In C++, `using` and `typedef` are both used to create type aliases, but `using` is preferred in modern code for its clarity and ability to alias template types.
Here’s a code snippet demonstrating both:
#include <iostream>
#include <vector>
// Using typedef to create type aliases
typedef std::vector<int> IntVector1;
// Using using to create type aliases
using IntVector2 = std::vector<int>;
int main() {
IntVector1 vec1 = {1, 2, 3};
IntVector2 vec2 = {4, 5, 6};
// Displaying the contents of the vectors
for (int i : vec1) std::cout << i << " ";
std::cout << std::endl;
for (int i : vec2) std::cout << i << " ";
return 0;
}
Understanding Type Aliases in C++
What are Type Aliases?
In C++, a type alias is a newer name for an existing type. Type aliases aid in making code more readable and maintainable by giving a new, often more meaningful, name to complex data types. This is particularly useful when dealing with intricate template types that might be harder to read and understand if left with their original names.
The Evolution of Type Aliasing in C++
The need for clear and readable type definitions led to the introduction of `typedef` in earlier versions of C++. However, the advent of C++11 brought about a more intuitive keyword: `using`. This evolution reflects C++’s move towards a more modern and developer-friendly approach to programming.
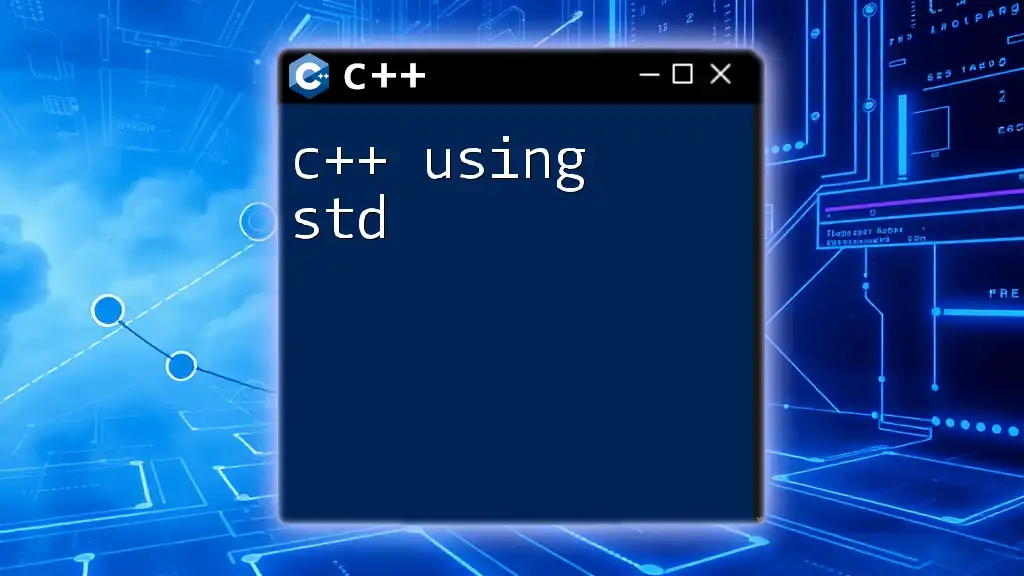
The `typedef` Keyword
Syntax and Basic Usage
The `typedef` keyword allows programmers to create aliases for existing types, which can simplify complex declarations. Here's the basic syntax for `typedef`:
typedef original_type new_name;
For example, to define an alias for `unsigned long`, one might write:
typedef unsigned long ulong;
// Usage
ulong counter = 1000;
In this example, `ulong` can now be used in place of `unsigned long`, providing an easier way to reference this type in code.
Advantages and Disadvantages
Advantages of Using `typedef`
- Simplicity: It can help simplify code by allowing a shorter reference to complex types.
- Readability: By using descriptive aliases, the intent of the code can be made clearer, which is crucial for team collaboration and long-term maintenance.
Disadvantages of Using `typedef`
- Complexity with Templates: `typedef` cannot be used effectively with templates, making it less versatile than `using`.
- Potential Confusion: When working with complex types, programmers might find it confusing if the alias does not convey enough context.
