C++ 2008 refers to the C++ programming language standard finalized in 2008, introducing features that enhance performance and usability, such as improved type inference and the addition of the `nullptr` keyword for null pointer representation.
Here's a simple code snippet demonstrating the use of `nullptr`:
#include <iostream>
int main() {
int* ptr = nullptr; // Use nullptr instead of NULL
if (ptr == nullptr) {
std::cout << "Pointer is null." << std::endl;
}
return 0;
}
Key Features of C++ 2008
New Language Features
Range-based For Loops have transformed how we iterate over collections. No longer must we deal with traditional for loops and iterators; the new syntax simplifies code for increased readability and brevity.
Example using a range-based for loop:
std::vector<int> nums = {1, 2, 3, 4, 5};
for (int num : nums) {
std::cout << num << " ";
}
In this example, `num` will take on each element in `nums`, making it easy to read and reducing the chances of off-by-one errors.
Lambda Expressions offer a way to define anonymous functions directly within your code. This improves code modularity and can be particularly useful for tasks such as sorting or filtering data.
Example of using lambda expressions:
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Output: 8
In this case, the lambda function `add` can be invoked in-line, streamlining operations without needing separate function definitions.
Improved Standard Library
One of the notable enhancements in C++ 2008 includes Introduction of New Libraries. These libraries, such as those for handling regular expressions, provide versatile tools for text processing, making tasks easier and more efficient.
Regular Expressions allow programmers to search, match, and manipulate strings with ease. By using regex in C++, you can significantly reduce the complexity often involved in string handling.
Example of using regular expressions:
#include <regex>
std::regex regex("a.b");
std::string str = "acb";
std::cout << std::regex_match(str, regex); // Output: 1 (true)
This example checks if a string matches the specified pattern, where the dot `.` acts as a wildcard, greatly simplifying string validation processes.
Type Traits and Smart Pointers
Type Traits are an essential feature, allowing programmers to inspect and modify the properties of types at compile-time. They pave the way for more type-safe programming and eliminate several potential run-time errors.
Smart Pointers replace traditional pointers in C++ and provide automatic memory management. This enhances stability and efficiency by reducing memory leaks and dangling pointers.
Example using `std::unique_ptr`:
std::unique_ptr<int> ptr(new int(5));
std::cout << *ptr; // Output: 5
In this snippet, `std::unique_ptr` manages the dynamically allocated integer, ensuring that the memory is released automatically when it goes out of scope. This is an effective practice for resource management in modern C++.
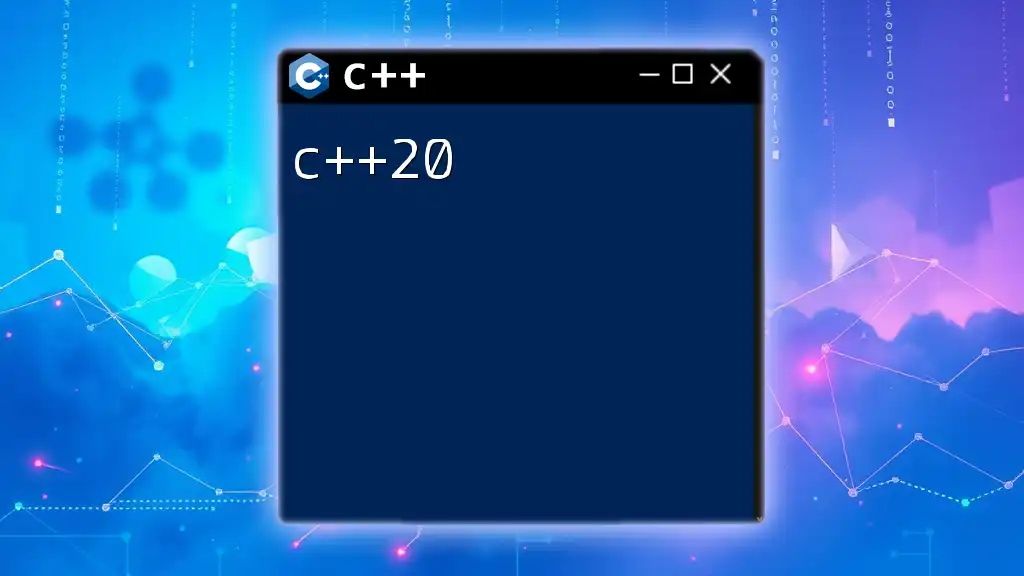
Visual C++ 2008
Overview of Visual C++ 2008
Visual C++ 2008 is an integrated development environment (IDE) designed for C++ developers. It offers a comprehensive set of tools that streamline the coding, debugging, and deployment processes. The IDE supports the latest features introduced in C++ 2008, allowing developers to make full use of the enhancements.
