In C++, logical operators allow you to combine multiple boolean expressions to evaluate conditions more effectively, with `&&` (logical AND) and `||` (logical OR) being the most commonly used operators.
Here’s a simple example demonstrating the logical AND operator:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a && b) {
cout << "Both conditions are true." << endl;
} else {
cout << "At least one condition is false." << endl;
}
return 0;
}
What is Logic in Programming?
Logic serves as the cornerstone of programming, allowing developers to make decisions and control the flow of their applications. It involves reasoning patterns that let programmers evaluate conditions and execute specific actions based on those evaluations. Mastering logic is essential for effective programming in any language, and C++ is no exception.
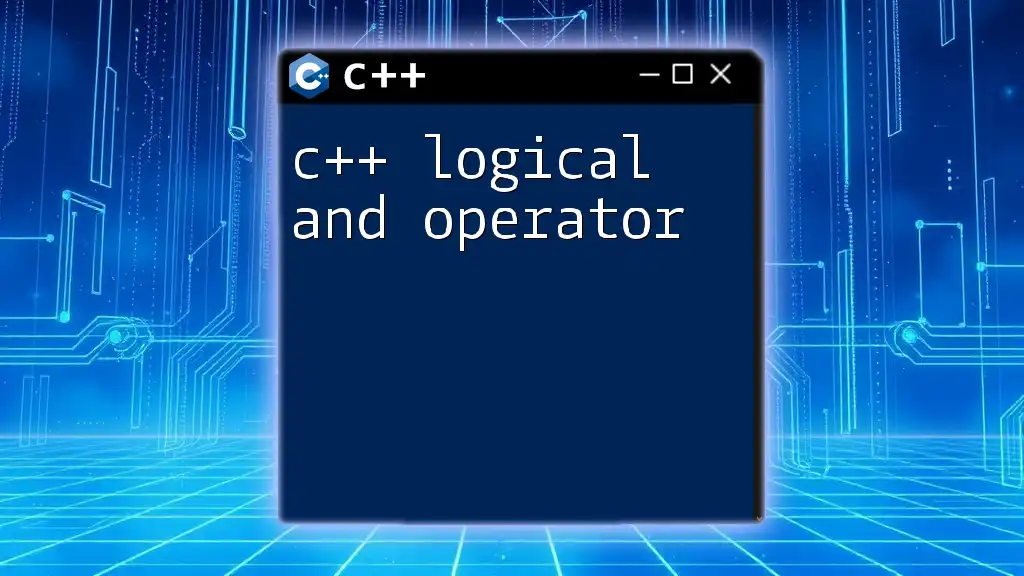
Overview of C++ Logical Operators
In C++, logical operators are fundamental tools used to perform logical operations on boolean values. The primary logical operators in C++ include AND, OR, and NOT. Understanding these operators is crucial for implementing complex decision-making constructs within your code.
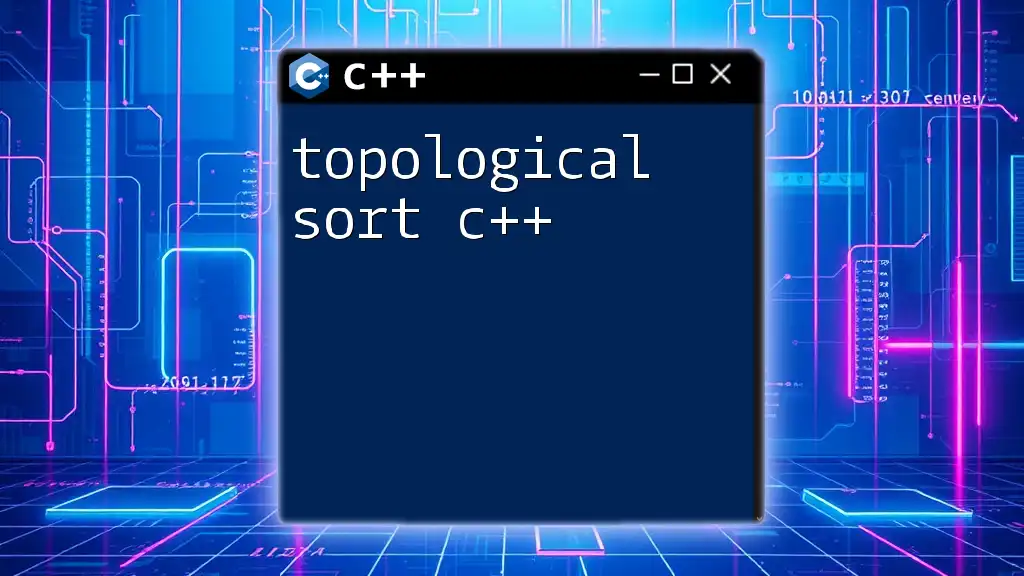
Understanding Logical Operators in C++
What Are Logical Operators in C++?
