The `sizeof` operator in C++ is used to determine the size, in bytes, of the `char` data type, which is typically 1 byte on most systems.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Size of char: " << sizeof(char) << " byte(s)" << std::endl;
return 0;
}
Understanding the sizeof Operator in C++
In C++, the sizeof operator is a powerful tool that allows programmers to determine the size, in bytes, of various data types including variables, structures, and classes. The primary purpose of `sizeof` is to return the total number of bytes that a particular data type occupies in memory.
Syntax of the sizeof Operator
The syntax for using the sizeof operator is straightforward:
sizeof(type)
or
sizeof variable_name
Using `sizeof` with types is particularly helpful, as it enables programmers to understand how much memory each type requires.
Example Code Snippet Demonstrating sizeof
Here's a simple example of using `sizeof` to print the sizes of various types:
#include <iostream>
int main() {
std::cout << "Size of int: " << sizeof(int) << " byte(s)" << std::endl;
std::cout << "Size of float: " << sizeof(float) << " byte(s)" << std::endl;
std::cout << "Size of double: " << sizeof(double) << " byte(s)" << std::endl;
return 0;
}
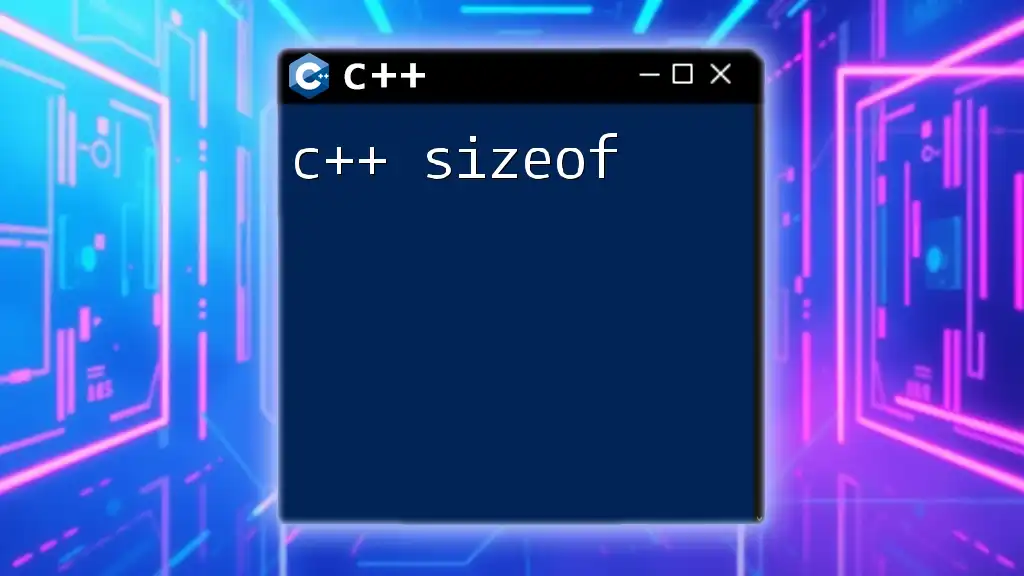
What is the Size of Char in C++?
In C++, the size of the char data type is a foundational aspect that every programmer should understand. By definition, the size of a `char` is guaranteed to be at least 1 byte. In many systems, a byte consists of 8 bits, which is the most common representation of a character.
Importance of Understanding Char Size for Memory Management
Knowing how much space a char consumes is crucial, especially in low-level programming, network communication, or memory-constrained environments. It allows developers to optimize memory usage and ensure efficient data storage.
Example of Checking Char Size Using sizeof
To check the size of char, one can simply write the following code:
#include <iostream>
int main() {
std::cout << "Size of char: " << sizeof(char) << " byte(s)" << std::endl;
return 0;
}
Upon execution, this code snippet will confirm that the size of a char is 1 byte.
