In C++, the `empty()` function is typically used with containers like `std::string` and `std::vector` to check if they are devoid of elements or characters.
#include <iostream>
#include <vector>
#include <string>
int main() {
std::string str = "";
std::vector<int> vec;
std::cout << "Is string empty? " << std::boolalpha << str.empty() << std::endl; // Outputs: true
std::cout << "Is vector empty? " << std::boolalpha << vec.empty() << std::endl; // Outputs: true
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-level programming language that was developed as an extension of the C programming language. It introduced object-oriented features and is widely used in various domains such as game development, system programming, and application software. C++ is known for its performance, allowing developers to perform low-level memory manipulation while maintaining high-level programming features.
Why Learn C++?
Learning C++ can be immensely beneficial for any programmer. This language is the backbone of many systems and applications. Its ability to enhance control over system resources and memory management makes it a preferred choice in performance-critical applications. Mastering C++ pays off in job opportunities and equips developers with a solid understanding of programming principles that apply across many languages.

The Concept of "Empty" in C++
What Does "Empty" Mean?
In programming, "empty" generally refers to data structures that contain no elements. In C++, this can apply to various constructs such as vectors, strings, sets, and classes. Understanding how to handle these empty constructs is crucial for reliable and efficient programming.
Empty in C++ Standard Library
Empty Vectors
Vectors are dynamic arrays provided by C++ Standard Library. They can change size easily, making them a popular choice for storing collections of data. Checking if a vector is empty is straightforward:
std::vector<int> vec;
if (vec.empty()) {
std::cout << "Vector is empty!" << std::endl;
}
The `empty()` method returns a boolean value indicating whether the vector has any elements. An empty vector can lead to various scenarios where your algorithms should behave differently, such as avoiding unnecessary computations or handling edge cases effectively.
Empty Strings
Strings in C++ are represented by the `std::string` class. An empty string is one that has no characters. You can check if a string is empty similarly:
std::string str;
if (str.empty()) {
std::cout << "String is empty!" << std::endl;
}
Empty strings are often encountered in text processing, formatting, or input validation tasks. It's important to realize that an empty string can still be a valid input in many situations, so handling it correctly can save you from unexpected issues in your applications.
Empty Sets and Maps
Sets and maps are associative containers in C++. A set stores unique elements, while a map stores key-value pairs. To check if either of these is empty, you can use a similar approach:
std::set<int> mySet;
if (mySet.empty()) {
std::cout << "Set is empty!" << std::endl;
}
Understanding when a set or map is empty can facilitate efficient resource allocation and ensure that operations like traversal or searching do not yield errors or unnecessary computations.

Creating "Empty" Classes
Defining an Empty Class
An empty class in C++ is a class that does not have any member variables or methods. Although it may seem trivial, it serves important purposes in C++. Below is an example of how to define an empty class:
class MyEmptyClass {};
An empty class can still occupy space in memory even if it appears to do nothing. This space is generally 1 byte in size, ensuring that different instances of the empty class can still have distinct addresses.
Use Cases for Empty Classes
Polymorphism
Empty classes can play a crucial role in defining the base for inheritance. When combined with pure virtual functions, these classes can become a base for polymorphic behavior, enabling the creation of robust and scalable systems.
Placeholder in Template Programming
Empty classes are also used as placeholders in templates. This provides flexibility and can enhance code readability and maintainability while allowing developers to focus on specific implementations later.

Best Practices with Empty Elements in C++
Avoiding Performance Pitfalls
When working with collections, it's crucial to manage empty states correctly. Relying excessively on checks for emptiness can lead to performance issues. As a best practice, initialize your collections appropriately or use the `reserve()` method to preallocate resources, thus minimizing the overhead of dynamic resizing.
Meaningful Definitions
Understanding the significance of being "empty" is essential. Rather than merely checking states, consider the implications of emptiness within your algorithms. Choose meaningful names for your functions and variables related to "empty" constructs to make your code more self-explanatory.

Common Mistakes with Empty in C++
Beginners often overlook how to handle empty constructs effectively. Common mistakes include assuming a collection is non-empty without verifying it or treating an empty structure as an error case. This often leads to runtime errors, logic flaws, and inefficient code. Recognizing the importance of error handling and using guard clauses can help mitigate these issues, ensuring that your code robustly addresses various scenarios involving empty states.
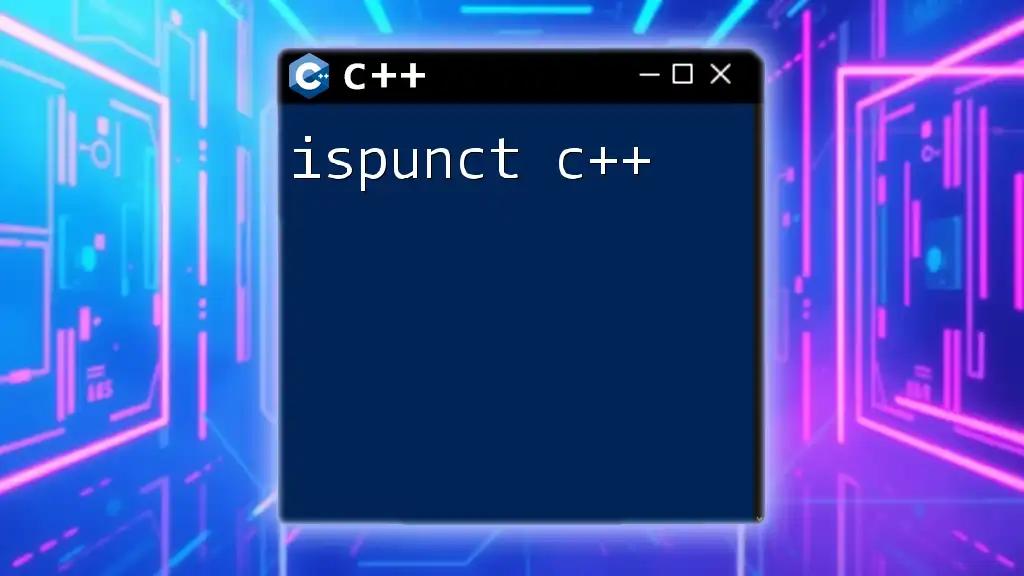
Conclusion
In summary, understanding the concept of "empty" in C++ is foundational for any programmer. Whether you are working with vectors, strings, sets, or creating classes, knowing how to efficiently manage emptiness will enhance your coding practices significantly. Mastering this topic aids in developing better algorithms and instilling confidence in your programming capabilities.

Additional Resources
Recommended Books and Online Courses
For further learning, consider resources such as C++ Primer by Stanley B. Lippman or The C++ Programming Language by Bjarne Stroustrup.
Coding Platforms for Practical Experience
Websites like LeetCode, HackerRank, and Codecademy offer a practical coding environment to reinforce your understanding of C++ constructs.
Community and Forums
Engaging with communities like Stack Overflow or the C++ subreddit can provide answers to specific questions and connect you with experienced developers willing to share insights.