The C++ `acos` function computes the arc cosine of a given value, returning the angle in radians whose cosine is that value.
#include <iostream>
#include <cmath>
int main() {
double value = 0.5;
double result = acos(value);
std::cout << "The arc cosine of " << value << " is: " << result << " radians." << std::endl;
return 0;
}
Understanding the Mathematical Background
What is the Arccosine?
The arccosine (or inverse cosine) function, denoted as arccos(x), is the inverse operation to the cosine function. It returns the angle whose cosine is the specified number, which can range from -1 to 1. For example, if cos(θ) = x, then arccos(x) will yield θ, yielding results in radians.
The graphical representation of the arccosine function illustrates how it operates, where the output is derived from the x-axis values ranging from -1 to 1. This function is critical in trigonometry, calculus, and in various fields that require angular computations.
Range and Domain of acos
The domain of the acos function is restricted to the interval \([-1, 1]\), meaning that it only accepts inputs within this range. Any value outside this boundary will result in an error.
The range of the result provided by acos is \([0, \pi]\) radians, which corresponds to the angles 0 to 180 degrees. This range ensures that the function produces a unique output, which is essential in mathematical applications.
Applications of acos in C++ Programming
C++ developers often utilize the acos function in various practical applications, such as:
- Engineering Calculations: When computing angles in mechanical systems or structural analyses.
- Graphics Programming: For rendering 2D and 3D transformations, where angles must be calculated between objects.
- Data Analysis: In machine learning and statistical methods, especially when working with vectors and angles in multi-dimensional spaces.
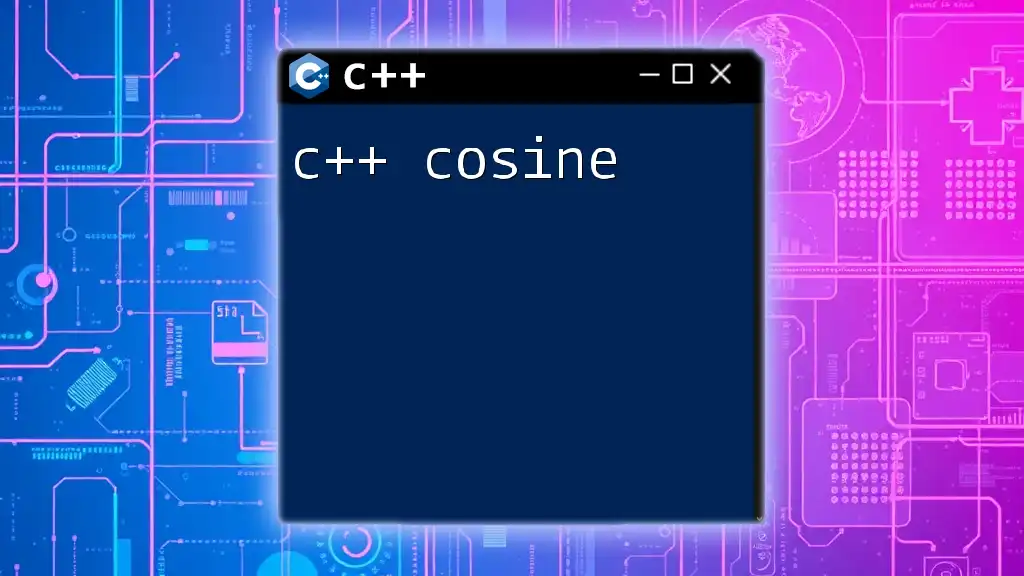
Getting Started with C++ acos
To use the acos function in your C++ program, you need to include the required header file:
#include <cmath>
Including this library is essential, as it provides access to a variety of mathematical functions, including trigonometric ones like acos.
