The `typedef struct` in C++ allows you to define a new name for a structure type, enabling simpler usage throughout your code.
Here's a code snippet demonstrating its use:
typedef struct {
int id;
char name[50];
} Student;
Student s1; // now you can use Student directly without the 'struct' keyword
Understanding the Basics of Structs in C++
What is a Struct?
In C++, a struct is a user-defined data type that groups related variables under one name. Unlike classes, structs are mainly used for passive data structures that hold variables, while classes are more geared towards encapsulating behaviors and methods.
The basic syntax for defining a struct is as follows:
struct Point {
int x;
int y;
};
In this example, `Point` is a struct that contains two integer variables, `x` and `y`. This allows you to handle related data in a single unit, making your code cleaner and more manageable.
Example of a Simple Struct
Let’s take a closer look at our `Point` struct:
struct Point {
int x;
int y;
};
Point p; // Create a Point variable
p.x = 5; // Assign value to x
p.y = 10; // Assign value to y
Here, we have created a struct type called `Point` and instantiated it. By using this approach, you can easily manage points in a 2D space as a single unit.
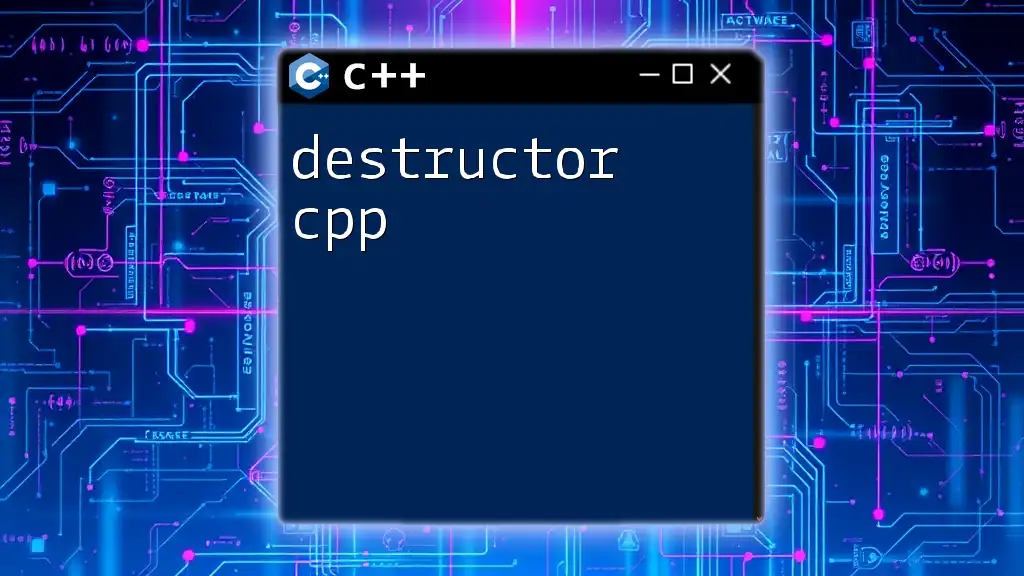
The Role of `typedef` in C++
What is `typedef`?
The typedef keyword in C++ allows you to create an alias for an existing data type, making your code more readable and simplifying the definitions of complex types. When used effectively, `typedef` enhances code clarity and can save you time in writing repetitive type declarations.
Example of Using `typedef`
Consider the following example:
typedef unsigned long ulong;
In this case, we have created an alias `ulong` for the type `unsigned long`. This lets us use `ulong` conveniently throughout our program without constantly typing out the longer type name.
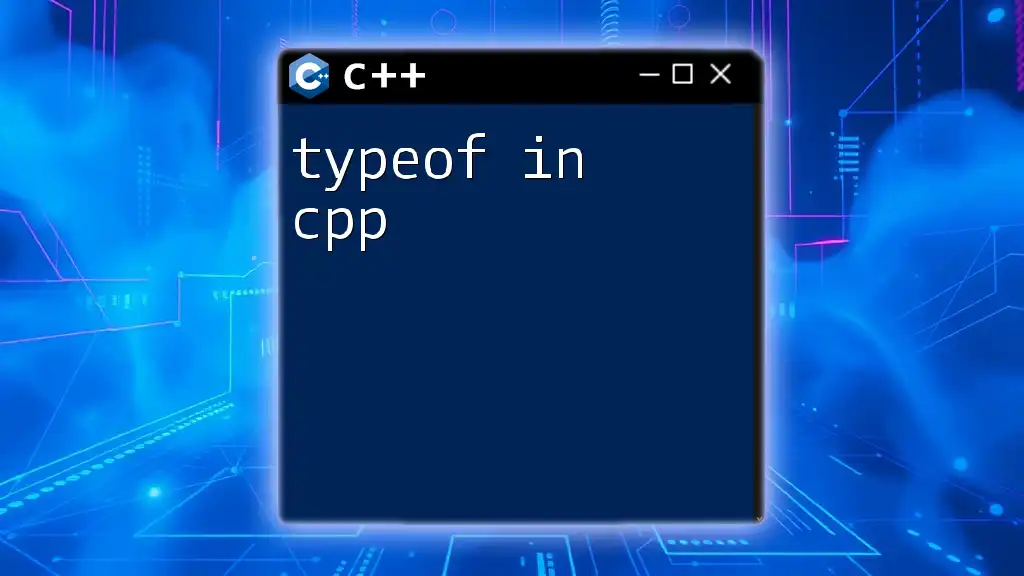
Combining `typedef` and `struct`
Why Use `typedef struct`?