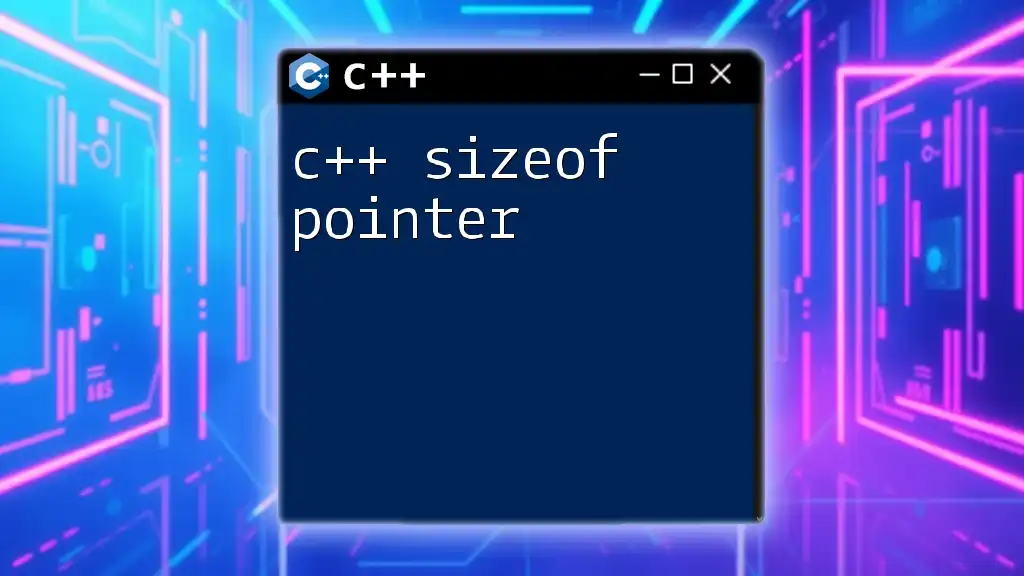
Char Size in C++ Standard
The C++ standard specifies that the size of a char must be exactly 1 byte. However, what constitutes a byte can vary across different architectures. For practical purposes, a byte is often synonymous with 8 bits, but this is not universal. Thus, the size of char provides an important baseline against which other types are measured.
Relationship Between Char, Signed Char, and Unsigned Char
It's also valuable to note that:
- char: A basic character type, typically used for individual characters.
- signed char: Can hold positive and negative values.
- unsigned char: Can only hold positive values.
The sizes of `signed char` and `unsigned char` are also guaranteed to be 1 byte.
Examples
You can test the sizes of different char types with this code:
#include <iostream>
int main() {
std::cout << "Size of signed char: " << sizeof(signed char) << " byte(s)" << std::endl;
std::cout << "Size of unsigned char: " << sizeof(unsigned char) << " byte(s)" << std::endl;
return 0;
}
This will confirm that both `signed char` and `unsigned char` occupy the same size as `char`, i.e., 1 byte.
