Java is a high-level, platform-independent programming language known for its object-oriented features, while C++ is a powerful, compiled language that allows for low-level memory manipulation and performance optimization.
Here’s a simple code snippet demonstrating “Hello, World!” in both languages:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of Java and C++
What is Java?
Java is an object-oriented programming language developed by Sun Microsystems in the mid-1990s. Its key characteristics include:
- Platform Independence: Java programs can run on any device that has the Java Virtual Machine (JVM) installed, offering remarkable portability across different systems.
- Robustness: Java's strict compile-time and runtime checking helps minimize errors, making it a reliable choice for large-scale applications.
- Large Standard Library: Java has a rich set of libraries and frameworks that streamline development, especially for web and enterprise applications.
With its ubiquity, Java is widely used in industries ranging from finance and insurance to enterprise-level applications, making it one of the most popular programming languages today.
What is C++?
C++ is a powerful, high-performance programming language that extends the C language with object-oriented features. Key characteristics of C++ include:
- Low-Level Manipulation: C++ allows for low-level memory manipulation, which can lead to increased performance in resource-intensive applications.
- Multi-Paradigm: While primarily an object-oriented language, C++ also supports procedural and functional programming paradigms, giving developers the flexibility to choose their approach.
- Rich Standard Library: C++ provides a Standard Template Library (STL) that offers ready-to-use classes for data structures and algorithms, enhancing productivity.
C++ is widely used in system/software development, game development, and applications where performance is crucial.
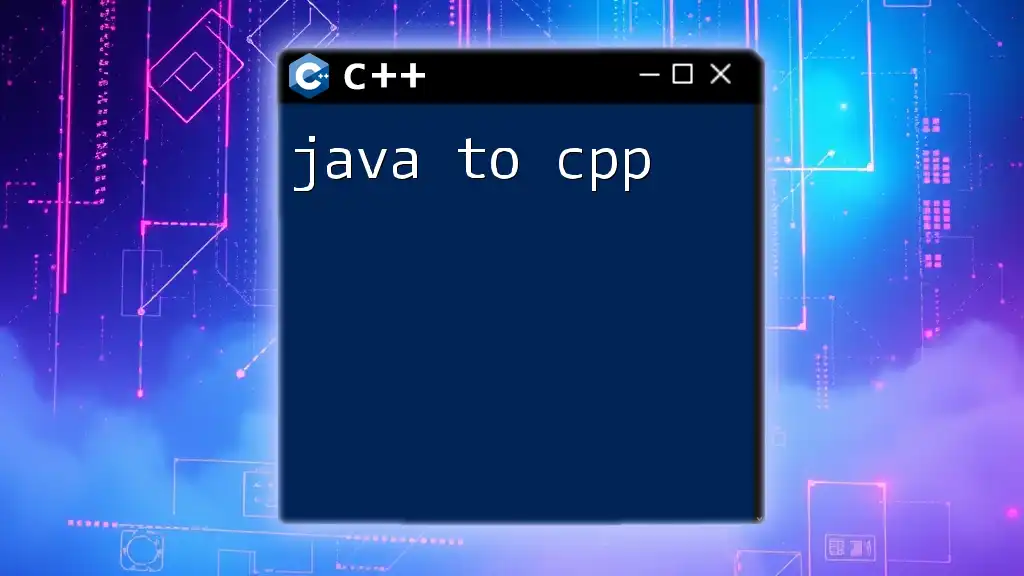
Fundamental Differences Between Java and C++
Memory Management
One of the most significant differences in the memory management of Java and C++ lies in their approaches:
-
Garbage Collection in Java: Java utilizes a garbage collector that automatically deallocates memory that is no longer in use, simplifying memory management for developers. This means less risk of memory leaks, but it can introduce unpredictability in performance due to garbage collection cycles.
// Java Memory Allocation String example = new String("Hello, World!");
-
Manual Memory Management in C++: In C++, developers are responsible for allocating and deallocating memory using operators such as `new` and `delete`. Although this offers more control, it also demands careful management to avoid memory leaks.