Using `typedef struct` takes advantage of both `typedef` and `struct` to create concise and clear type definitions. This approach not only improves readability but also provides a simplified way to instantiate structs without mentioning the `struct` keyword every time.
Syntax and Structure of `typedef struct`
The general syntax when combining `typedef` with `struct` is as follows:
typedef struct {
int id;
char name[50];
} Employee;
In this example, we define an anonymous struct that holds an integer `id` and a character array `name`, representing an employee. With this combined approach, the `Employee` type can be used directly with `Employee emp;` without the `struct` keyword.
Example of Typedef Struct
Here’s another example:
typedef struct {
double radius;
} Circle;
Here, we define a struct to represent a circle, holding only the radius as a member. By using `typedef`, we can easily instantiate it as `Circle c;`.
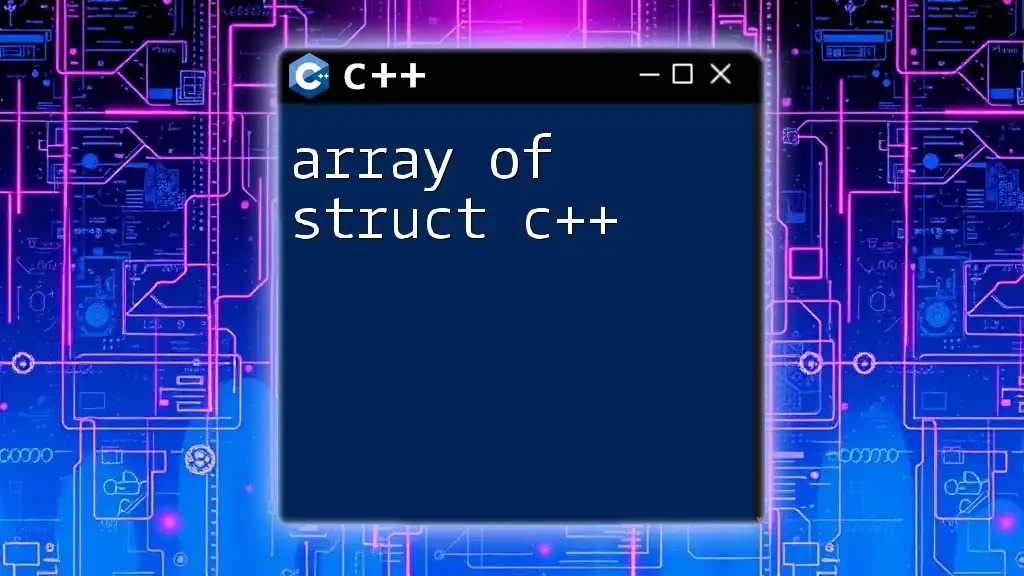
Practical Use Cases for `typedef struct`
Use Case: Managing Complex Data Structures
When dealing with complex data structures, using `typedef struct` can simplify management. For instance, if you’re working on a program that processes personal information, you may design a struct as follows:
typedef struct {
char name[100];
int age;
float height;
} Person;
This `Person` struct nicely encapsulates related personal data, allowing for easier passing around and manipulation of complex information.
Use Case: Creating Linked Lists
The linked list is a classic data structure example where `typedef struct` proves invaluable. Each node in a linked list is itself a struct, commonly defined with a pointer to the next node. Here’s how you might implement a linked list node:
typedef struct Node {
int data;
struct Node* next;
} Node;
In this code snippet, we define a `Node` struct that holds an integer `data` and a pointer `next` to another `Node`. This allows for the creation of linked lists, a powerful way to manage dynamic data storage.
