In C++, you can convert an ASCII value to its corresponding character using type casting or the `static_cast` operator.
Here's a code snippet demonstrating this:
#include <iostream>
int main() {
int asciiValue = 65; // ASCII value for 'A'
char character = static_cast<char>(asciiValue);
std::cout << "The character for ASCII " << asciiValue << " is " << character << std::endl;
return 0;
}
Understanding ASCII
What is ASCII?
ASCII, or American Standard Code for Information Interchange, is a character encoding standard that uses numbers to represent text in computers and other devices that work with text. Developed in the 1960s, ASCII allows for 128 unique symbols, which include letters, digits, punctuation marks, and control characters. This standardization makes it possible for various devices and applications to communicate text data effectively.
ASCII Table Overview
The ASCII table outlines the complete set of values defined by ASCII. Each character corresponds to a unique numeric value ranging from 0 to 127. For example:
- The character 'A' has an ASCII value of 65.
- The character 'a' has an ASCII value of 97.
- The digit '0' has an ASCII value of 48.
Understanding the ASCII values helps in situations where developers need to manipulate characters programmatically in C++. The ability to convert between ASCII values and characters enables efficient data handling and processing.
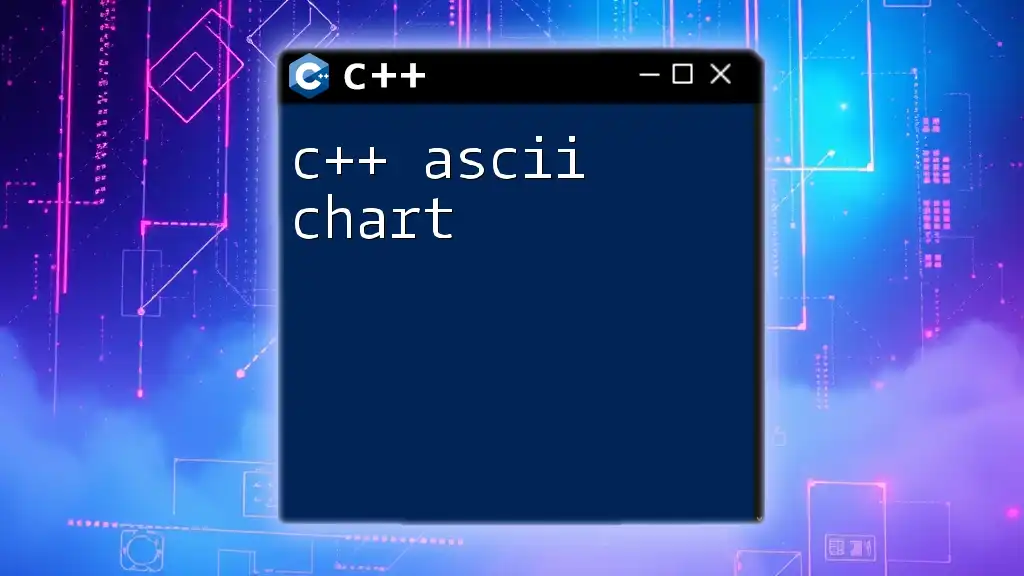
The C++ `char` Data Type
Understanding the `char` Type
In C++, the `char` data type is used to represent single characters. It occupies one byte of memory and can hold any character from the ASCII set.
The `char` type can be characterized as follows:
- Size: Typically 1 byte (8 bits).
- Range: Values can range from -128 to 127 (for signed `char`) or 0 to 255 (for unsigned `char`).
How Characters are Represented
Character encoding is the method used to convert characters into byte values. In C++, a `char` is usually represented as an integer value in the background, permitting straightforward manipulation and arithmetic operations. Comparing `char` to other data types like `int` or `unsigned char` helps to understand their usage:
- `char`: Intended for storing characters.
- `int`: Used for numeric operations, but can also represent ASCII values via typecasting.
- `unsigned char`: Similar to `char` but avoids negative values, giving a larger positive range for ASCII characters.
