MD5 hash in C++ can be computed using a library such as OpenSSL to transform data into a fixed-length 128-bit hash, commonly represented as a 32-character hexadecimal number.
#include <iostream>
#include <openssl/md5.h>
std::string md5(const std::string &data) {
unsigned char digest[MD5_DIGEST_LENGTH];
MD5((unsigned char*)data.c_str(), data.size(), (unsigned char*)&digest);
char md5str[33];
for(int i = 0; i < 16; ++i) {
sprintf(&md5str[i*2], "%02x", (unsigned int)digest[i]);
}
return std::string(md5str);
}
int main() {
std::string input = "Hello, World!";
std::cout << "MD5 Hash: " << md5(input) << std::endl;
return 0;
}
What is MD5?
MD5, or Message-Digest Algorithm 5, is a widely used hashing algorithm that produces a 128-bit (16-byte) hash value, typically represented as a 32-digit hexadecimal number. Developed by Ronald Rivest in 1991, MD5 was designed as a cryptographic hash function intended to provide a unique identifier for data.
Importance of Hashing in Computing
Hashing serves various essential roles in computing, including:
- Data Integrity: Ensuring that data has not been altered.
- Digital Signatures: Verifying the authenticity and origin of messages.
- Checksums: Quickly validating data integrity for files and transmissions.
Why Use MD5 in C++?
MD5 is particularly useful in C++ for several reasons:
- Performance: MD5 is relatively fast and efficient for many applications.
- Simplicity: The algorithm is straightforward to implement, making it friendly for developers.
- Wide Adoption: MD5 is widely recognized, meaning many libraries and tools support it.
However, it is essential to be aware of its vulnerabilities; while it is suitable for checksums and data integrity tasks, using MD5 for security-sensitive applications is not recommended.
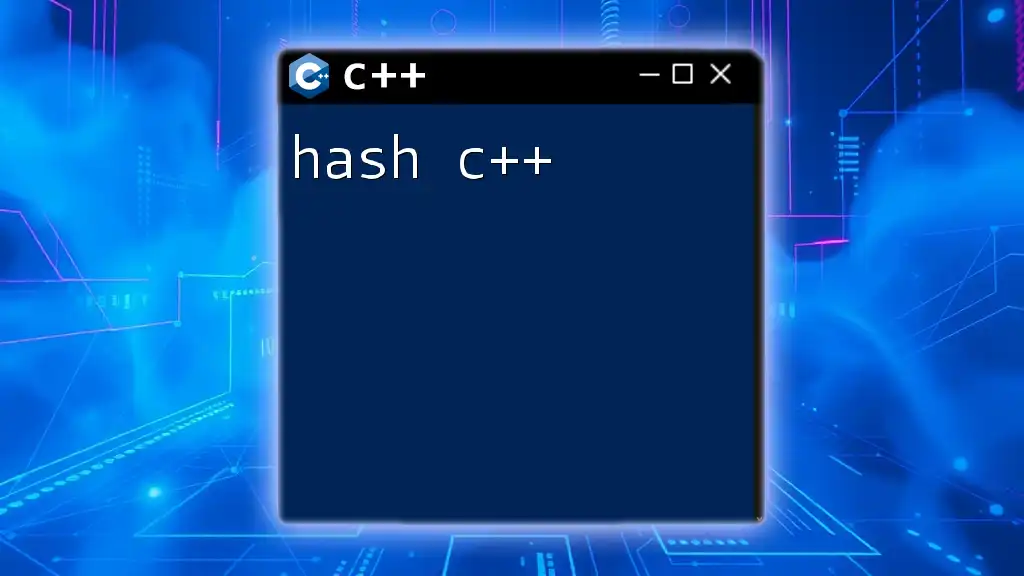
How MD5 Works
The MD5 algorithm operates through a series of steps that transform input data into a fixed-size output:
- Padding the Input: The data is padded so that its length is congruent to 448 modulo 512.
- Appending Length: The length of the original message (in bits) is appended as a 64-bit integer.
- Processing in Blocks: The padded data is processed in 512-bit chunks through a series of operations.
- Producing Output: The resulting hash is typically represented in hexadecimal format.
Key Features of the MD5 Hash Function
- Deterministic: The same input will always produce the same output.
- Fixed Size: Regardless of input size, the output is always 128 bits.
- Fast Computation: MD5 is efficient for computing hashes, allowing quick verification of data integrity.