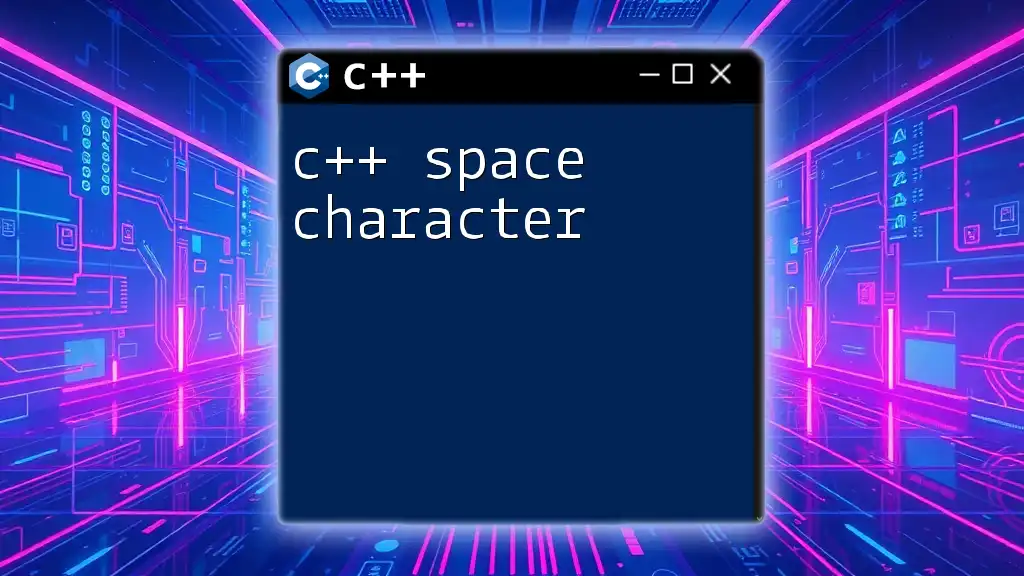
Char Size vs. Other Data Types in C++
Understanding the size of char in relation to other fundamental types in C++ can provide context for how data is stored and managed in your applications.
Comparison of Char Size with Other Fundamental Types
For instance, consider how sizes stack up:
- char: 1 byte
- int: Typically 4 bytes (but can vary)
- float: Typically 4 bytes
- double: Typically 8 bytes
The following code demonstrates this comparison:
#include <iostream>
int main() {
std::cout << "Size of char: " << sizeof(char) << " byte(s)" << std::endl;
std::cout << "Size of int: " << sizeof(int) << " byte(s)" << std::endl;
std::cout << "Size of float: " << sizeof(float) << " byte(s)" << std::endl;
std::cout << "Size of double: " << sizeof(double) << " byte(s)" << std::endl;
return 0;
}
This comparison highlights the efficiency of using `char` for storing single-character values versus the larger data types used for numbers.
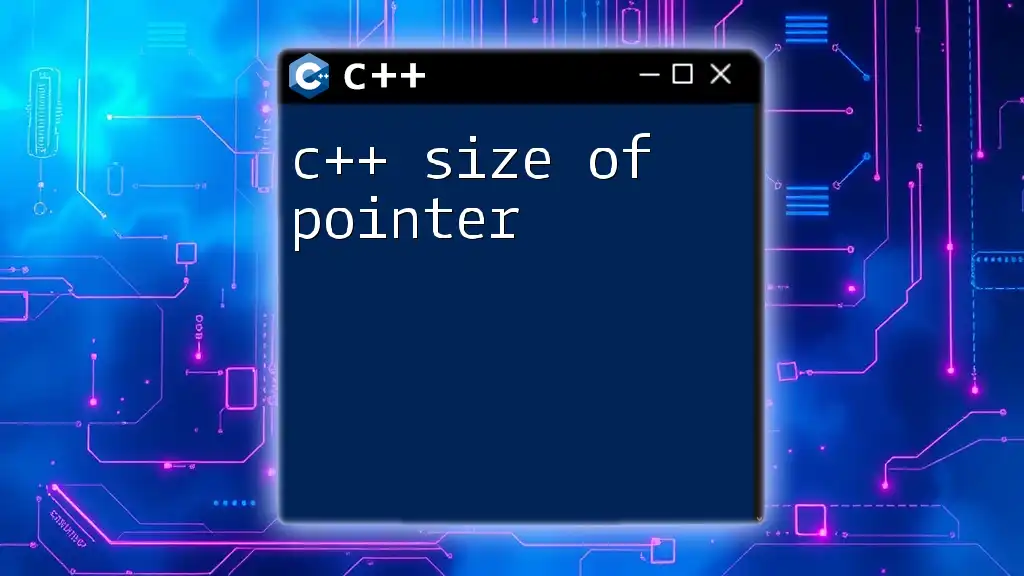
Practical Implications of Char Size
Understanding the size of char has real-world applications that can affect software performance and memory optimization.
