In C++, the `#include <queue>` directive is necessary to use the `priority_queue` container, which allows for storage of elements in a way that always retrieves the largest (or smallest) element first, based on specific comparison criteria.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> pq;
pq.push(10);
pq.push(5);
pq.push(15);
while (!pq.empty()) {
std::cout << pq.top() << " "; // Outputs: 15 10 5
pq.pop();
}
return 0;
}
Setting Up Your C++ Environment
Before diving into the intricacies of priority queues, it’s essential to have a proper C++ development setup. To get started, you will need a C++ compiler. Some of the recommended compilers include:
- Windows: MinGW, Visual Studio
- macOS: Xcode, Homebrew
- Linux: g++, available on most distributions
Once your compiler is ready, you need to include the right libraries. For working with priority queues in C++, the essential header file is `<queue>`. You would typically include it at the top of your code as shown below:
#include <queue>
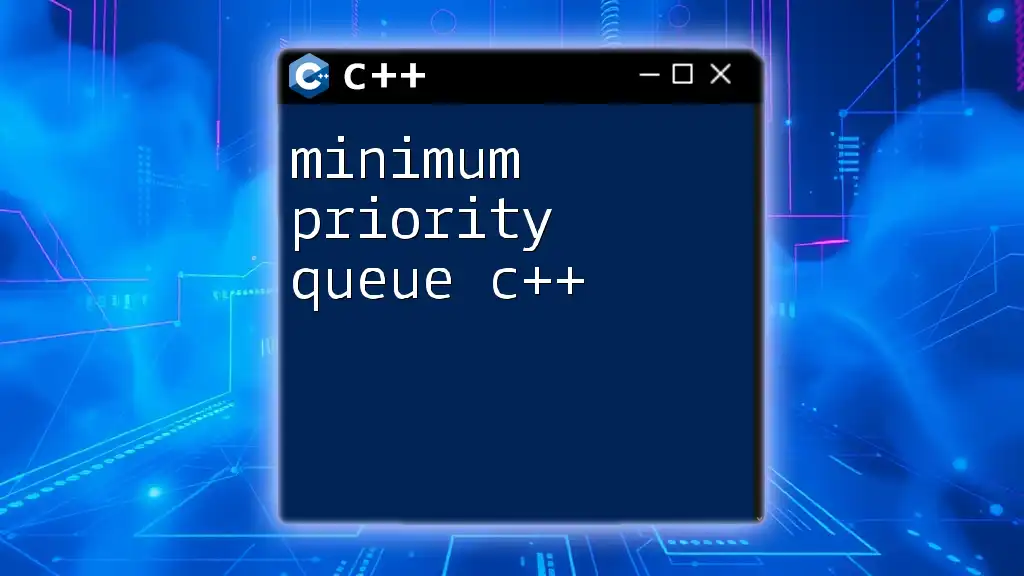
Understanding the Priority Queue in C++
Basic Structure of a Priority Queue
A priority queue is an abstract data type that provides an efficient way to manage a collection of items, where each item has a priority associated with it. In a priority queue, elements are accessed based on their priority rather than the order they were added. This means that the element with the highest (or lowest, depending on implementation) priority is served before others.
Types of Priority Queues
There are two primary types of priority queues:
-
Max Priority Queue: This type ensures that the highest value element has the utmost priority. It is useful in scenarios where the most important task needs to be executed first.
-
Min Priority Queue: Conversely, in a min priority queue, the element with the lowest value has the highest priority. This setup is beneficial in situations where the least time-consuming task should be performed first.
