Access specifiers in C++ control the accessibility of class members, with three primary types: `public`, `protected`, and `private`, determining how members can be accessed outside the class.
class Example {
public:
int publicVar;
protected:
int protectedVar;
private:
int privateVar;
};
Understanding Access Specifiers
What are Access Specifiers?
Access specifiers are crucial components of object-oriented programming in C++. They control the visibility and accessibility of class members, offering a mechanism for encapsulation and data hiding. By enforcing strict access rules, developers can ensure that the internal state of an object is protected from unauthorized access, creating a more robust program structure.
Types of Access Specifiers
C++ provides three primary access specifiers that dictate how and where class members can be accessed. Understanding each of them is essential for building secure and maintainable code.
-
Public
- The public access specifier allows members (variables and functions) to be accessed from anywhere in the program. It is primarily used for operations that need to be exposed to the outside world.
- Example:
class Sample { public: void show() { cout << "Public Access Specifier"; } };
- In this example, the `show` function can be called without any restrictions, making it suitable for user interactions or other external access points.
-
Private
- Private members are only accessible from within the same class. This encapsulation allows data to be hidden from outside interference, ensuring that the internal implementation can change without affecting other parts of the program.
- Example:
class Sample { private: int secret; public: void setSecret(int s) { secret = s; } };
- The `secret` variable is private and cannot be accessed directly from outside the `Sample` class. Access is provided only through the `setSecret` public method.
-
Protected
- The protected access specifier is a middle ground between public and private. Although it behaves like private in that it restricts access to the class itself, it also allows derived classes to access these members.
- Example:
class Base { protected: int value; public: void setValue(int v) { value = v; } };
- Here, `value` can be manipulated within the `Base` class and any class derived from it, facilitating code reuse while maintaining some level of protection.
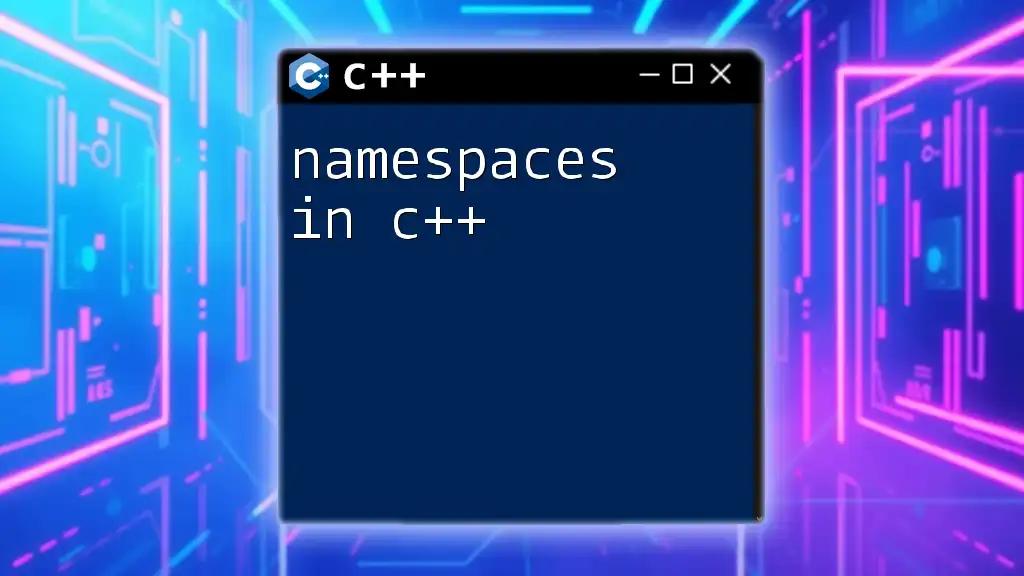
The Implications of Each Access Specifier
Public Access Specifier
Using the public specifier is ideal when the functionality needs to be exposed to other classes or external entities. Public members can be called without any restrictions, which is useful for methods that perform actions that need to be triggered by the user or other systems.
