In C++, an "undeclared identifier" error occurs when the compiler encounters a variable or function that has not yet been defined, leading to a failure in the code compilation.
Here's a code snippet demonstrating the error:
#include <iostream>
int main() {
std::cout << myVariable << std::endl; // Error: use of undeclared identifier 'myVariable'
return 0;
}
Understanding the Compilation Process
In C++, the compiler plays a critical role in determining whether your code will execute as intended. It analyzes your program in stages, including preprocessing, compilation, and linking. During this process, the compiler examines the identifiers you've used in your code to ensure they are declared properly.
When an undeclared identifier is encountered, the compiler raises a compilation error. Understanding where this error originates from helps you prevent it in the future. Typically, error messages related to undeclared identifiers might resemble this format:
error: 'identifier' was not declared in this scope
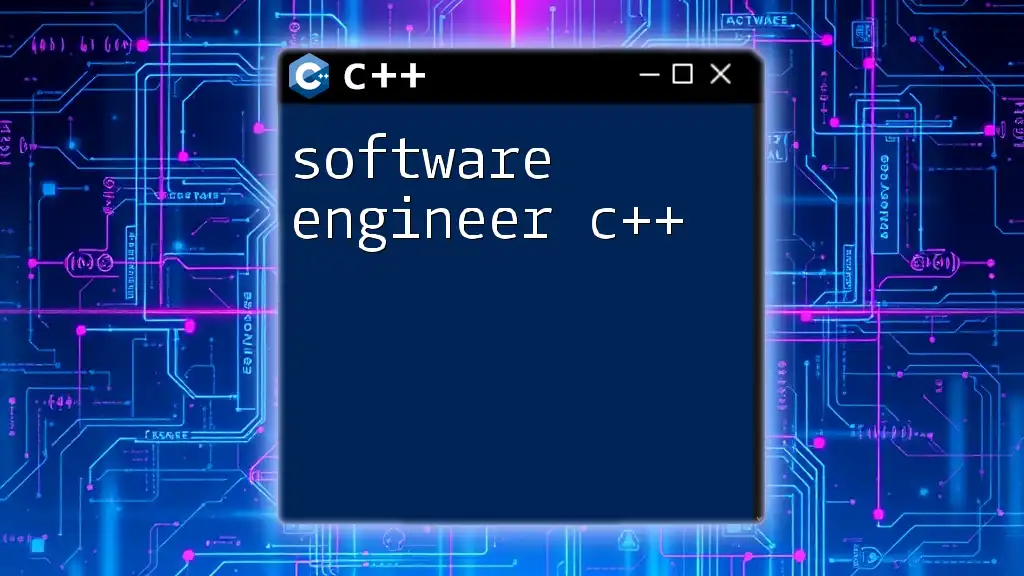
Causes of Undeclared Identifier Errors
The use of undeclared identifiers in C++ can stem from several common mistakes, often resulting from human error or misunderstanding of the principles of C++ programming.
Misspelling Identifiers
One of the simplest yet most frequent causes of undeclared identifier errors is a misspelled variable name. C++ is case-sensitive, meaning that `Variable` and `variable` would be considered two distinct identifiers. For example:
int myVariable = 10;
cout << myVariabl; // Error: 'myVariabl' was not declared in this scope
In the above snippet, the misspelled `myVariabl` leads to a compilation error because it has not been defined.
Using Identifiers Before Declaration
C++ requires that variables be declared before they are used. Attempting to use an identifier before its declaration will trigger an undeclared identifier error:
cout << myVariable; // Error: 'myVariable' was not declared in this scope
int myVariable = 10;
In this scenario, `myVariable` is referenced before it has been declared, resulting in a compilation error.
Scope of Identifiers
Identifiers in C++ are also subject to scope rules. An identifier declared in a specific scope (like within a function) is not accessible outside of it. Here's an example:
void myFunction() {
int localVariable = 5;
}
cout << localVariable; // Error: 'localVariable' was not declared in this scope
Here, `localVariable` is declared within `myFunction` and is inaccessible outside of that function, leading to an undeclared identifier error.