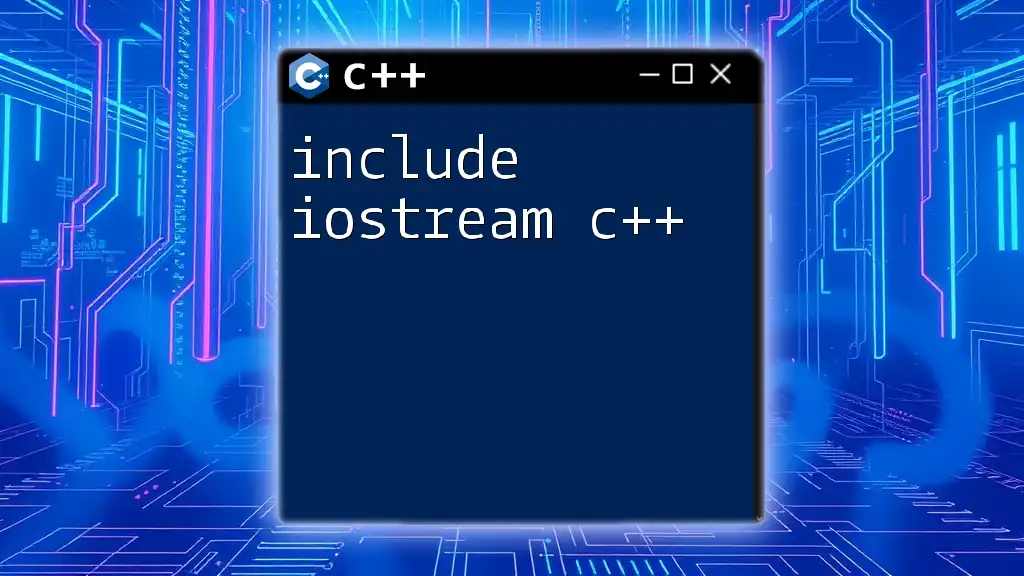
Working with Priority Queue in C++
Creating a Priority Queue
Creating a priority queue in C++ is straightforward. You can declare it using the following syntax, which will create a max priority queue by default:
std::priority_queue<int> maxHeap;
Basic Operations on Priority Queue
With the priority queue created, you can perform several essential operations:
- Inserting Elements
You can insert elements into a queue using the `push()` function. This adds the element to the queue while maintaining the heap property.
maxHeap.push(10);
maxHeap.push(5);
maxHeap.push(30);
- Accessing the Top Element
To access the element with the highest priority, you utilize the `top()` function. This function returns the value of the top element without removing it from the queue.
int highest = maxHeap.top(); // highest will be 30
- Removing the Top Element
When you need to remove the highest priority element from the queue, you use the `pop()` function. This function removes the top element and rearranges the remaining elements to maintain priority order.
maxHeap.pop(); // removes 30
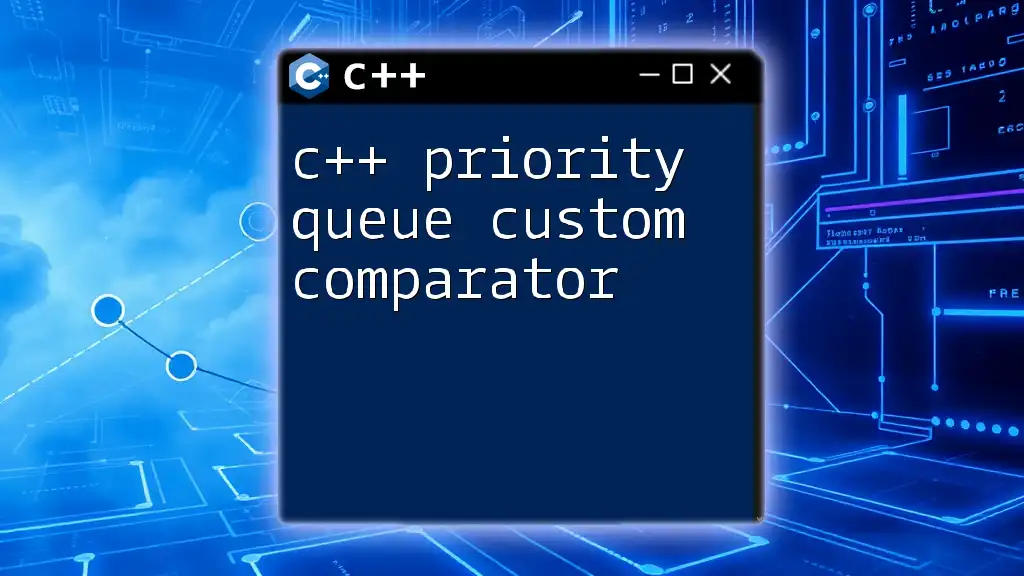
Customizing the Priority Queue
To customize the behavior of your priority queue, you can create your own comparator. This allows you to define what constitutes "priority" within your queue.