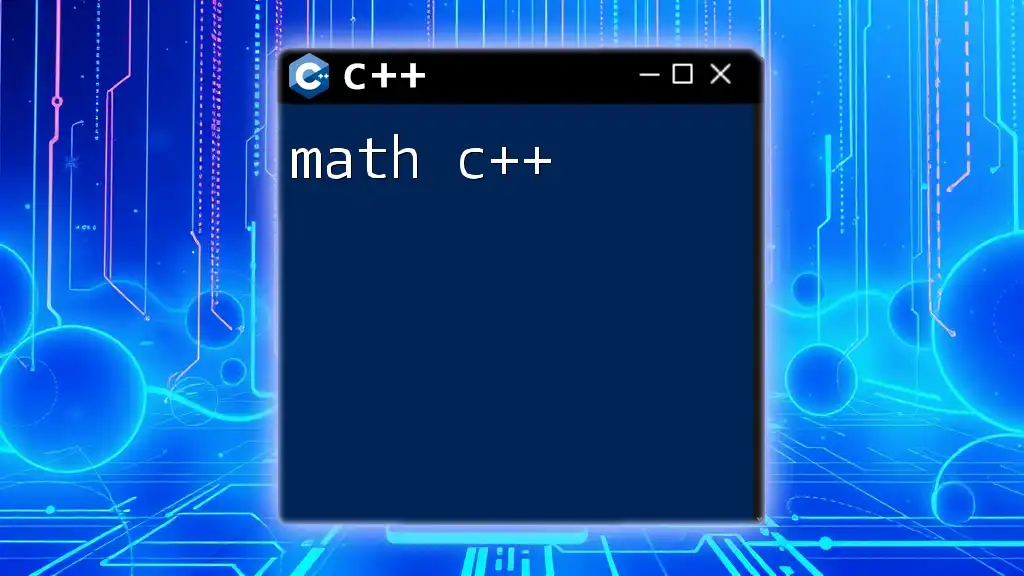
Setting Up Your C++ Environment
Requirements for C++ MD5 Implementation
To implement MD5 hashing in C++, you need:
- A compatible C++ compiler (such as GCC or MSVC).
- Relevant libraries to facilitate MD5 operations (e.g., OpenSSL or Crypto++).
Installing Required Libraries
Follow these steps to install OpenSSL, a widely used library for cryptography:
- Linux:
sudo apt-get install libssl-dev
- Windows: You can download precompiled binaries from the official OpenSSL website or use package managers like vcpkg or Conan.
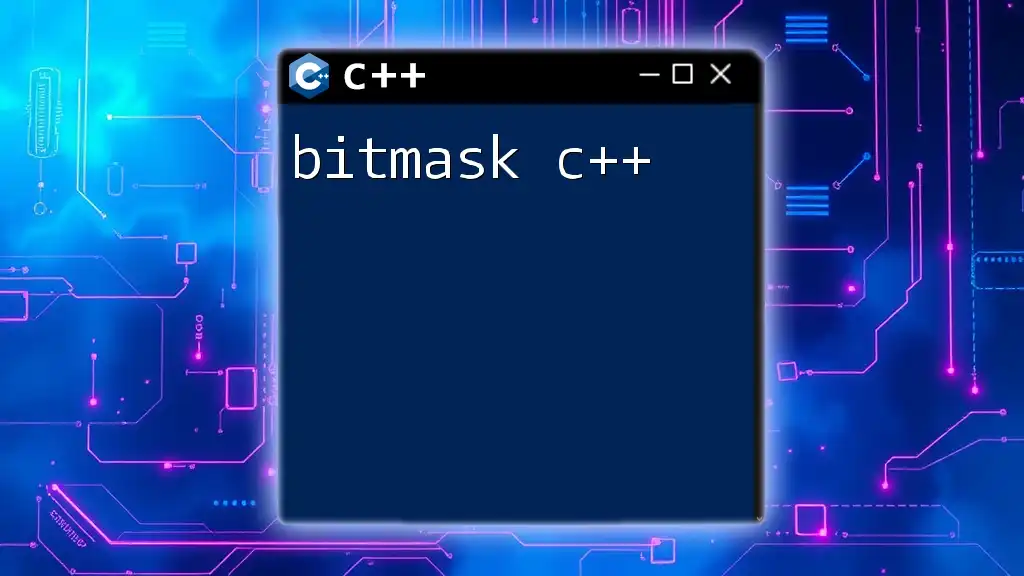
Implementing MD5 Hashing in C++
Writing Your First MD5 Function
To start, you can create a simple MD5 hashing function in C++ utilizing the OpenSSL library. Here's a code snippet to illustrate this:
#include <iostream>
#include <iomanip>
#include <sstream>
#include <openssl/md5.h> // Include necessary header for OpenSSL
std::string calculateMD5(const std::string &input) {
unsigned char digest[MD5_DIGEST_LENGTH];
MD5((unsigned char*)input.c_str(), input.size(), digest);
std::stringstream ss;
for(int i = 0; i < MD5_DIGEST_LENGTH; i++)
ss << std::hex << std::setw(2) << std::setfill('0') << (int)digest[i];
return ss.str();
}
Explanation of the Code Snippet
- Include Dependencies: The script starts by including necessary headers, particularly `<openssl/md5.h>`, which provides the functionality for MD5 hashing.