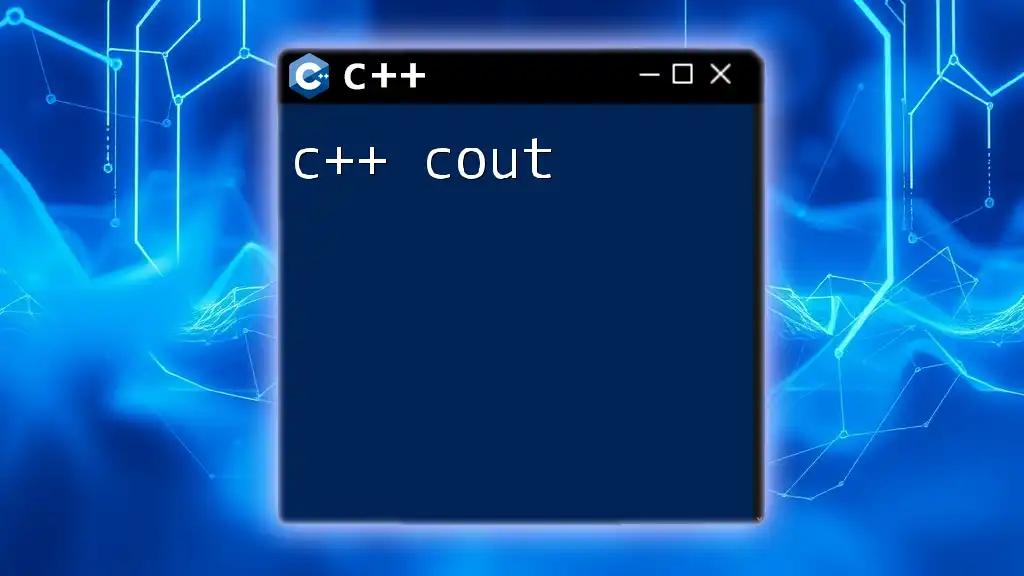
Syntax of acos Function in C++
The basic syntax of the acos function is as follows:
double acos(double x);
Parameters and Return Value
The function takes a single parameter, `x`, which is a double precision floating-point number. The function returns the arccosine of `x` in radians. If `x` is not within the valid range of \([-1, 1]\), the function may produce a domain error.
Example: Basic Usage of acos
Here’s a simple example demonstrating how to use the acos function:
#include <iostream>
#include <cmath>
int main() {
double value = 0.5;
double result = acos(value);
std::cout << "The arccosine of " << value << " is: " << result << " radians." << std::endl;
return 0;
}
In this code snippet, we calculate the arccosine of 0.5. The expected output will be:
The arccosine of 0.5 is: 1.0472 radians.
This translates to approximately 60 degrees, highlighting the relationship between radians and degrees.
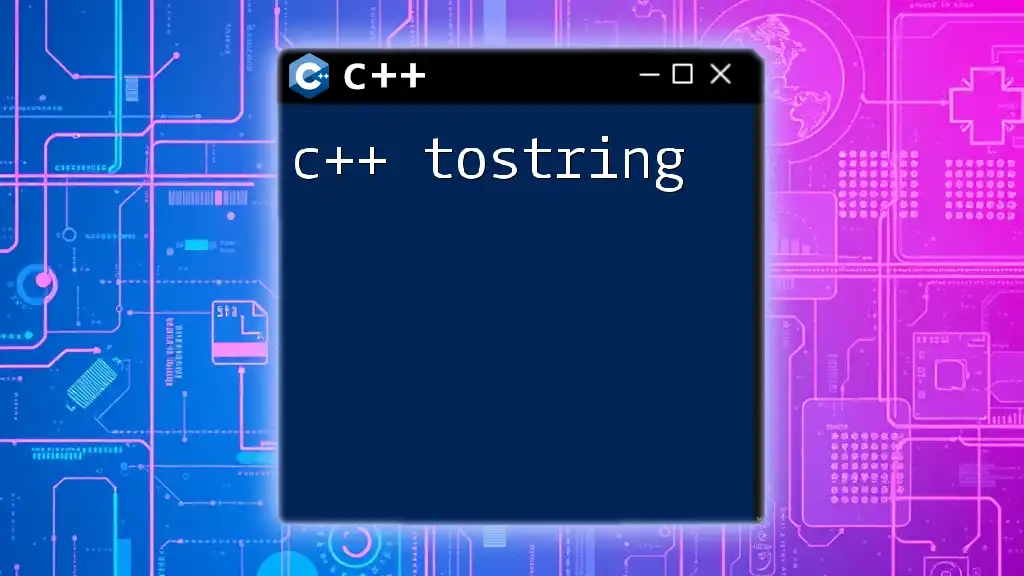
Error Handling with acos
Dealing with Invalid Inputs
It is vital to handle potential errors when using the acos function. If the input provided is outside the range of \([-1, 1]\), the function can lead to unexpected results or runtime errors. Most compilers will throw a domain error if an invalid input is processed.