Private Access Specifier
Private access is essential for data encapsulation. By keeping class members private, you significantly reduce the risk of unintended interference or corruption of critical data. Private members can only be manipulated through public methods, allowing for controlled access and validation of data. This promotes the integrity of the object's state.
Protected Access Specifier
The protected specifier fosters inheritance by allowing derived classes to access base class members. This is particularly useful in creating a hierarchy of classes where common functionality can be shared while still restricting access to other, non-derived classes.
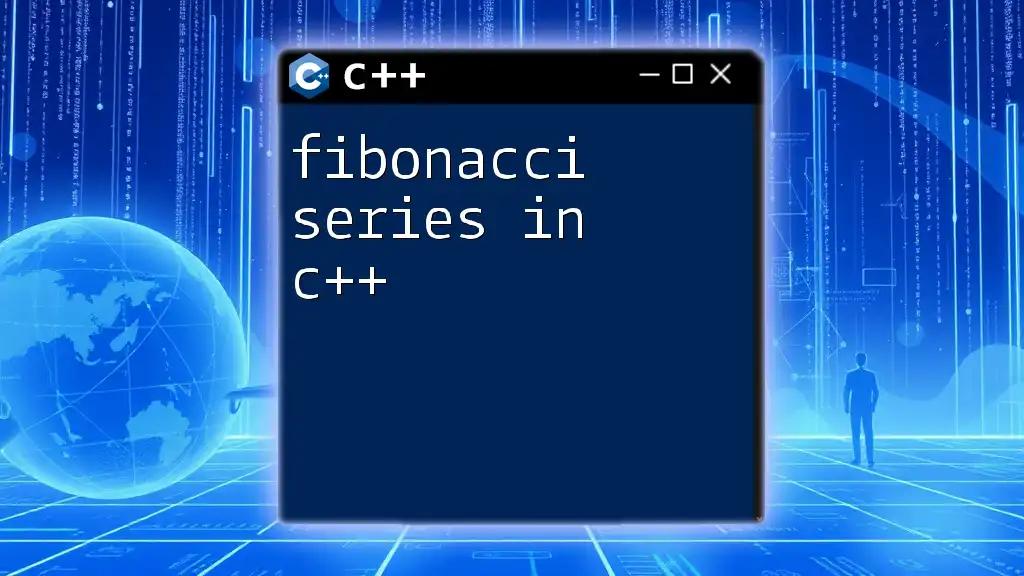
Access Specifiers and Inheritance
Base Class vs. Derived Class
In C++, the access specifiers significantly influence the behavior of classes and their derived counterparts. While public members of a base class remain accessible in derived classes, private members do not. This delineation enforces a strong encapsulation principle that can be tricky to navigate for novice programmers.
Access Specifier Effects on Inheritance
Let's look at a simple example to illustrate these concepts:
class Base {
public:
void pub() {}
protected:
void prot() {}
private:
void priv() {}
};
class Derived : public Base {
void show() {
pub(); // Accessible
prot(); // Accessible
// priv(); // Not Accessible
}
};
In this code snippet, `pub` and `prot` can be accessed by the `Derived` class, but `priv` remains off-limits. This illustrates how access specifiers help maintain clear boundaries between classes in an inheritance hierarchy.