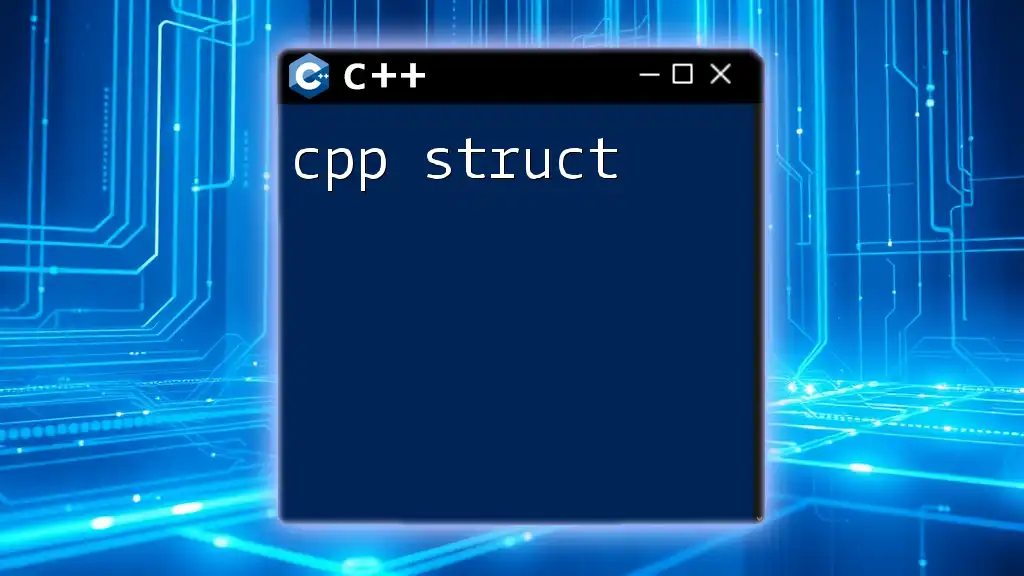
Common Mistakes to Avoid
Forgetting to Use `typedef`
A common mistake when using structs is neglecting `typedef`. This oversight can lead to cumbersome type names and make the code less clean. For instance, each use of the struct will require the prefix `struct`. Instead, using `typedef struct` can streamline your code.
Incorrectly Defining Structs
Struct definitions must be approached carefully. Omitting semicolons or using incorrect access specifiers can lead to compilation errors. Always ensure your struct has a proper definition with the necessary syntax.
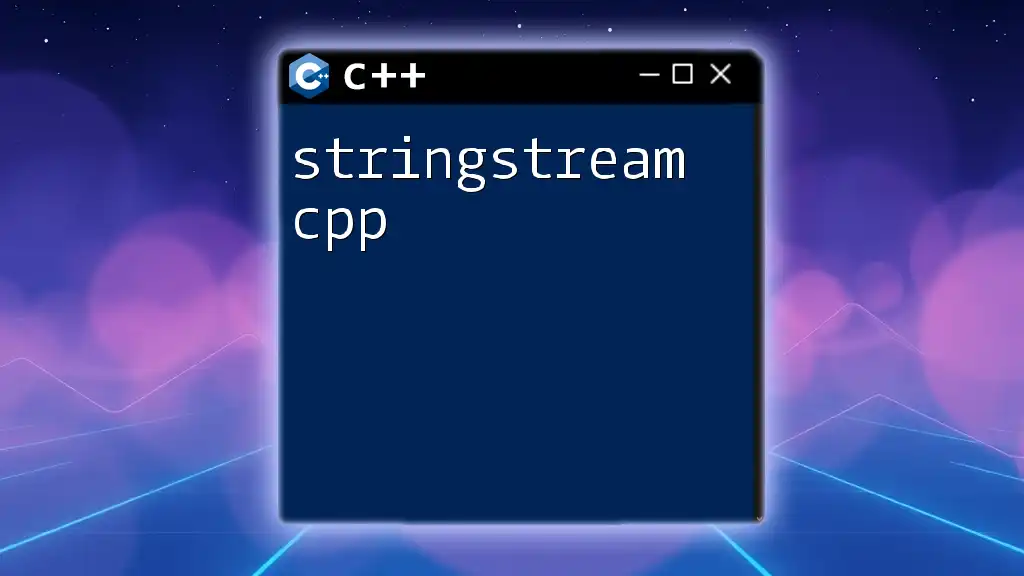
Best Practices for Using `typedef struct`
Keep It Simple and Descriptive
When naming your typedef structs, prioritize descriptive names that convey clear meaning. Simplicity aids maintainability and makes it easier for others to understand your code at a glance. Avoid excessively long or complex names.
