Unreal Engine 5 (UE5) utilizes C++ for high-performance game development, allowing developers to create custom gameplay elements and enhance game mechanics seamlessly.
Here's a simple example of a C++ class in UE5 that creates a basic actor:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Understanding Unreal Engine 5
What is Unreal Engine 5?
Unreal Engine 5 (UE5) is a powerful game development platform by Epic Games, widely recognized for its robust features and capabilities. It allows developers to create stunning, high-fidelity visuals and immersive interactive experiences. With its advanced tools, such as Nanite for virtualized geometry and Lumen for real-time global illumination, UE5 enables the creation of visually impressive environments without the need for extensive manual optimization.
Why Use C++ in UE5?
Using C++ in UE5 significantly enhances your game development process for several reasons:
-
Performance: C++ is closer to the hardware level and offers high performance suitable for complex gameplay mechanics.
-
Control: By programming in C++, you gain granular control over game mechanics, allowing you to implement complex algorithms and optimize performance.
-
Community Support: The UE5 community is vast, with numerous resources, third-party libraries, and forums available for assistance and collaboration.
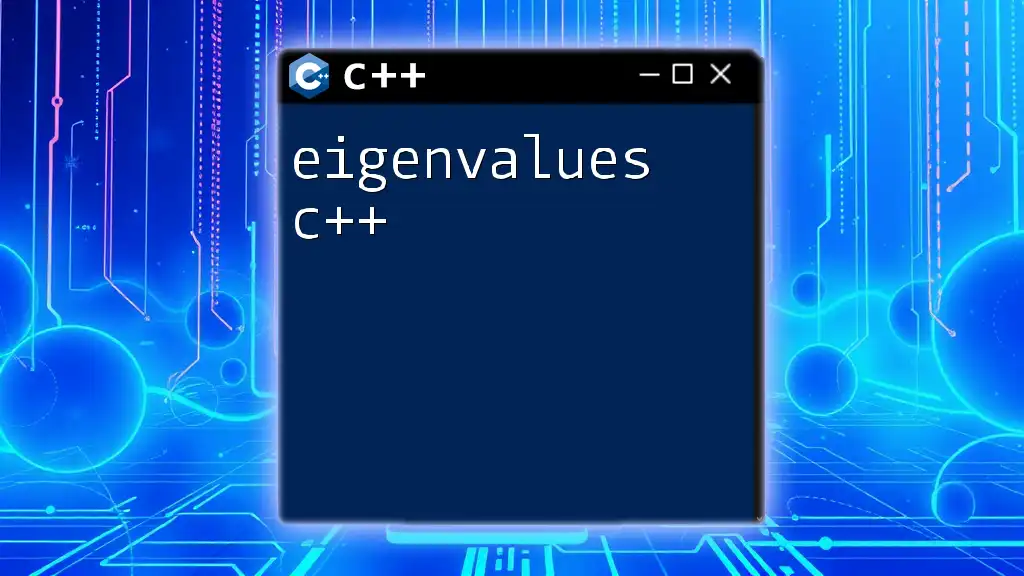
Setting Up Your Development Environment
Required Software
To get started with UE5 and C++, download and install Unreal Engine 5 from the Epic Games launcher. It is also recommended to install an Integrated Development Environment (IDE) for coding. Some of the best IDEs for C++ development with UE5 include:
-
Visual Studio: The most commonly used IDE, offering extensive C++ support and integration with UE.
-
Rider for Unreal: A cross-platform IDE tailored for Unreal Engine development, providing unique features for a streamlined coding experience.
Configuring Your IDE
After installing your chosen IDE, configure it to work seamlessly with UE5:
- Set up project files by creating a new C++ project in Unreal Engine.
- Ensure that your IDE is integrated with UE5, allowing it to recognize the project structure.
- Organize your code files within appropriate folders, such as placing specialized code in the “Source” directory to maintain clarity and consistency.