Best Practices for Input Validation
To prevent such errors, it’s advisable to validate user inputs. Here’s a simple approach using conditional statements:
#include <iostream>
#include <cmath>
int main() {
double value;
std::cout << "Enter a value between -1 and 1: ";
std::cin >> value;
if (value < -1 || value > 1) {
std::cout << "Invalid input! Please provide a value between -1 and 1." << std::endl;
} else {
double result = acos(value);
std::cout << "The arccosine of " << value << " is: " << result << " radians." << std::endl;
}
return 0;
}
This validation mechanism ensures that the function only processes valid inputs, thus preventing runtime errors.
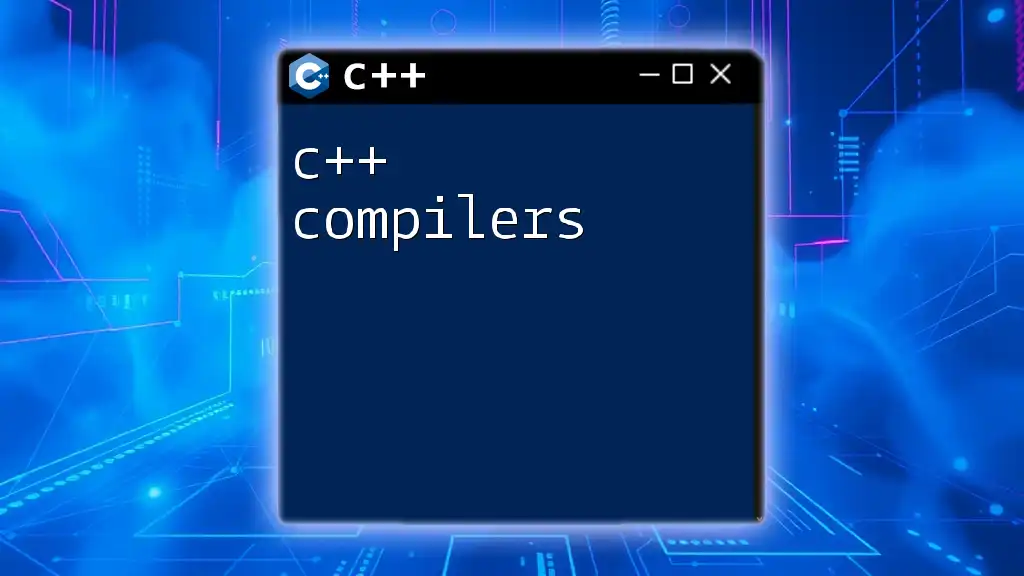
Converting Radians to Degrees
Why Conversion is Necessary
Often in programming, there is a need to convert radians to degrees for better readability or when displaying results to users. Since the output from acos is in radians, converting it can enhance user experience.
Code Example for Conversion
The conversion from radians to degrees can be implemented using the following formula:
double radiansToDegrees(double radians) {
return radians * (180.0 / M_PI);
}
int main() {
double value = 0.5;
double result = acos(value);
std::cout << "The arccosine of " << value << " in degrees is: " << radiansToDegrees(result) << " degrees." << std::endl;
return 0;
}
In this example, the `radiansToDegrees` function converts the result from radians to degrees using the factor \( \frac{180}{\pi} \). The result now becomes user-friendly, as users generally understand degrees better than radians.
