer to a null-terminated character string. It returns a `double` value representing the converted floating-point number.
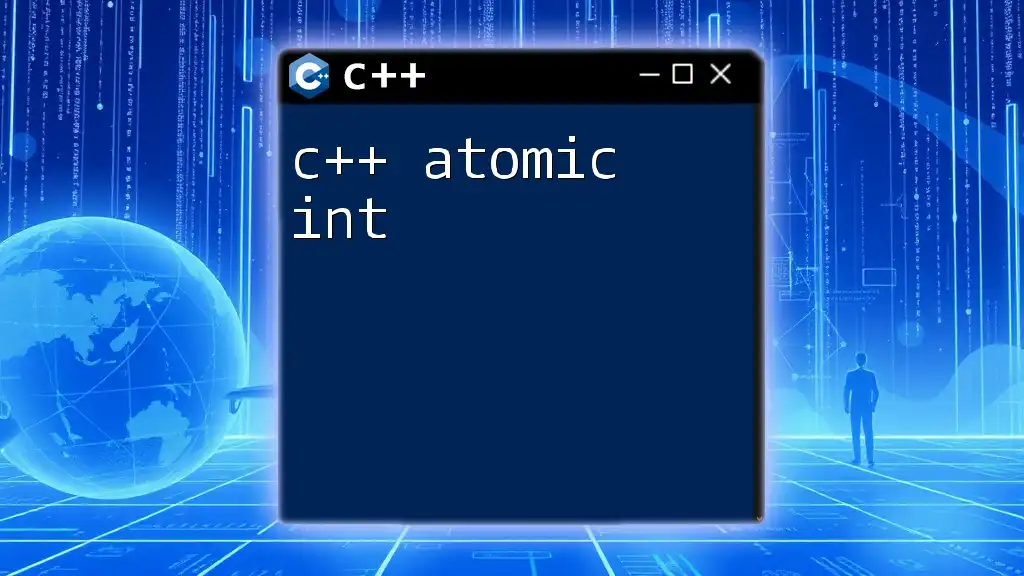
How to Use atof in C++
Basic Example
To understand how to utilize `atof`, here is a straightforward code example:
#include <iostream>
#include <cstdlib> // for atof
int main() {
const char *str = "3.14";
double value = atof(str);
std::cout << value << std::endl; // Output: 3.14
return 0;
}
In this example, the string `"3.14"` is passed to `atof`, which converts it to the floating-point number `3.14`, and outputs it.
Handling Invalid Input
One of the characteristics of `atof` is its behavior when dealing with invalid input. If the input string does not represent a valid number, `atof` will return `0.0`. Here is a code snippet to illustrate this:
#include <iostream>
#include <cstdlib>
int main() {
const char *invalidStr = "abc";
double value = atof(invalidStr);
std::cout << value << std::endl; // Output: 0
return 0;
}
In this instance, since `"abc"` is not a valid number, `atof` returns `0`, which can sometimes lead to misinterpretation of the value. This highlights a limitation of using `atof`, as it does not indicate if an error occurred.
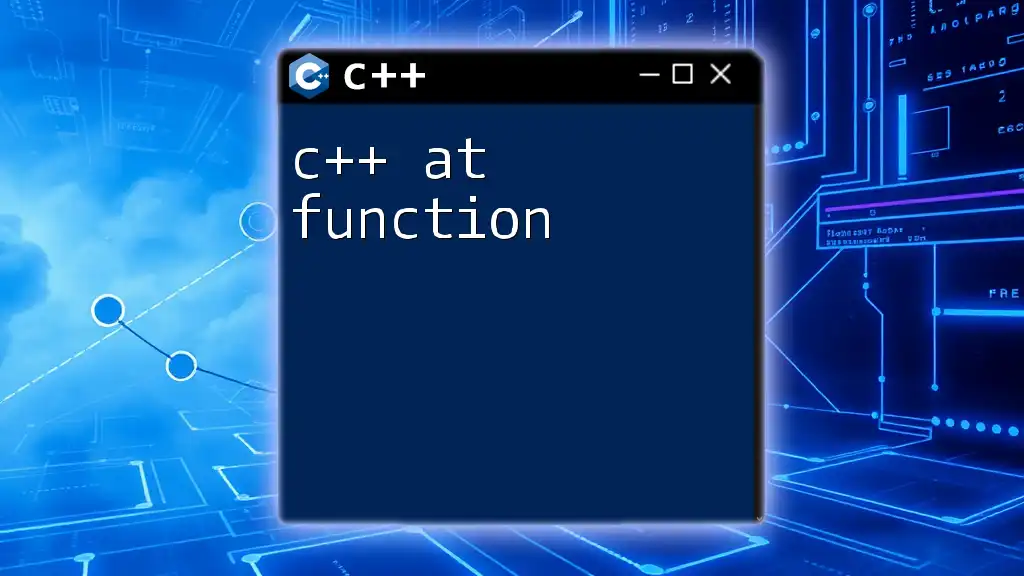
Key Features of atof
Automatic Handling of Whitespaces
One significant advantage of `atof` is that it automatically handles leading whitespace in the input string. This means that you can safely pass strings with spaces without worrying about errors. Here's an example:
#include <iostream>
#include <cstdlib>
int main() {
const char *str = " 2.7";
double value = atof(str);
std::cout << value << std::endl; // Output: 2.7
return 0;
}
In this code, `atof` correctly processes the string, ignoring the spaces, and outputs `2.7`.