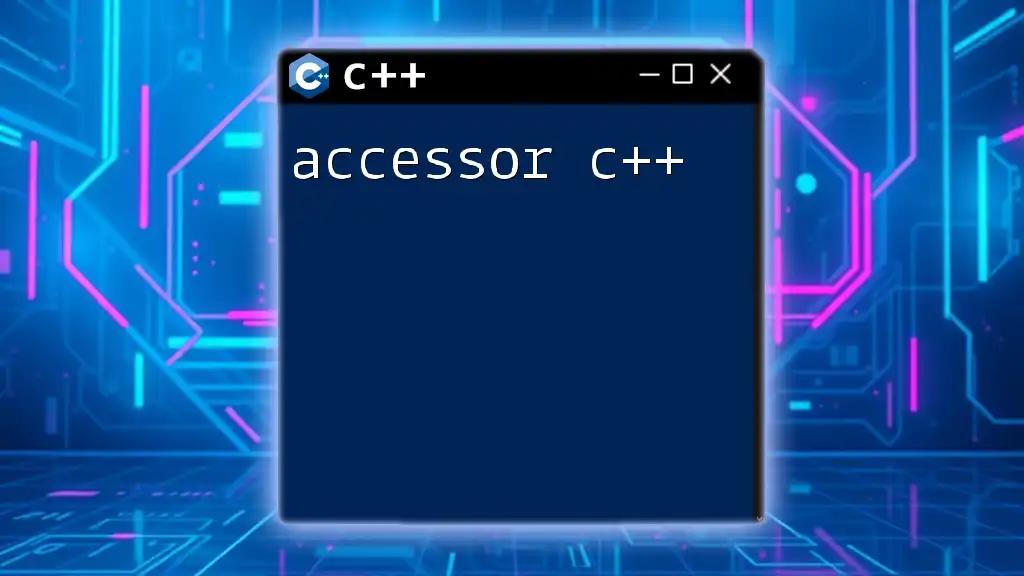
Best Practices for Using Access Specifiers
General Guidelines
For best results, use the most restrictive access level that meets your design requirements. This not only helps protect your data but also facilitates easier maintenance in the long run. A general approach is to keep members private unless you specifically need them to be public or protected.
Common Mistakes to Avoid
Avoid making all members of a class public unless absolutely necessary. This defeats the purpose of encapsulation and can lead to fragile code that is prone to bugs. Additionally, overlook protected members but use them judiciously only when you have a clear understanding of your class hierarchy, as they can lead to unintended access issues.

Conclusion
Mastering access specifiers in C++ is vital for effective object-oriented programming. They define how components of your program interact, ensuring that your data remains secure while allowing appropriate access where needed. By adopting best practices with access specifiers, you can develop cleaner, more maintainable, and robust code. As you continue to explore the depths of C++, always keep access control in mind for more secure software development.
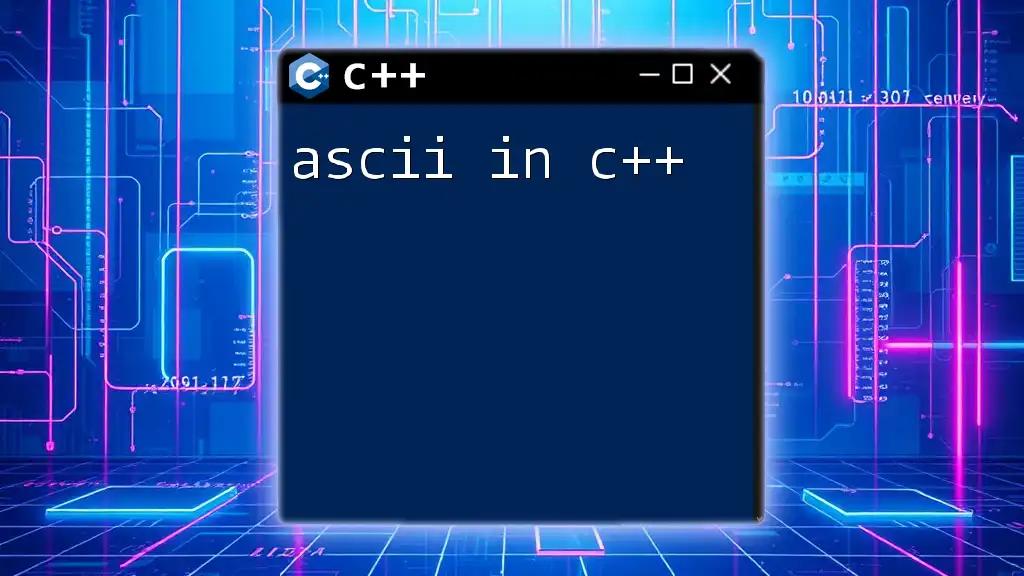
Additional Resources
For those wishing to deepen their understanding of access specifiers and overall C++ design principles, consider exploring recommended books and online tutorials. Engaging with interactive coding platforms can also provide practical experience, enhancing your learning journey in the world of C++.