The "bmp c++" topic involves working with bitmap image files in C++, allowing you to read and manipulate pixel data effectively.
Here's a simple example of loading and displaying a BMP image's header:
#include <iostream>
#include <fstream>
#pragma pack(push, 1) // Ensure the structure is packed
struct BMPHeader {
uint16_t file_type; // File type, should always be "BM"
uint32_t file_size; // Size of the file in bytes
uint16_t reserved1; // Reserved; must be 0
uint16_t reserved2; // Reserved; must be 0
uint32_t offset_data; // Offset to image data
};
int main() {
std::ifstream bmp_file("example.bmp", std::ios::binary);
BMPHeader header;
if (bmp_file.read(reinterpret_cast<char*>(&header), sizeof(header))) {
std::cout << "File Type: " << header.file_type << std::endl;
std::cout << "File Size: " << header.file_size << std::endl;
std::cout << "Data Offset: " << header.offset_data << std::endl;
}
bmp_file.close();
return 0;
}
This code snippet reads the BMP file header and outputs its essential components.
What is a BMP File?
A BMP (Bitmap) file is a raster graphics image file format used to store bitmap digital images. BMP files are commonly utilized in software applications for displaying high-quality images due to their simplicity and lack of compression, which preserves image quality. They are extensively used in Windows environments and are favored by software developers when manipulating graphics.
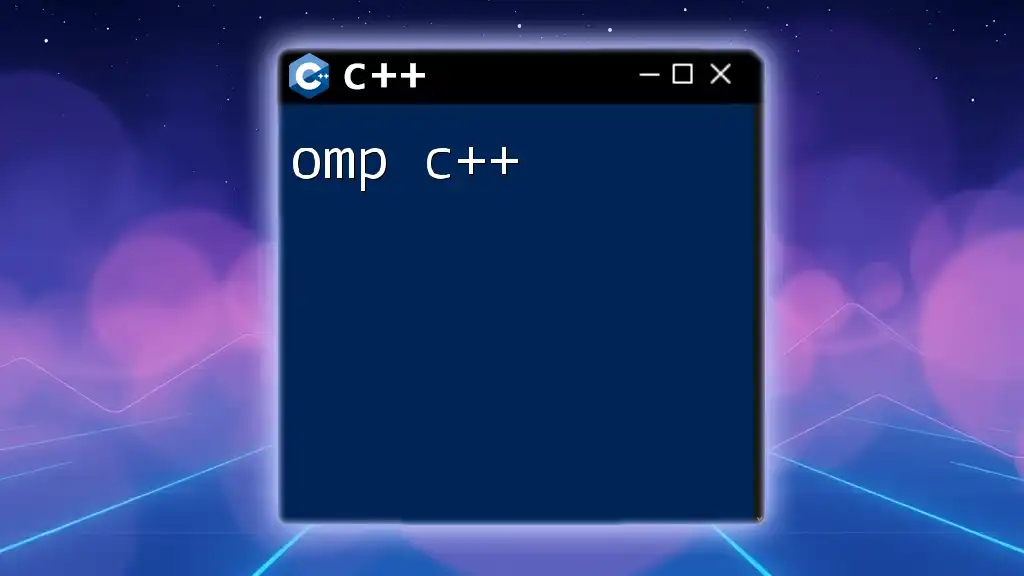
Characteristics of BMP Files
Uncompressed vs. Compressed Formats
BMP files are principally uncompressed, leading to larger file sizes compared to other formats such as JPEG or PNG. This results in high fidelity and no loss in image quality during saving. However, the trade-off is that BMP files may require significantly more storage space.
Key Attributes: Pixel Depth, Headers, and Metadata
BMP files offer versatile pixel depth options: typically 1, 4, 8, 16, 24, and 32 bits per pixel. Higher pixel depths afford a wider range of colors, directly impacting image detail and quality. BMP structures contain two important headers—the BMP header and the DIB (Device Independent Bitmap) header—which store essential metadata about the image, such as dimensions and compression methods.