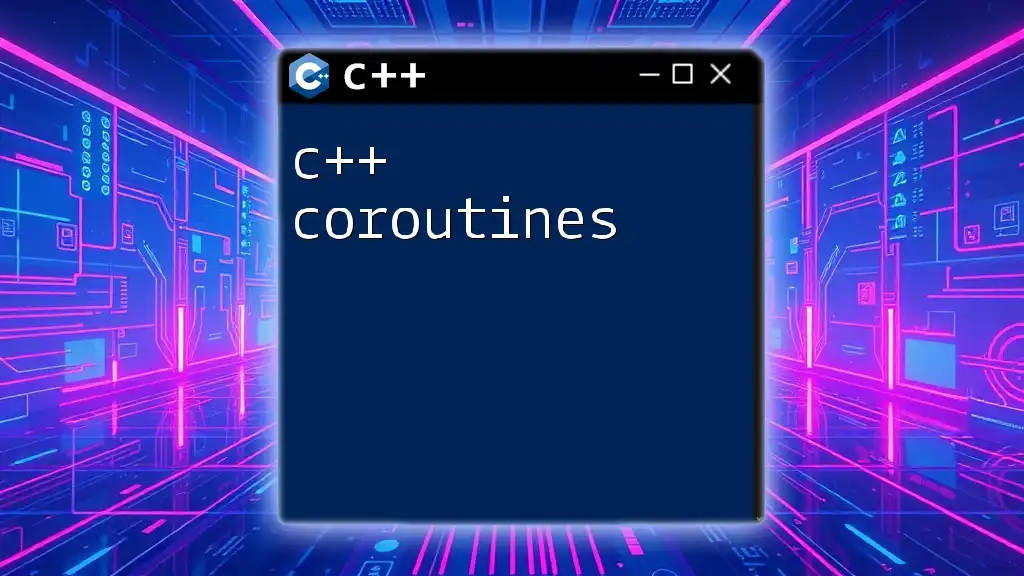
Practical Applications of acos in C++
Use Cases in Engineering
In engineering, the acos function is pivotal in systems where angles are derived from vector calculations or physical models. For example, in determining the angle between two vectors in 3D space, acos is used frequently to derive the angle from the dot product.
Graphics and Gaming
In graphics programming and game design, acos is essential for determining angles when positioning objects in virtual environments. For example, it can be used to calculate the angle between a player's viewpoint and a target, crucial for determining line-of-sight or when aiming.
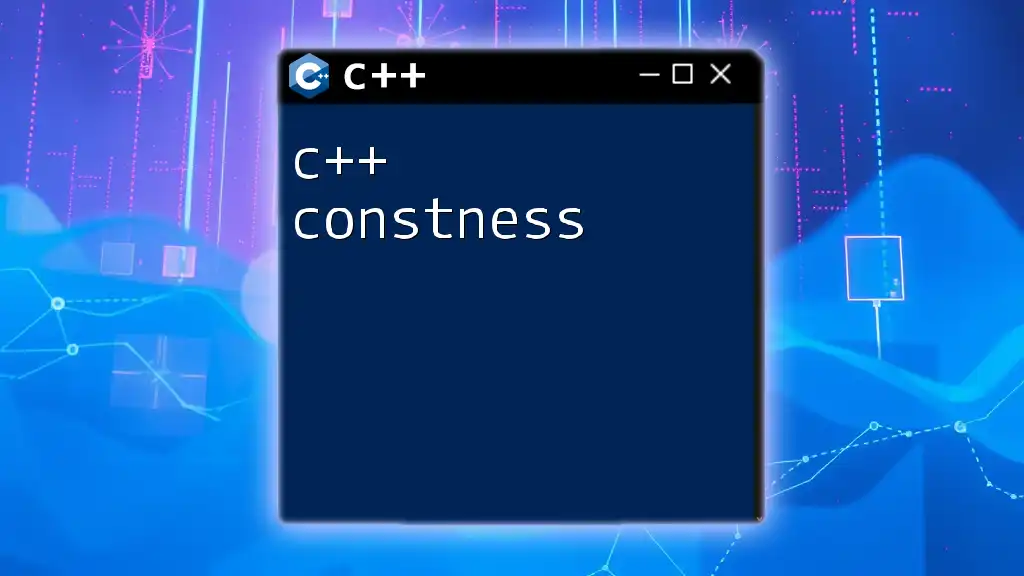
Common Pitfalls and Troubleshooting
Common Mistakes When Using acos
Developers new to trigonometric functions often make errors such as forgetting to account for the input range of acos. This oversight can lead to the program crashing or producing incorrect outputs. Always ensure inputs are validated.
Fixing Floating-point Precision Issues
Floating-point numbers can be tricky due to how they are represented in memory. When using acos, small errors in precision can lead to results that are slightly outside the expected range. To address this, consider using rounding techniques or setting a tolerance threshold when validating inputs.