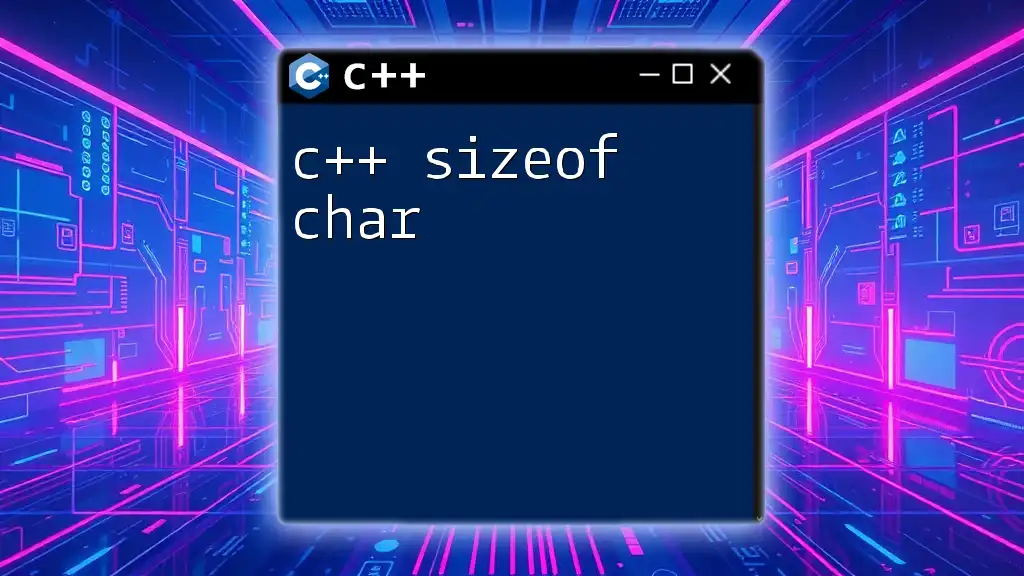
Conversion from ASCII to `char`
Why Convert ASCII to `char`?
Converting ASCII values to `char` is crucial in various contexts, such as:
- Reading input from devices or sensors that output ASCII values.
- Manipulating strings and characters in text processing applications.
- Implementing features that require character display based on user input or data processing.
Methods for Conversion
Using Typecasting
Typecasting is one of the simplest methods to convert an ASCII value to a `char`. The syntax involves casting an integer to a `char`.
For instance:
int asciiValue = 65; // ASCII for 'A'
char character = (char)asciiValue;
In this example, the ASCII value of `65` is directly converted to the character 'A'. This straightforward approach makes typecasting a common practice in C++ for ASCII to `char` conversions.
Using the `static_cast` Operator
Alternatively, the `static_cast` operator offers a more robust and type-safe way to perform conversions.
The code snippet below demonstrates this:
int asciiValue = 66; // ASCII for 'B'
char character = static_cast<char>(asciiValue);
Using `static_cast` is recommended as it explicitly states the intention of the conversion and aids in maintaining type safety, thus avoiding unexpected behaviors.
Function to Convert ASCII to `char`
Creating a reusable function simplifies repeated conversions throughout your code. Here is a sample function for this purpose:
char asciiToChar(int ascii) {
return static_cast<char>(ascii);
}
This function takes an integer `ascii` as a parameter and returns the corresponding character by converting it using `static_cast`. This modular approach not only enhances code readability but also allows for consistent use throughout the program.
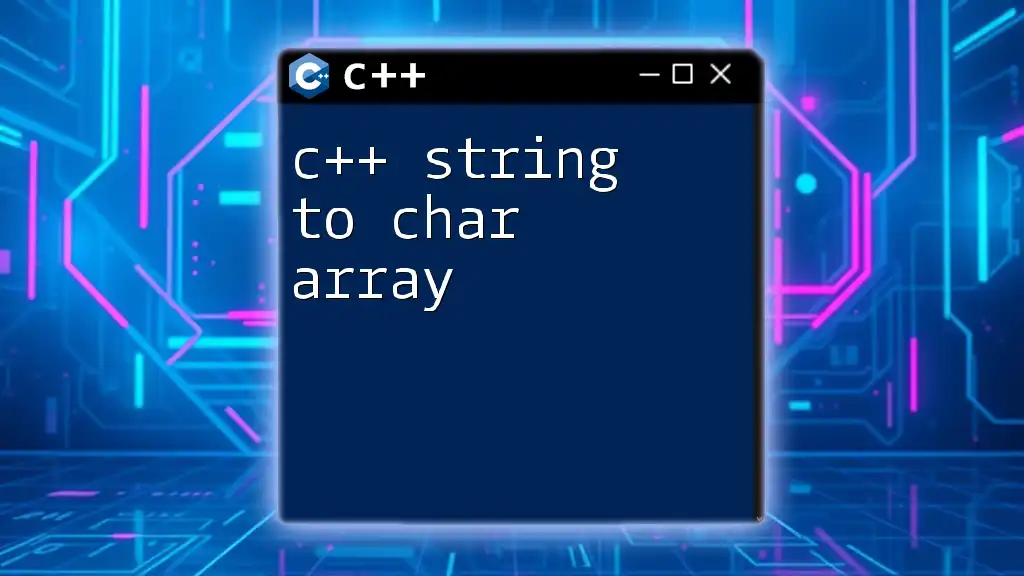
Handling Edge Cases
What Happens with Invalid ASCII Values?
It's vital to ensure the ASCII value lies within a valid range. The standard ASCII range is from 0 to 127. For values outside this range, unexpected behaviors may occur.
For example:
int asciiValue = 128; // Invalid in standard ASCII
char character;
if (asciiValue >= 0 && asciiValue <= 127) {
character = static_cast<char>(asciiValue);
} else {
// Handle error
}
In this snippet, the code checks if the `asciiValue` is valid. If it isn't, appropriate error handling can be implemented to avoid issues in the program.