Support for Scientific Notation
`atof` also supports strings in scientific notation, allowing you to convert values like `1.23e4` directly into floating-point numbers. Below is an example demonstrating this feature:
#include <iostream>
#include <cstdlib>
int main() {
const char *scientificStr = "1.23e4";
double value = atof(scientificStr);
std::cout << value << std::endl; // Output: 12300
return 0;
}
Here, the string `"1.23e4"` is converted to `12300`, showcasing the ability of `atof` to interpret exponential notation.
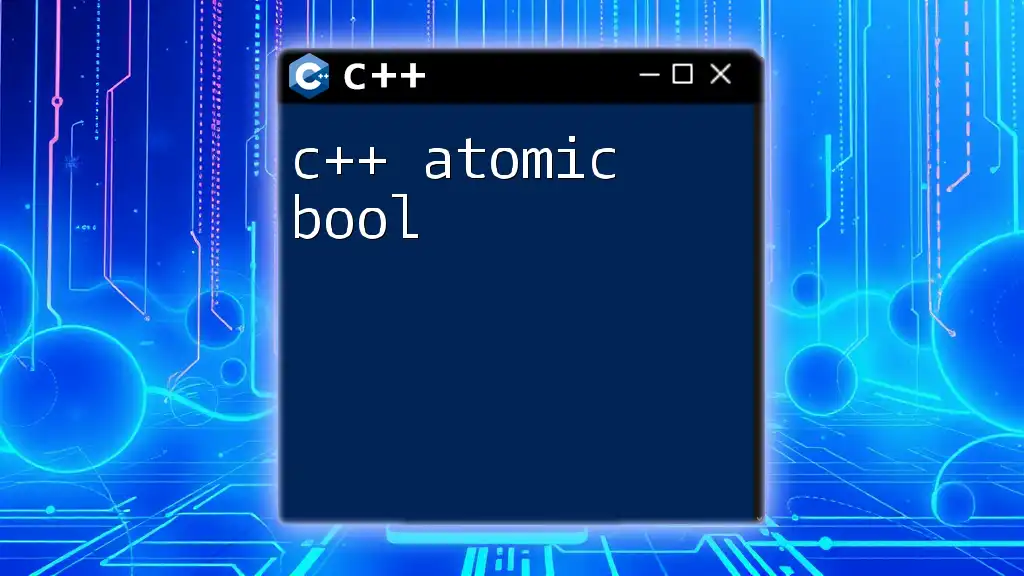
Common Pitfalls When Using atof
No Error Handling
One of the main drawbacks of `atof` is its lack of error handling. Unlike `strtod`, `atof` cannot indicate whether the conversion was successful or if an error occurred (e.g., if the string input was invalid). This could lead to confusion for developers, especially in critical applications where valid numerical input is required.
Incorrect Type of Input
When user inputs may lead to unexpected results, programmers should be cautious. If a user tries to input non-numeric characters, `atof` will simply return `0.0`, which can be misleading. Here’s an example to illustrate this concern:
#include <iostream>
#include <cstdlib>
int main() {
const char *userInput = "5.76xyz"; // invalid input
double value = atof(userInput); // will return 5.76
std::cout << value << std::endl; // Output: 5.76
return 0;
}
In the example above, even though the string is invalid after the number, `atof` will stop reading at the first invalid character and return a partially converted value instead of indicating an error.