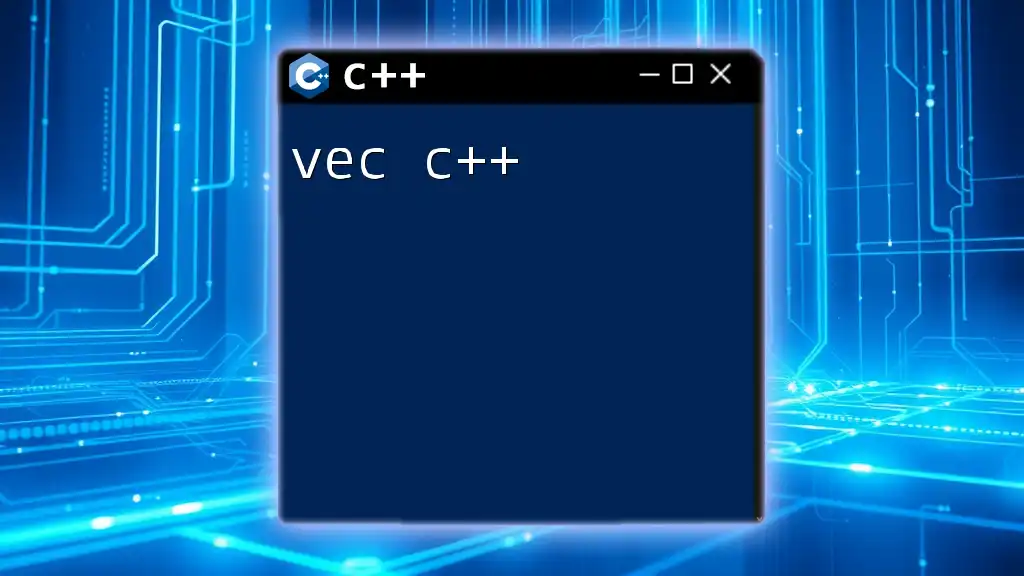
Fundamentals of C++ in UE5
Understanding the Basics of C++
Before diving deeper, it’s essential to understand some foundational concepts of C++:
-
Variables and Data Types: Variables store data. Understanding types—like `int`, `float`, and `string`—is fundamental in defining what kind of data you're working with.
-
Control Structures: These allow for decision-making within your programs. Use `if` statements for conditional logic and loops (`for`, `while`) for repetitive tasks.
-
Functions and Classes: Functions are blocks of code designed to perform tasks, while classes are blueprints for creating objects, encapsulating data, and methods that manipulate that data.
UE5 C++ Project Structure
Understanding the project structure within UE5 is vital for efficient development. The main components include:
-
Source Folder: Contains all the C++ source files. The folder structure is usually organized by modules.
-
Config Folder: Holds configuration files that determine settings for the entire project.
-
Plugins: Extensible modules that add additional features to UE5, allowing for broader capabilities.
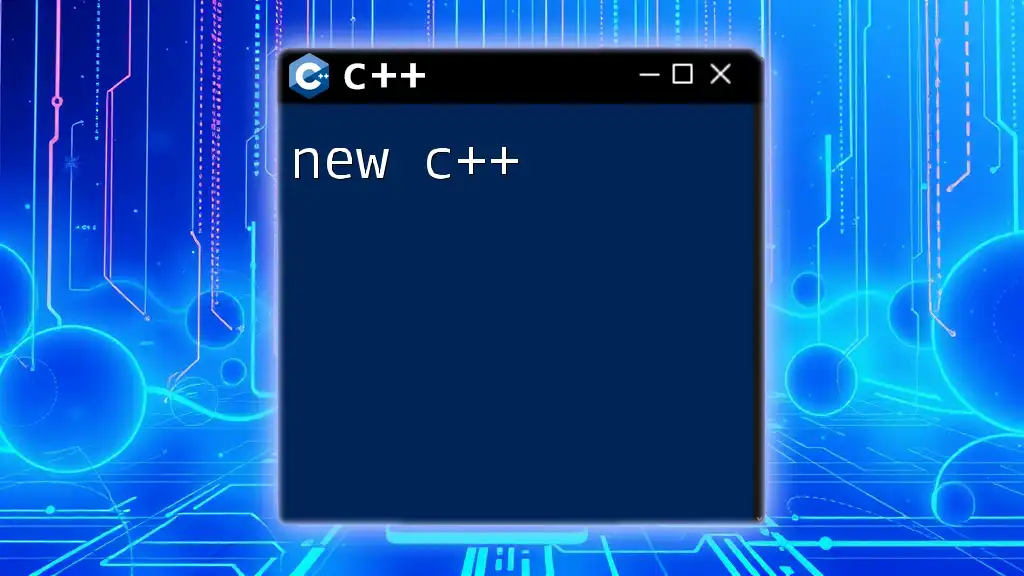
Creating Your First C++ Class in UE5
Setting Up a New C++ Class
To kickstart your programming journey in UE5, you need to create a new C++ class. Here’s a step-by-step guide:
- Open Unreal Engine and create a new project or open an existing one.