Consistency in Use
Once you adopt `typedef struct` in your project, maintain consistency. Use it uniformly across your codebase to avoid confusion, especially in larger projects where varied definitions can lead to misunderstandings.
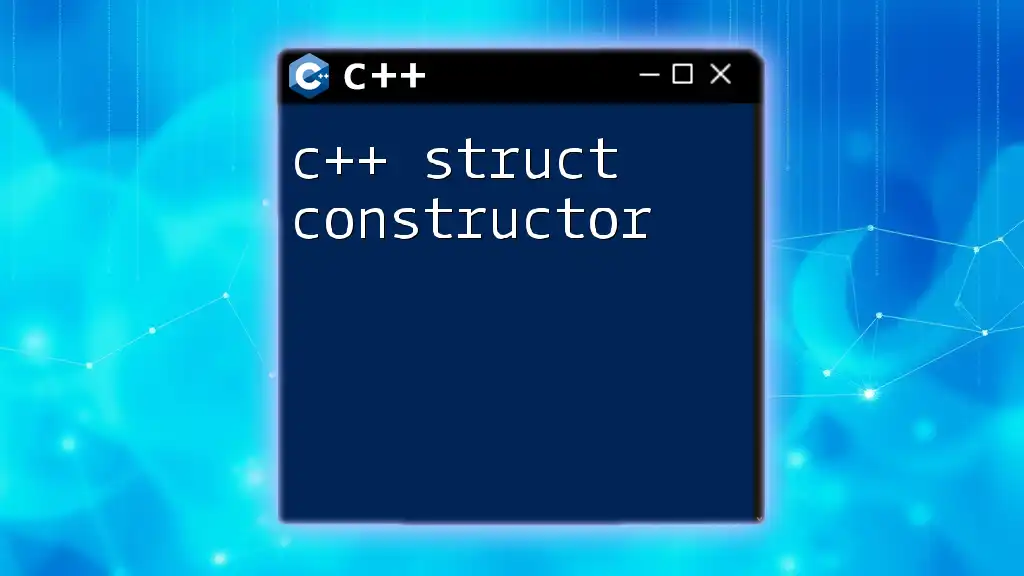
Conclusion
In summary, understanding how to effectively use `typedef struct cpp` significantly enhances the clarity and maintainability of your code. By combining the strengths of both `typedef` and `struct`, you can create efficient data types that simplify complex programming tasks. Practicing these techniques in your personal projects will equip you with valuable skills as you continue on your C++ journey.
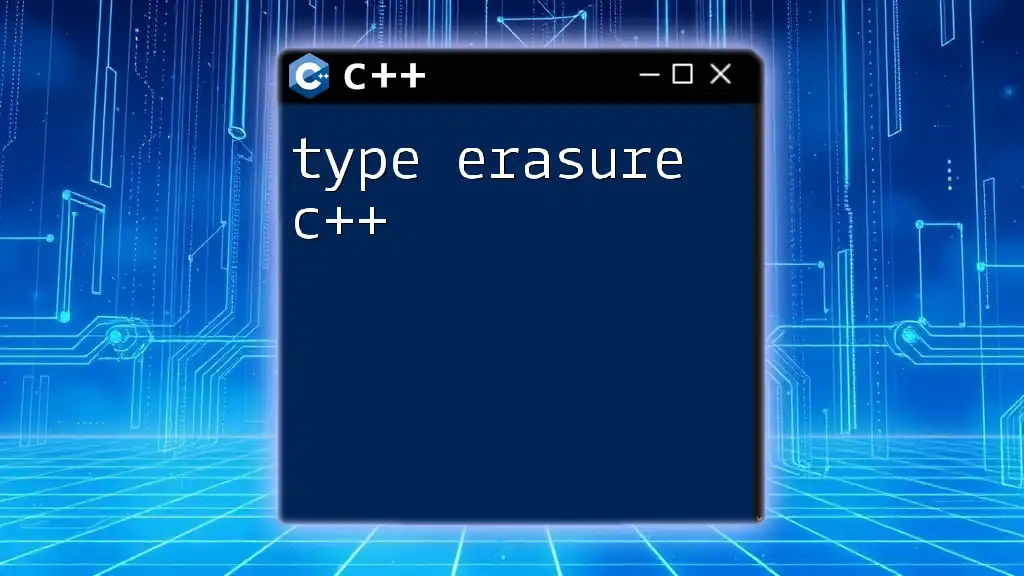
Additional Resources
To further your understanding and proficiency with `typedef struct`, explore additional readings and resources, including online tutorials, forums, and textbooks focused on C++ programming. Engaging with these materials will deepen your knowledge and help you apply what you've learned effectively in real-world scenarios.