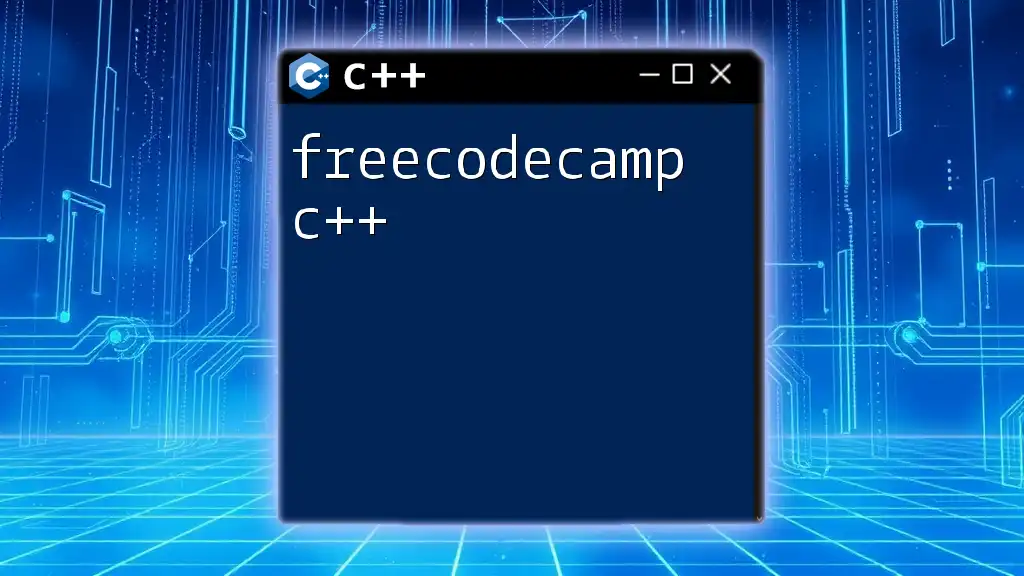
Understanding the BMP File Structure
BMP File Header
The BMP file header is crucial as it defines the format and layout of the entire file. It specifies the type of file (generally identified as "BM" or 0x4D42), image size, and pointers to important data within the file.
DIB Header (Device Independent Bitmap)
The DIB header provides detailed information about the bitmap data. It can vary in size, but the BITMAPINFOHEADER is commonly used. This header defines characteristics like the width and height of the bitmap, number of color planes, and pixel format.
Pixel Array
The pixel array section of a BMP file is where the actual image data is stored. Pixels can represent various formats, such as RGB or Grayscale. Each pixel’s representation depends on the defined pixel depth. In a 24-bit BMP, for instance, each pixel comprises three bytes: one each for red, green, and blue.
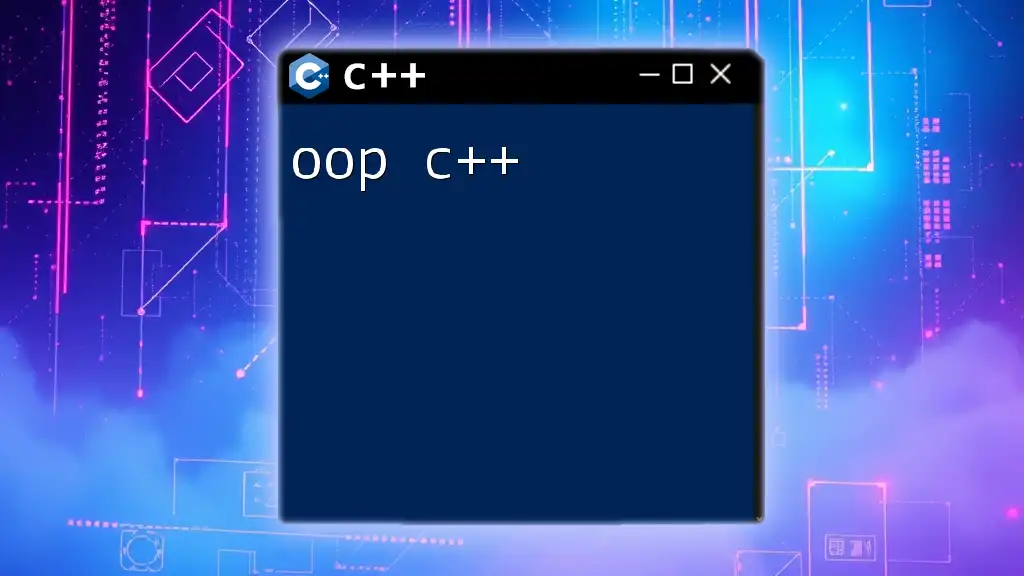
Reading BMP Files in C++
Setting Up the Environment
To read BMP files in C++, you need to include necessary libraries like `<fstream>` for file operations and `<iostream>` for console input and output. Ensure your compiler supports C++ standards that facilitate file manipulation.
