The Vigenère cipher is a method of encrypting alphabetic text by using a simple form of polyalphabetic substitution based on a keyword. Here’s a code snippet that demonstrates a basic implementation of the Vigenère cipher in C++:
#include <iostream>
#include <string>
std::string vigenereCipher(const std::string& text, const std::string& key) {
std::string cipher;
for (size_t i = 0, j = 0; i < text.size(); ++i) {
if (isalpha(text[i])) {
char offset = isupper(text[i]) ? 'A' : 'a';
cipher += char((text[i] + key[j % key.size()] - 2 * offset) % 26 + offset);
j++;
} else {
cipher += text[i];
}
}
return cipher;
}
int main() {
std::string text = "HELLO WORLD";
std::string key = "KEY";
std::cout << "Ciphertext: " << vigenereCipher(text, key) << std::endl;
return 0;
}
Understanding the Vigenère Cipher
What is the Vigenère Cipher?
The Vigenère Cipher is a method of encrypting alphabetic text by using a simple form of polyalphabetic substitution. Developed in the 16th century, this cipher was named after Blaise de Vigenère, who created a more complex version of the cipher. It is crucial in the history of cryptography because it introduces the concept of using multiple shifted alphabets based on a keyword, making the cipher more resilient to frequency analysis compared to its predecessors like the Caesar cipher.
How the Vigenère Cipher Works
At its core, the Vigenère Cipher operates based on three components: plaintext, ciphertext, and key. When encrypting, each letter of the plaintext is shifted along the alphabet using a corresponding letter from the key. The alignment of the key with the plaintext is cyclic, meaning that once the end of the key is reached, it loops back to the beginning. The result is a ciphertext that appears more random and more secure against attacks.
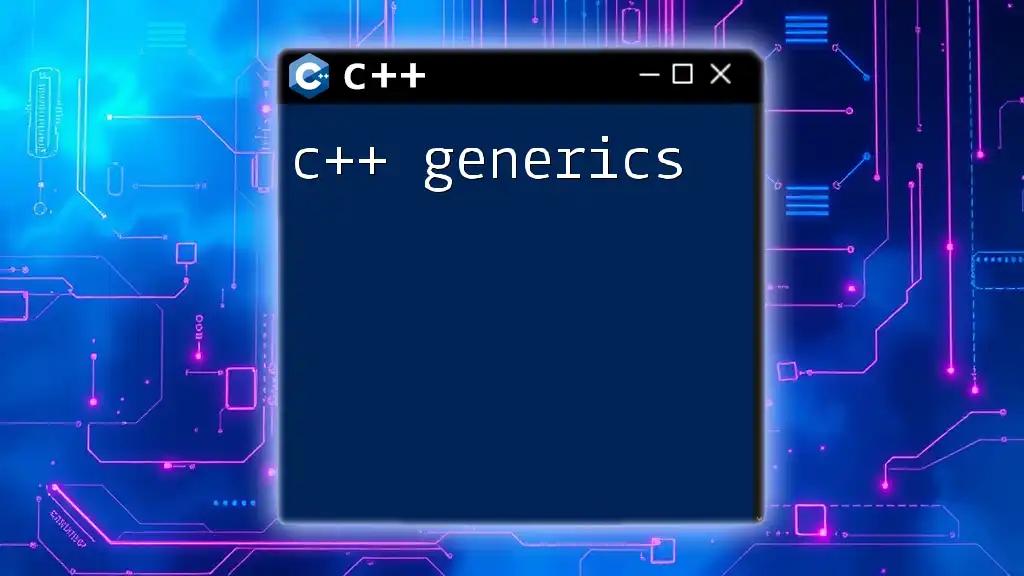
Setting Up Your C++ Environment
Required Software and Tools
To implement the C++ Vigenère Cipher, you'll need a C++ compiler. Popular choices include:
- GCC (GNU Compiler Collection)
- Microsoft Visual Studio
Make sure to have the latest version installed for compatibility with modern C++ features.