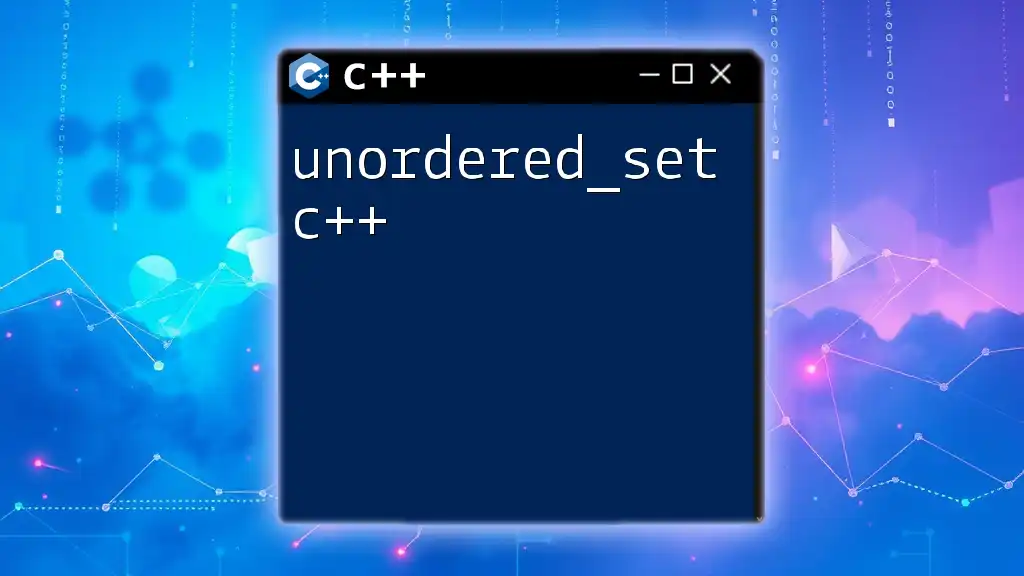
How to Fix Undeclared Identifier Errors
Fixing undeclared identifier errors often involves a few simple strategies that enhance code clarity and organization.
Checking for Typos and Spelling Mistakes
The first fix should always be to check your code for any typos or spelling mistakes. In large codebases, it's easy to overlook simple errors:
int myVariable = 10;
cout << myVariabl; // Fix: Correctly spell it
Changing `myVariabl` to `myVariable` resolves the error.
Declaring Variables Before Use
In C++, always declare your variables before any usage. Ensuring the correct order promotes better coding practices:
int myVariable = 10; // Declaration first
cout << myVariable; // Now it works!
This will avoid errors related to usage before declaration.
Understanding Scope and Lifetime
Comprehending how scope works in C++ helps you to prevent scope-related undeclared identifier issues. Ensure that your identifiers are declared in the correct scope to be accessible from where they are needed:
int globalVariable = 20; // Global scope
void myFunction() {
cout << globalVariable; // Accessible here
}
In the example above, `globalVariable` is accessible inside `myFunction` due to its global scope.
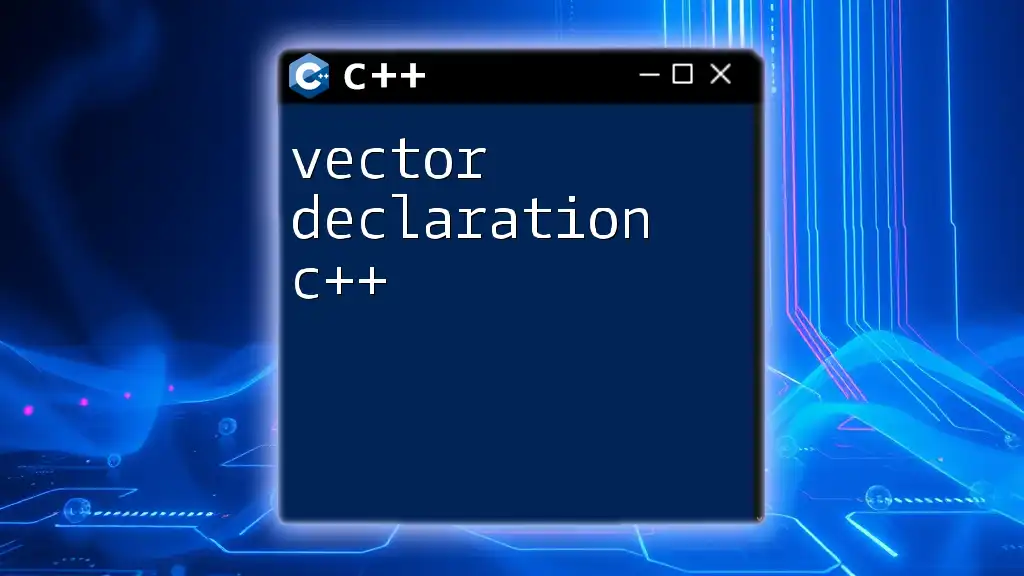
Best Practices to Avoid Undeclared Identifier Issues
To minimize the occurrence of undeclared identifier errors in your C++ code, implement some best practices that bolster code quality and readability.
Consistent Naming Conventions
Adhering to consistent naming conventions makes your code easier to read and reduces the risk of errors. By using descriptive and clear names, you can avoid confusion:
int age; // Good
int a; // Bad
Choosing meaningful names supports maintaining and understanding the code in the long run.
Code Organization and Structure
Effective code organization is essential for clarity and maintenance. Consider modular programming techniques by using header files and separating your implementation into different source files. This approach not only organizes your identifiers but also provides a cleaner compilation interface.
For example, declare your variables in header files:
// header.h
extern int myVariable; // Declaration
// main.cpp
#include "header.h"
int myVariable = 10; // Definition
This structure avoids potential compilation issues while dealing with numerous identifiers.
Utilizing IDE Features
Take full advantage of features provided by Integrated Development Environments (IDEs). Most modern IDEs come equipped with code autocompletion tools that help identify undeclared identifiers as you type, reducing errors significantly.