Creating a Custom Comparator
To implement a min priority queue, for instance, you can define a custom comparator like this:
struct CustomCompare {
bool operator()(int a, int b) {
return a > b; // this ensures smallest value has highest priority
}
};
std::priority_queue<int, std::vector<int>, CustomCompare> minHeap;
With the comparator in place, you can now insert numbers into `minHeap`, and it will ensure that the smallest number will always be on top.
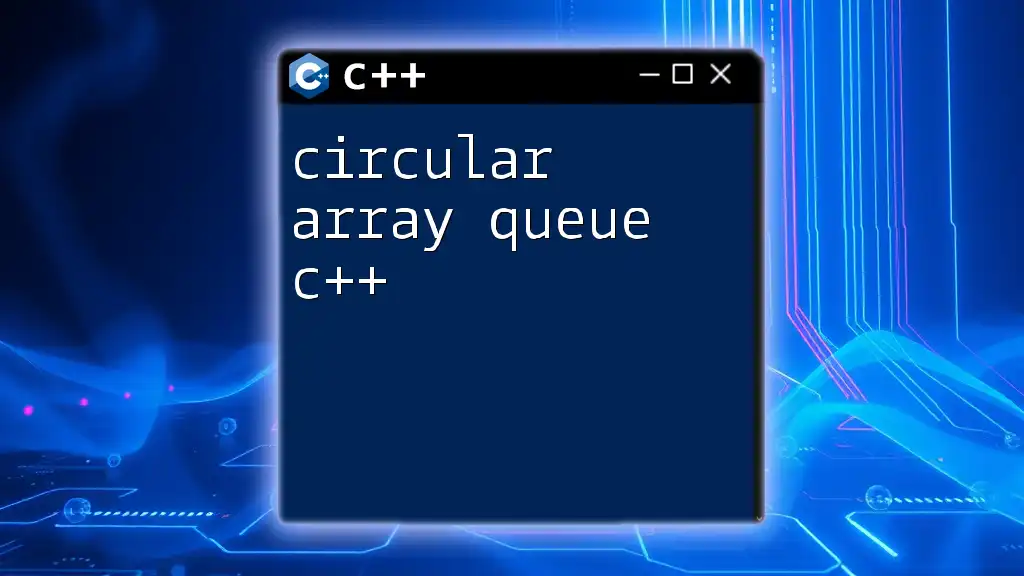
Real-Life Applications of Priority Queues
Job Scheduling
Priority queues are instrumental in job scheduling systems where tasks are assigned based on their urgency and importance. By leveraging a priority queue, systems can efficiently manage and execute tasks like batch processing or operating system task scheduling.
Dijkstra's Algorithm
Priority queues are widely used in Dijkstra's algorithm for finding the shortest path in graphs. In this algorithm, nodes are processed based on their tentative distances, ensuring that the closest node is always explored first, enhancing efficiency significantly.
Event Simulation
In discrete event simulation systems, priority queues handle events where the order is paramount. Events are managed based on their scheduled time, ensuring that the next event to occur is always processed, which is vital in simulations for systems like telecommunication networks or traffic systems.
