Java generally exhibits slower performance compared to C++ due to its managed runtime environment and garbage collection, while C++ allows for greater control over system resources and memory management, resulting in faster execution times.
#include <iostream>
using namespace std;
int main() {
for(int i = 0; i < 1000000; i++) {
// Perform some calculations
int square = i * i;
}
cout << "Calculation complete." << endl;
return 0;
}
Understanding Performance Metrics
What is Performance in Programming?
Performance in programming refers to how effectively a program utilizes system resources to execute tasks within a reasonable timeframe. Key metrics that define performance include execution speed, memory usage, and compilation time. Understanding how these factors affect your application can directly influence both user experience and system efficiency.
Why Speed Matters in Programming
In the fast-paced world of technology, the speed of an application can significantly impact its credibility and usability. Applications that have high performance lead to increased user satisfaction and overall effectiveness. In certain use cases—such as gaming, real-time systems, and high-frequency trading—performance becomes crucial. Here, milliseconds can mean the difference between success and failure.

C++ vs Java Performance
Overview of C++
C++ is an extension of the C programming language and is often viewed as a premier choice for developing performance-critical applications. Known for its low-level memory manipulation capabilities, C++ allows developers to manage memory directly (e.g., using pointers), giving them fine-grained control over system resources. This feature dramatically enhances performance when properly utilized, but it also places more responsibility on the developer.
Overview of Java
Java, on the other hand, emphasizes simplicity and portability over raw performance. It runs on the Java Virtual Machine (JVM), which allows applications to be platform-independent but adds a layer of abstraction that can slow down execution speed. Though Java employs garbage collection to manage memory automatically, this process can introduce latency in performance-sensitive applications.

Java Performance vs C++
Execution Speed
Comparing Runtime Efficiency
When it comes to runtime efficiency, C++ typically outperforms Java. This is fundamentally due to the fact that C++ compiles directly to native machine code, while Java is compiled into bytecode that runs on the JVM. Thus, C++ programs tend to execute faster. Nevertheless, the extent of this speed advantage can vary based on the application.
To illustrate the difference, consider the following implementations of the Fibonacci sequence:
// Example: Fibonacci in C++
int fibonacci(int n) {
return (n <= 1) ? n : fibonacci(n - 1) + fibonacci(n - 2);
}
// Example: Fibonacci in Java
public class Fibonacci {
public static int fibonacci(int n) {
return (n <= 1) ? n : fibonacci(n - 1) + fibonacci(n - 2);
}
}
In a benchmark test, the C++ implementation would likely run significantly faster than its Java counterpart due to the overhead of the JVM.
Memory Management
C++ Manual Management vs Java Garbage Collection
C++ offers precise control over memory management through manual allocation and deallocation. Developers use `new` to allocate memory and `delete` to free it:
int* arr = new int[10]; // Allocating an array of 10 integers
delete[] arr; // Deallocating the array
While this control can enhance performance, it also has risks, such as memory leaks and pointer errors. Developers need to maintain diligent oversight of memory use.
In contrast, Java employs garbage collection to reclaim memory automatically. This convenience can reduce the likelihood of memory leaks and segfaults; however, garbage collection can introduce unpredictable latency due to the background processes that identify and reclaim unused memory. In performance-critical applications, this could lead to noticeable slowdowns.
Compilation Time
C++ Compilation vs Java Bytecode
C++ is a compiled language that generates machine code directly, which can take time but optimizes execution speed at runtime. The trade-off is that longer compilation times could delay iterations during development.
Java, meanwhile, compiles to bytecode, which is processed by the JVM. This typically results in faster compile times but may include additional overhead during execution as the JVM interprets the bytecode. As a result, the compilation process influences not just performance, but the overall development workflow.

C++ vs Java Speed in Real-World Applications
Game Development
C++ is often the language of choice for game development, primarily because of its superior performance. Many popular game engines, including Unreal Engine, are built in C++, enabling developers to maximize performance and minimize latency experiences. In a performance-intensive environment such as gaming, the manual memory management and low-level capabilities of C++ can lead to more responsive and visually appealing experiences.
Enterprise Applications
Java shines in enterprise application development. Its rich set of libraries and frameworks provides developers with the tools needed to build scalable applications efficiently. While Java may not match the raw execution speed of C++, it offers other advantages, such as ease of maintenance and robust community support. Many enterprise solutions opt for Java's performance, which, while slower than C++, is often more than sufficient for business needs.
Mobile Applications
In mobile development, the choice between C++ and Java often hinges on the specific requirements of the application. For Android applications, Java is commonly used due to its seamless integration with Android SDKs. However, performance-sensitive applications may benefit from using C++ for computationally intensive tasks, such as graphics rendering, allowing developers to strike a balance between performance and convenience.