Step-by-Step Code to Read a BMP File
Below is a sample implementation to read a BMP file:
#include <iostream>
#include <fstream>
#include <cstdint>
#include <vector>
// Define structures for BMP headers
#pragma pack(push, 1)
struct BMPHeader {
uint16_t bfType;
uint32_t bfSize;
uint16_t bfReserved1;
uint16_t bfReserved2;
uint32_t bfOffBits;
};
struct DIBHeader {
uint32_t biSize;
int32_t biWidth;
int32_t biHeight;
uint16_t biPlanes;
uint16_t biBitCount;
uint32_t biCompression;
uint32_t biSizeImage;
int32_t biXPelsPerMeter;
int32_t biYPelsPerMeter;
uint32_t biClrUsed;
uint32_t biClrImportant;
};
#pragma pack(pop)
void readBMP(const char* filename) {
std::ifstream bmpFile(filename, std::ios::binary);
if (!bmpFile) {
std::cerr << "Error opening file!" << std::endl;
return;
}
BMPHeader bmpHeader;
bmpFile.read(reinterpret_cast<char*>(&bmpHeader), sizeof(bmpHeader));
if (bmpHeader.bfType != 0x4D42) {
std::cerr << "Not a valid BMP file!" << std::endl;
return;
}
DIBHeader dibHeader;
bmpFile.read(reinterpret_cast<char*>(&dibHeader), sizeof(dibHeader));
std::cout << "Width: " << dibHeader.biWidth << ", Height: " << dibHeader.biHeight << std::endl;
// Additional code to read the pixel array will go here
bmpFile.close();
}
int main() {
readBMP("example.bmp");
return 0;
}
Explanation of the Code
In this code snippet, we define the `BMPHeader` and `DIBHeader` structures to encapsulate the relevant data from the BMP file. We then create a function `readBMP` that opens and reads the BMP file, validating its type. The console output displays key image dimensions, aiding in further processing of the pixel data.
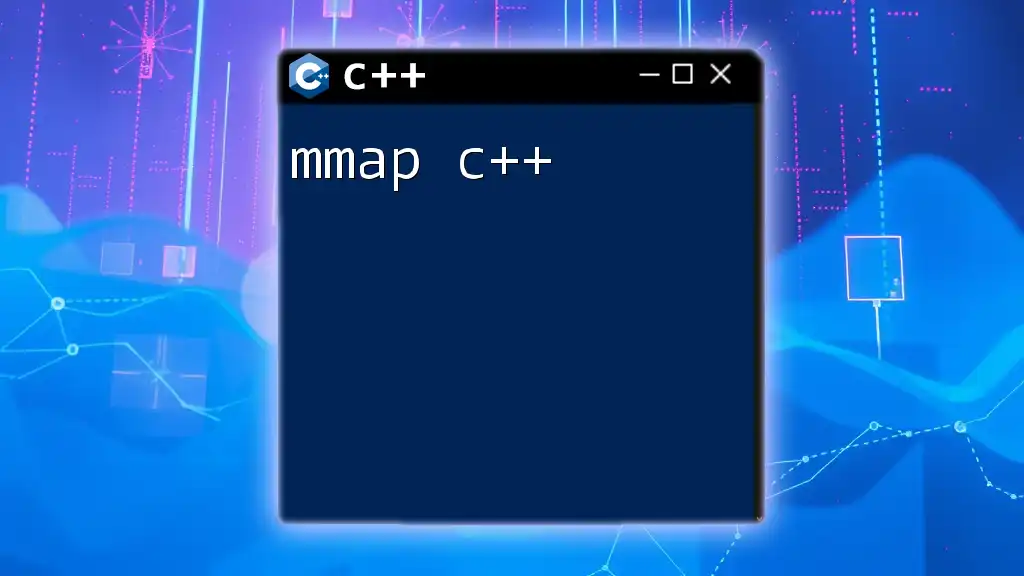
Writing BMP Files in C++
Creating a BMP File Structure
When writing a BMP file, it's essential to create the necessary headers and populate them with relevant data. You need to determine the pixel format and ensure the pixel data is represented correctly in memory.
