Format specifiers in C++ are placeholders used in input/output functions to control the formatting of the data being displayed or read, commonly used in conjunction with `printf` or `cout`.
Here’s a code snippet demonstrating format specifiers with `printf`:
#include <cstdio>
int main() {
int age = 25;
printf("I am %d years old.\n", age); // %d is a format specifier for integers
return 0;
}
Understanding the `printf` Function
What is the `printf` Function?
The `printf` function is a standard library function in C and C++ used primarily for outputting formatted text to the console. Unlike the C++ standard output streams, which utilize the `<<` operator, `printf` provides a way to finely control the format of the output. This makes it especially useful when precise control over text representation is required, such as in logging or when dealing with legacy systems.
Syntax of the `printf` Function
The basic syntax for using `printf` is:
printf(format_string, arguments...);
The `format_string` contains text along with format specifiers that dictate how subsequent arguments should be formatted within the output. For example:
printf("Hello, World!\n");
This outputs a simple greeting to the console.
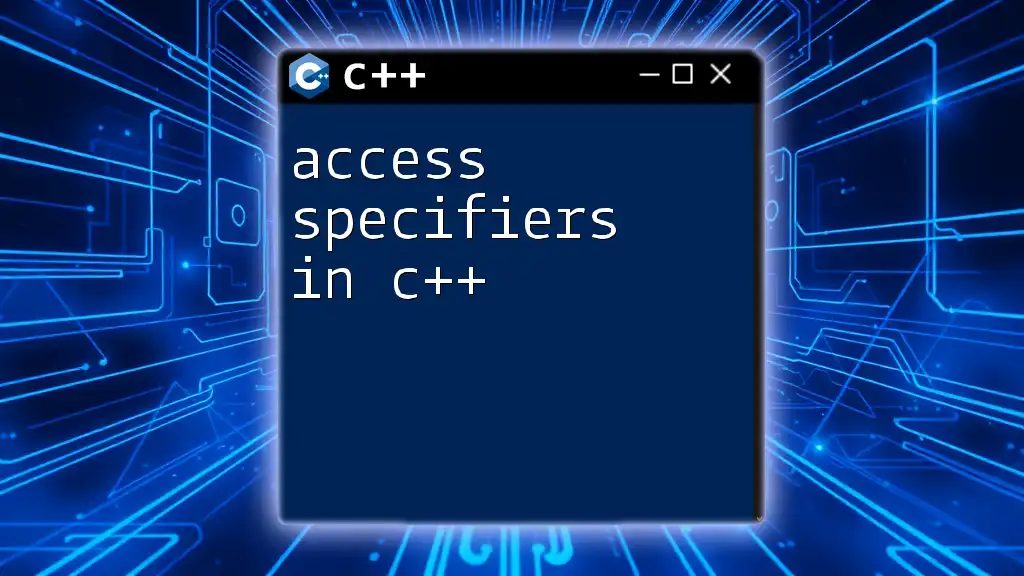
Breakdown of Format Specifiers in C++
What are Format Specifiers?
Format specifiers are placeholders within the format string that indicate how to format corresponding variables in the output. Using these specifiers correctly is crucial for displaying data types as intended. Learning and mastering format specifiers in C++ can significantly enhance your coding efficiency and output clarity.
Common Format Specifiers
Here are some of the most commonly used format specifiers in C++;
-
%d: Used for integer values. For example:
int num = 10; printf("The number is %d\n", num);
-
%f: Used for floating-point values. You can control the number of decimal places with precision. For example:
float price = 10.50; printf("The price is %.2f\n", price); // Displays: The price is 10.50
-
%c: Used for characters. For example:
char ch = 'A'; printf("Character: %c\n", ch); // Displays: Character: A
-
%s: Used for strings. For example:
char str[] = "Hello"; printf("String: %s\n", str); // Displays: String: Hello
Advanced Format Specifiers
Specifying Field Width
Field width can control how many characters to use in the output, padding with spaces if necessary. For example:
printf("|%10d|\n", 123); // Output: | 123|
Precision Control
For floating-point numbers, precision defines how many digits to display after the decimal point. For example:
printf("%.3f\n", 1.23456); // Output: 1.235
Flags in Format Specifiers
Format specifiers can include flags that alter how they behave:
-
-: Left-justify within the specified width.
printf("|%-.5d|\n", 7); // Output: |7 |
-
0: Zero-padding for numeric output.
