File input and output in C++ allows programs to read data from files and write data to files using streams, enabling persistent storage of information. Here's a simple example of file input and output:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
outFile << "Hello, World!" << std::endl;
outFile.close();
std::ifstream inFile("example.txt");
std::string line;
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
Understanding File Streams in C++
File input output in C++ is handled using file streams. A file stream is an abstraction that allows you to read from and write to files as if they were ordinary input and output devices. The primary classes used in C++ for file handling are:
- `ifstream`: This class is utilized for reading input from files.
- `ofstream`: This class is used for outputting data to files.
- `fstream`: This versatile class can be used for both input and output operations on files.
By understanding these classes, you can effectively manage your file operations in C++.

Opening Files in C++
To work with a file, you first need to open it. This is done by creating an object of the relevant file stream class.
Syntax and Methods for Opening Files
The syntax for opening files varies slightly depending on the class used. Here’s how you can do it:
#include <fstream>
#include <iostream>
std::ifstream inputFile("example.txt");
std::ofstream outputFile("example.txt");
std::fstream readWriteFile("example.txt", std::ios::in | std::ios::out);
In the example above, the file `example.txt` is opened with different modes depending on the operation to be performed.
Error Handling While Opening Files
It’s essential to verify if a file was opened successfully. C++ provides mechanisms to check the state of the file stream. Here’s how to handle errors:
if (!inputFile.is_open()) {
std::cerr << "Error opening the file." << std::endl;
}
This code checks whether `inputFile` has opened successfully and will output an error message if it hasn't.
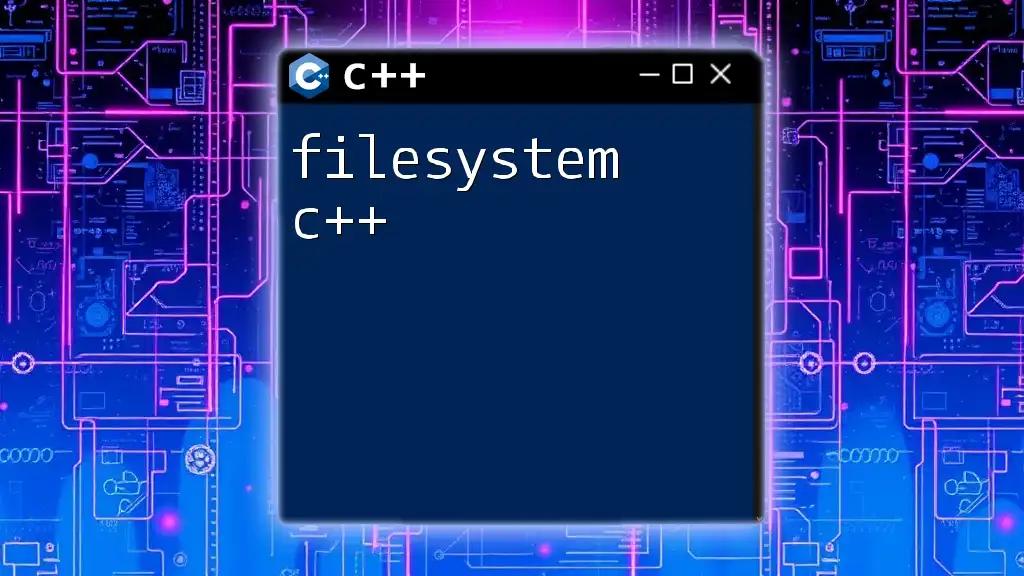
Writing to Files in C++
Writing data to files using `ofstream` is straightforward. You can output various types of data, such as strings, integers, and floats.
Basic Writing Example
Here’s an example of writing a string to a file:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("output.txt");
if (outputFile.is_open()) {
outputFile << "Hello, World!" << std::endl;
}
outputFile.close();
return 0;
}
In this code, we first open `output.txt` for writing, then check if it's open, and finally write "Hello, World!" to the file.
Writing Different Data Types
You can also write multiple data types to a file. Here’s an example:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("output.txt");
if (outputFile.is_open()) {
int number = 42;
float decimal = 3.14;
outputFile << "Number: " << number << std::endl;
outputFile << "Decimal: " << decimal << std::endl;
}
outputFile.close();
return 0;
}
Closing the File After Writing
Closing the file after writing is essential to ensure that the data is flushed and resources are freed. Always use the `close()` function, as shown in previous examples.
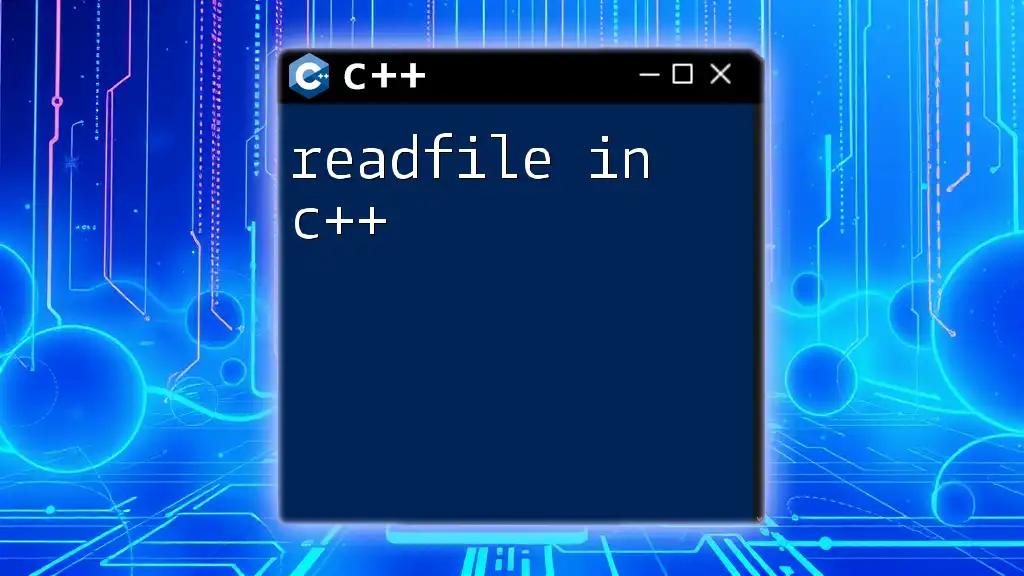
Reading from Files in C++
Reading data from files is done using the `ifstream` class. C++ makes it easy to extract data from files using the input stream operators.
Basic Reading Example
Here’s an example of how to read a line from a file:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream inputFile("input.txt");
std::string line;
if (inputFile.is_open()) {
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
}
inputFile.close();
return 0;
}
In this code, we open `input.txt` and read it line by line until the end of the file (EOF).
Reading Different Data Types
You can read various types by using the input stream operators. Here’s an example for reading an integer and a float:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inputFile("input.txt");
int number;
float decimal;
if (inputFile.is_open()) {
inputFile >> number >> decimal;
std::cout << "Number: " << number << ", Decimal: " << decimal << std::endl;
}
inputFile.close();
return 0;
}
Error Handling While Reading
Always check for EOF while reading to avoid accessing out-of-bounds memory. Here’s how you can do it:
if (inputFile.eof()) {
std::cout << "Reached the end of the file." << std::endl;
}

