C++ was first introduced by Bjarne Stroustrup in 1983 as an enhancement to the C programming language, incorporating object-oriented features.
Here's a simple C++ code snippet demonstrating a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Birth of C++
Starting off with the origins of C, we cannot overlook the influence C had on programming languages in the 1970s. Developed by Dennis Ritchie at Bell Labs, C emerged as an essential tool for system programming and Unix development. Its procedural paradigm and powerful features made it widely popular, and as developers began to see the benefits of object-oriented programming (OOP), the seeds for C++ were sown.
The Vision of Bjarne Stroustrup
Bjarne Stroustrup, a Danish computer scientist, envisioned a powerful extension to the C language. He started this journey in the late 1970s, initially developing a new language called C with Classes at Bell Labs in 1979. Stroustrup aimed to integrate the benefits of OOP into C without losing the language's efficiency or its low-level capabilities. Recognizing the potential for better software design and maintainability, he sought to create a language that offered both high-level abstractions and performance, a vision that ultimately led to the creation of C++.
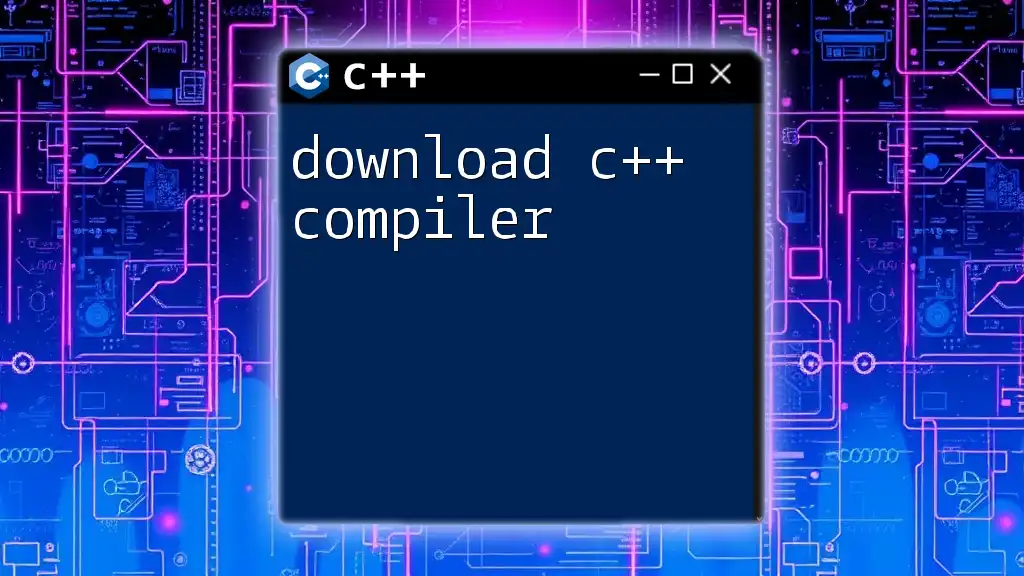
The Initial Release of C++
C++ officially made its debut in 1985 with the release of "The C++ Programming Language." This initial version was both a continuation and an enhancement of C with Classes. Stroustrup’s aim was to create a language that could handle complex data structures and promote more abstract thinking while retaining C's efficiency.
Features Introduced in the First Version
The first version of C++ brought several noteworthy features to the table, including:
- Classes and Objects: The ability to create user-defined types that encapsulated data and behaviors.
- Basic Inheritance: Allowing developers to create new classes based on existing ones, thus promoting code reuse.
- Function Overloading: Introducing the capacity for multiple functions to share the same name but differ in parameter types.