Code Structure Overview
Organizing your project files is essential to maintain clarity in your code. A recommended structure is:
- main.cpp: Contains the main function and code execution.
- cipher.h: Header file declaring the Vigenère Cipher class.
- cipher.cpp: Implementation file defining the functions declared in cipher.h.
Using this structure promotes modularity, making debugging and enhancements easier.
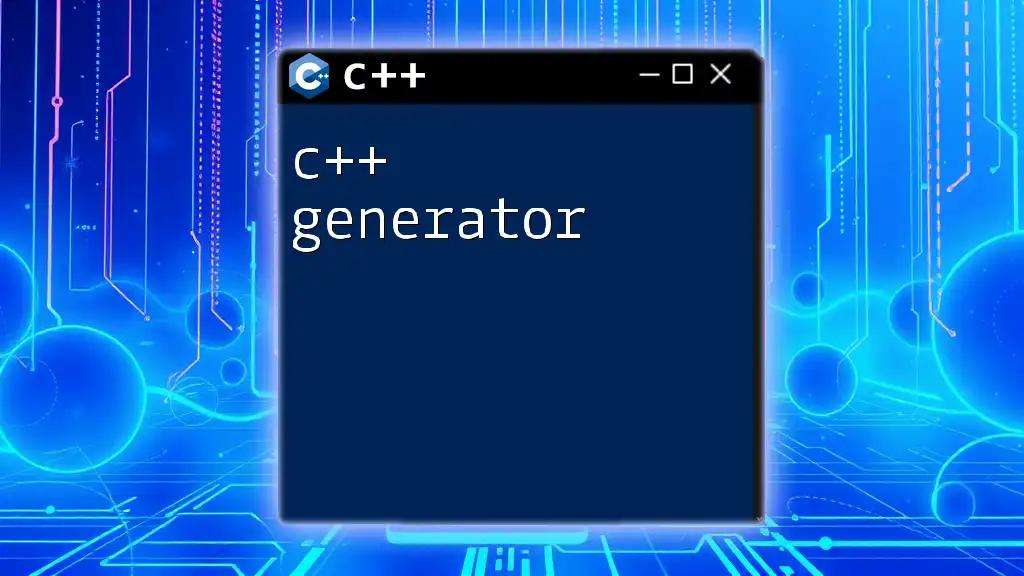
Implementing the Vigenère Cipher in C++
Writing the Cipher Class
Class Definition
The first step in creating the C++ Vigenère Cipher is to define a class. This class will encapsulate the functionalities of both encryption and decryption.
Code Snippet: Basic Class Structure
class VigenereCipher {
public:
void setKey(const std::string& inputKey);
std::string encrypt(const std::string& plaintext);
std::string decrypt(const std::string& ciphertext);
private:
std::string key;
};
Key Management
The choice and management of the encryption key is critical. The key should be adequately sized and complex enough to ensure security. A simple function can be implemented to check the validity of the key, ensuring it consists of letters only and is reasonably long.
Code Snippet: Handling Keys
void VigenereCipher::setKey(const std::string& inputKey) {
// Convert inputKey to uppercase and validate
key = ...; // implementation details
}
Encryption Process
Encrypting the Plaintext
The core of the cipher is its encryption mechanism. For every letter in the plaintext, you will determine its equivalent in the key and apply a shift based on their respective positions in the alphabet.
For example, if your plaintext is "HELLO" and your key is "KEY", the encryption will involve calculating shifts:
- H (7) + K (10) = R (17)
- E (4) + E (4) = I (8)
Continue this way for the entire plaintext.
Code Implementation of Encryption
Code Snippet: Encrypt Function
std::string VigenereCipher::encrypt(const std::string& plaintext) {
std::string ciphertext;
size_t keyIndex = 0;
for(char letter : plaintext) {
if (isalpha(letter)) {
// Handle letter shifting logic here
keyIndex = (keyIndex + 1) % key.length();
}
}
return ciphertext;
}
Decryption Process
Decrypting the Ciphertext
The decryption process is the inverse of encryption. Each letter of the ciphertext needs to shift back according to the key letters to recover the original plaintext.