Step-by-Step Code to Write a BMP File
The following example demonstrates how to create and write a BMP file:
#include <vector>
#include <fstream>
void writeBMP(const char* filename, const std::vector<uint8_t>& pixelData, int width, int height) {
BMPHeader bmpHeader = {};
DIBHeader dibHeader = {};
// Populate headers with correct data
bmpHeader.bfType = 0x4D42; // 'BM'
bmpHeader.bfSize = sizeof(BMPHeader) + sizeof(DIBHeader) + pixelData.size();
bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(DIBHeader);
dibHeader.biSize = sizeof(DIBHeader);
dibHeader.biWidth = width;
dibHeader.biHeight = height;
dibHeader.biPlanes = 1;
dibHeader.biBitCount = 24; // Assuming 24 bits per pixel
dibHeader.biCompression = 0; // No compression
std::ofstream bmpFile(filename, std::ios::binary);
bmpFile.write(reinterpret_cast<char*>(&bmpHeader), sizeof(bmpHeader));
bmpFile.write(reinterpret_cast<char*>(&dibHeader), sizeof(dibHeader));
bmpFile.write(reinterpret_cast<const char*>(pixelData.data()), pixelData.size());
bmpFile.close();
}
int main() {
// Example pixel data (red square)
std::vector<uint8_t> pixels(100 * 100 * 3, 0);
for (int y = 0; y < 100; ++y) {
for (int x = 0; x < 100; ++x) {
pixels[(y * 100 + x) * 3 + 0] = 255; // R
pixels[(y * 100 + x) * 3 + 1] = 0; // G
pixels[(y * 100 + x) * 3 + 2] = 0; // B
}
}
writeBMP("output.bmp", pixels, 100, 100);
return 0;
}
Explanation of Writing Code
This snippet defines a function `writeBMP` that constructs the BMP and DIB headers based on the provided pixel data. It calculates the total file size and successfully writes the headers and pixel array to a new BMP file.
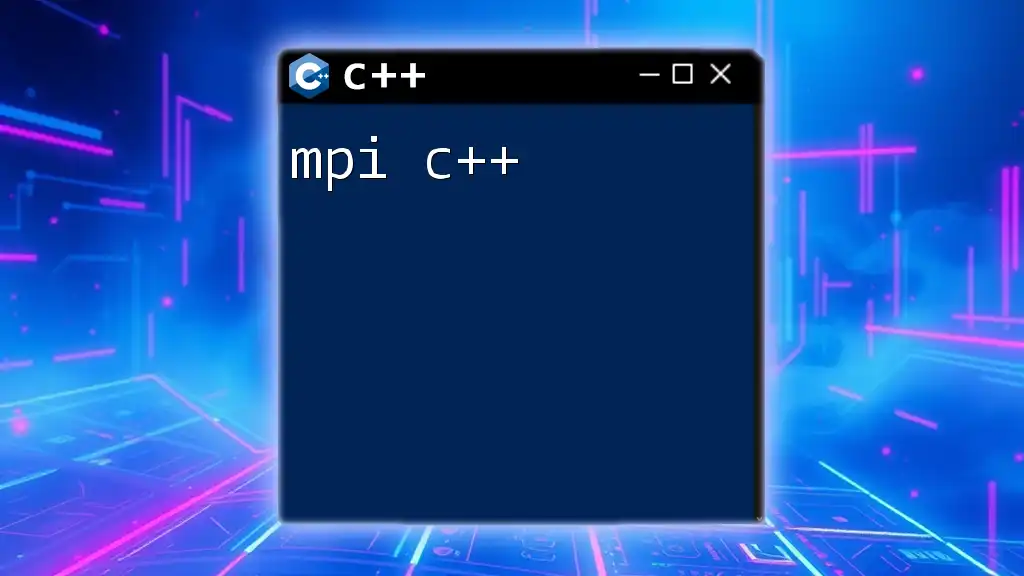
Manipulating BMP Files with C++
Image Processing Basics
Manipulating BMP files allows for various image processing techniques, such as rotating, flipping, resizing, and color adjustments. Understanding pixel manipulation at this level grants developers enhanced control over how images are rendered and displayed.