Memory Allocation Considerations
When allocating memory for a character array or string, it's essential to consider the size of char. For example, if you are allocating array space for 100 characters, you can use:
char myArray[100]; // Allocates 100 bytes of memory
If you were to incorrectly assume that char takes up more or less space, it could lead to memory-related issues like overflow or underutilization.
Implications on Data Transmission and Processing
In scenarios like data transmission, knowing that a char takes 1 byte can help you calculate how many characters can fit within a particular bandwidth or storage space.
Example Scenario:
If you need to send a string of 100 characters over a network, and each char takes 1 byte, you’ll require at least 100 bytes of data capacity.
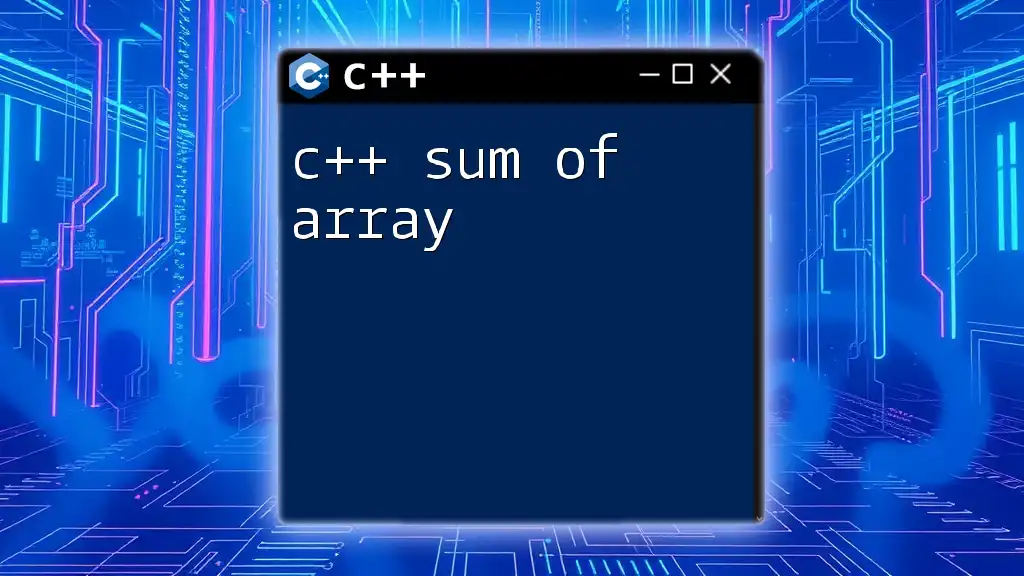
Char Type and Encoding in C++
Different character encoding formats can also influence how char type is handled in C++. The two most common encoding systems are ASCII and UTF-8.
How Char Size Plays a Role in Encoding Types
- ASCII: Each ASCII character is represented by 1 byte.
- UTF-8: A variable-length encoding where characters can use from 1 to 4 bytes based on the character being represented.
Example Explaining Storage for Different Encodings
char myChar = 'A'; // ASCII representation
char myUTF8Char[] = "あ"; // UTF-8 encoded character
In this example, `myChar` uses 1 byte, while `myUTF8Char` may occupy more than one byte, depending on how many characters are included.