Installing Visual C++ 2008
To start developing with Visual C++ 2008, you need to follow a few straightforward steps:
- Downloading the Installer: Visit Microsoft’s official website or trusted sources to get the Visual C++ 2008 installer.
- Configuration Settings During Installation: Select the components and configurations you wish to include, such as desktop development features.
- Setting Up Environment Variables: After installation, configure your environment variables for optimal development experience.
Using Visual C++ 2008 Effectively
Setting Up Your First Project is straightforward in Visual C++ 2008. Start by creating a new project, selecting the type of application (console, GUI, etc.), and exploring the available templates.
One significant aspect of Exploring IDE Features is understanding the built-in editor, which offers features such as syntax highlighting, auto-completion, and error detection. Additionally, powerful debugging tools enable step-by-step execution to track down issues.
To enhance your productivity, consider implementing Tips and Best Practices:
- Utilize templates for generic programming to minimize code duplication.
- Employ namespaces diligently to avoid naming conflicts, particularly in larger projects.
- Implement robust error handling mechanisms to manage exceptions effectively.
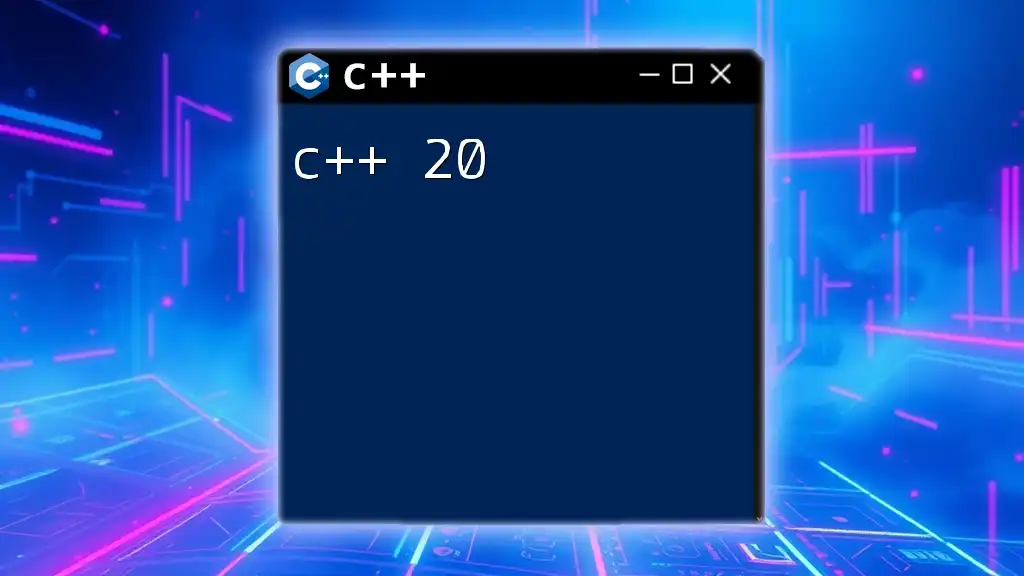
Visual C++ Redistributable 2008
What is Visual C++ Redistributable 2008?
The Visual C++ Redistributable 2008 is an essential package that provides a set of runtime components necessary for applications built with Visual C++ 2008. It ensures that end-users can run these applications without needing to install the entire Visual Studio.
Common Issues with Redistributable
While the redistributable is designed to work seamlessly, it’s not uncommon to encounter some challenges:
- Debugging and Troubleshooting: Applications may fail to launch due to missing redistributable files. Error messages often guide users to the installation.
- Maintaining Libraries: Regularly check for updates to avoid security vulnerabilities and compatibility issues, and understand how to rollback in case of problems.