printf("|%05d|\n", 42); // Output: |00042|
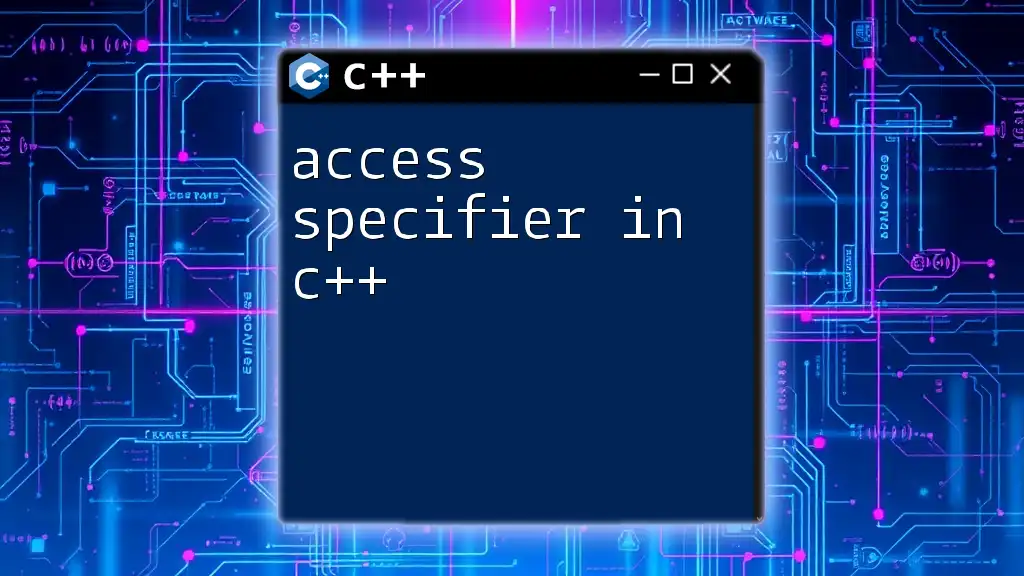
Formatting with Multiple Specifiers
It is also possible to combine multiple format specifiers in a single `printf` statement. This flexibility allows for robust and diverse output formatting. Here’s an example:
int age = 30;
float height = 5.8;
printf("Age: %d, Height: %.1f\n", age, height); // Displays: Age: 30, Height: 5.8
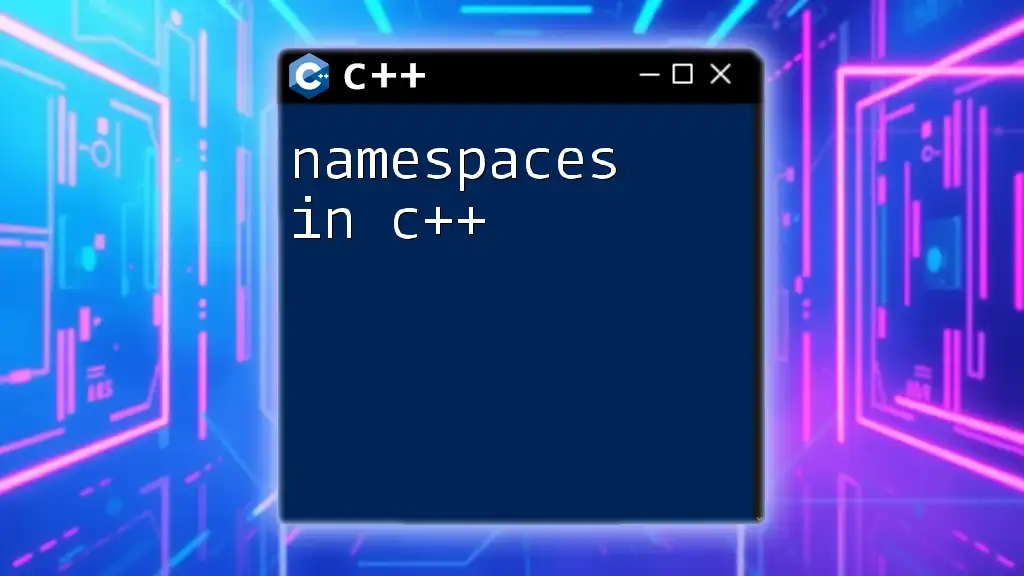
Best Practices for Using `printf`
When to Use `printf` vs. C++ Streams
While `printf` is great for formatted text output, C++'s standard input/output streams (like `std::cout`) are generally preferred for more complex C++ applications. Use `printf` when dealing with structured data or when you require precise control of formatting for tasks like logging.
Common Pitfalls to Avoid
When using `printf`, adhere to the following guidelines to avoid errors:
-
Mismatched Types: Ensure that the type of argument matches its corresponding format specifier. A common mistake is trying to print a float using the integer specifier, which can lead to undefined behavior.