Logical operators are used to create expressions that evaluate to either true or false. In C++, these operators play a significant role in control structures such as `if` statements, loops, and even in complex algorithms where multiple conditions must be evaluated simultaneously.
Types of Logical Operators in C++
C++ Logical AND (&&)
Explanation of Logical AND
The AND operator (`&&`) returns `true` only if both of its operands are true. This means that if either operand is false, the entire expression evaluates to false. Its functionality can be illustrated with a truth table:
A | B | A && B |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
Usage in C++
To use the AND operator in C++, consider the following syntax:
if (a > 0 && b > 0) {
// This block is executed only if both a and b are greater than 0
}
In this snippet, the conditional block executes only when both conditions are satisfied.
C++ Logical OR (||)
Explanation of Logical OR
The OR operator (`||`) returns `true` if at least one of its operands is true. If both operands are false, only then does the expression evaluate to false. The corresponding truth table looks like this:
| A | B | A || B | |-------|-------|--------| | true | true | true | | true | false | true | | false | true | true | | false | false | false |
Usage in C++
The OR operator can be utilized in C++ as follows:
if (a > 0 || b > 0) {
// This block is executed if either a or b is greater than 0
}
Here, the block executes if at least one of the conditions holds true.
C++ Logical NOT (!)
Explanation of Logical NOT
The NOT operator (`!`) negates the boolean value of its operand. If the operand is true, the NOT operator makes it false, and vice versa. The truth table for the NOT operator is simple:
A | !A |
---|---|
true | false |
false | true |
Usage in C++
You can use the NOT operator in your conditional statements like so:
if (!(a > 0)) {
// This block is executed if a is NOT greater than 0
}
This example demonstrates how the NOT operator is leveraged to invert the outcome of a boolean expression.
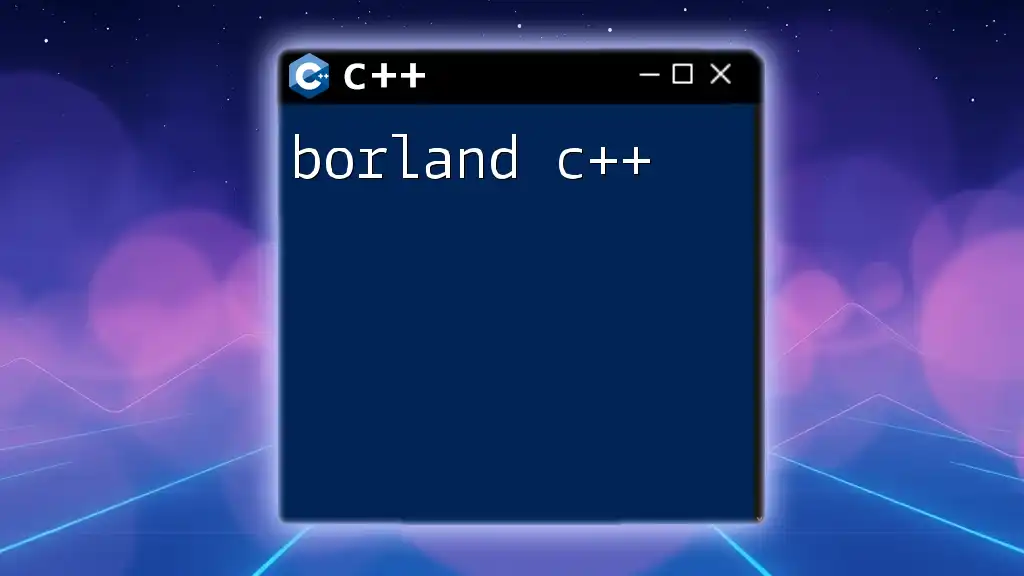
Combining Logical Operators in C++
C++ Logical Expressions
Logical operators can be combined to form complex expressions. When used together, the outcome is determined based on the rules of boolean algebra. For example:
if ((a > 0 && b > 0) || (c != 0)) {
// This block executes if both a and b are greater than 0,
// or if c is not equal to 0
}
In this complex expression, the program evaluates conditions for `a` and `b` and includes an additional check for `c`.