For instance, if the ciphertext is "RIP", using the key "KEY":
- R (17) - K (10) = H (7)
- I (8) - E (4) = E (4) Continue this for the entire ciphertext.
Code Implementation of Decryption
Code Snippet: Decrypt Function
std::string VigenereCipher::decrypt(const std::string& ciphertext) {
std::string plaintext;
size_t keyIndex = 0;
for (char letter : ciphertext) {
if (isalpha(letter)) {
// Shift back logic
keyIndex = (keyIndex + 1) % key.length();
}
}
return plaintext;
}
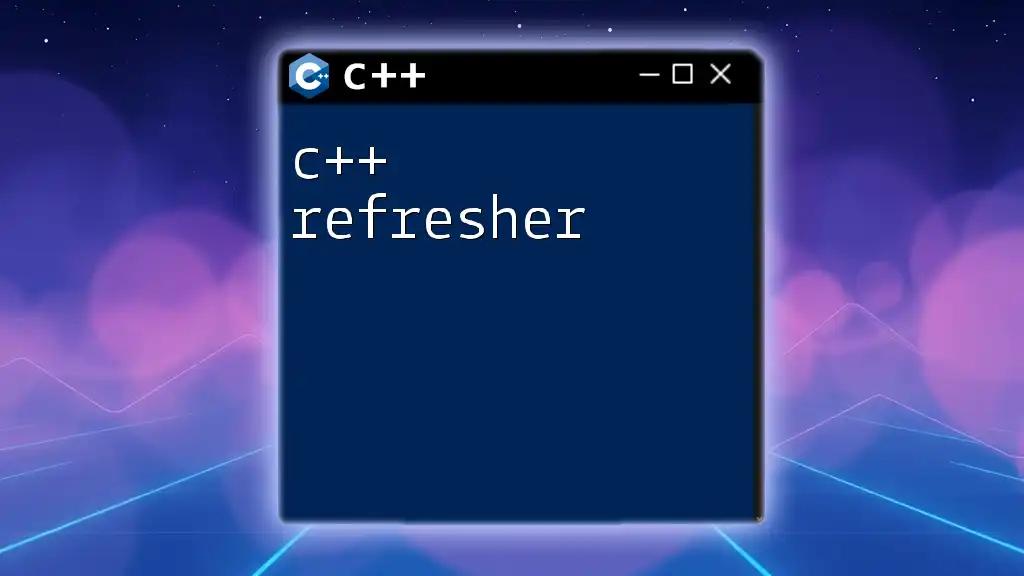
Optimizing the Cipher Implementation
Error Handling
It's essential to incorporate error handling in your implementation. This includes checking for null or empty keys and ensuring characters are alphabetic. Robust error management prevents runtime failures and enhances user experience.
Code Snippet: Error Handling Mechanisms
if (key.empty()) {
throw std::invalid_argument("Key cannot be empty!");
}
Enhancements
Consider potential enhancements such as implementing alternative ciphers or modifying the internal structure for better performance. Techniques like using dynamic key lengths or input sanitization can add layers of security.
