In C++, you can call a base class method from a derived class using the scope resolution operator `::` along with the base class name.
#include <iostream>
class Base {
public:
void show() {
std::cout << "Base class method called." << std::endl;
}
};
class Derived : public Base {
public:
void callBaseShow() {
Base::show(); // Calling base class method
}
};
int main() {
Derived obj;
obj.callBaseShow(); // Output: Base class method called.
return 0;
}
What is a Base Class?
In C++, a base class serves as a foundational class from which other classes (known as derived classes) can inherit properties and methods. This is a key aspect of object-oriented programming (OOP) and promotes code reuse and organization.
Key Characteristics
Base classes contain common functionality that can be shared across multiple derived classes. They help in establishing a hierarchy, allowing derived classes to inherit members (variables and functions) from the base class. Importantly, derived classes can also override base class methods to provide specific implementations while still gaining access to the base functionality.

Understanding Inheritance in C++
What is Inheritance?
Inheritance is a mechanism in C++ that allows a class (derived class) to inherit attributes and behaviors from another class (base class). Inheritance promotes an "is-a" relationship, enabling simpler and more manageable code.
Types of inheritance in C++ include:
- Single Inheritance: A derived class inherits from one base class.
- Multiple Inheritance: A derived class inherits from multiple base classes.
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
How Inheritance Works
When a derived class inherits from a base class, it can access the public and protected members of the base class. This is crucial in ensuring that we can create new classes that build upon existing code. Furthermore, method overriding allows a derived class to provide a specific implementation for a method that it inherits from the base class, enhancing flexibility and functionality.

Calling Base Class Methods in C++
Syntax for Calling Base Class Methods
To call a base class method from a derived class, you utilize the scope resolution operator `::`. This operator helps differentiate base class members from those of the derived class.
Code Snippet
class Base {
public:
void display() {
std::cout << "This is the Base class method." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
// Calling base class method
Base::display();
}
};
Why and When to Call Base Class Methods
Understanding when and why to call base class methods is vital in programming. If you wish to leverage standard behaviors defined in your base classes, calling these methods in derived classes can save time and maintain consistency. Additionally, using base class functionality ensures that the core behaviors remain intact, even if the derived class adds enhancements or specialized functionality.

Practical Examples
Example 1: Basic Method Call
To illustrate how to call a base class method, consider the following example:
Code Snippet
#include <iostream>
using namespace std;
class Animal {
public:
void sound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void bark() {
// Call base class method
Animal::sound();
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Calls both the base and derived class methods
return 0;
}
In this example, the `Dog` class inherits from the `Animal` class. Inside the `bark()` method of `Dog`, we call the `sound()` method from `Animal`. This demonstrates how the derived class can access and utilize functionality defined in its base class.
Example 2: Overriding Base Class Methods
You can override a method inherited from the base class while still retaining access to it. The following example showcases this approach:
Code Snippet
#include <iostream>
using namespace std;
class Vehicle {
public:
virtual void start() {
cout << "Vehicle is starting" << endl;
}
};
class Car : public Vehicle {
public:
void start() override {
// Call base class method
Vehicle::start();
cout << "Car is starting with a roar!" << endl;
}
};
int main() {
Car myCar;
myCar.start(); // Calls overridden method and base class method
return 0;
}
In this case, the `Car` class overrides the `start()` method from the `Vehicle` class. Inside the overridden method, we still invoke `Vehicle::start()`, which allows us to maintain the base method's behavior while adding new functionality.
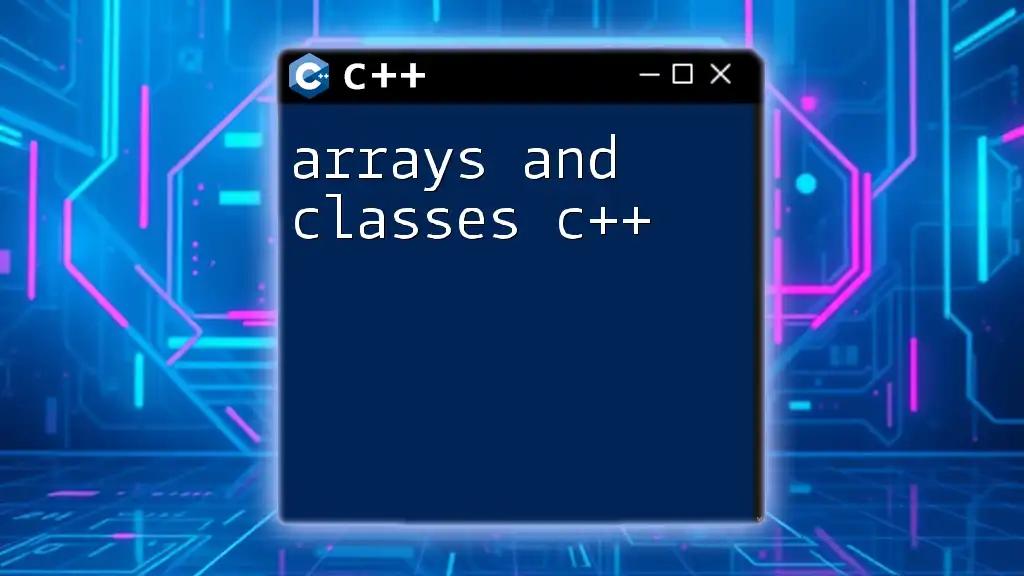
Common Mistakes to Avoid
Forgetting to Use the Scope Resolution Operator
One common mistake new C++ programmers make is forgetting to use the scope resolution operator when calling a base class method. This can lead to ambiguous method calls or runtime errors, especially in cases of method overriding.
Overriding Without Calling Base Methods
When overriding methods in derived classes, failing to call the base class method can result in critical functionality being omitted, which may lead to unexpected behavior in your application. It’s essential to understand the logic behind the base class’s implementation and to ensure that you invoke it when needed.

Conclusion
In summary, understanding how to call base class methods in C++ is fundamental for mastering the principles of inheritance and polymorphism. By using the scope resolution operator wisely, you can access base class functionality and enhance your derived classes’ capabilities. Practicing these concepts will vastly improve your C++ programming skills and lead to cleaner, more efficient code. Make sure to explore further examples and create your own implementations to solidify your understanding of this topic.