- Go to the menu bar, click on Add New, select C++ Class.
- Choose Actor as the parent class, name it (e.g., `MyActor`), and click Create Class.
Code Snippet: Basic Actor Class
Now let's see how a basic Actor class looks:
#include "MyActor.h"
AMyActor::AMyActor()
{
PrimaryActorTick.bCanEverTick = true;
}
void AMyActor::BeginPlay()
{
Super::BeginPlay();
}
void AMyActor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
In this code:
- `AMyActor::AMyActor()` is the constructor where you can initialize any properties.
- `BeginPlay()` is called when the game starts, useful for starting configurations.
- `Tick()` is called every frame, providing a way to update your object continuously.
Compiling and Testing Your Class
After creating your class, it’s time to compile and test it:
- Click on Compile in the toolbar of the UE5 editor after making changes to your C++ class.
- Once your project compiles successfully, drag your Actor (`MyActor`) into the level from the content browser.
- Press the Play button and observe your Actor in action within the game world.
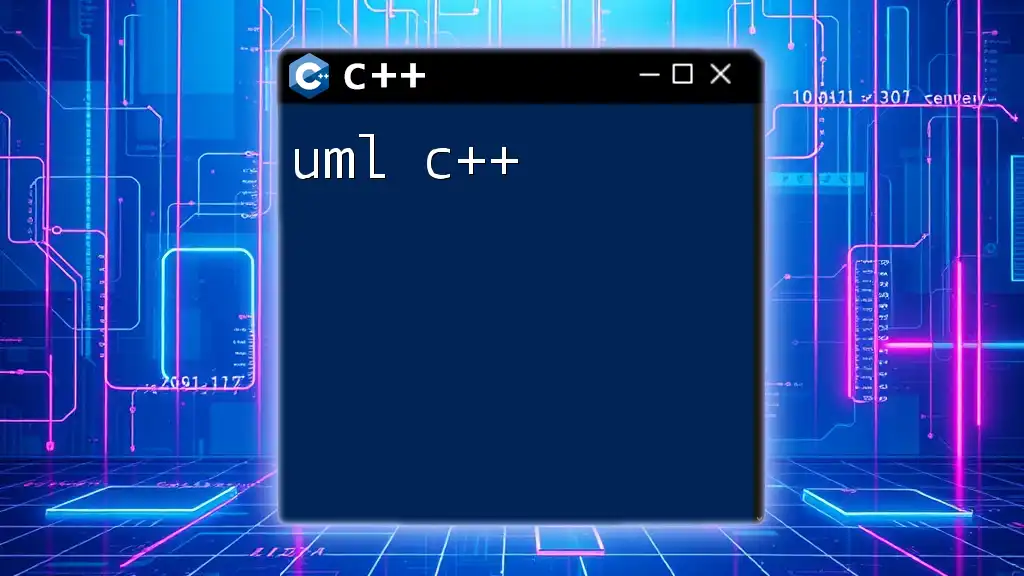
Key Features of C++ in UE5
Blueprints vs C++
UE5 offers a hybrid development environment where you can choose between C++ and Blueprints (the visual scripting system):
-
Blueprints: Ideal for designers and those not deeply familiar with programming. It enables rapid prototyping and iteration on gameplay mechanics.
-
C++: Best for performance-critical tasks or scenarios requiring complex computations. You can extend Blueprint functionality with custom C++ code, providing the best of both worlds.
