In C++, you can round a floating-point number to two decimal places using the `std::setprecision` function from the `<iomanip>` library alongside `std::fixed` for formatting.
#include <iostream>
#include <iomanip>
int main() {
double num = 3.14159;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 3.14
return 0;
}
Understanding Rounding
Rounding in numerical computations refers to the process of adjusting a number to a specified degree of accuracy. This is vital in programming as it allows developers to ensure that the results of calculations meet a format that is often required, particularly in financial applications where monetary values must adhere to a specific number of decimal places.
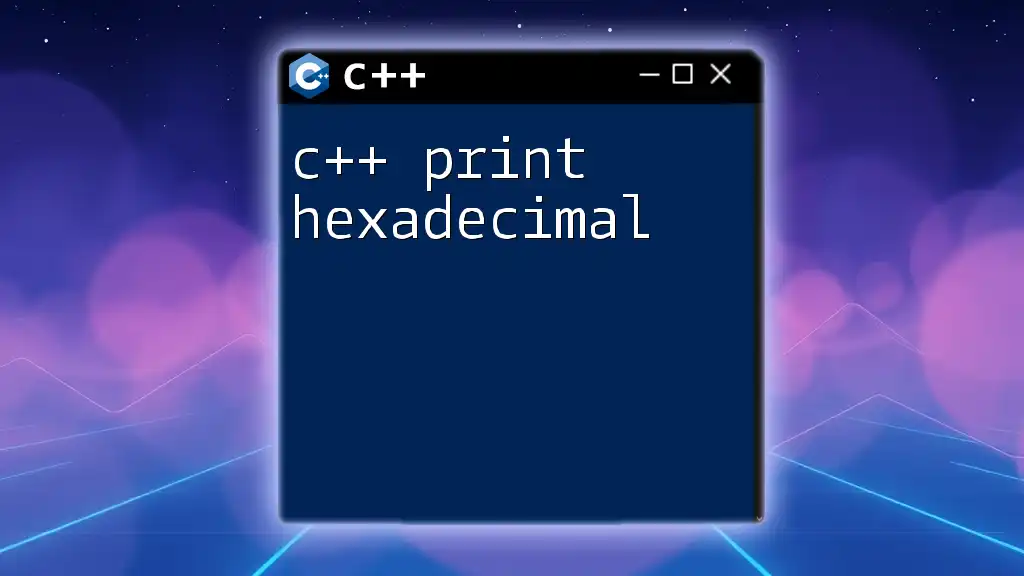
Rounding in C++
C++ offers several built-in functions for rounding numbers. Understanding these functions is key when you need to perform operations that require precision, such as displaying currency or measurement values.
Standard Rounding Techniques
In C++, the following functions are commonly used for rounding:
- `std::round`: Rounds to the nearest integer. If the number is halfway between two integers, it rounds away from zero.
- `std::floor`: Rounds down to the nearest integer.
- `std::ceil`: Rounds up to the nearest integer.
Understanding when to use each of these functions will help in achieving accurate results depending on your rounding needs.
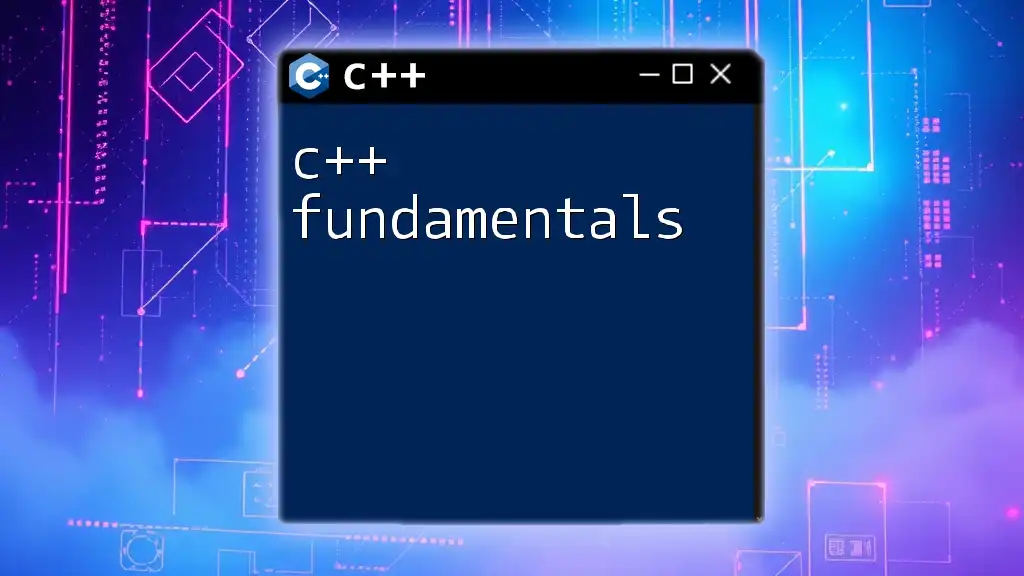
Rounding to 2 Decimal Places
Why Rounding to 2 Decimal Places is Useful
Rounding to two decimal places is particularly important in financial applications, where cents matter. Improper rounding could lead to significant financial discrepancies over time, making it essential to develop reliable techniques for rounding numbers.