Example: Flipping BMP Vertically
You can create a function to flip a BMP image vertically. The logic will involve reading the pixel array, swapping pixel rows, and then writing the modified array to a new file.
void flipBMP(const char* inputFile, const char* outputFile) {
// Read the input file (similar to readBMP)
// Retrieve the pixel data
// Flip the pixel array vertically
// Write the modified pixel array to output file (similar to writeBMP)
}
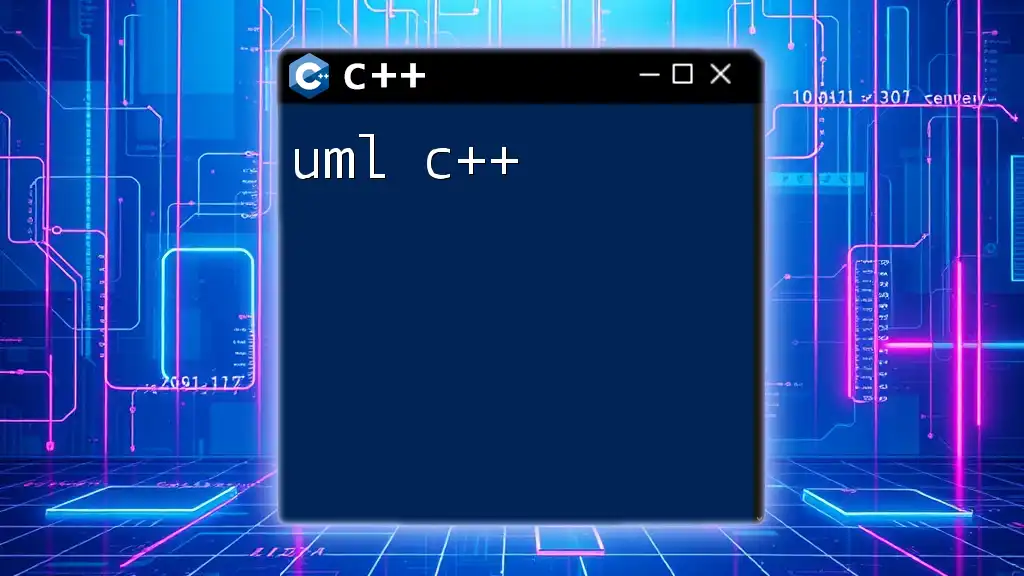
Recap of Key Concepts
In this guide, we explored the BMP file format and its significance in graphics programming. We delved into the file's structure, learned how to read and write BMP files using C++, and even covered basic image manipulation techniques. Understanding BMP files enhances your capability to tailor graphics applications, making you an effective programmer.
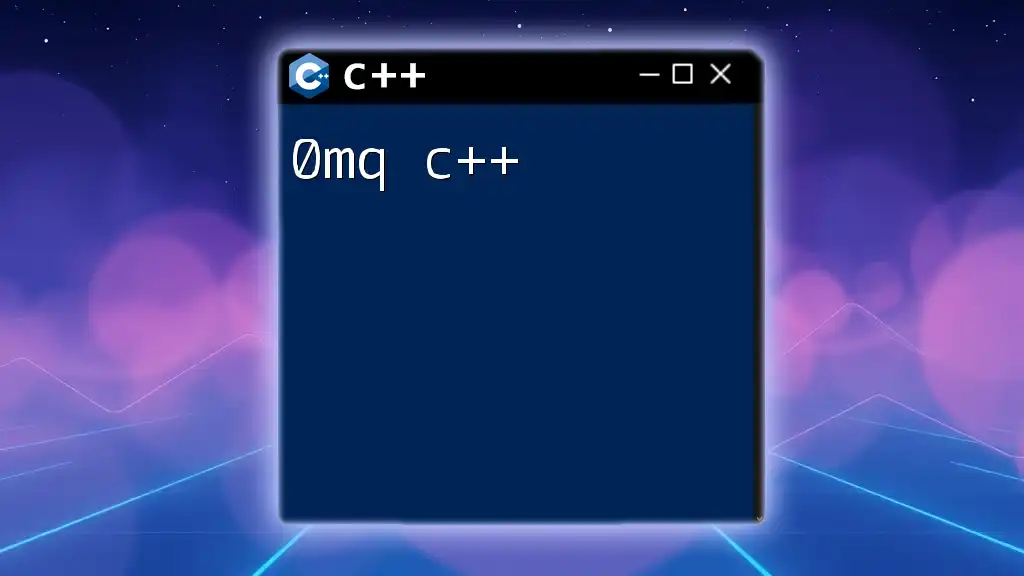
Further Reading and Resources
For those eager to dive deeper into C++ graphics programming and BMP manipulation, consider exploring additional resources such as:
- Textbooks focused on graphics programming
- Online coding platforms with C++ tutorials
- Libraries like OpenCV for advanced image processing capabilities