Memory Management in C++
Memory management is critical in game development for performance and stability. C++ uses pointers to manage memory allocation:
Utilize smart pointers to manage memory effectively, reducing the chances of memory leaks:
Code Example: Using Smart Pointers
TSharedPtr<MyClass> MyObject = MakeShareable(new MyClass());
Here, `TSharedPtr` helps manage memory allocation and deallocation automatically, ensuring that resources are freed when no longer needed.
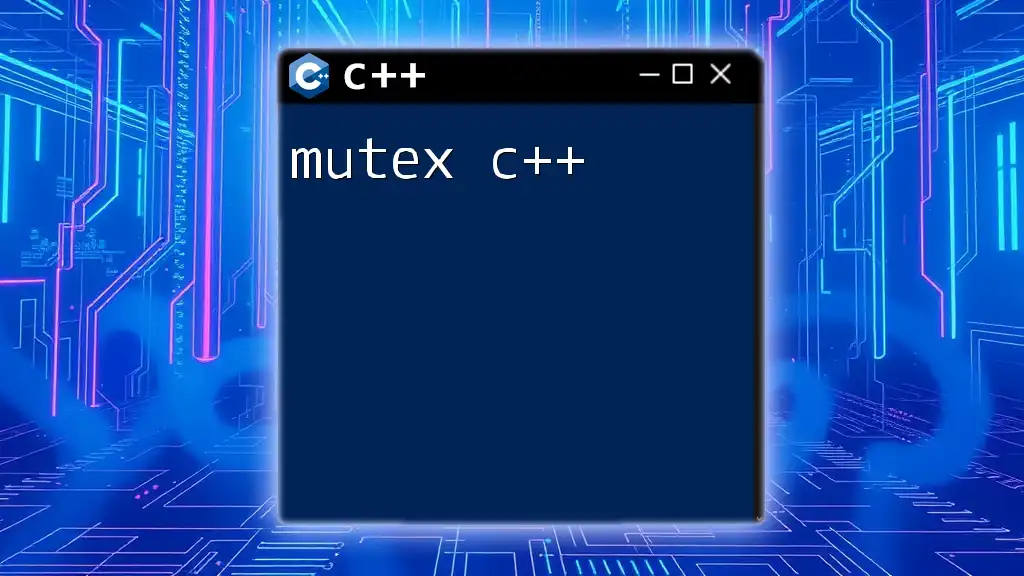
Interfacing C++ with UE5 Components
Working with Actors and Components
UE5 allows for seamless interaction between C++ code and game components:
-
Actors represent objects that can be placed in the game world. You can specify behaviors, appearances, and interactions for them via C++.
-
Custom Components can be created to add specific functionalities to Actors without modifying the Actor class directly.
Events and Delegates
Working with events in C++ allows for responsive game design. UE5 uses delegates to bind functions to events:
For example, if you want a button to call a function when clicked, you can set it up like this:
Code Snippet: Event Binding
Button->OnClicked.AddDynamic(this, &AMyActor::OnButtonClicked);
This line binds the button's click event to the `OnButtonClicked` function within your Actor class. Creating dynamic links between events and functions significantly enhances interactivity.