// C++ Memory Allocation std::string* example = new std::string("Hello, World!"); delete example; // Manual cleanup
Object-Oriented Programming
Both languages support object-oriented programming, but they approach it differently:
-
Java's Strict Object Orientation: Everything in Java is an object, even primitive types are wrapped as objects (e.g., `Integer`, `Double`). This enforces the use of object-oriented principles throughout the code.
-
C++’s Flexibility: C++ allows both procedural and object-oriented programming. Developers can write functions that aren't associated with any class, offering greater flexibility in their coding practices.
// Java Class Example public class Example { public void display() { System.out.println("Hello, Java!"); } }
// C++ Class Example class Example { public: void display() { std::cout << "Hello, C++!" << std::endl; } };
Syntax and Language Structure
While both languages share some syntax due to C roots, notable differences exist:
-
Braces and Semicolons: Both languages use braces `{}` to enclose blocks of code, and statements in both languages typically end with a semicolon `;`.
-
Variable Declaration and Initialization: In Java, variable types must be declared explicitly, while C++ allows for a more flexible approach using `auto` for type inference.
// Java Variable Declaration int number = 5;
// C++ Variable Declaration auto number = 5; // Type inferred as int
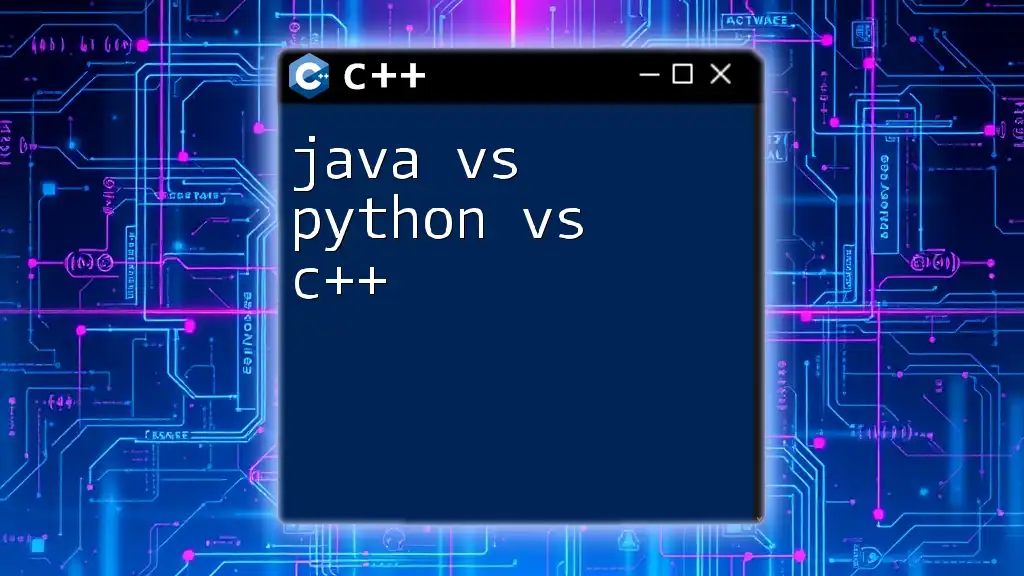
Performance and Efficiency
Execution Speed
One of the critical considerations in the java vs cpp debate is execution speed:
-
Compiled vs Interpreted: Java is compiled into bytecode that runs on the JVM, which can introduce overhead during execution. This can lead to slower performance when compared to C++, which compiles directly to machine code.
-
Performance Benchmarks: In numerous performance benchmarks, C++ often outperforms Java in CPU-bound tasks due to its more efficient memory management and lower abstraction levels.
Resource Optimization
C++ provides robust tools for optimizing performance:
-
Fine-grained Control: C++ allows developers to control hardware-level functionalities, enabling optimizations that can significantly improve resource usage.
-
Example: Resource allocation can be achieved using smart pointers in C++, which automate memory management while ensuring efficiency.
// Smart Pointer Example std::unique_ptr<std::string> smartPointer = std::make_unique<std::string>("Hello, Smart Pointer!");
In contrast, Java abstracts away many low-level functionalities, which can lead to simpler but less optimized applications.