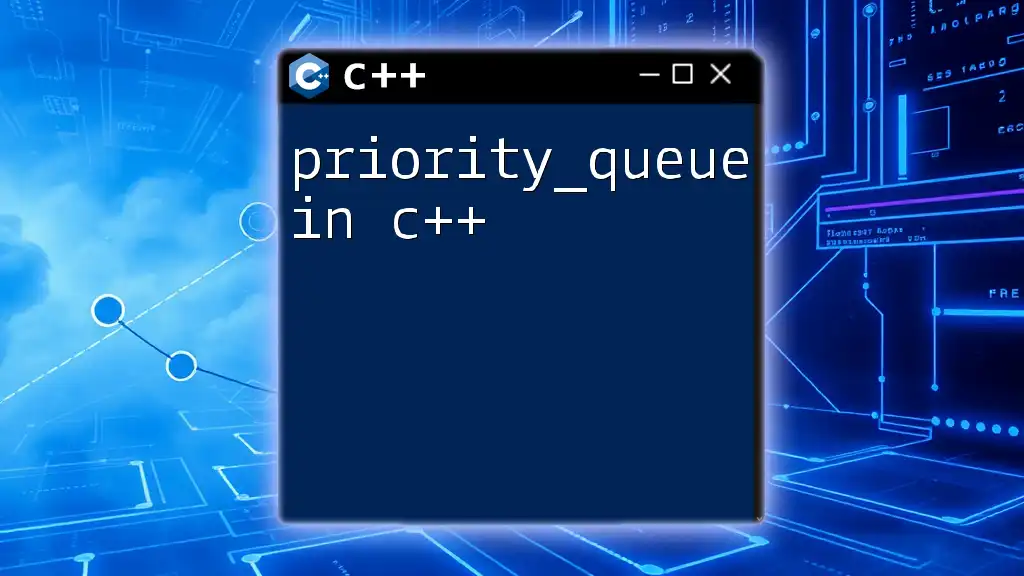
Common Mistakes and Troubleshooting
One common misunderstanding when working with priority queues is the misinterpretation of queue ordering. Developers may think that the order of elements in the queue reflects how they were added, whereas in reality, it is determined by their priorities. Being aware of this distinction can prevent logical errors in program flow.
Additionally, queue overflow is a problem that may occur if too many elements are inserted—especially in constrained environments. To manage this effectively, ensure that you check the size of the queue before inserting new elements.
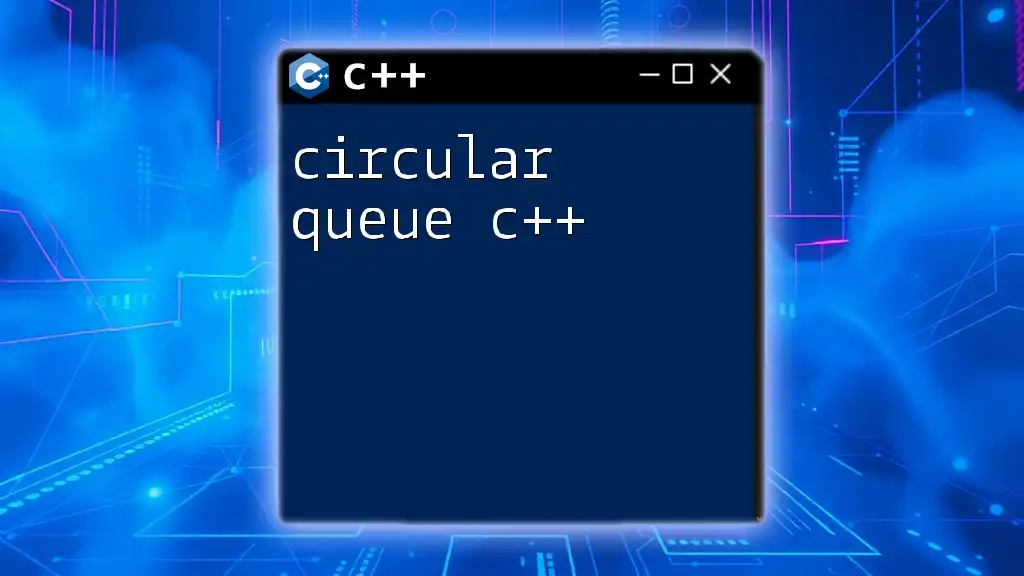
Performance Analysis
Time Complexity of Operations
Understanding the time complexity of operations on priority queues helps in choosing the right data structure for your application:
- Insertion: O(log n)
- Accessing the Top Element: O(1)
- Removing the Top Element: O(log n)
These efficiencies make priority queues a preferred choice in many computational scenarios.
Comparing Priority Queues with Other Data Structures
When compared to other data structures such as regular queues or stacks, priority queues stand out due to their ability to efficiently manage unpredictably prioritized tasks. However, choosing the correct structure depends on the specific requirements and constraints of your project.