Tools and Techniques for Measuring Performance
Benchmarking Tools for C++
To effectively measure and compare the performance of C++ applications, developers can utilize benchmarking tools like Google Benchmark. This library provides an easy way to run performance tests and analyze the execution time of specific code segments.
Benchmarking Tools for Java
For Java, the JMH (Java Microbenchmark Harness) is the go-to tool for measuring performance. It helps in writing accurate and reliable benchmarks, enabling developers to capture nuances in execution speed comprehensively.
Integrated Performance Metrics
When measuring performance, it's crucial to establish a common ground for results between C++ and Java. Each language has distinct profiling and benchmarking tools, but understanding the various metrics and how they interact can facilitate more effective comparisons.
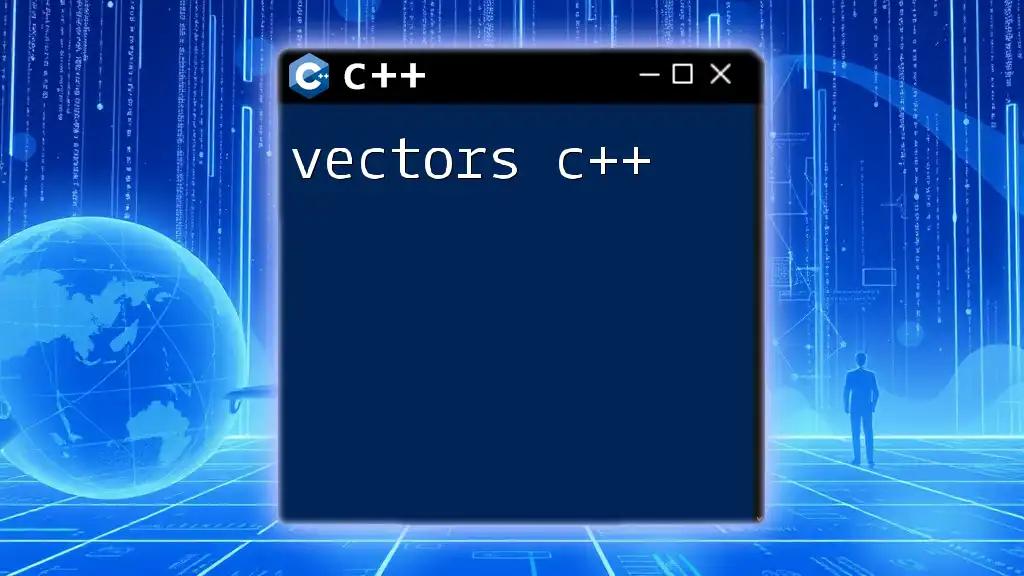
Case Studies
High-Performance Computing
C++ is widely utilized in high-performance computing (HPC) scenarios. For example, many scientific simulations and complex computations rely on C++'s performance advantages. By utilizing multi-threading alongside manual memory management, C++ can significantly outperform high-level languages like Java in these scenarios, leading to faster processing times and enhanced computational capabilities.
Web Applications
Java dominates the web application space, especially in enterprise settings where scalability and maintainability are crucial. Many large-scale systems have been built using Java, and while performance may lag compared to C++, the trade-offs in development speed, library access, and ease of maintenance can often outweigh the disadvantages, particularly when optimized correctly.

Conclusion
In the ongoing debate of Java speed vs C++, it is evident that each language has its strengths and limitations. C++ generally offers superior execution speed due to direct compilation into native code and manual memory management capabilities. On the other hand, Java provides ease of use and considerable support for enterprise-level applications at the cost of some performance.
Ultimately, the choice between C++ and Java should depend on the specific requirements of the project. Understanding the nuances of each language's performance characteristics can help developers make informed decisions that align with their goals. Whether you prioritize speed or prefer a more developer-friendly environment, familiarity with these two programming powerhouses will undoubtedly benefit your programming journey.

Additional Resources
To expand your knowledge further, consider diving into specialized readings, participating in online communities, and exploring additional benchmarking tools tailored to your needs in C++ and Java. Understanding performance is a continuous journey, and the more informed you are, the better equipped you'll be to harness the potential of these powerful programming languages.