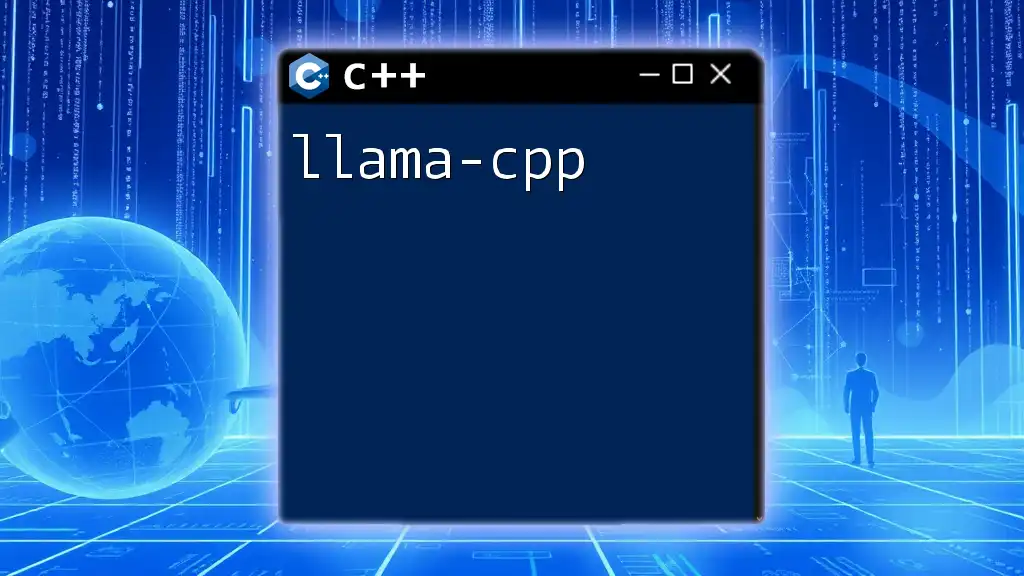
Exception Handling
Error Management Techniques
Exception handling methodologies differ significantly between Java and C++:
-
Java's Try-Catch Blocks: Java provides a structured way to handle exceptions through try-catch blocks, and all checked exceptions must be either caught or declared to be thrown.
// Java Exception Handling try { int result = 10 / 0; } catch (ArithmeticException e) { System.out.println("Division by zero error."); }
-
Error Handling in C++: C++ also uses exceptions, but developers can use alternative methods, such as return codes or assertions, providing more flexibility but also potentially introducing risk if error conditions are overlooked.
// C++ Exception Handling try { throw std::runtime_error("This is an error."); } catch (const std::exception& e) { std::cout << e.what() << std::endl; }
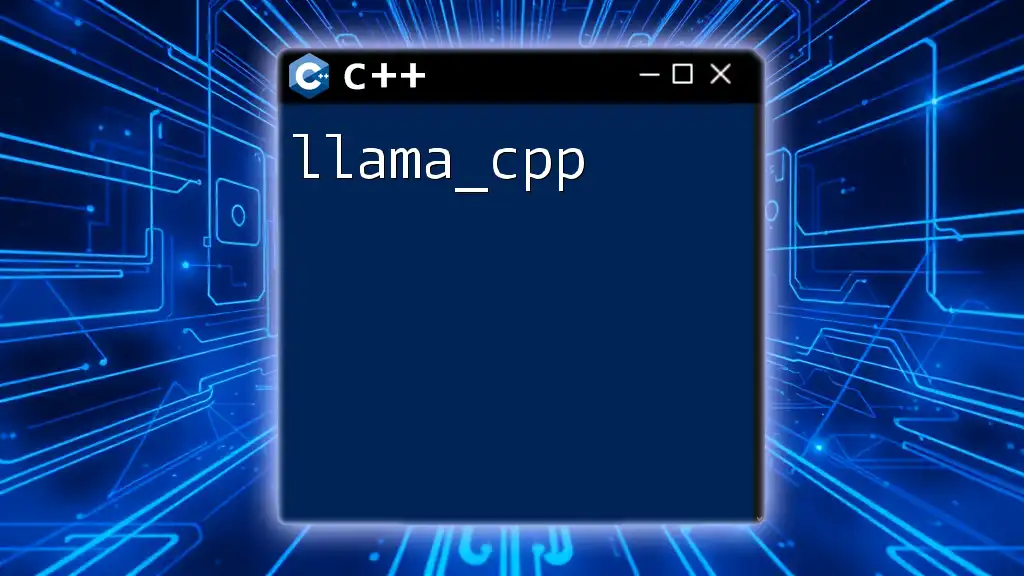
Typing System
Static vs Dynamic Typing
The typing systems of Java and C++ present significant differences:
-
Static Typing in C++: C++ is statically typed, meaning types are checked at compile time, which can lead to increased performance but also requires careful management by the developer.