Here is a simple example of defining a class in C++:
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
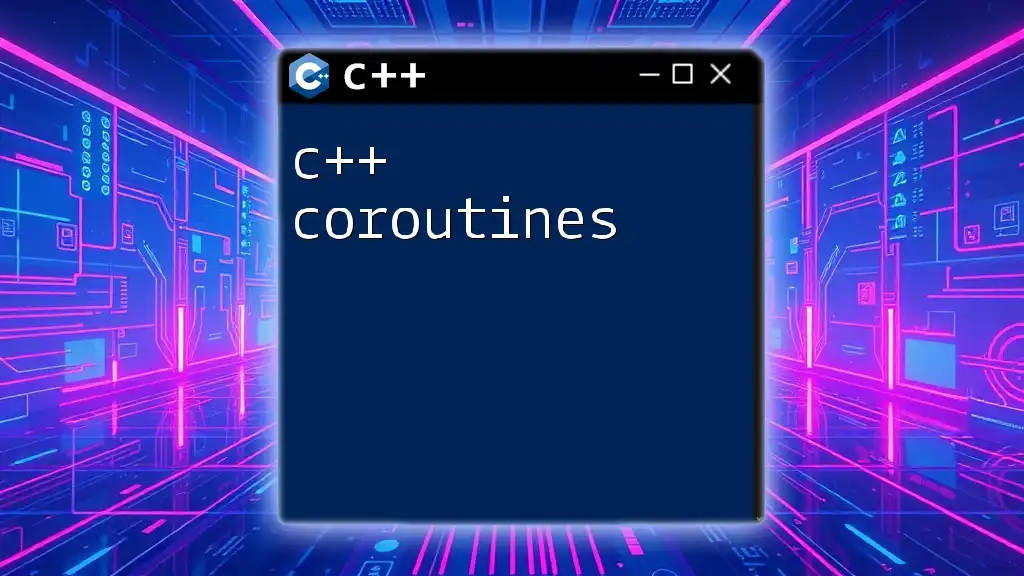
The Evolution: C++98 and Beyond
After its introduction, C++ experienced a period of evolution that led to the standardization process. In 1998, C++ became an official ISO standard, referred to as C++98. This monumental step marked C++’s transition from a proprietary language to a universally accepted standard.
C++98 and Key Additional Features
C++98 introduced several critical features including:
- Templates: Enabling generic programming, allowing functions and classes to operate with any data type.
- Exception Handling: Providing a robust mechanism to handle errors and exceptional situations.
A simple example of template usage in C++ is as follows:
template <typename T>
T add(T a, T b) {
return a + b;
}
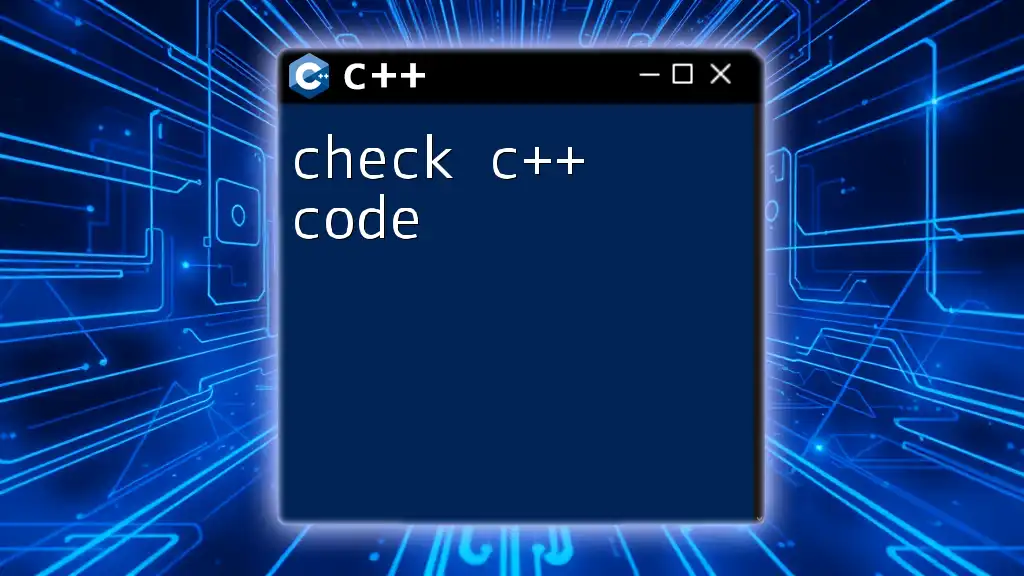
Major C++ Standards Over the Years
The C++ Standardization Process
The process of standardization for C++ involved the ISO C++ committee, which plays a crucial role in affirming and enhancing the language over time. New features and enhancements are proposed, debated, and refined, ensuring the language stays relevant and powerful for modern programming needs.
C++03: An Update
In 2003, a minor update known as C++03 was released. This version concentrated on fixing technical issues and ensuring better consistency without introducing major features. The update was essential in clarifying ambiguities and improving the overall language quality.