-
Forgetting Additional Arguments: Always ensure that the number of format specifiers equals the number of arguments provided. Missing an argument can generate unexpected results or errors.
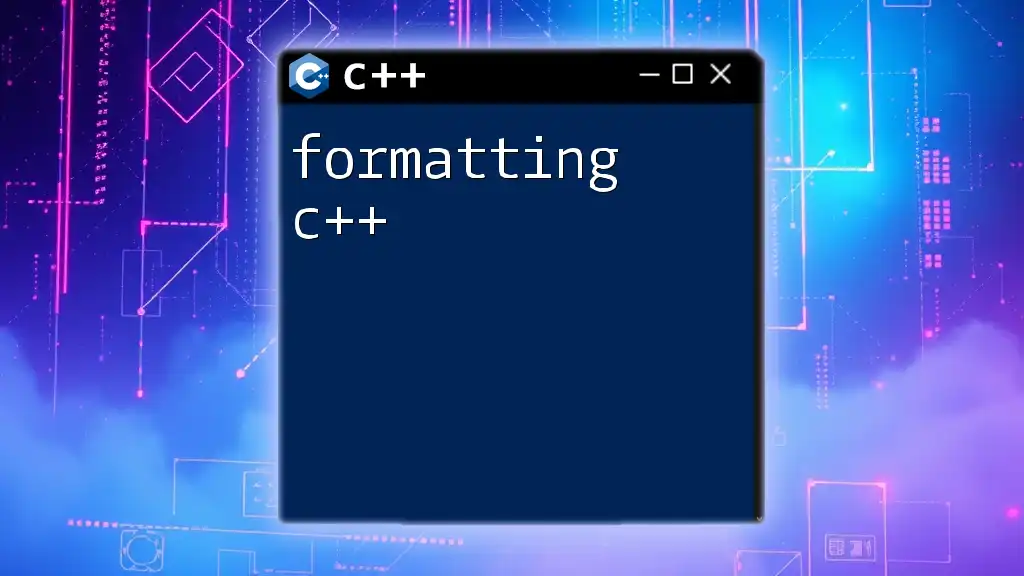
Conclusion
Understanding and effectively using format specifiers in C++ is crucial for creating outputs that communicate data clearly and efficiently. By familiarizing yourself with the various specifiers, flags, and best practices, you will be well-equipped to master formatting in your C++ programming endeavors. Practice using these format specifiers and explore different variations to become proficient and enhance your coding skills further.
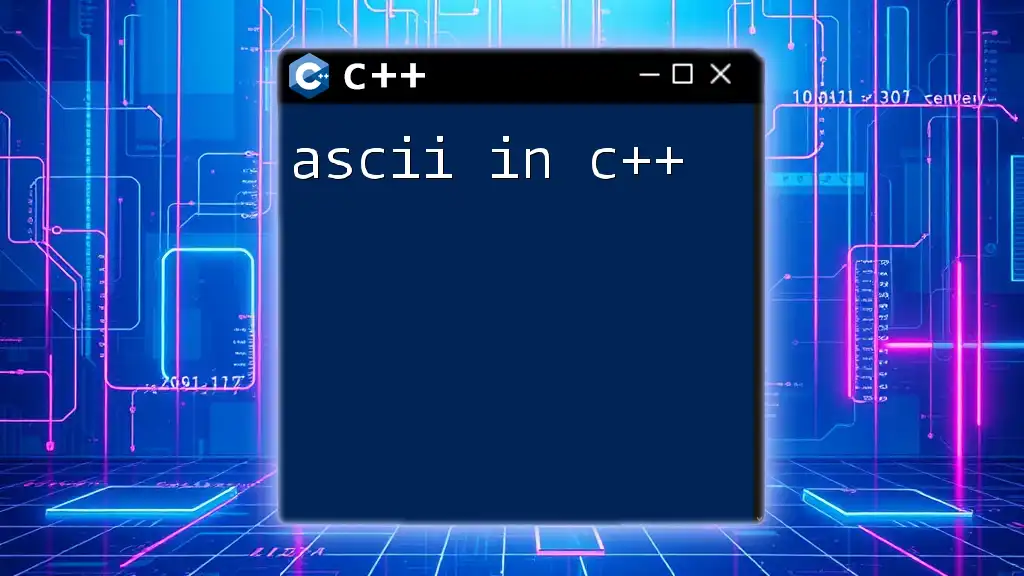
Additional Resources
For further reading on `printf` and formatting in C++, refer to documentation resources or online tutorials. Engaging in hands-on exercises can also deepen your understanding of format specifiers in practice, allowing you to apply what you’ve learned to real-world programming challenges.