- Function Structure: The function `calculateMD5` takes a string as input, processes it through the MD5 function, and returns the resulting hash as a hexadecimal string.
- Digest Array: It initializes an array to hold the MD5 digest.
- Hex Conversion: Using `stringstream`, it converts the raw digest into a human-readable hexadecimal format.
Example of Using the MD5 Function
To demonstrate how to use the MD5 function, consider the following complete example:
int main() {
std::string data = "Hello, World!";
std::string md5Hash = calculateMD5(data);
std::cout << "MD5 Hash: " << md5Hash << std::endl;
return 0;
}
Running the above program will produce the MD5 hash of the input string "Hello, World!", showcasing a straightforward implementation of MD5 in C++.
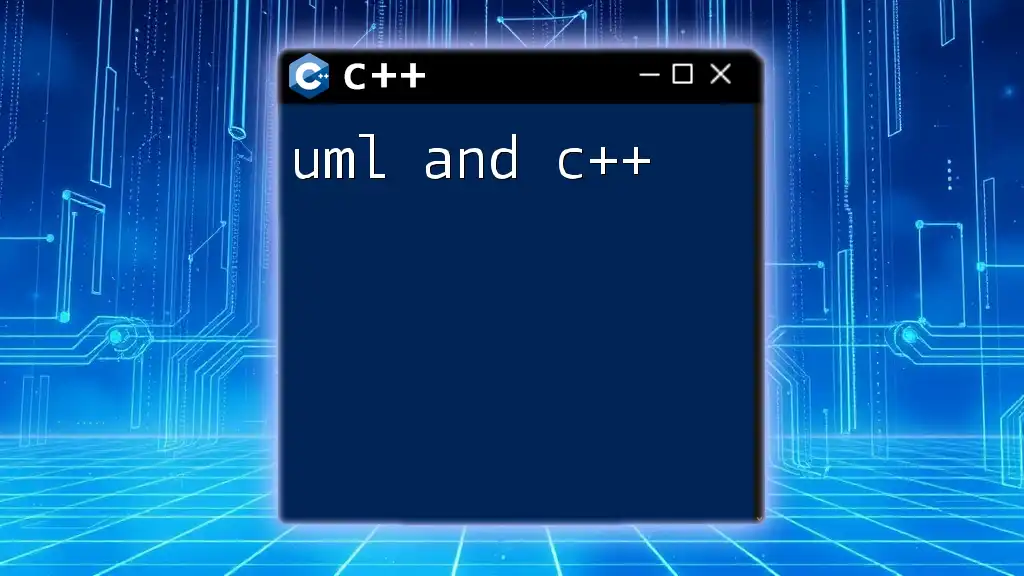
Advanced Usage of MD5 in C++
Handling Large Data Inputs
When dealing with large datasets, hashing them all at once is often inefficient. Instead, you can process the data in smaller chunks. The code below illustrates how to handle larger data streams using OpenSSL:
std::string calculateMD5Chunked(std::istream &input) {
MD5_CTX md5Context;
MD5_Init(&md5Context);
char buffer[1024];
while (input.read(buffer, sizeof(buffer))) {
MD5_Update(&md5Context, buffer, input.gcount());
}
MD5_Update(&md5Context, buffer, input.gcount()); // process any remaining bytes
unsigned char digest[MD5_DIGEST_LENGTH];
MD5_Final(digest, &md5Context);
std::stringstream ss;
for (int i = 0; i < MD5_DIGEST_LENGTH; i++)
ss << std::hex << std::setw(2) << std::setfill('0') << (int)digest[i];
return ss.str();
}
Comparing Two MD5 Hashes
You may often need to compare hashes to verify data integrity. This simple function checks if two hashes match:
bool compareHashes(const std::string &hash1, const std::string &hash2) {
return hash1 == hash2;
}
Using this function allows you to ensure that two sets of data are identical or have not been altered.
