A C++ priority queue can be customized by providing a comparator function that defines the ordering of the elements, allowing for different arrangements such as ascending or descending priority.
Here's a code snippet demonstrating how to implement a custom comparator for a priority queue:
#include <iostream>
#include <queue>
#include <vector>
struct CustomComparator {
bool operator()(const int &a, const int &b) {
return a > b; // Min-Heap: smaller elements have higher priority
}
};
int main() {
std::priority_queue<int, std::vector<int>, CustomComparator> pq;
pq.push(5);
pq.push(1);
pq.push(3);
while (!pq.empty()) {
std::cout << pq.top() << " "; // Outputs: 1 3 5
pq.pop();
}
return 0;
}
Understanding C++ Priority Queues
Overview of the Standard Library
The C++ Standard Template Library (STL) offers a rich collection of template classes and functions that provide the functionality to work with data structures and algorithms efficiently. One of these components is the priority queue, an abstract data structure that allows for the retrieval of the highest (or lowest) priority element swiftly.
Defining a Priority Queue in C++
To create a basic priority queue in C++, one can leverage the `std::priority_queue` template class. By default, this class implements a max-heap, meaning that the largest element has the highest priority.
To include the necessary features for working with a priority queue, make sure to include the following header in your code:
#include <queue>
You can then define a basic priority queue as follows:
std::priority_queue<int> pq;
This creates a priority queue that can store integers, ordering them in descending order by default.
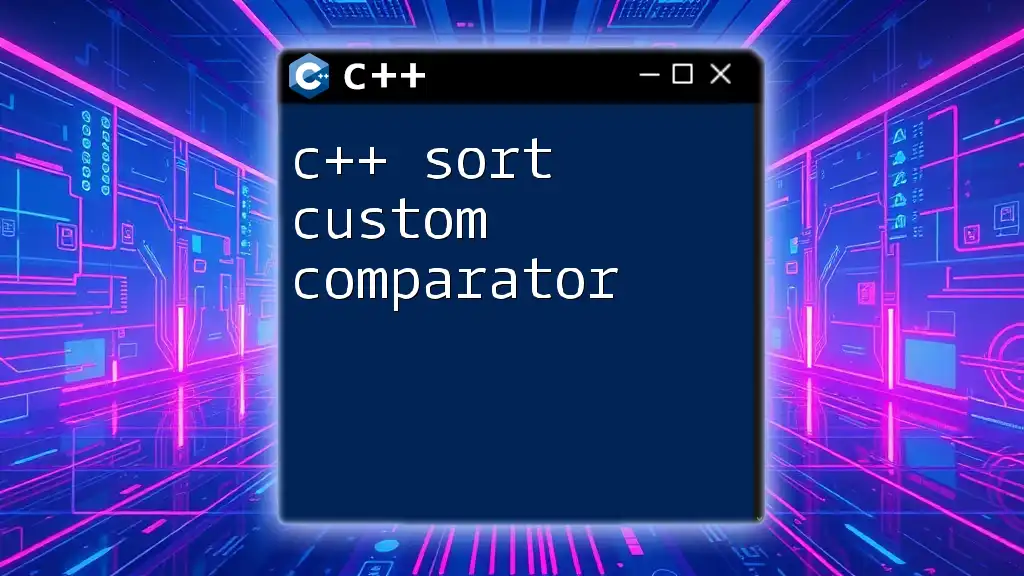
Custom Comparator in Priority Queues
What is a Custom Comparator?
A custom comparator is a user-defined way to dictate how elements in a priority queue should be ordered. By specifying a custom comparator, you can modify the default behavior of the priority queue to suit different needs or use cases. This flexibility is crucial when the required ordering differs from the natural order defined by data types.
Implementing a Custom Comparator
Lambda Function Approach
Lambda functions, introduced in C++11, offer a concise way to implement comparators directly within your code without needing separate function definitions. Here’s how you can create a priority queue sorted in ascending order using a lambda function:
auto cmp = [](int left, int right) { return left > right; };
std::priority_queue<int, std::vector<int>, decltype(cmp)> pq(cmp);
In this example:
- The `cmp` lambda function compares two integers and returns `true` if the left integer is greater than the right. This results in a min-heap where the smallest element has the highest priority.
- The `decltype(cmp)` is used to define the type of the priority queue, allowing it to use the custom comparator.
Functor Class Approach
A functor, or function object, allows you to define a class that can be used to compare elements. This is beneficial when you need the comparator to maintain state or require more complex comparison logic.