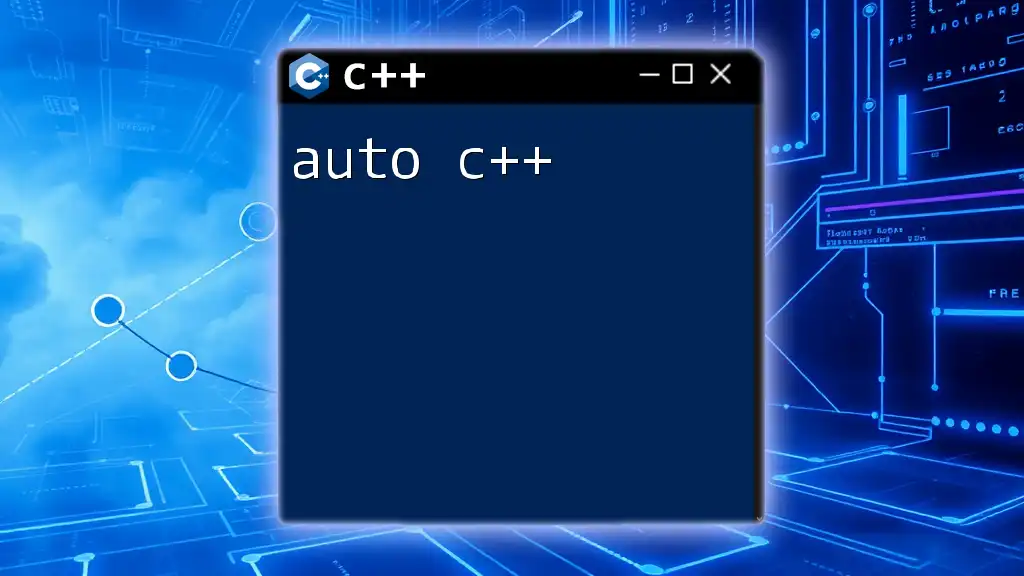
Enhancing Your C++ Skills in UE5
Best Practices for C++ Coding in UE5
To write clean and efficient C++ code in Unreal Engine:
- Follow coding standards and maintain consistent naming conventions.
- Avoid using global variables to enhance modularity and maintainability.
- Comment your code to explain complex logic, improving readability for yourself and other developers.
Resources for Further Learning
To further your knowledge in C++ programming for UE5:
- Explore Unreal Engine documentation, which offers extensive guidelines and tutorials.
- Consider enrolling in reputable online courses focused on UE5 and C++ development.
- Engage with the community through forums and social media platforms to exchange ideas and solutions.
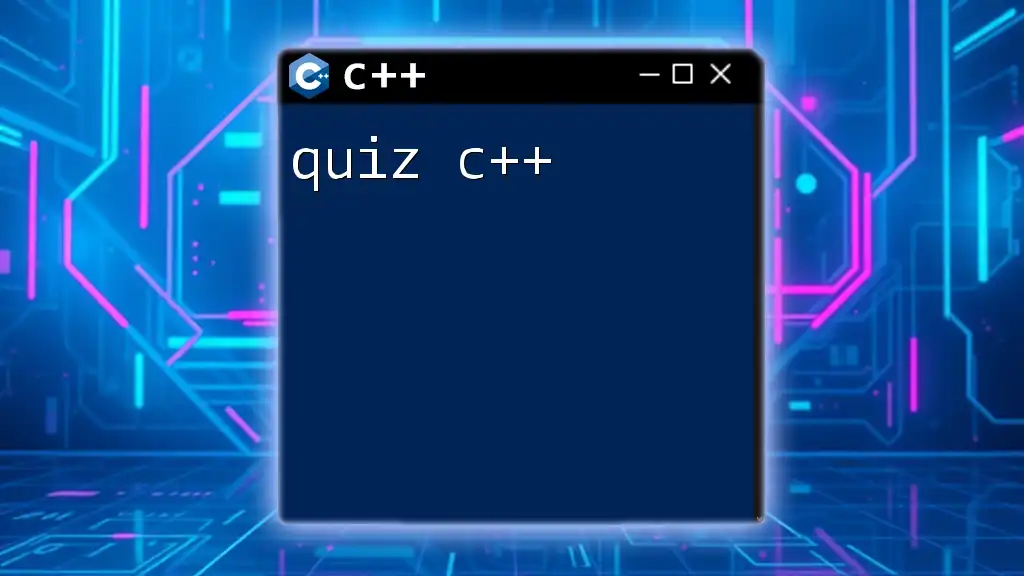
Conclusion
In this guide, we explored the critical aspects of using C++ in Unreal Engine 5, including the setup of your development environment, basics of C++, creating your first class, and utilizing key features of UE5. Embracing C++ can substantially elevate your game development skills, allowing you to harness the full power of UE5.
Practice is essential, so start experimenting with your C++ projects in UE5 today and unlock the potential that lies within this powerful game engine. Join our community to stay updated on more tutorials and resources designed to help you master the art of C++ programming in Unreal Engine 5.