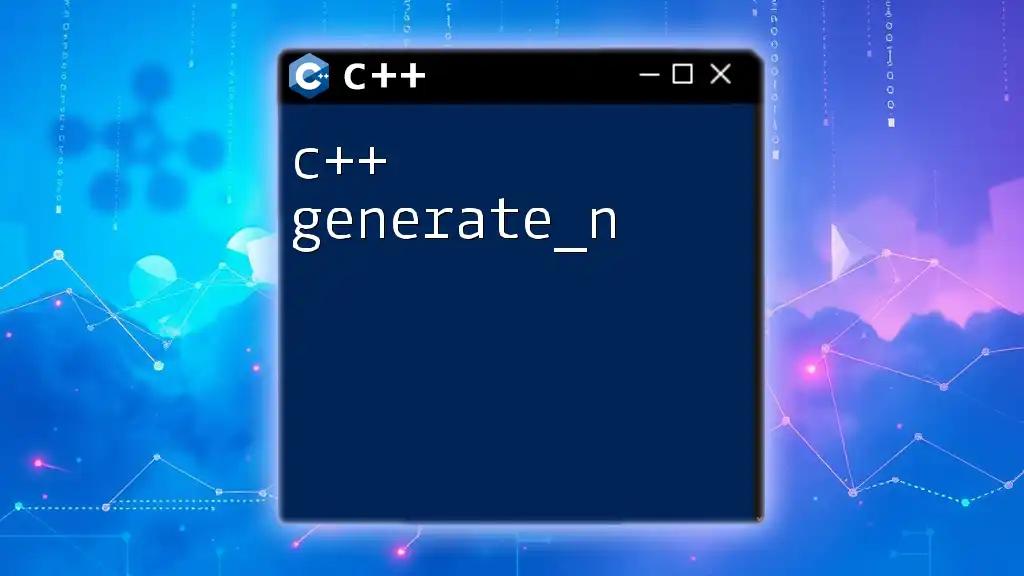
Example Usage of the Vigenère Cipher Class
Main Function Implementation
Finally, integrating the classes and functions allows us to demonstrate the functionality of the C++ Vigenère Cipher.
Complete Code Example
int main() {
VigenereCipher vc;
vc.setKey("KEY");
std::string encrypted = vc.encrypt("HELLO");
std::string decrypted = vc.decrypt(encrypted);
std::cout << "Encrypted: " << encrypted << std::endl;
std::cout << "Decrypted: " << decrypted << std::endl;
}
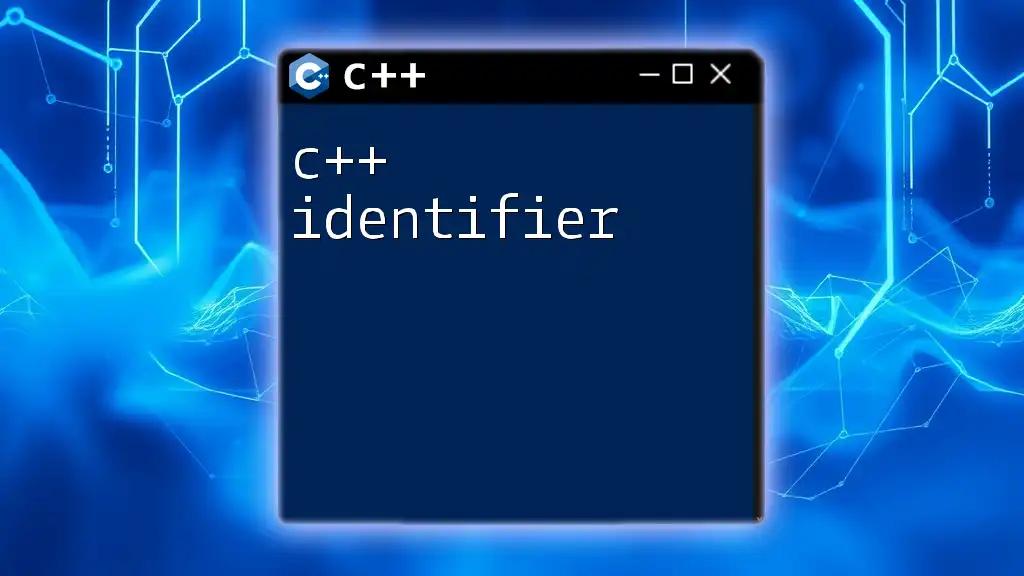
Conclusion
Summary of Key Concepts
In summary, the C++ Vigenère Cipher provides an excellent introduction to classical cryptography. By understanding and implementing the encryption and decryption processes, you have a foundation to explore more advanced cryptographic techniques.
Next Steps for Learning
To further your skills, consider delving into topics such as modern cryptography, asymmetric keys, or even studying different ciphers. Experimenting with different approaches to the Vigenère Cipher can deepen your understanding and sharpen your programming abilities.