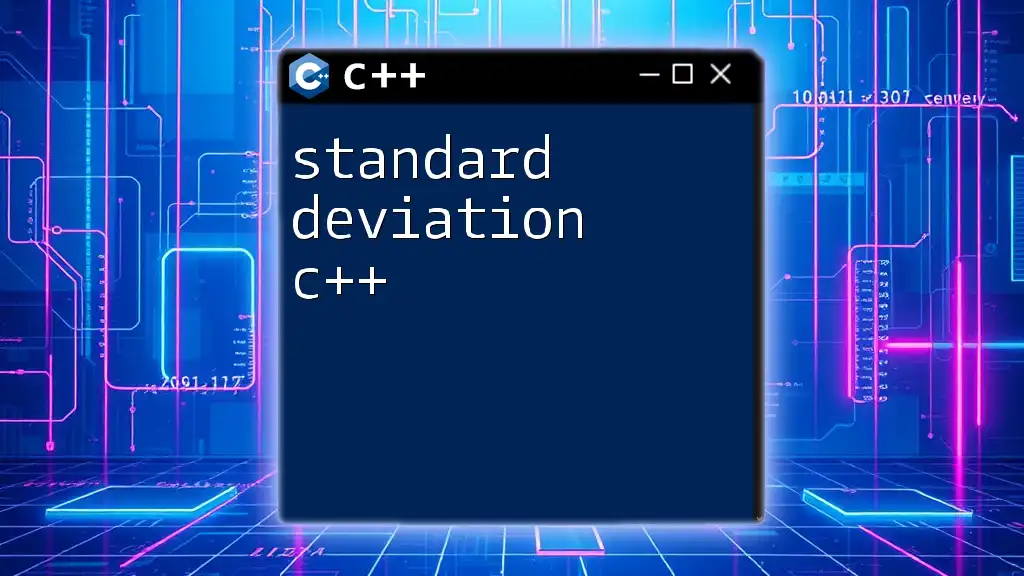
Tools and Resources for Troubleshooting
If you encounter persistence with undeclared identifiers, various tools are available to assist in troubleshooting and understanding C++.
Compiler Options and Flags
Utilize compiler options and flags to generate more informative error messages. For instance, using the flags `-Wall` and `-Werror` can help catch undeclared identifiers as warnings or errors during the compilation process:
g++ -Wall -Werror my_program.cpp
This way, you catch these issues promptly during development.
Online C++ Resources
You can find a wealth of information on online resources for troubleshooting C++ errors. Websites like Stack Overflow or cppreference.com offer community-driven solutions and detailed programming references.
C++ Best Practice Books and Tutorials
Investing time in C++ literature and tutorials can deepen your understanding and improve your programming skills. Books that focus on effective C++ practices can provide insights into common pitfalls and how to avoid them.
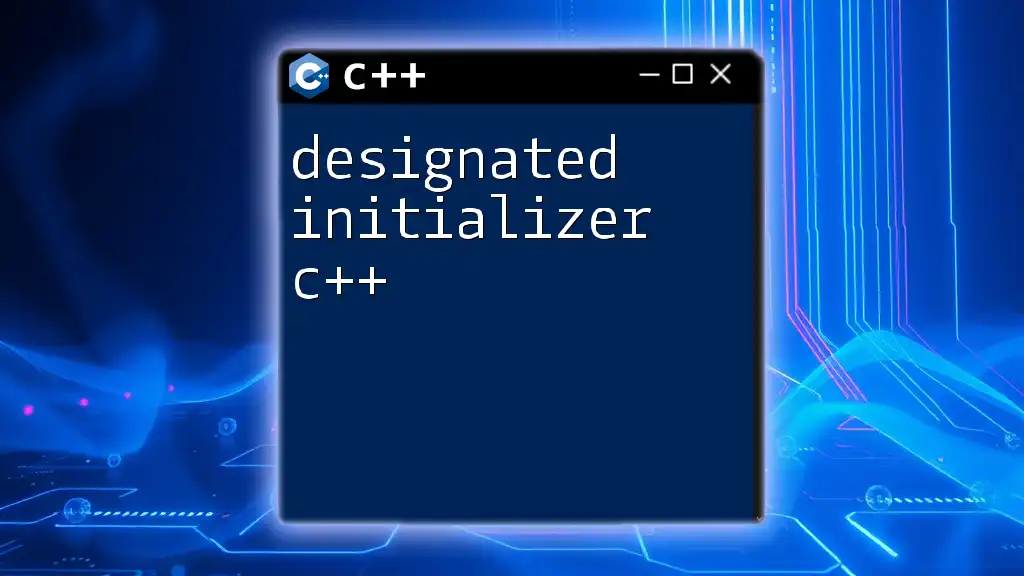
Conclusion
In summary, the use of undeclared identifiers in C++ can create frustrating obstacles during development. However, by understanding the compilation process, recognizing common causes, and implementing straightforward fixes, you can enhance your coding practices significantly.
Remember to adopt best practices that encourage clean, maintainable code, and utilize the available tools and resources to troubleshoot efficiently. With diligence and awareness, you can navigate the complexities of undeclared identifiers in C++ and write more robust code.