A C++ text editor is a software application that allows developers to write, edit, and manage C++ code efficiently, often providing features like syntax highlighting and debugging tools.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Text Editors
What is a Text Editor?
A text editor is a software application that allows users to create and modify plain text files. It serves as a fundamental tool for programming, enabling programmers to write code efficiently. Unlike Integrated Development Environments (IDEs), which provide a more feature-rich suite of tools for software development—including debugging and project management—text editors often focus more on code writing.
Types of Text Editors
Basic Text Editors
Basic text editors, such as Notepad on Windows and Nano on Unix-based systems, are lightweight applications designed for simple text editing tasks. They are quick to load and can be used for quick changes without many distractions. However, they lack advanced features that many C++ developers might find beneficial, such as syntax highlighting or code autocompletion.
Advanced Text Editors
Advanced text editors, such as Visual Studio Code, Sublime Text, and Atom, are designed with programmers in mind. These editors offer a robust set of features, including:
- Plugins and Extensions: Allowing users to add functionality according to their needs.
- Syntax Highlighting: Helps make source code easier to read.
- Integrated Terminal: Facilitates testing and running code from within the editor.
These advanced editors can greatly enhance the coding experience, particularly for C++ developers.

Popular C++ Text Editors
Visual Studio Code
Visual Studio Code (VS Code) is one of the most popular choices among C++ developers due to its flexibility and rich feature set.
Why VS Code is Great for C++
VS Code combines lightweight performance with powerful features. Its extension marketplace allows you to enhance its functionality significantly. For C++ programming, you can install extensions that provide:
- Language Support: Implement C++ syntax highlighting and IntelliSense for autocompletion.
- Debugging Tools: A built-in debugger that allows you to set breakpoints and inspect variables.
Setting Up C++ in VS Code
To configure VS Code for C++, follow these steps:
- Install VS Code: Download and install from the official website.
- Install the C++ Extension: Access the extension marketplace by clicking on the Extensions icon and search for "C++". Install the necessary extensions that include C/C++ from Microsoft.
- Create a Configuration File: Next, set up your `tasks.json` and `launch.json` files for building and debugging your C++ projects.
Example of a `tasks.json` configuration for building a C++ program:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-g",
"${file}",
"-o",
"${fileDirname}/${fileBasenameNoExtension}.out"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": ["$gcc"]
}
]
}
Sublime Text
Sublime Text is renowned for its speed and responsiveness, making it a favorite among many developers.
Features and Benefits
Sublime Text offers a minimalist interface with extensive customization options. Some key advantages for C++ development include:
- Multiple Cursors: Edit multiple lines of code simultaneously, speeding up your workflow.
- Package Control: Easily install and manage plugins tailored for C++.
Customizing Sublime Text for C++ Development
To customize Sublime Text for C++, you can install the C++ Package, which adds numerous features designed specifically for C++.
Example of a simple C++ snippet for a Hello World program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Atom
Atom is an open-source text editor developed by GitHub, designed to be easily customizable.
Unique Features of Atom
One of Atom's standout features is its inherent hackability. Users can modify its core to fit their needs, and it includes a built-in package manager for adding functionality. Atom also supports collaboration with live sharing capabilities.
Getting Started with C++ in Atom
To set up C++ in Atom, follow these simple steps:
- Download Atom: Install Atom from the official website.
- Install Packages: Add useful packages like gpp-compiler for compiling C++ code and script for running code directly from the editor.
Sample code layout in Atom for a simple function:
#include <iostream>
void greet() {
std::cout << "Greetings from Atom!" << std::endl;
}
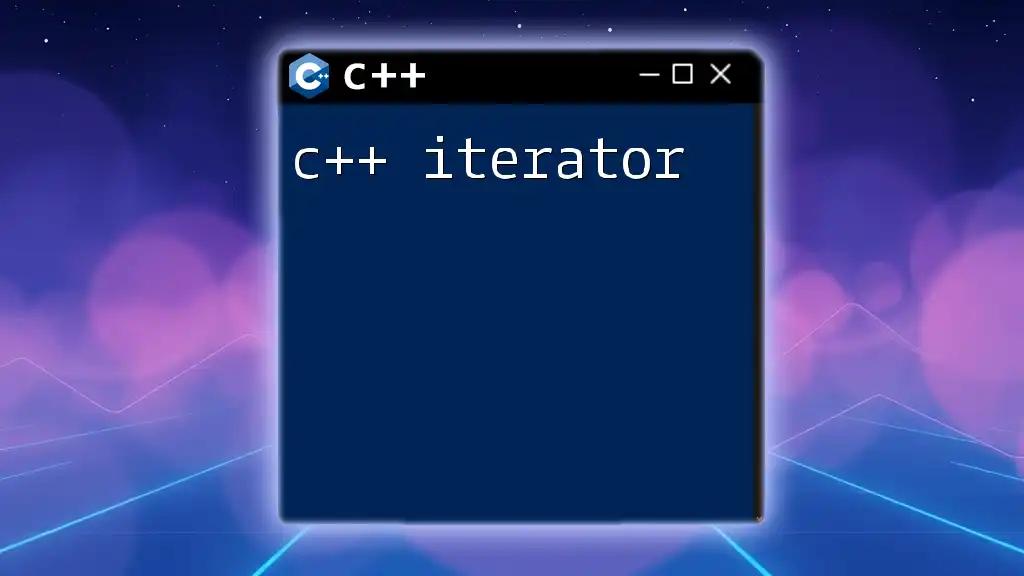
Essential Features in C++ Text Editors
Syntax Highlighting
Syntax highlighting is a critical feature in text editors that colors different parts of the code. This enhancement improves readability and makes it easier to spot errors, particularly in complex C++ codebases.