Using std::round with Multiplication and Division
One straightforward approach to c++ round to 2 decimals is leveraging the `std::round` function combined with multiplication and division. By multiplying the value by 100, rounding to the nearest integer, and then dividing back by 100, we achieve the desired precision.
Here’s how to implement it:
#include <cmath>
double roundToTwoDecimals(double value) {
return std::round(value * 100.0) / 100.0;
}
Example Explanation
In the function `roundToTwoDecimals`, multiplying the value by 100 shifts the decimal point two places to the right. The `std::round` function then rounds this value to the nearest integer. Finally, dividing by 100 shifts the decimal point back to its original position, resulting in a number rounded to two decimal places.
Using the std::setprecision Function
Another effective method for rounding to two decimal places is utilizing the `std::setprecision` function from the `<iomanip>` library. This allows formatted output while ensuring the displayed precision.
Here's a simple example:
#include <iostream>
#include <iomanip>
void printRounded(double value) {
std::cout << std::fixed << std::setprecision(2) << value << std::endl;
}
Example Output
Using the `printRounded` function, any double value passed will be displayed with exactly two decimal places.
For instance:
printRounded(10.12345); // Output: 10.12
printRounded(10.12678); // Output: 10.13
Custom Rounding Functions
In some cases, the standard rounding functions may not suffice, especially when you need to round to different decimal places dynamically. You can create a custom rounding function that takes the number of decimal places as a parameter.
Here is an example:
#include <cmath>
double customRound(double value, int decimals) {
double p = std::pow(10.0, decimals);
return std::round(value * p) / p;
}
Example Usage
You can use `customRound` to handle various decimal precision requirements easily:
double roundedValue = customRound(2.34567, 2); // Output: 2.35
double roundedValue2 = customRound(2.34123, 3); // Output: 2.341
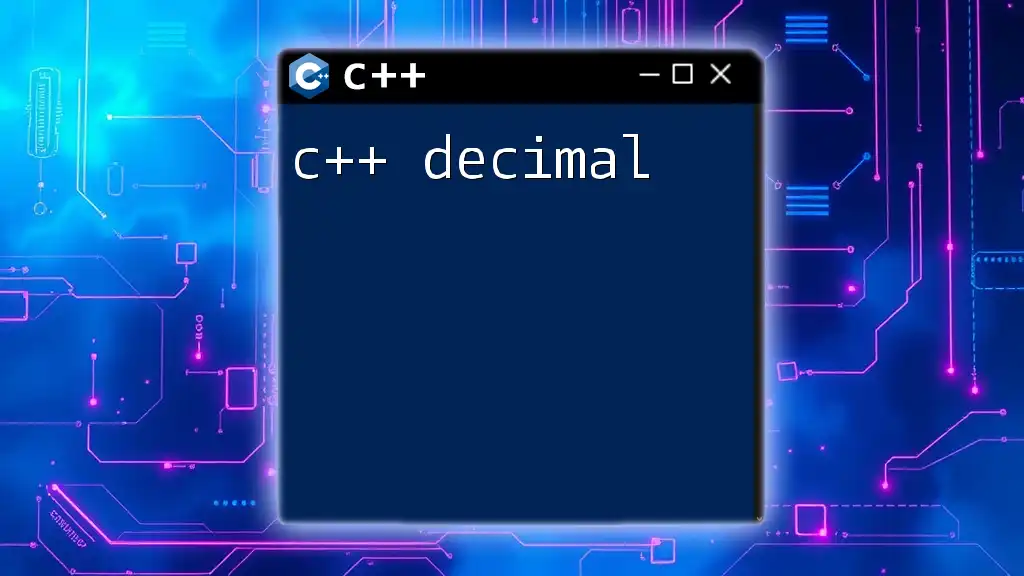
Handling Special Cases
When rounding numbers, it's crucial to consider special cases. For instance, rounding negative numbers requires careful handling to ensure that values are rounded according to the intended rules.