C++11: A Major Leap
The release of C++11 marked a pivotal moment for the language, introducing a flurry of new features that significantly enhanced productivity and performance. Key features include:
- Range-based for Loops: Simplifying the syntax when iterating through collections.
- Lambda Expressions: Allowing the creation of anonymous functions.
- Smart Pointers: Introducing automatic memory management with features like `std::unique_ptr` and `std::shared_ptr`.
Here's an example of a lambda function in C++11:
auto add = [](int a, int b) -> int {
return a + b;
};
C++14 to C++20: Evolutionary Steps
C++ continued to evolve with further updates:
- C++14 further relaxed the constraints on `constexpr` functions, enabling more compile-time computations.
- C++17 introduced features like `std::optional`, `std::variant`, and enhancements in standard library algorithms.
- C++20 brought ground-breaking changes, such as concepts for constraining templates and ranges for simplifying data manipulation.
A simple code snippet using `std::optional` in C++17 might look like:
#include <optional>
std::optional<int> findValue(int key) {
// Assume we search for a value and return it as an optional
return std::nullopt; // Represents no result found
}
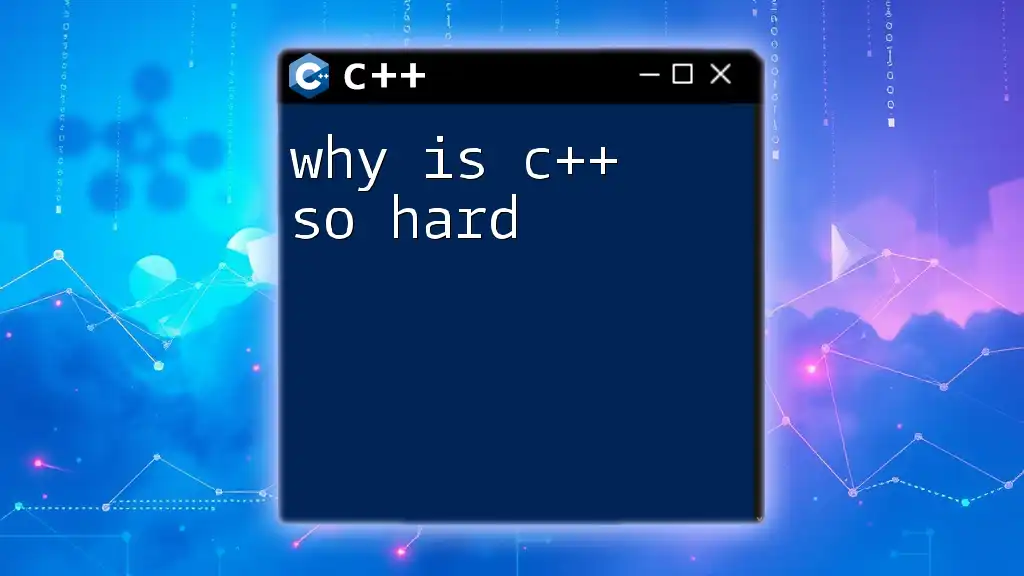
The Role of C++ in Today’s Programming Landscape
Popularity and Usage
C++ continues to hold a vital place in the programming landscape as it remains widely used in areas that demand high performance and control, such as:
- Game Development: Many popular game engines like Unreal Engine are built using C++.
- System Programming: Operating systems and compilers extensively utilize C++ for their development.
C++ in the Era of Modern Programming
Even with the rise of newer languages such as Python and Java, C++ remains relevant. Its ability to manage hardware resources and perform at high speeds gives it a unique position that many modern languages do not fulfill. C++ libraries continue to evolve, helping programmers solve complex problems while maintaining control over system resources.