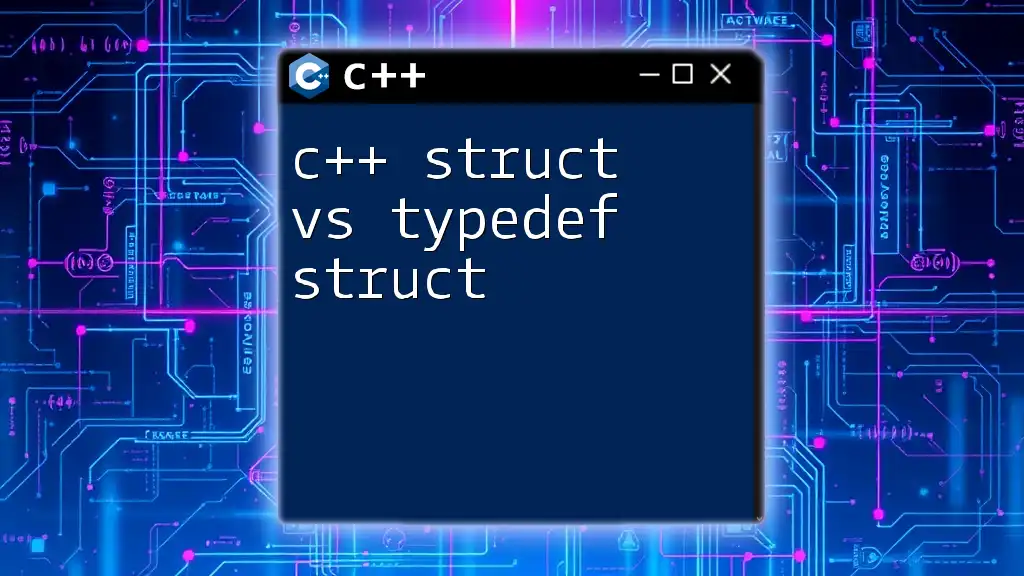
The `using` Keyword
Syntax and Basic Usage
The `using` keyword serves a similar purpose to `typedef` but comes with improved readability and usability, especially when dealing with templates. The syntax for `using` is:
using new_name = original_type;
For instance, creating a type alias for `unsigned long` would look like this:
using ulong = unsigned long;
// Usage
ulong counter = 1000;
This assignment is functionally identical to the `typedef` example discussed earlier but is often considered clearer and more readable.
Comparing `using` and `typedef`
While both `using` and `typedef` serve to create type aliases, they are not interchangeable. Here are some concise comparisons:
- Readability: `using` reads more clearly when defining templates. For example, with `using`, you can create an alias for a vector of integers as follows:
using intVec = std::vector<int>;
This format allows for easier reading and comprehension.
- Support for Templates: `using` can define type aliases with templates, which `typedef` cannot accomplish. This creates a significant advantage for generic programming methodologies.
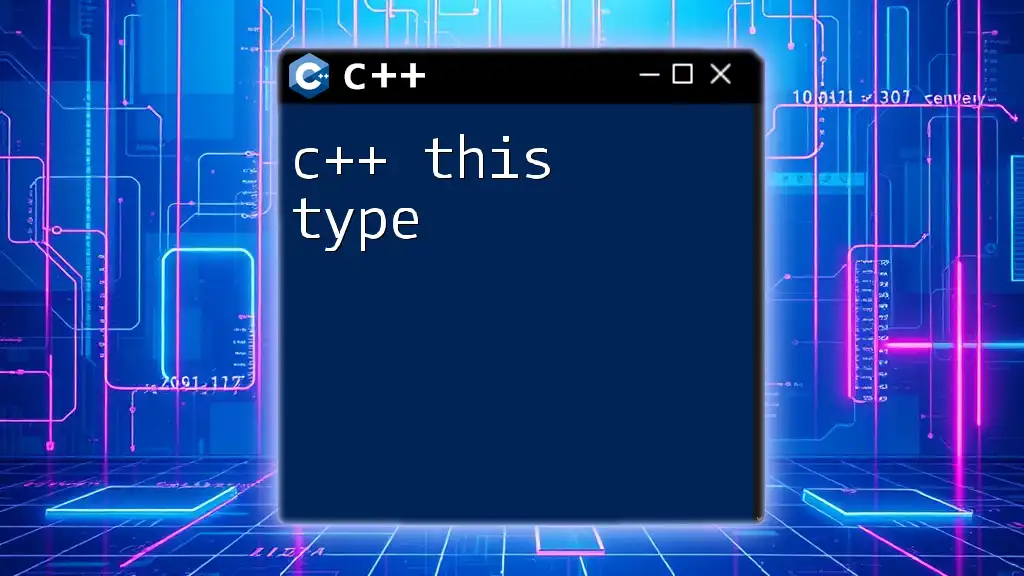
Advanced Usage of Type Aliases
Using Type Aliases with Templates
`using` with Templates
C++ allows the creation of aliases for templates using the `using` keyword. Consider this example of defining a type alias for a vector of any type:
template <typename T>
using vec = std::vector<T>;
// Usage
vec<int> intVec; // Creates a vector of integers
vec<std::string> strVec; // Creates a vector of strings
This flexibility in type aliasing with `using` helps streamline code that involves templates.