Additionally, floating-point numbers can cause rounding errors due to the limitations of binary representation. Both very small and very large numerals can lead to inaccuracies, so understanding how C++ handles these scenarios is important for robust programming.
Rounding Errors
Floating-point precision issues may arise during computations. For instance, operations involving very large or small numbers could lead to unexpected results due to how these numbers are represented in memory. To minimize rounding errors, it’s vital to use appropriate data types and rounding techniques tailored to your specific needs.
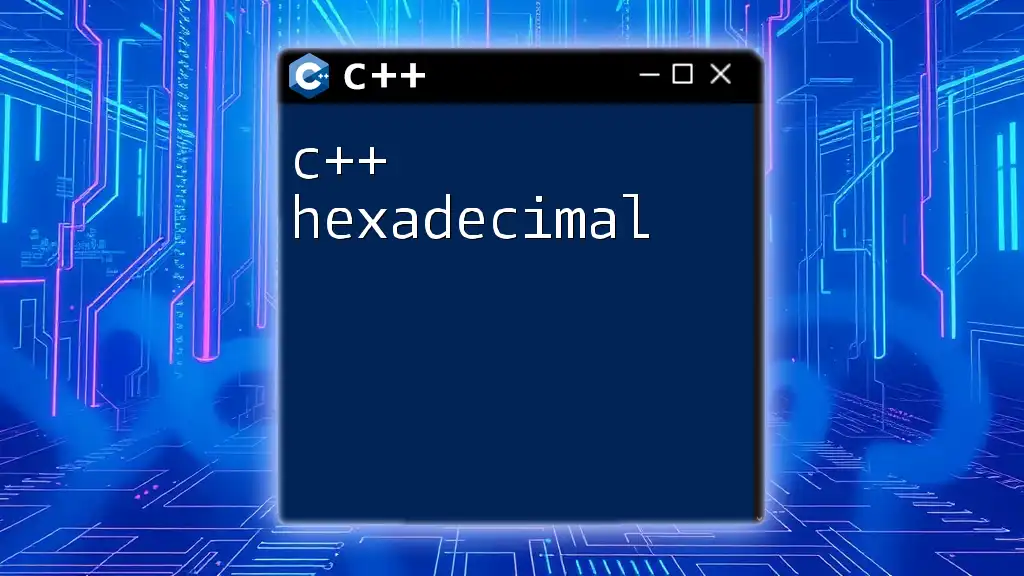
Conclusion
In summary, when working with C++ and in particular looking to c++ round to 2 decimals, several methods are at your disposal, including the `std::round` method, `std::setprecision` for output formatting, and custom rounding functions for flexibility. Each approach has its strengths, so understanding the context in which you operate will dictate the best choice. Rounding is more than just a simple function call; it's a critical component of ensuring the accuracy and reliability of numeric computations in your applications.