-
Dynamic Typing in Java: Java utilizes dynamic typing for its objects, allowing for greater flexibility but also making it less performant in certain scenarios.
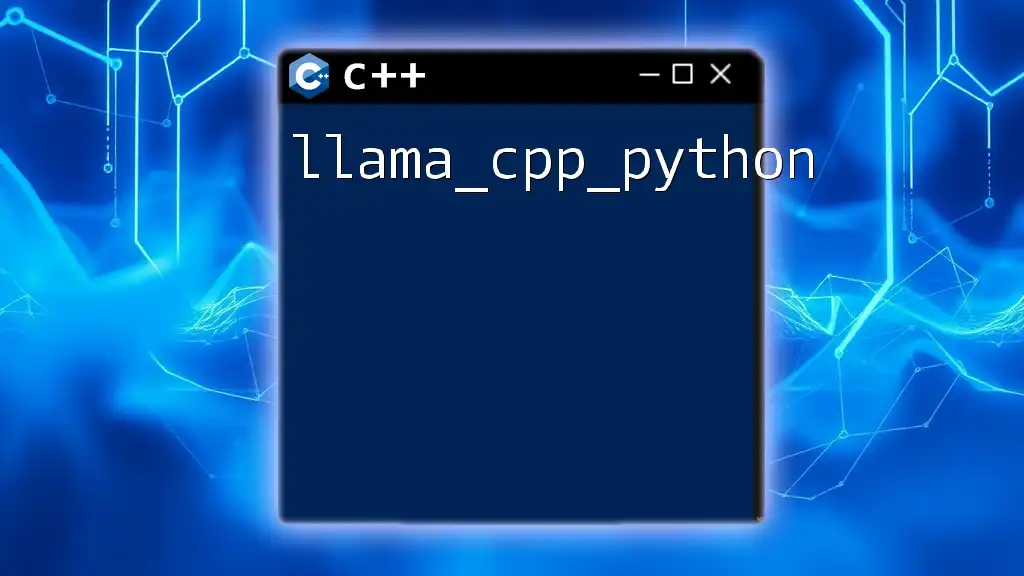
Use Cases and Applications
When to Use Java
Java excels in several areas:
- Enterprise Applications: Java is the go-to language for large systems that require scalability, transactional support, and reliability.
- Mobile Applications: With Android being Java-based, it is indispensable for mobile app development.
When to Use C++
C++ is preferred in scenarios where performance is paramount:
- Game Development: Game engines often utilize C++ for its high performance and resource management capabilities.
- System-Level Programming: Operating systems and embedded systems frequently employ C++ for its granular control over hardware.