Combined Input and Output with `fstream`
The `fstream` class allows both reading from and writing to the same file. This is particularly useful when you want to modify the content of a file while reading it.
Example of Combined Input/Output
Here’s how you can achieve both input and output using `fstream`:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::fstream myFile("example.txt", std::ios::in | std::ios::out);
std::string line;
if (myFile.is_open()) {
while (std::getline(myFile, line)) {
std::cout << line << std::endl;
myFile << " - Read" << std::endl; // Append read acknowledgment
}
}
myFile.close();
return 0;
}
In this example, you can read the content of `example.txt` while writing acknowledgments of the read operation.
Use Cases for `fstream`
Using `fstream` is particularly advantageous when you need to:
- Modify the content of a file without creating a temporary copy.
- Read data while processing it, such as logging read operations.

Handling Text and Binary Files
Text files are files that contain data in a human-readable format, while binary files contain data in a format that is typically unreadable to humans. To work effectively with file input output in C++, it’s crucial to understand the differences between these two types.
How to Open, Read, and Write Binary Files
To work with binary files, you must specify the binary mode when opening a file. Here’s how you can do it:
#include <fstream>
std::ofstream binaryFile("binary.dat", std::ios::binary);
Similarly, you can read and write data in binary format:
#include <fstream>
int main() {
std::ofstream binaryFile("binary.dat", std::ios::binary);
int value = 12345;
binaryFile.write(reinterpret_cast<char*>(&value), sizeof(value));
binaryFile.close();
std::ifstream readBinary("binary.dat", std::ios::binary);
int readValue;
readBinary.read(reinterpret_cast<char*>(&readValue), sizeof(readValue));
readBinary.close();
std::cout << "Read Value: " << readValue << std::endl;
return 0;
}
Pros and Cons of Text vs Binary
Text Files:
- Advantages: Easier to read and debug; portable across platforms.
- Disadvantages: Larger file size due to formatting.
Binary Files:
- Advantages: More compact and faster to read/write; better for large datasets.
- Disadvantages: Not human-readable, and can lead to compatibility issues between platforms.