Here’s an example of a comparator functor:
struct Compare {
bool operator()(int left, int right) {
return left > right;
}
};
std::priority_queue<int, std::vector<int>, Compare> pq;
In the code above:
- The `Compare` struct defines an overloaded `operator()` that implements the comparison logic.
- When this functor is used with the priority queue, it results in a min-heap.
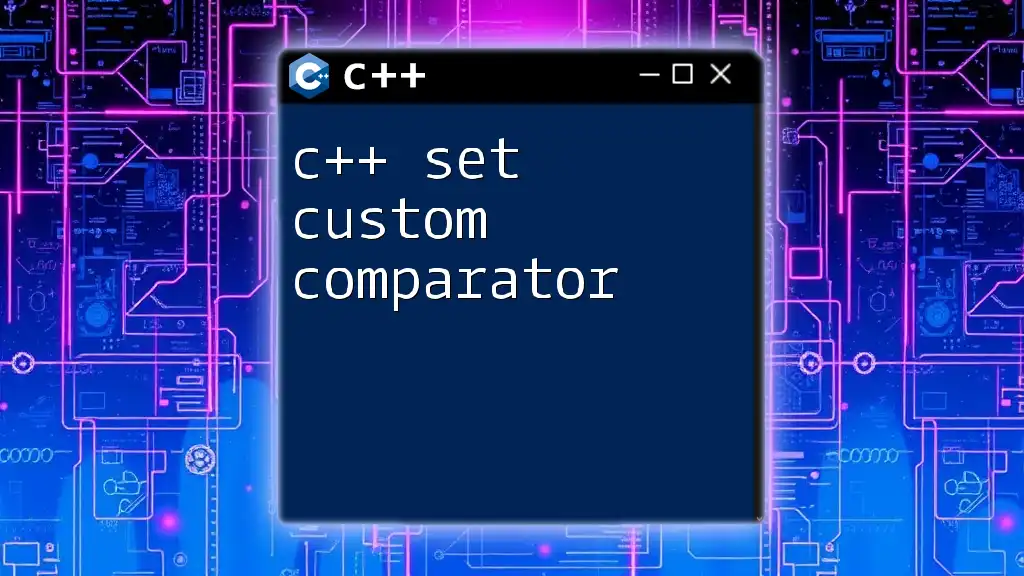
Practical Examples
Example 1: Min-Heap vs. Max-Heap
To demonstrate the differences, consider the following example where we create a min-heap utilizing the lambda comparator:
auto cmp = [](int left, int right) { return left > right; };
std::priority_queue<int, std::vector<int>, decltype(cmp)> minHeap(cmp);
minHeap.push(10);
minHeap.push(5);
minHeap.push(20);
// Pop elements from the min-heap
while (!minHeap.empty()) {
std::cout << minHeap.top() << '\n'; // Outputs: 5, 10, 20
minHeap.pop();
}
In this snippet, you can see that even though `20` was added last, the output shows that `5` was retrieved first because it holds the highest priority in a min-heap.
Example 2: Priority Queue of Custom Objects
Custom objects can also make use of comparators in a priority queue. For instance, consider a scenario where you want to manage tasks based on their priority levels.
First, define a `Task` structure:
struct Task {
int priority;
std::string name;
};
Then create a custom comparator for the `Task` structure:
struct TaskCompare {
bool operator()(const Task& t1, const Task& t2) {
return t1.priority < t2.priority; // Lower priority gets popped first
}
};
std::priority_queue<Task, std::vector<Task>, TaskCompare> taskQueue;
You can then use the priority queue as follows:
Task task1 = {2, "Task 1"};
Task task2 = {1, "Task 2"};
Task task3 = {3, "Task 3"};
taskQueue.push(task1);
taskQueue.push(task2);
taskQueue.push(task3);
// Pop tasks based on priority
while (!taskQueue.empty()) {
Task t = taskQueue.top();
std::cout << "Processing " << t.name << " with priority " << t.priority << '\n';
taskQueue.pop();
}
The output will show that tasks are processed according to their defined priority. In this case, `Task 2` would be processed first due to its higher priority level.