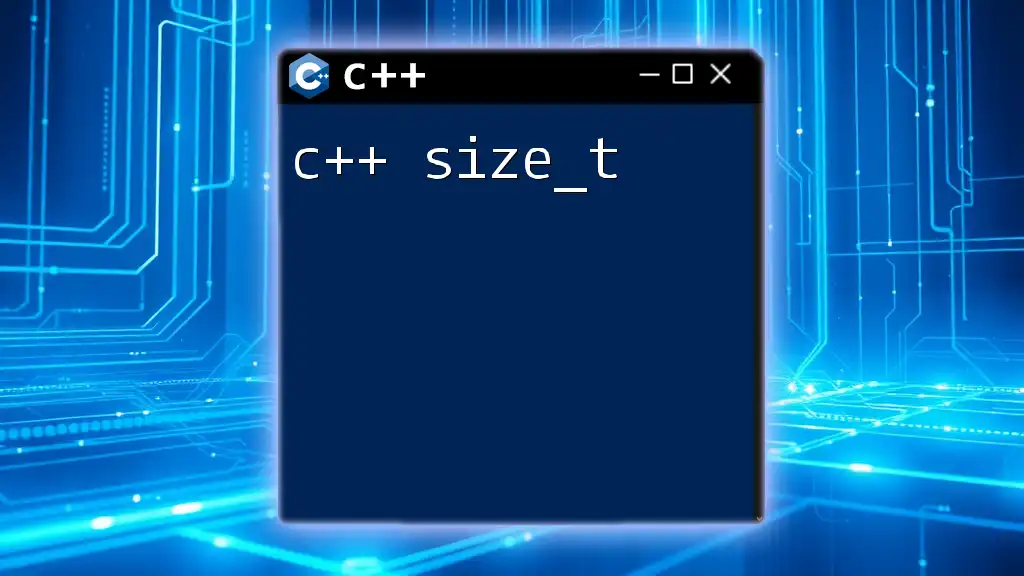
Conclusion
In summary, understanding `c++ sizeof char` is fundamental for C++ developers, especially when dealing with data management, memory optimization, and encoding. The guaranteed size of char provides a reliable benchmark for programmers to create efficient, robust applications. By knowing how char size relates to other types and understanding its implications in practical scenarios, developers can enhance their coding practices and improve the quality of their software.
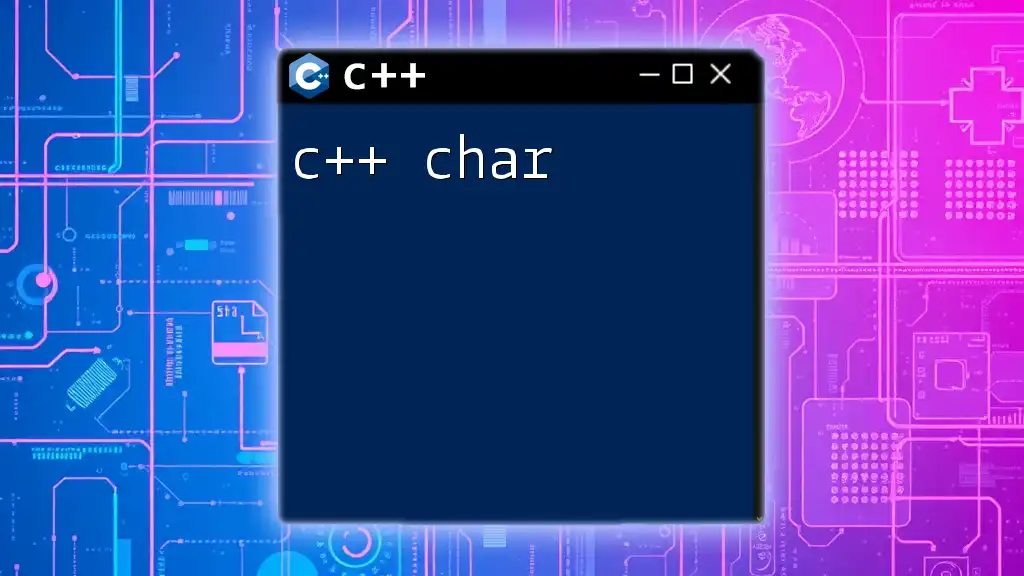
Additional Resources
For further exploration into this topic, consider checking out the official C++ documentation on data types, recommended textbooks on C++ programming, and online forums for community support and knowledge sharing. These resources can supplement your understanding of char size in C++, taking your skills to the next level.