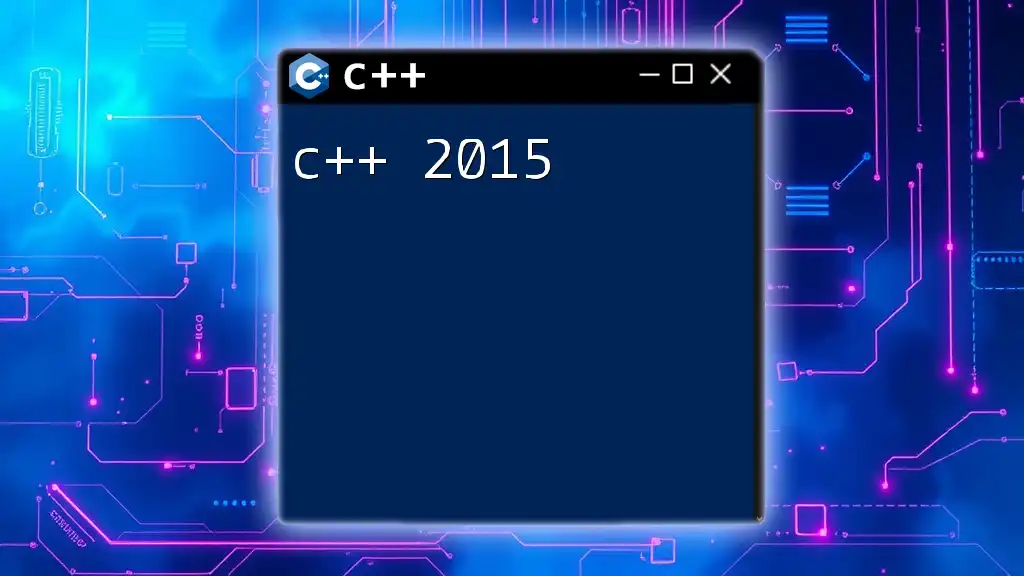
Conclusion
C++ 2008 has substantially impacted software development by introducing features that enhance code clarity, efficiency, and safety. With the improvements in the standard library and the arrival of Visual C++ 2008, developers are better equipped to create robust applications.
The future of C++ remains bright, continuously evolving beyond 2008 to include even more features and improvements. As you embark on your C++ programming journey, know that there are numerous resources available to help you master this powerful language. Embrace these advancements, and you’ll unlock new possibilities in your development work.
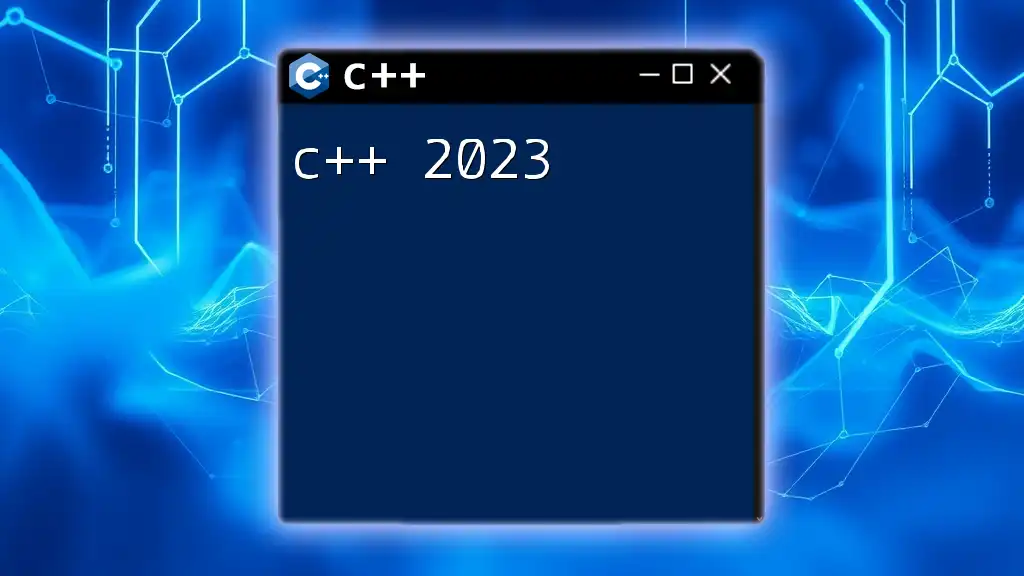
Additional Resources
For further enrichment and learning, consider exploring books, articles, and communities that delve deeper into C++ programming. Engaging with peers through online forums can foster knowledge sharing and support as you navigate the C++ landscape.