Operator Precedence
Operator precedence defines the order in which parts of an expression are evaluated. Logical operators have a specific precedence that determines how expressions are interpreted. Using parentheses can help solidify the intended logic and improve readability:
if ((a > 0 || b < 0) && c == 5) {
// The conditions are grouped for clarity
}
This ensures that the correct logical evaluation occurs, emphasizing the importance of clear coding practices.
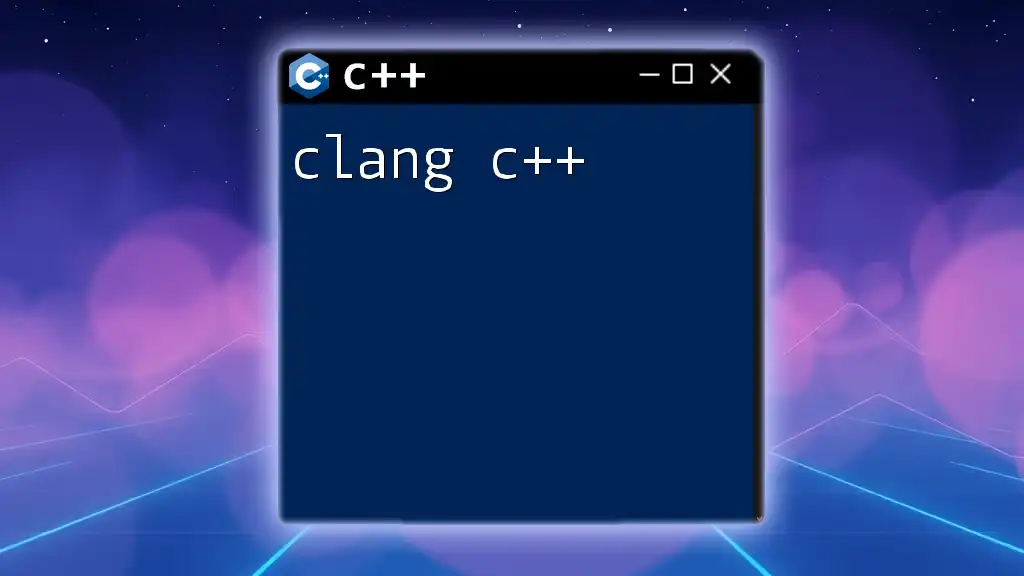
Practical Applications of Logical Operators
Decision Making in C++
Logical operators are instrumental in decision-making processes within C++. They allow programmers to create robust control structures. For example, using logical operators in a `while` loop can maintain conditions over multiple iterations:
while (a < 10 && b < 10) {
// Continue looping as long as both a and b are below 10
}
Here, the loop executes as long as both conditions remain true, making it essential for flow control.
Real-World Examples
Form Validation
Logical operators can be essential in form validation scenarios. Consider a login verification system:
if (username == "admin" && password == "1234") {
// Allow access to the admin panel
}
In this case, both conditions need to be true for the user to gain access to the administration section.
Flag Checks in Games
In game development, logical operators evaluate multiple game states efficiently. For example:
if (player.alive && player.hasKey || player.isAdmin) {
// Allow access to the restricted area
}
This code snippet demonstrates how logical operators check whether a player is alive, has a key, or is an admin, thereby granting access based on multiple conditions.