Signed vs. Unsigned Characters
The distinction between signed and unsigned characters is significant when handling ASCII values:
- Signed char allows representing negative values, which can lead to misinterpretation of certain characters when converted from ASCII.
- Unsigned char strictly represents positive values, corresponding directly to ASCII values, thus preventing potential errors during conversion.
Choosing between signed and unsigned depends on the application requirements, especially when working with a larger character set or non-standard ASCII values.
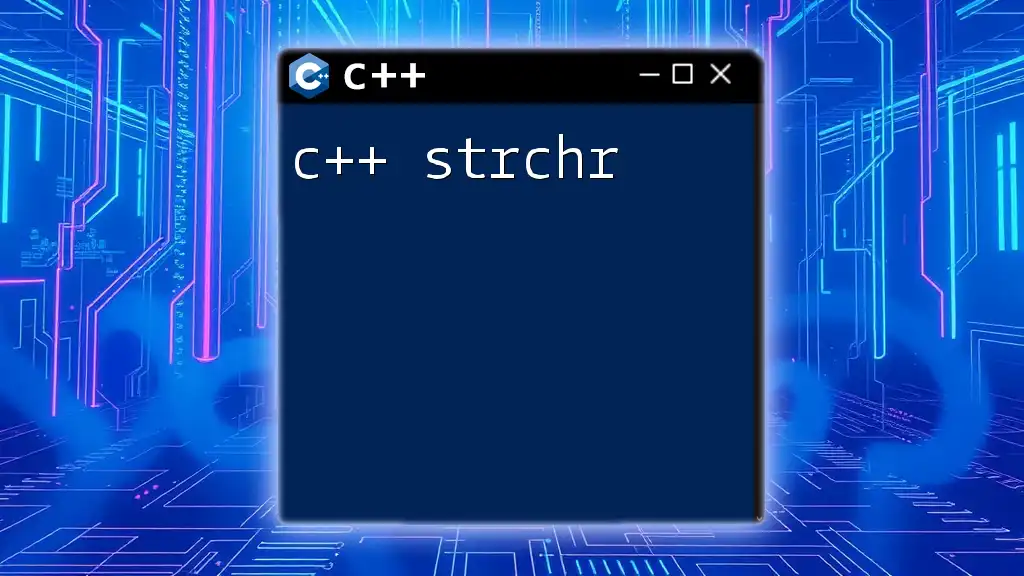
Conclusion
Understanding C++ ASCII to char conversion is essential for developers looking to manipulate characters effectively within their applications. With insights into ASCII, `char` data types, conversion methods, and handling edge cases, readers can confidently apply these concepts in their programming practices.
By familiarizing yourself with ASCII and the corresponding C++ mechanisms, you equip yourself with the tools needed to handle text data efficiently. Practice with provided examples and explore variations of this fundamental concept to deepen your comprehension and skillset.
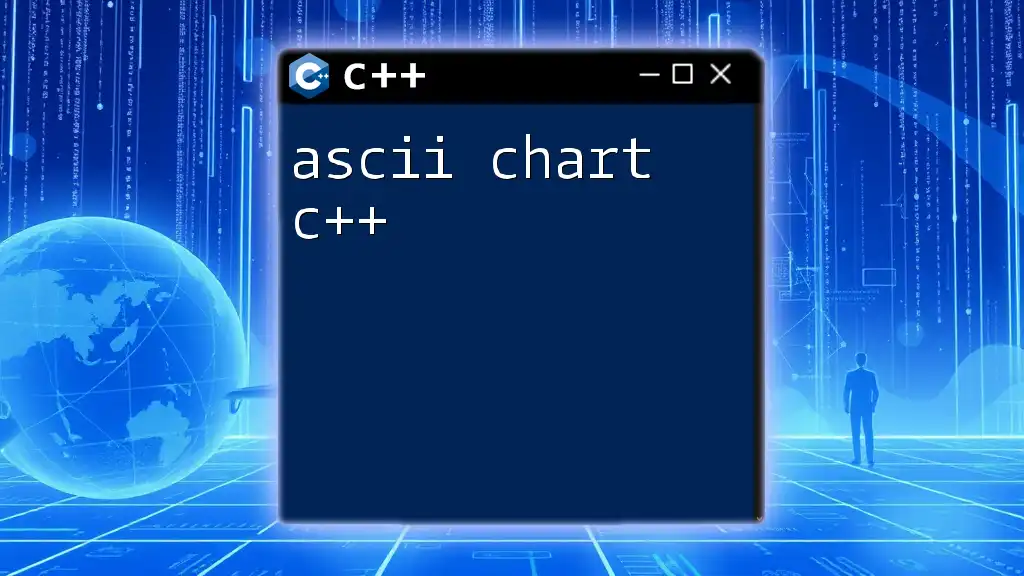
Additional Resources
For those seeking further knowledge, various digital resources such as official documentation, tutorials, and coding exercises are available to reinforce learning. Engage with hands-on coding challenges related to ASCII and `char` conversions to solidify your understanding and mastery of these vital programming skills.