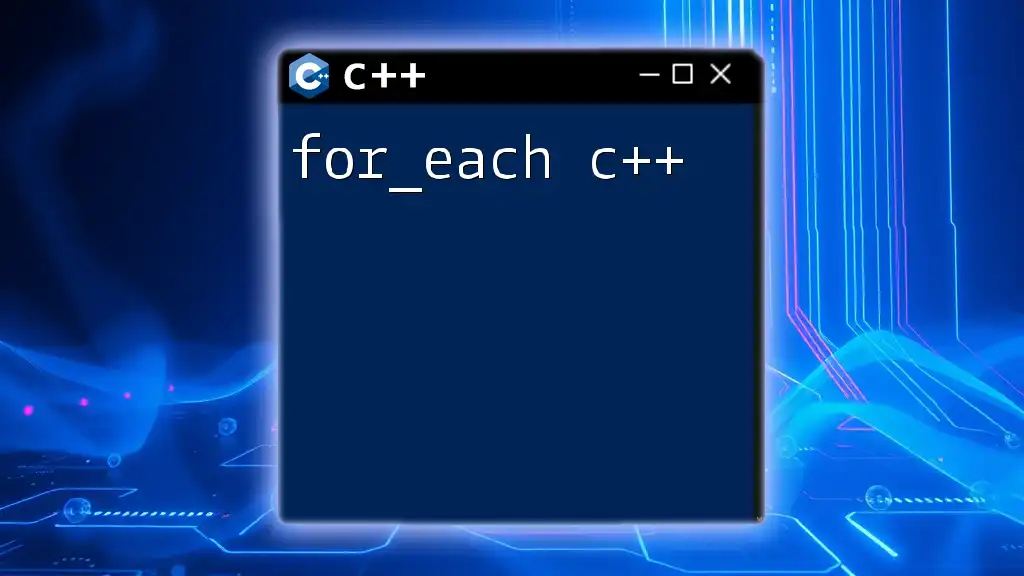
Use Cases and Best Practices
When to Use MD5 in Your Applications
MD5 can be utilized in applications such as:
- File Integrity Verification: Ensure that files downloaded from the internet are intact and unmodified.
- Checksums: Quickly check the state of blocks of data in various applications.
However, for security-critical applications such as password hashing or digital signatures, it is often better to consider more secure algorithms like SHA-256 or bcrypt, as MD5 is susceptible to collision attacks.
Best Practices for MD5 in C++
- Always validate user input before processing it with MD5.
- Consider using salted hashes if using MD5 for password storage, but still lean towards stronger algorithms for security.
- Keep libraries updated to ensure you have the latest patches and security improvements.
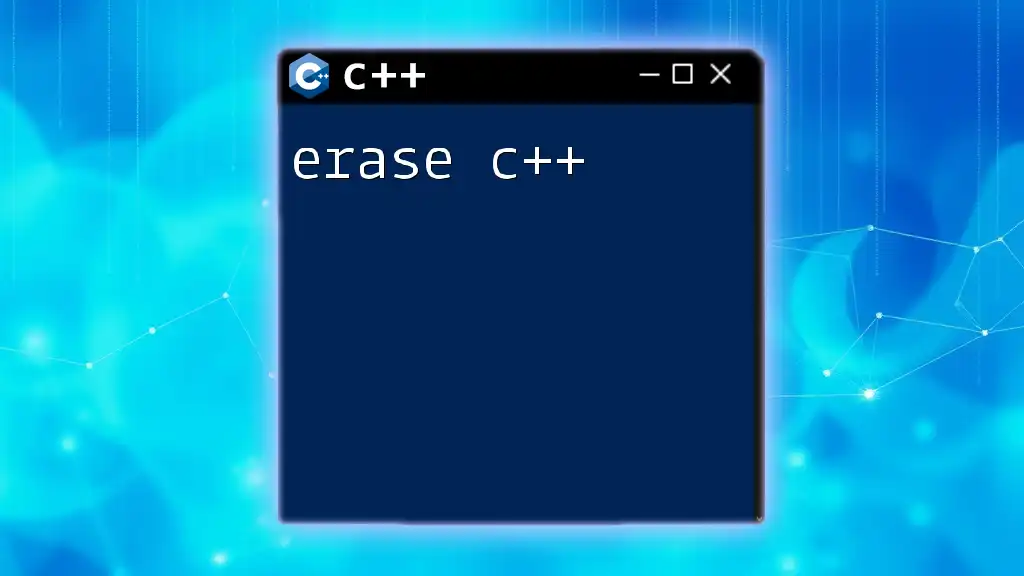
Conclusion
In summary, MD5 provides a convenient hashing solution for a variety of applications in C++, particularly for data integrity and checksums. Despite its limitations in security, it remains a useful tool in many scenarios. Understanding how to implement and use MD5 effectively requires practice, and incorporating best practices will help ensure safer operations in your programs.