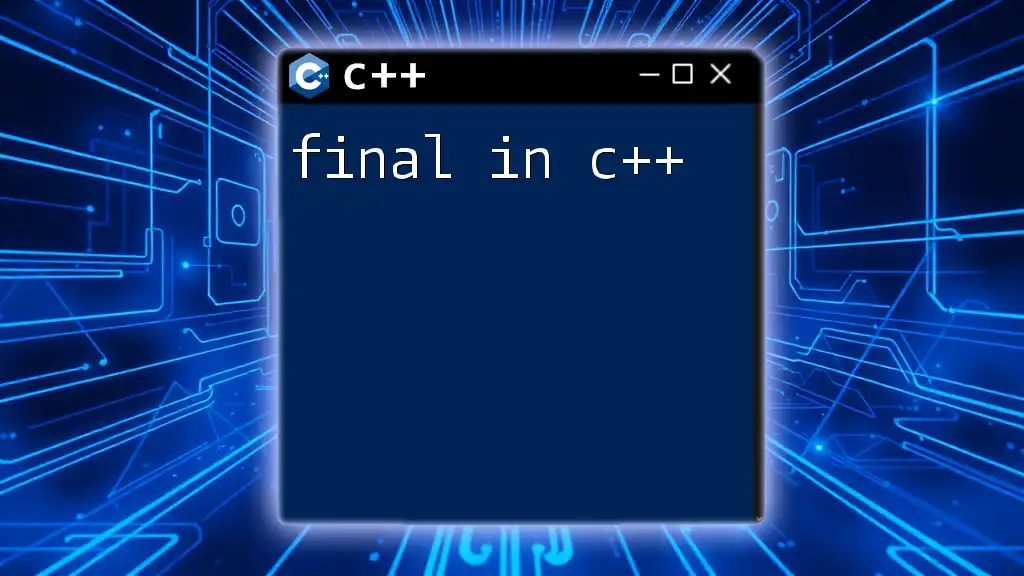
Conclusion
Understanding C++ logical operators is crucial for effective programming. Logical operators enable developers to create sophisticated conditions that dictate the flow of applications. By mastering logical AND, OR, and NOT, you empower your programming capabilities to handle complex logic in real-world scenarios. Practice using these operators through various coding exercises, and take your C++ skills to the next level!
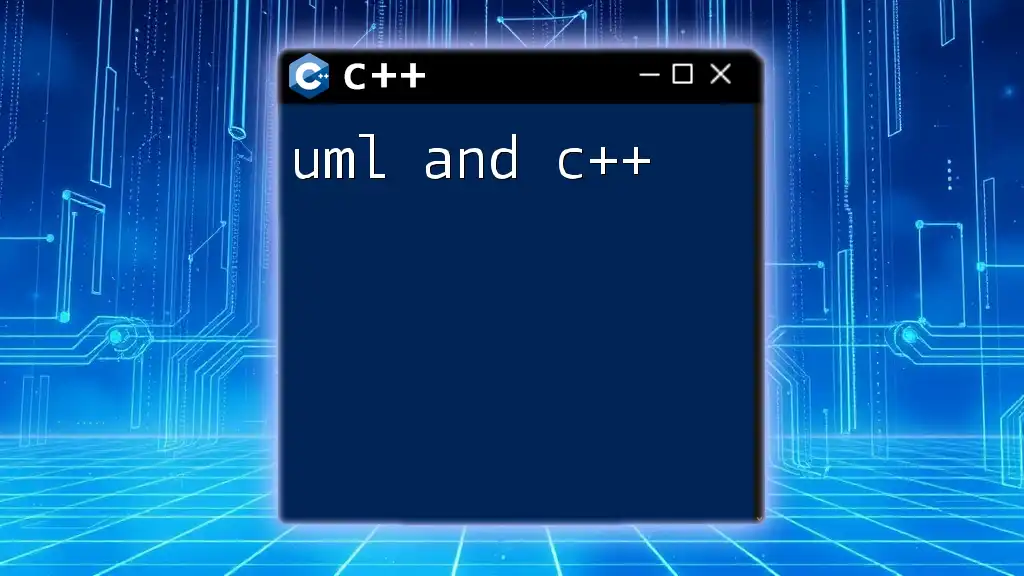
Additional Resources
For further learning, consider exploring C++ programming books, online tutorials, and coding platforms that offer practice exercises focused on logical operations and programming logic.