`typedef` Limitations with Templates
In contrast, the `typedef` keyword struggles with templates. Therefore, when programmers need to use type aliasing in templates, `using` is the preferred choice due to its flexibility.
Nested and Multi-Layered Type Aliases
Type aliases can also serve as building blocks for more complex types. Both `using` and `typedef` can be used to create these multi-layered definitions. For example:
using byte = unsigned char;
using buffer = std::array<byte, 256>;
Or using `typedef`:
typedef unsigned char byte;
typedef std::array<byte, 256> buffer;
In both cases, a new alias `buffer` is created that refers to an array of 256 bytes.
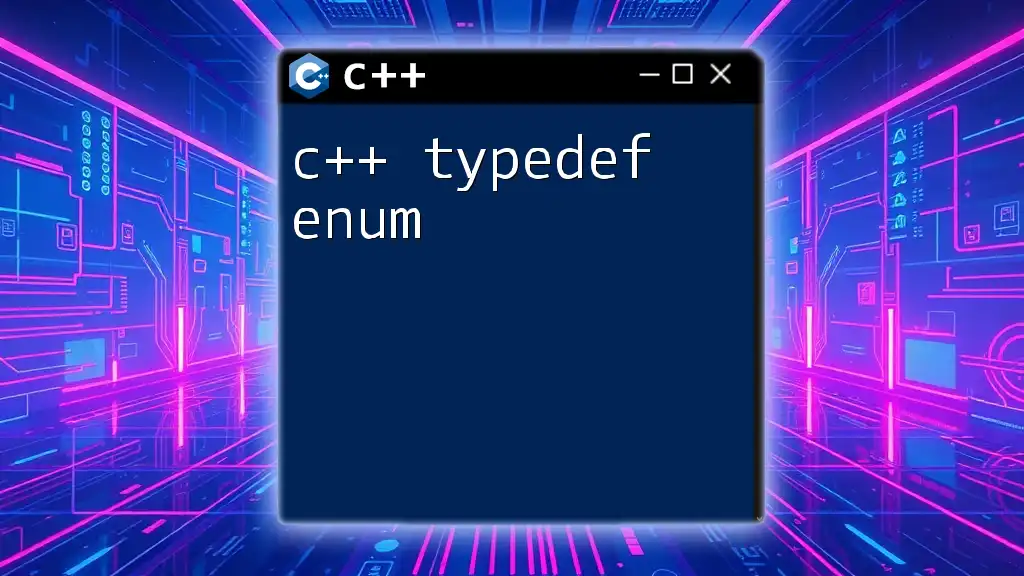
Practical Applications of Using and Typedef
Real-World Examples
In actual coding projects, you may encounter scenarios where type aliases greatly enhance code clarity. For instance, in large applications that deal with complex types, defining meaningful aliases can make the code significantly more manageable.
Here’s how you might declare a complex type:
typedef std::map<int, std::vector<std::string>> MapType;
// Usage
MapType idxToNames;
In this example, using `typedef` to create `MapType` simplifies interactions with maps of vectors of strings.
Best Practices for Using Type Aliases in C++
When deciding between `using` and `typedef`, consider the following guidelines:
- Prefer `using` for defining complex or template types due to its better readability.
- Use meaningful names for your type aliases to improve code maintainability.
- Consistency is key. Adhere to a style guide throughout your project for type aliasing to avoid confusion.