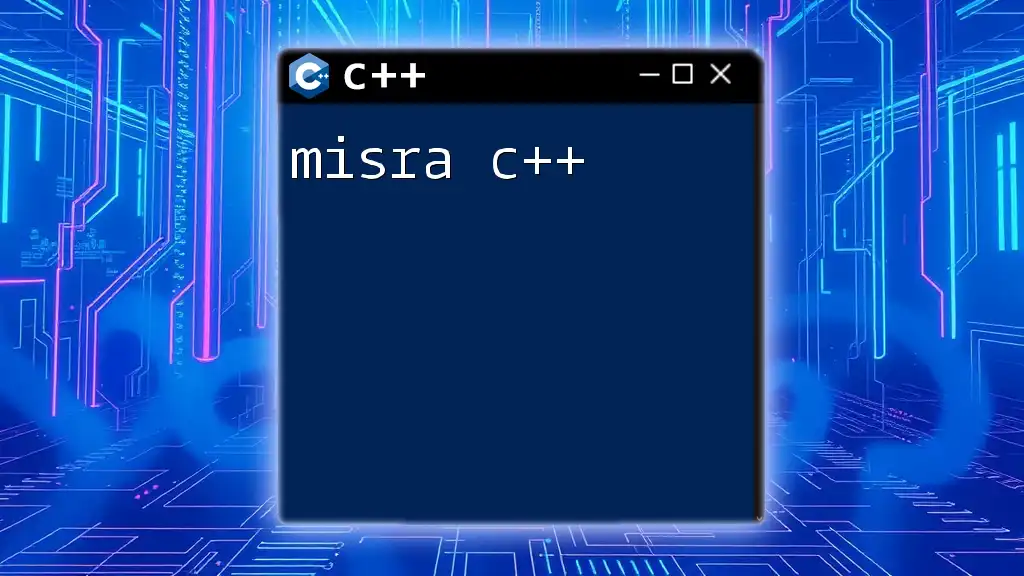
Further Reading and Resources
For those seeking to expand their knowledge further, consider exploring the following resources:
- The official [OpenSSL documentation](https://www.openssl.org/docs/)
- Books on C++ programming and cryptography
- Online coding resources and tutorials focused on hashing techniques
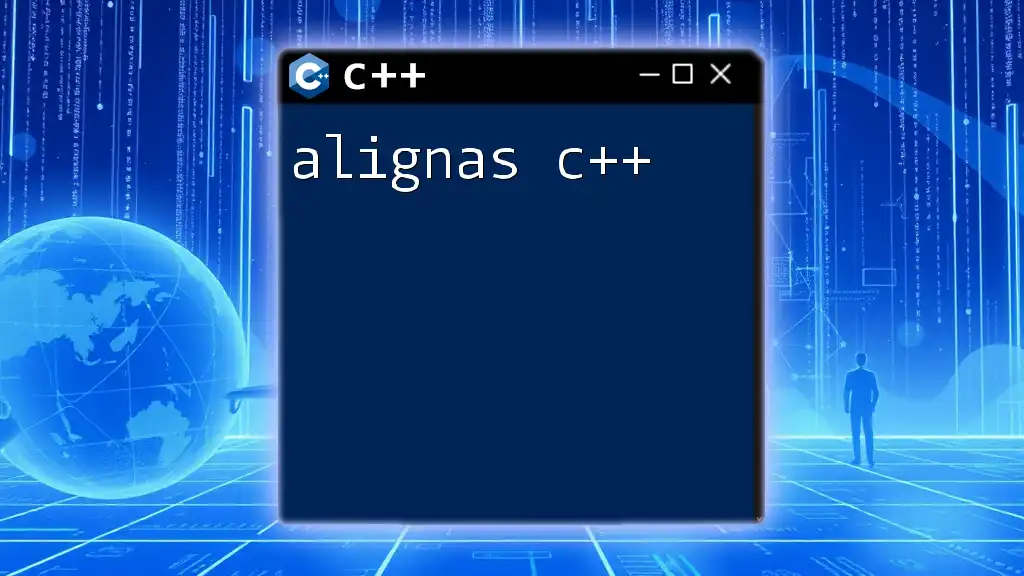
FAQs About MD5 in C++
What is the output of the MD5 function?
The output is a 128-bit hash represented as a 32-character hexadecimal string.
Can MD5 be reversed?
No, MD5 is a one-way hash function, meaning it is computationally infeasible to revert a hash back to its original input.
How secure is MD5 compared to other algorithms?
While MD5 is fast and efficient, it is no longer considered secure for cryptographic purposes due to vulnerabilities to collision attacks. Alternatives like SHA-256 provide stronger security for sensitive applications.