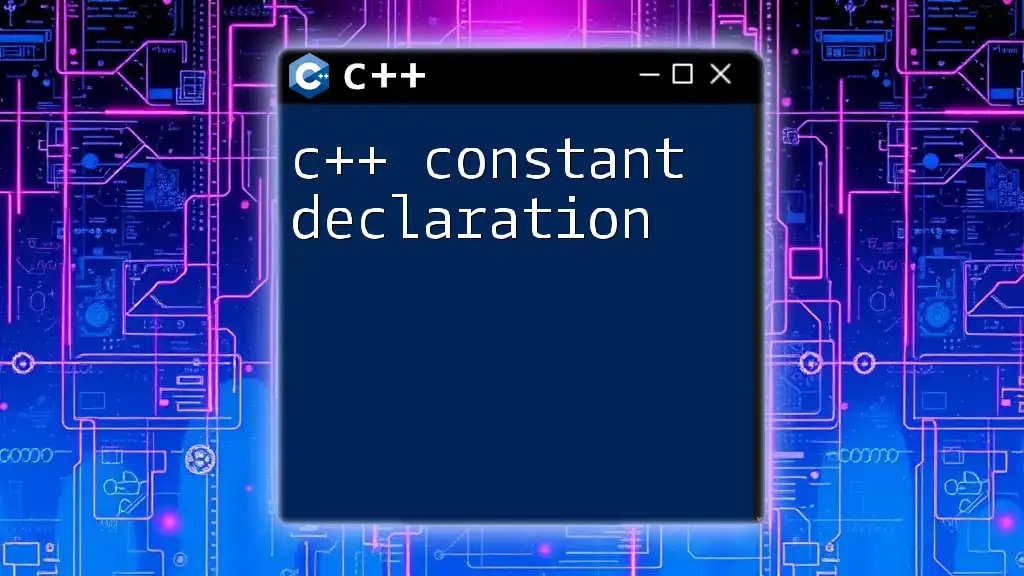
Additional Resources
For further exploration, check C++ documentation regarding rounding functions and precision methods. Engaging with comprehensive books or online resources can provide deeper insights into numerical operations in C++.
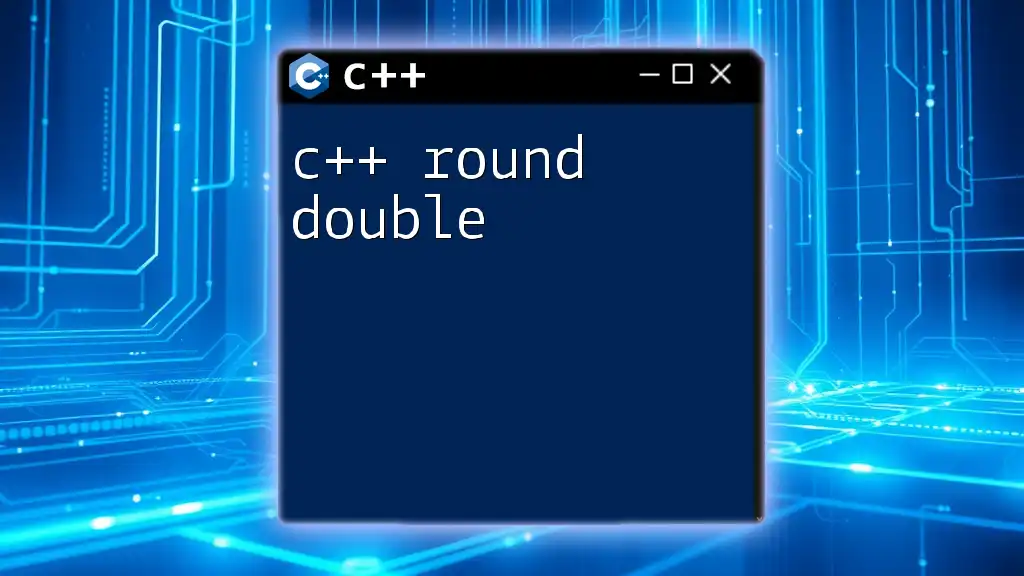
FAQs
What is the difference between `std::round` and `std::floor`?
`std::round` rounds to the nearest integer, while `std::floor` always rounds down, regardless of the decimal value.
How can I ensure correct rounding in financial applications?
Always utilize a specific rounding function tailored to monetary values, and consider using fixed-point arithmetic instead of floating-point, if possible.
What happens to rounding when dealing with negative numbers?
Negative numbers behave similarly in terms of how rounding works, but the outcomes will result in moving towards zero when using `std::round`. Hence, understanding the context is essential for accurate results.