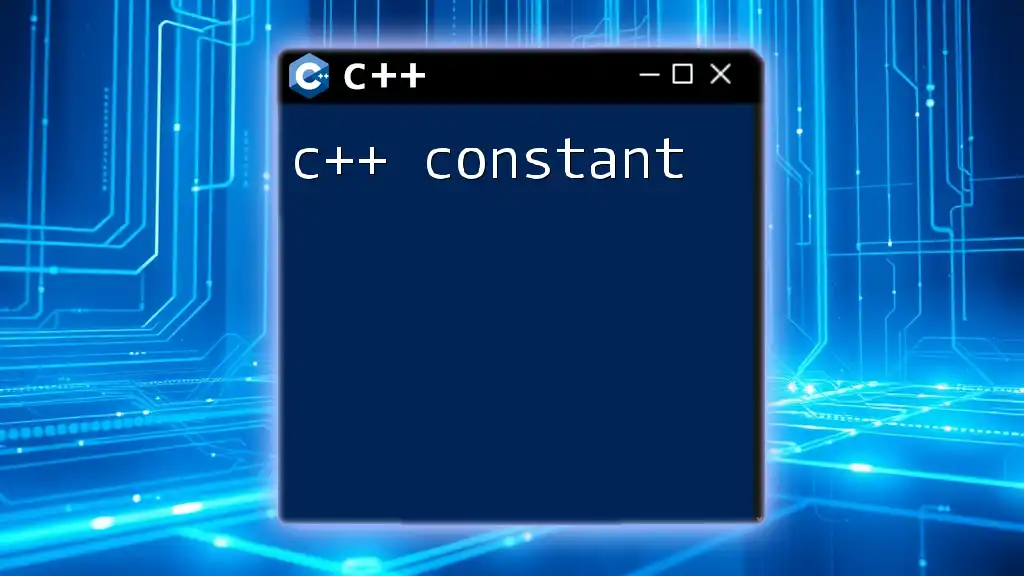
Conclusion
In summary, the C++ acos function plays a critical role in mathematical computations involving angles. By understanding its syntax, how to handle errors, and applying it practically, you can leverage this function in various domains.
Experimentation is key; try implementing acos in your own projects or exercises. As you deepen your knowledge, staying informed with additional resources can further enhance your programming skills related to trigonometric functions.
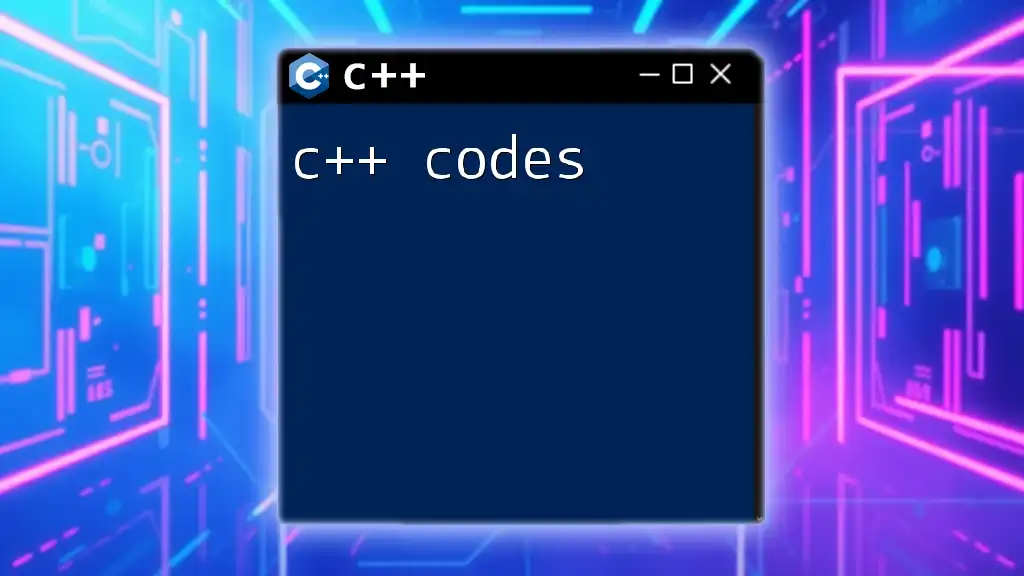
Frequently Asked Questions (FAQs)
What is arccosine in relation to C++?
Arccosine in C++ denotes the inverse operation of the cosine function, implemented through the acos function, enabling developers to calculate angles based on given cosine values.
When should I use acos?
You should use acos when you need to determine an angle whose cosine value is known, particularly in fields like engineering, mathematics, and computer graphics.
Can I use acos with types other than double?
The acos function is defined to accept `double` as its argument, but C++ allows implicit type conversions. However, it is recommended to work with `double` for higher precision when dealing with trigonometric functions.