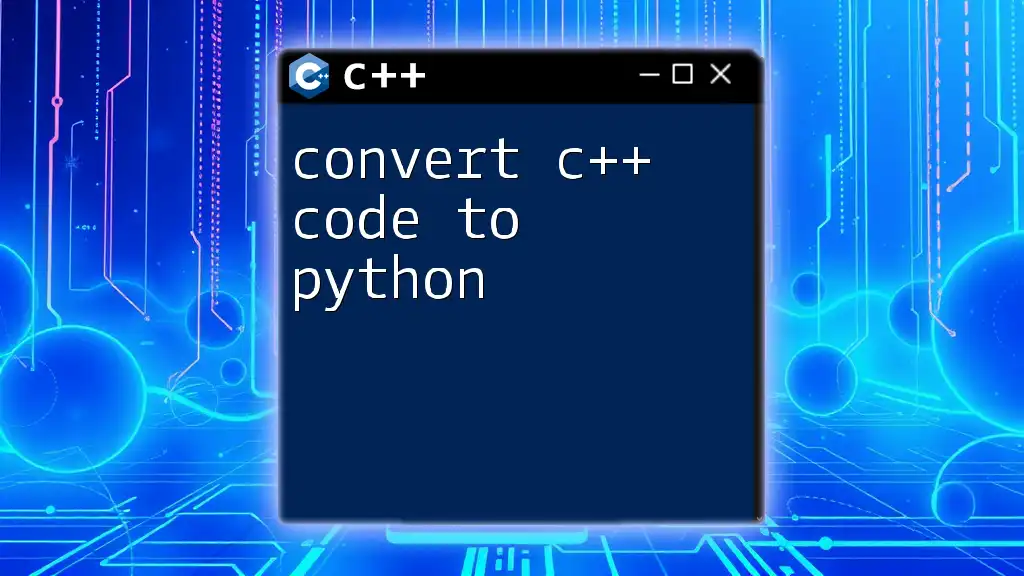
Learning C++
Why Learn C++?
Learning C++ is beneficial for various reasons:
- Foundation for Other Languages: Many programming languages are influenced by C++. A solid understanding of C++ helps in grasping other languages more easily.
- Performance: C++ allows fine control over system resources, making it an excellent choice for performance-critical applications.
Resources for Learning C++
There are numerous resources available for those looking to learn C++. Recommended materials include classic books like "The C++ Programming Language" by Bjarne Stroustrup, online courses through platforms like Coursera, and community-validated resources from sites like Stack Overflow and GitHub. Joining C++ forums and groups emphasizes collaboration and shared learning experiences, broadening one’s understanding of the language.
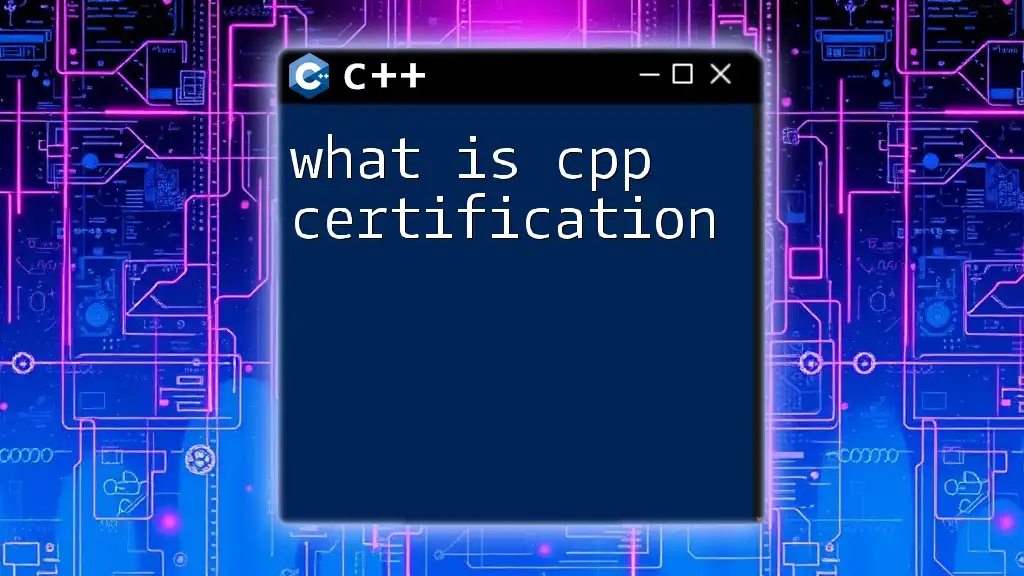
Conclusion
To summarize, C++ officially came out in 1985 and has undergone incredible transformations since then. From its inception as C with Classes to becoming a standardized language in C++98, and through numerous enhancements in subsequent versions, it remains relevant and widely used in various sectors of technology today. Understanding its history not only enriches your programming knowledge but also positions you to utilize C++ effectively in real-world applications. As you embark on your C++ learning journey, remember that the ability to harness both its low-level capabilities and high-level programming paradigms will set you apart as a skilled developer in the modern age.