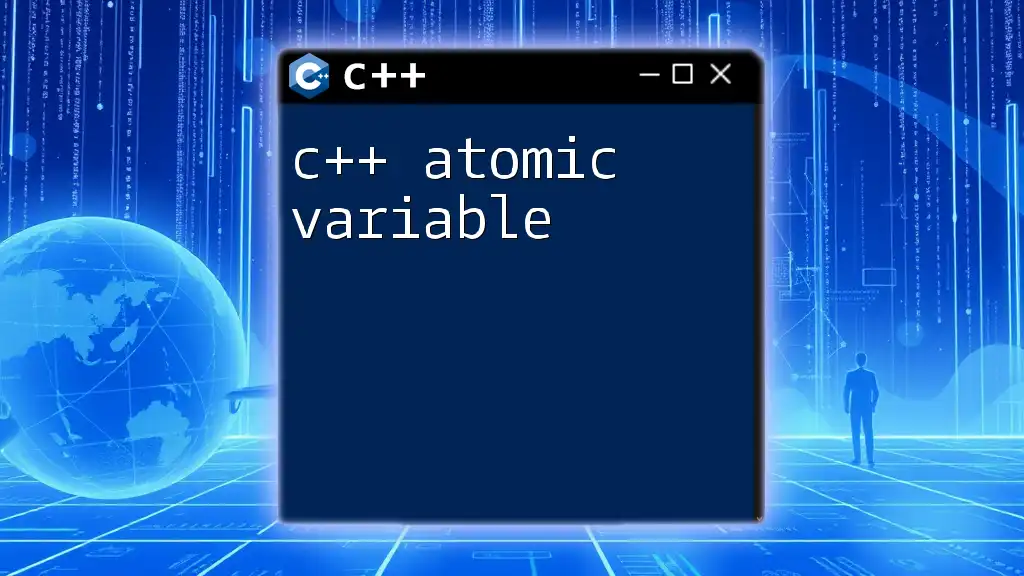
Best Practices for Using atof
When to Prefer atof
`atof` should be used in situations where you are confident that the string inputs are valid or controlled. For example, when reading static data files with known good formatting, `atof` is a quick and efficient option. However, if your application is prone to receiving unpredictable user input, consider alternatives for better safety.
Tips for Improved Code Quality
To maintain code quality while using `atof`, ensure that you validate all strings before conversion. Using direct assertions about the input format can help prevent runtime errors. Also, it’s wise to implement checks to ensure that the returned values are within the expected range.
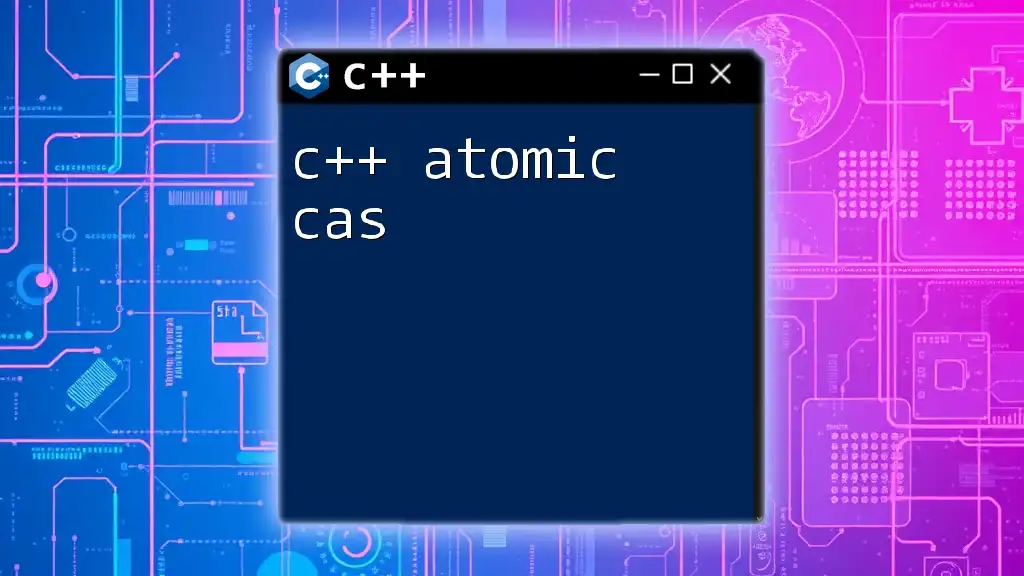
Alternative Functions to atof
Overview of strtod
`strtod` is a more flexible alternative to `atof`. It allows for error checking by providing a pointer to the character that stops the conversion. This can be beneficial for identifying reasons why a string might not convert properly.
Here’s a brief example of using `strtod`:
#include <iostream>
#include <cstdlib>
int main() {
const char *str = "3.14abc";
char *end;
double value = strtod(str, &end);
if (end == str) { // No conversion was performed
std::cout << "Conversion error!" << std::endl;
} else {
std::cout << value << std::endl; // Output: 3.14
}
return 0;
}
This code shows that `strtod` not only converts the valid part but also allows you to detect issues with the input string.
Comparison with Other Conversion Functions
When choosing between `atoi`, `atof`, and `strtod`, it's crucial to consider factors such as input validation and the expected range of data. `atoi` and `atof` are straightforward but lack error handling, while `strtod` offers more robustness at the cost of added complexity.