Code Autocompletion
Code autocompletion is another vital feature. It predicts the next inputs based on the context, allowing for quicker coding and reducing the chance of typos or syntax errors. For instance, when you begin typing a function name, the editor will suggest completed options.
Debugging Tools
Many advanced text editors, like VS Code, offer built-in debugging tools. These tools can set breakpoints, inspect variable values, and step through code line by line, making it significantly easier to identify and fix errors.
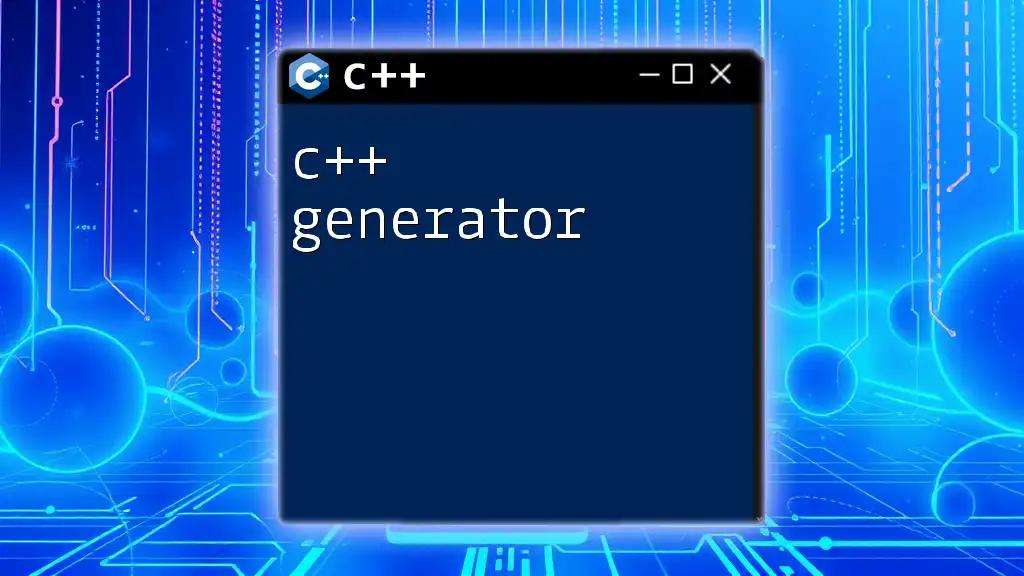
Configuration and Customization
Extensions and Plugins
Extensions are key to unlocking the full potential of your text editor. They provide additional functionality specifically tailored for C++. For example, with the C/C++ extension in VS Code, you gain debugging capabilities, code navigation, and auto-complete features that enhance your productivity while coding.
Theming and User Interface
Personalizing your text editor is essential for creating a comfortable coding environment. Many editors come with both light and dark themes. Choosing a theme that suits your preference can help reduce eye strain, especially during long coding sessions.

Best Practices for Using Text Editors for C++
Organizing Your Workspace
Effective workspace organization is crucial. Establishing a clear folder structure and managing your files systematically helps you navigate projects easily. Additionally, integrating version control, such as Git, helps keep your work organized and allows for easy collaboration with others.
Efficient Coding Strategies
Utilizing shortcuts and keybindings can dramatically improve your coding speed and efficiency. Familiarize yourself with common commands for compiling code, navigating through files, and utilizing search functions within your editor, as these can save a substantial amount of time versus using the mouse.
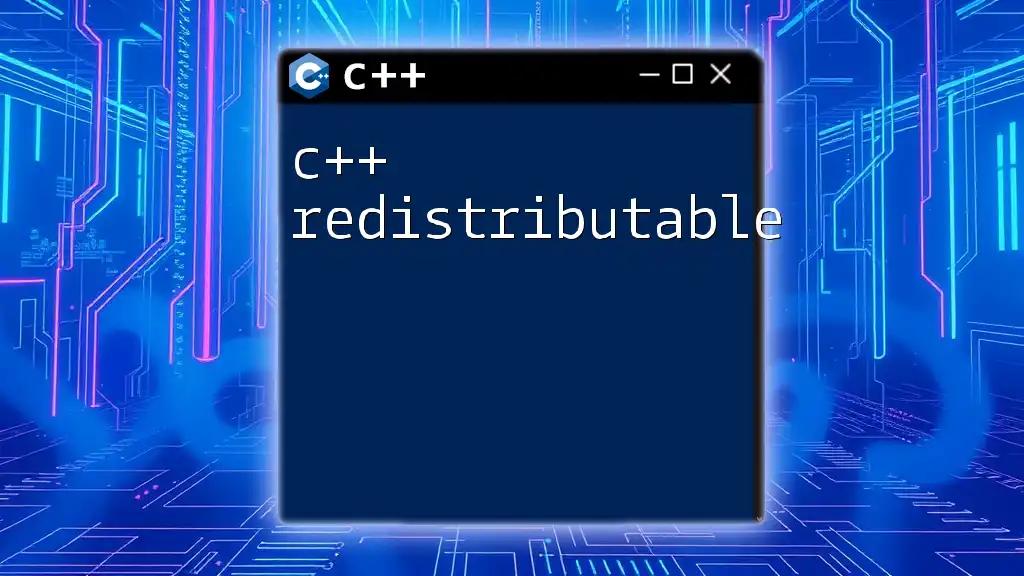
Conclusion
Choosing the right C++ text editor can significantly impact your programming workflow. From Visual Studio Code's extensive extensions to Sublime Text's speed and Atom's flexibility, each editor has unique advantages. Experimenting with these tools and customizing them to best suit your coding style will help you enhance your efficiency and productivity in C++ development. Explore the features that resonate most with you, and enjoy the journey of becoming more adept at programming in C++.