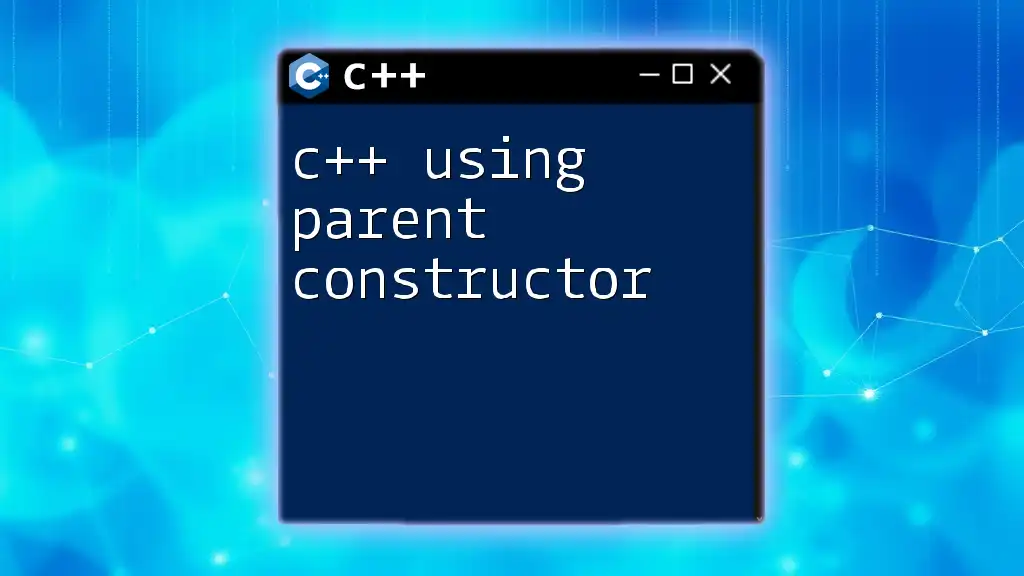
Conclusion
Understanding how to use `using` and `typedef` effectively is vital for every C++ programmer. With `using`, you gain readability and enhanced capabilities, especially when working with templates. On the other hand, `typedef` may still hold its ground for simpler use cases. Experimenting with both approaches will enable you to write clearer and more maintainable C++ code.
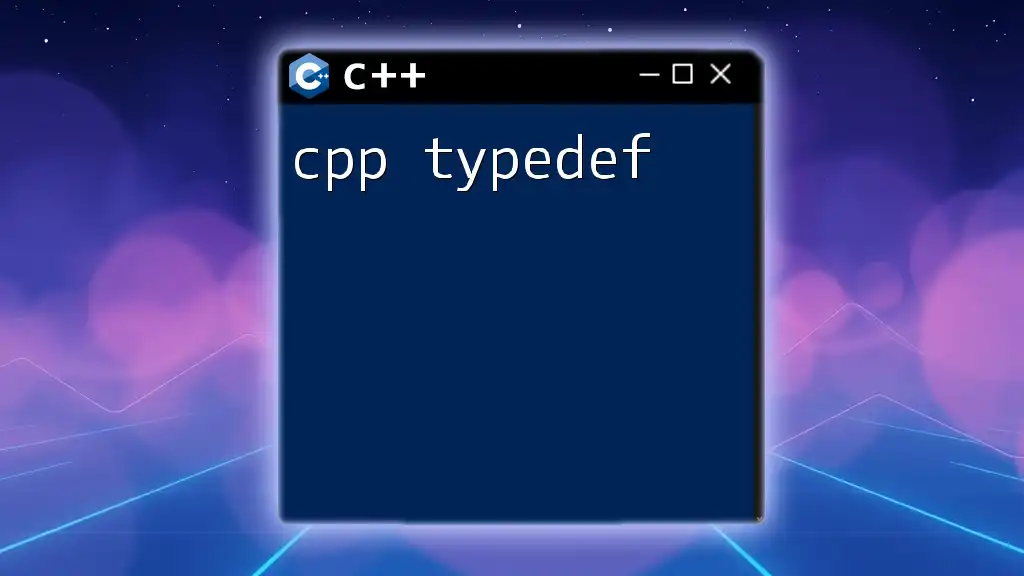
Additional Resources
For those looking to dive deeper, consider exploring C++ documentation, tutorials, and recommended books that focus on C++ best practices. Exploring these resources can provide further insights into improving your coding expertise.