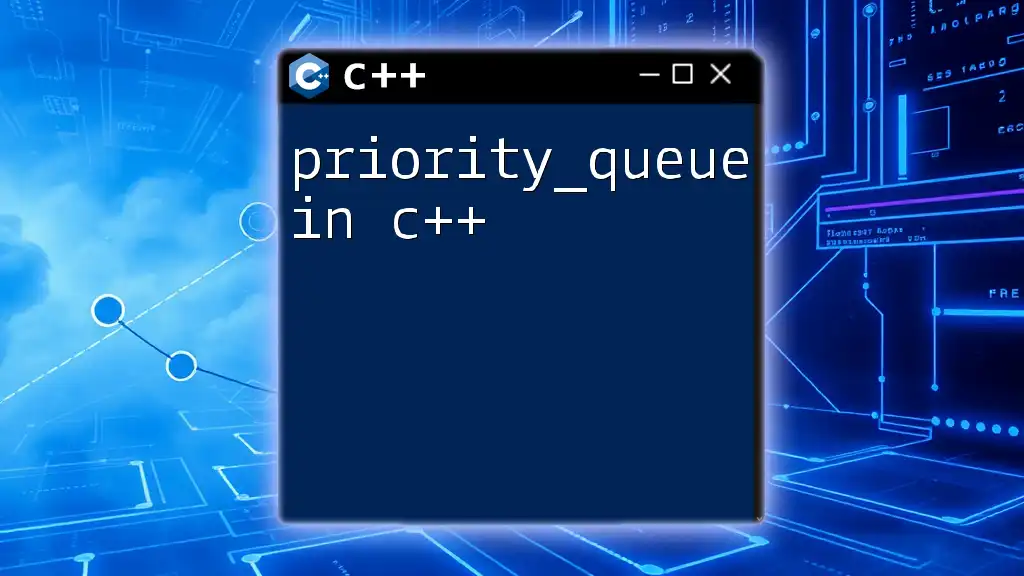
Best Practices
When to Use Custom Comparators
Using custom comparators can optimize your priority queue operations. Here are some guidelines on when to implement them:
- When default ordering is not sufficient: If you need to customize the sorting based on more than the natural order of the data type, such as coordinating between multiple criteria, a custom comparator is essential.
- When working with complex data types: If you need a priority queue that manages user-defined types, implement a custom comparator to explicitly define the order based on specific fields.
Performance Considerations
While custom comparators offer flexibility and control, they may come with additional overhead. Ensure:
- Efficiency: Keep the comparison logic as simple as possible for performance reasons.
- Maintainability: Make sure that the comparators are documented and understandable, aiding future developers (or yourself) when code maintenance is necessary.
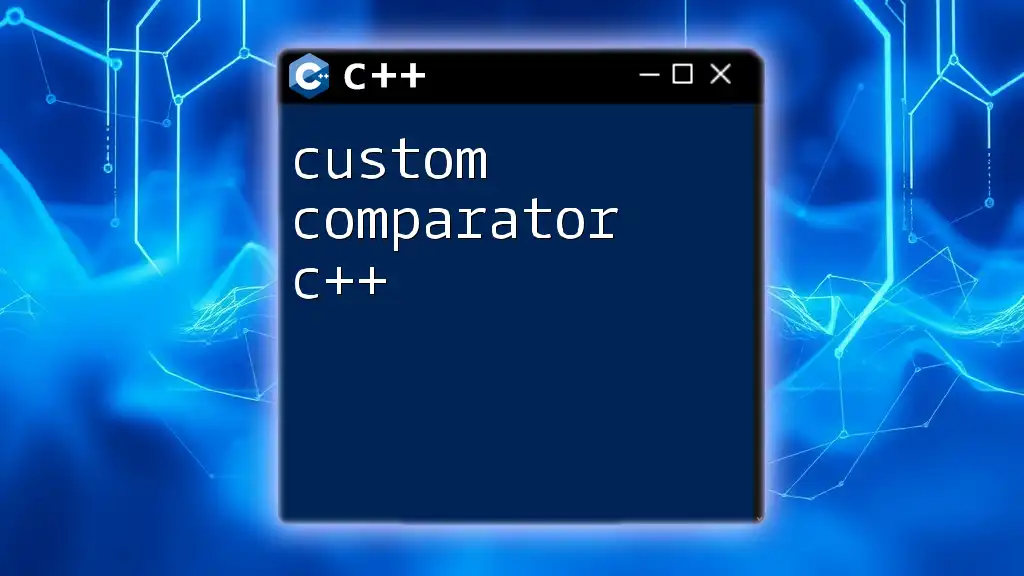
Conclusion
In summary, using a C++ priority queue custom comparator allows developers to exert fine control over the order of elements. Whether employing lambda functions or functors, custom comparators enhance flexibility, enabling the effective management of not only primitive types but also custom objects. By experimenting with different comparison strategies, you can tailor your data structures to perform under a variety of use cases.
We encourage you to try out the examples and experiment with creating your own comparators to see firsthand the potential they unlock in your applications.