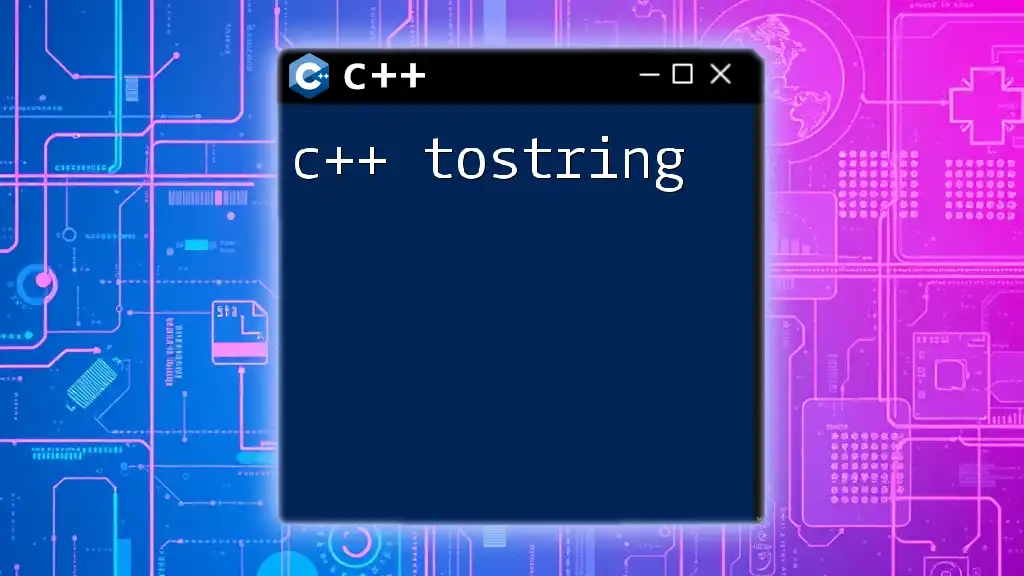
Conclusion
Summary of Key Takeaways
In summary, `atof` is a convenient function for converting strings to double-precision floating-point numbers, particularly in controlled environments. However, due to its limitations in error handling, it is best used in contexts where input validity is guaranteed. When dealing with user input or uncertain formatting, consider alternatives like `strtod` for more robust error checking.
Call to Action
If you found this guide on C++ atof helpful, consider exploring more concise C++ tutorials to enhance your understanding and proficiency with this essential programming language. Experiment with the examples provided, and don't hesitate to ask questions or seek further resources to deepen your knowledge.
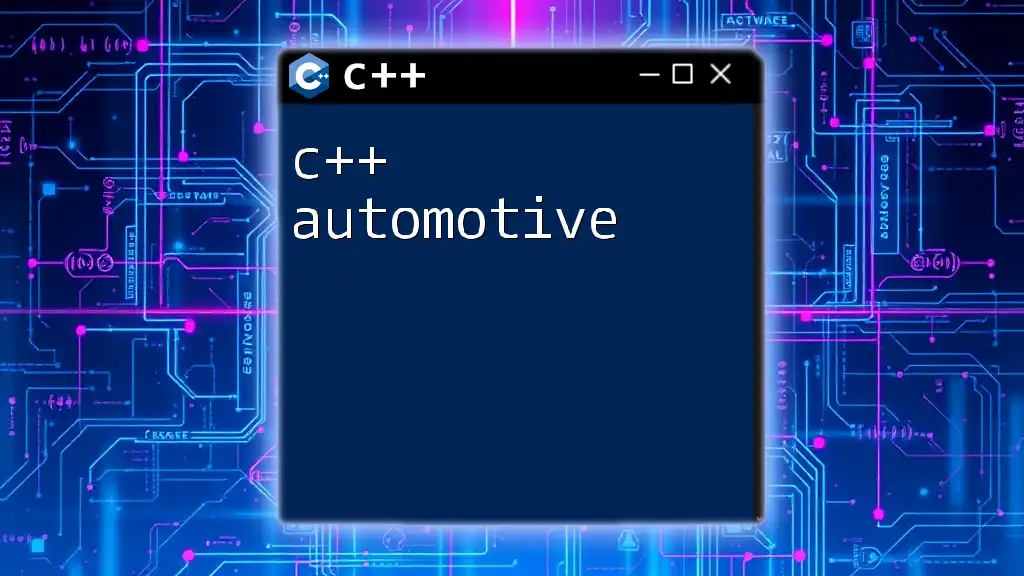
Additional Resources
Links to Official Documentation
To further your insights, refer to the official C++ standard library documentation and explore additional reading materials on string conversion functions. Explore tutorials focused on input validation and error handling strategies in C++.