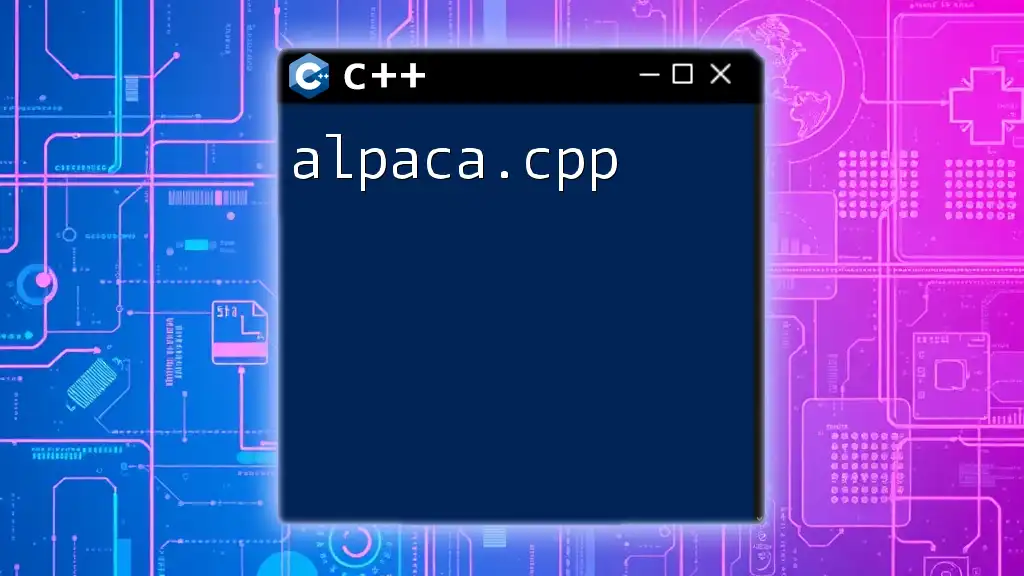
Community and Ecosystem
Development Tools
Both languages boast excellent development environments:
- Popular IDEs for Java: IntelliJ IDEA, Eclipse, and NetBeans are widely used.
- Popular IDEs for C++: Visual Studio, Code::Blocks, and CLion are favored among C++ developers.
Library Support
The availability of libraries enhances productivity in both languages:
- Notable Java Libraries: Frameworks like Spring and Hibernate streamline enterprise development and data handling.
- Notable C++ Libraries: STL and Boost provide robust tools for data structures, algorithms, and more.
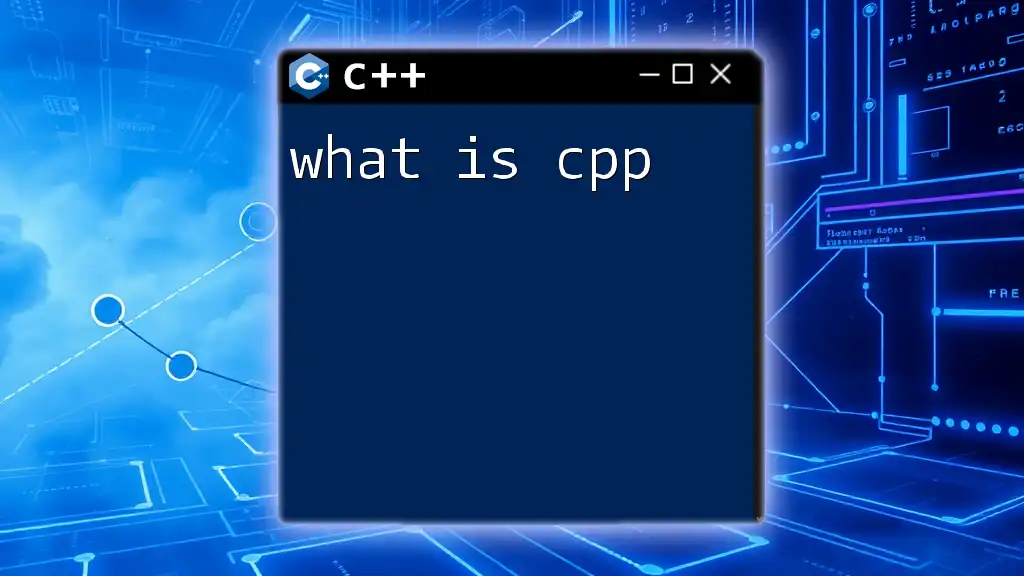
Learning Curve
Ease of Learning
The learning curve varies considerably between the two:
- Java's Simplicity: Due to built-in memory management and a straightforward syntax, beginners may find Java easier to grasp.
- C++ Complexity: Advanced features such as pointers, multiple inheritance, and templates can present challenges for newcomers.
Resources Available
Both languages offer a wealth of resources:
- Books, online courses, and programming communities are abundant for both Java and C++, making it easier for developers to learn and grow.