Best Practices for File Input and Output in C++
When working with file input output in C++, it's crucial to follow some best practices to avoid common issues:
- Always Check If Your Files Open Successfully: Before proceeding with reading or writing, always ensure the file stream is valid.
- Close Files: Always close your files with `close()` after operations to prevent memory leaks and corrupted data.
- Use Exception Handling: Implement try-catch blocks to handle potential errors more gracefully.
- Be Consistent with File Modes: Clearly specify the modes (`ios::in`, `ios::out`, `ios::binary`) to avoid logical errors.
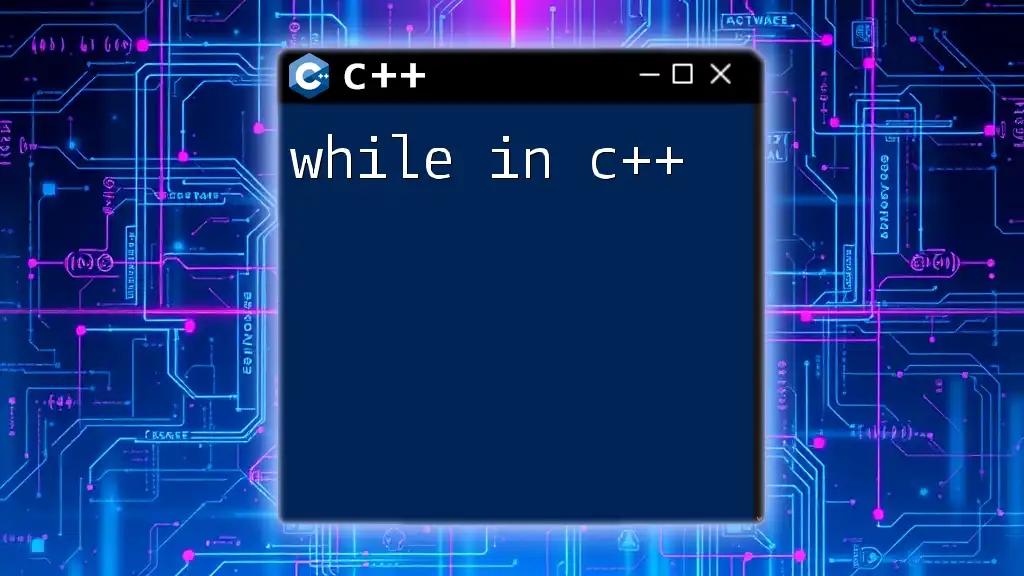
Common Mistakes to Avoid
When working with file input output in C++, programmers often run into common pitfalls:
- Forgetting to Close Files: Not closing files can lead to data corruption.
- Ignoring Error Handling: Failing to check if a file opened successfully or if data was read can lead to unexpected crashes.
- Using the Wrong Modes: Using text mode for binary files can corrupt your data.
For example, trying to read a binary file in text mode without specifying `std::ios::binary` will result in incorrect data being read.

Conclusion
Mastering file input output in C++ is a crucial skill for any programmer. Understanding the various classes, properly managing file operations, and implementing best practices can significantly enhance your programming capabilities. Experimenting with these concepts will deepen your understanding, making you more proficient in handling files effectively in C++.
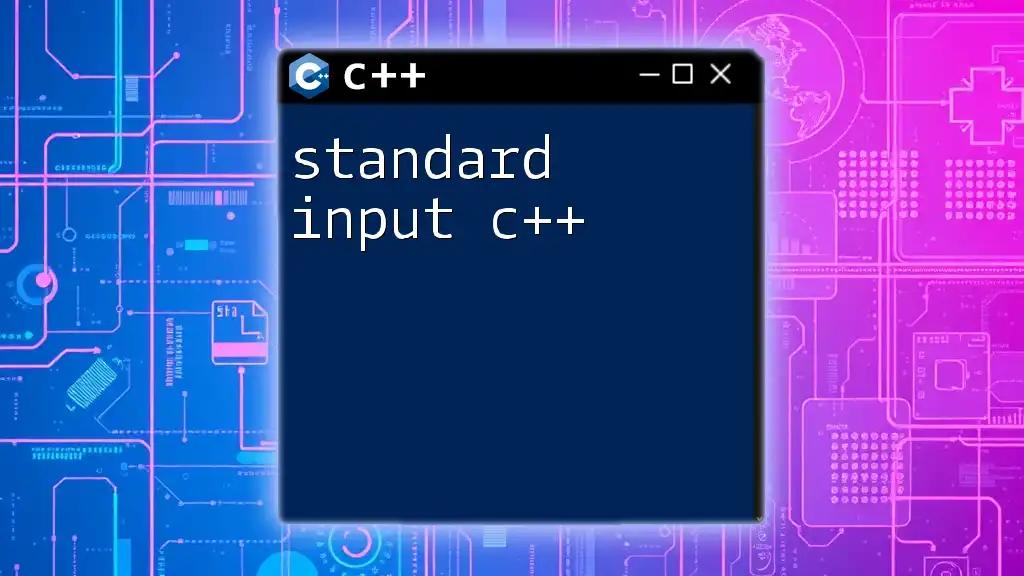
Further Resources
For those looking to deepen their understanding of file handling in C++, consider exploring additional resources such as books, online tutorials, or courses. Many platforms offer structured content to boost your C++ skills further.