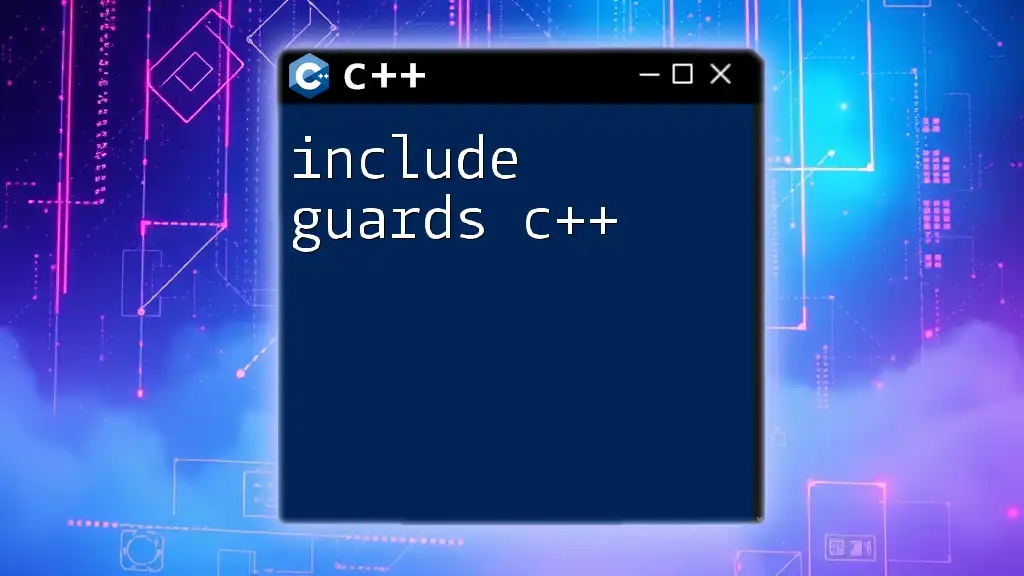
Conclusion
In summary, understanding how to include priority queue in C++ can greatly enhance your programming toolkit. Priority queues provide an optimal way to handle data that requires prioritized access, improving efficiency and responsiveness in various applications. As you delve further into C++, remember that mastering priority queues will not only add to your skills but also expand your capabilities in tackling more complex programming challenges.
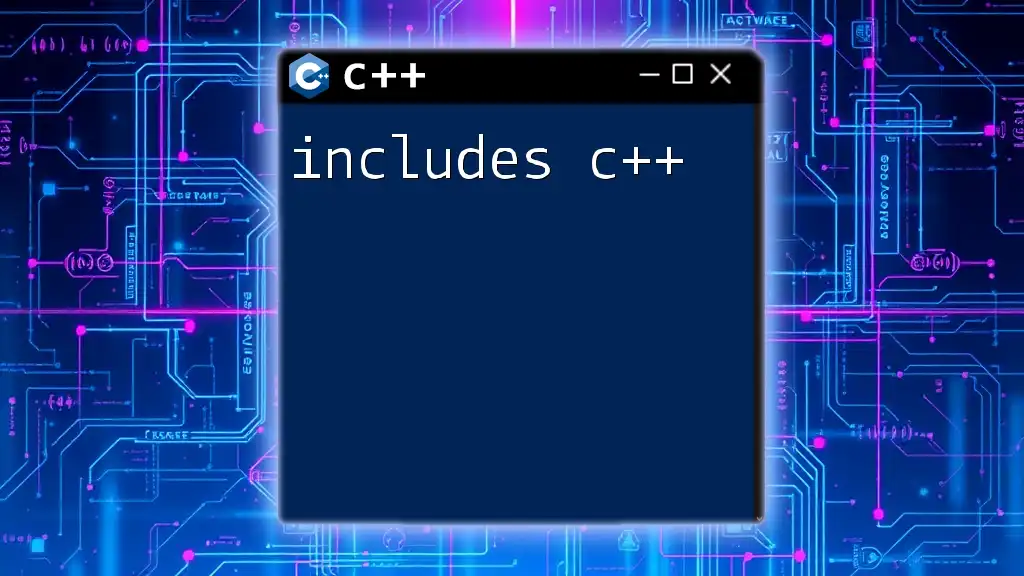
Call to Action
Stay curious and keep learning! There’s a wealth of knowledge waiting to be explored within C++. Subscribe for more insightful tips and tutorials, and take your C++ expertise to the next level!