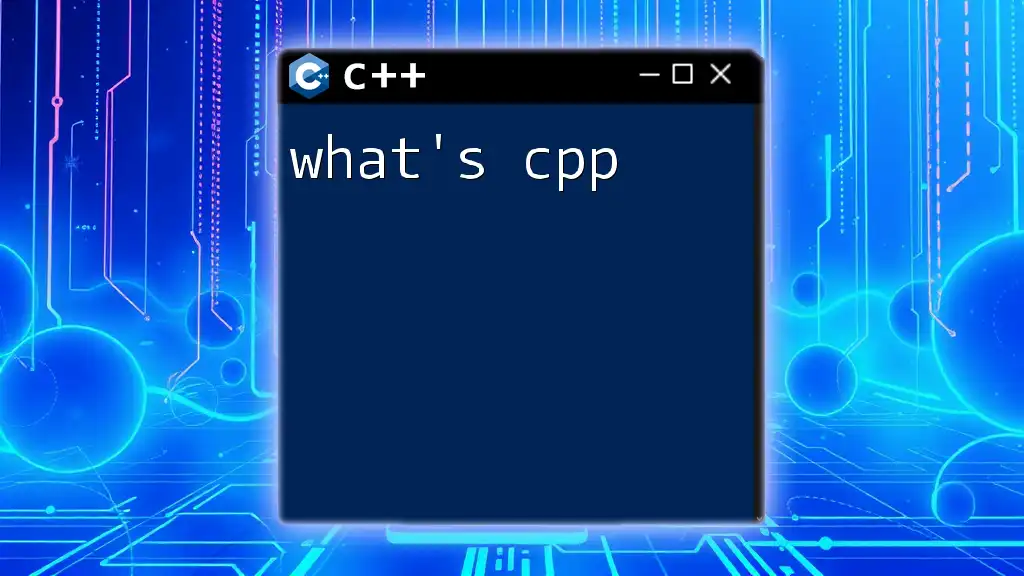
Conclusion
Understanding the nuances of java vs cpp is crucial for developers choosing the right tool for their programming needs. Java offers robustness, ease of use, and a rich ecosystem for enterprise applications, while C++ provides superior performance and low-level control, making it suitable for resource-intensive tasks.
Each language shines in its domain, and the choice ultimately depends on the specific requirements of the project at hand. Exploring both languages can significantly enhance one's skill set and versatility as a developer.
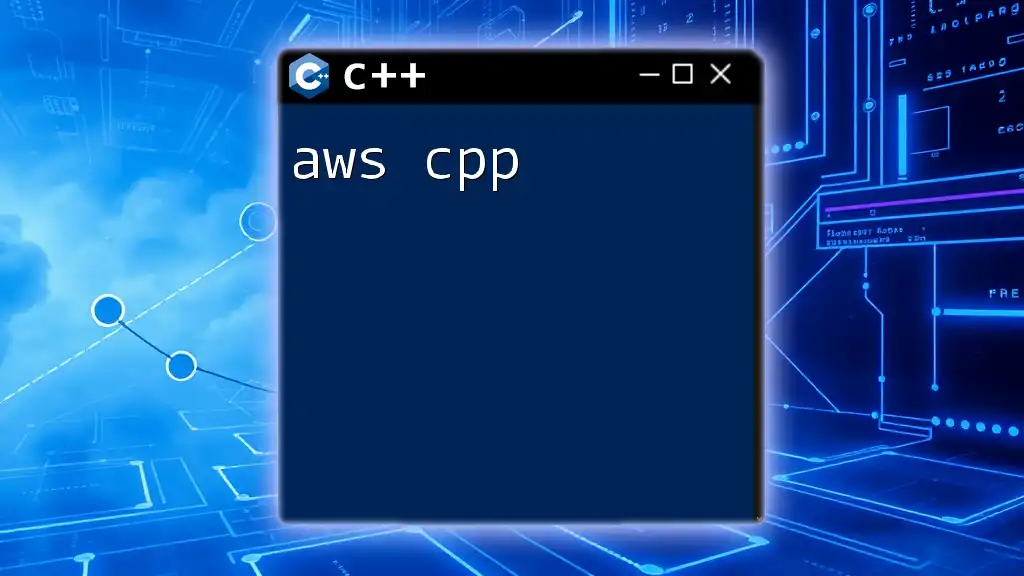
Call to Action
We invite you to share your experiences or preferences between Java and C++. Follow our company for more tips and resources on mastering C++ commands and improving your programming skills!