File input and output in C++ allows programmers to read from and write to files using streams, enabling the storage and retrieval of data efficiently.
Here's a simple code snippet demonstrating file input and output in C++:
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
outFile << "Hello, World!" << std::endl;
outFile.close();
// Reading from a file
std::ifstream inFile("example.txt");
std::string line;
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
Understanding File Streams in C++
What are File Streams?
File streams in C++ provide a powerful way to handle data stored in files, enabling seamless interaction between the program and files on the system. They are primarily used for reading input from files or writing output to them. The concept of file streams revolves around abstraction, allowing developers to treat file operations similarly to standard input and output operations.
The three key classes involved in file streams are:
- `ifstream`: Used for input file streams, which allow reading data from files.
- `ofstream`: Used for output file streams, which enable writing data to files.
- `fstream`: Combines the functionality of both input and output for files, allowing for reading and writing.
Basic Syntax for File Streams
To work with file streams, one must understand the basic syntax for creating them. Here’s how you create an input stream:
std::ifstream inputFile("data.txt");
This statement creates an instance of `ifstream` that attempts to open the file `data.txt` for reading.
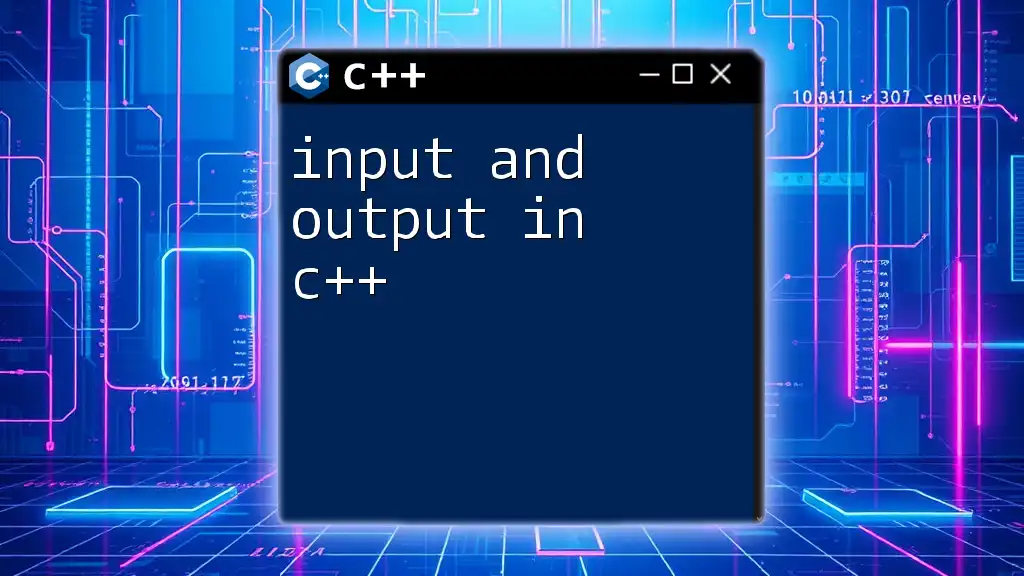
Opening and Closing Files
How to Open a File
Opening a file in C++ can be done in multiple ways. The most common method is to use the constructor or the `open()` function of the respective stream class. When file opening fails, it may lead to errors that can significantly affect your program.
Here’s an example of opening a file with error handling:
std::ifstream inputFile;
inputFile.open("data.txt");
if (!inputFile) {
std::cerr << "Error opening file." << std::endl;
}
In this example, we check if the file was opened successfully by evaluating `inputFile`. If it returns `false`, an error message is displayed.
How to Close a File
Closing files is crucial to avoid memory leaks and ensure that all data is flushed properly. You can close a file using the `close()` method:
inputFile.close();
Failure to close a file can lead to data corruption, especially if the program exits unexpectedly.
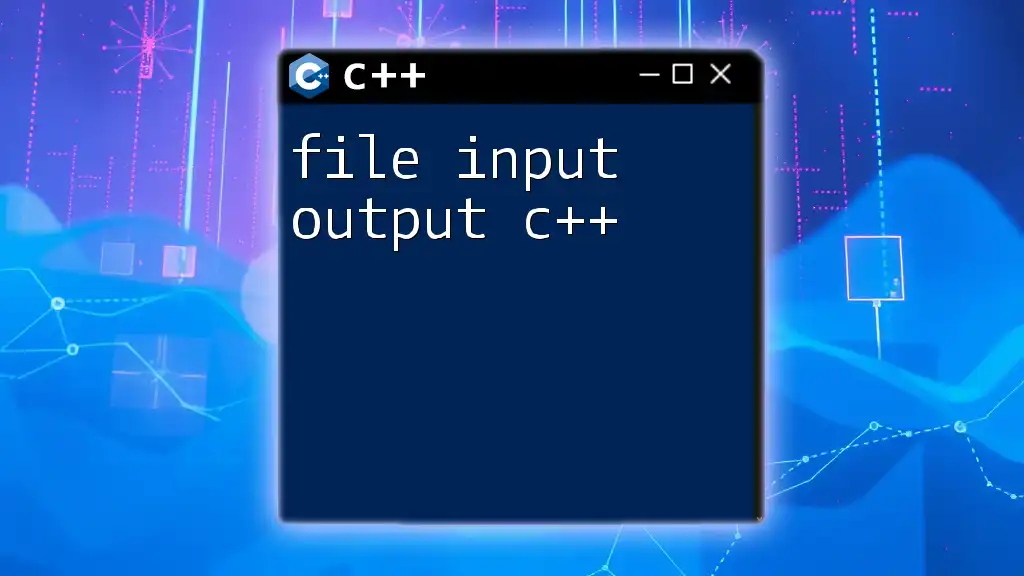
Reading from Files
Reading Text Files
To read data from text files, you use the `ifstream` class, which provides methods to read characters, lines, or formatted data. A common approach is to read line by line using `std::getline()`:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
This loop continues to read lines until the end of the file is reached, displaying each line read.
Reading Binary Files
Reading binary files differs from reading text files because binary files store data in a format that is not human-readable. The `ifstream` class can be set to read in binary mode using the `std::ios::binary` flag:
std::ifstream binaryFile("data.bin", std::ios::binary);
char buffer[100];
binaryFile.read(buffer, sizeof(buffer));
In this example, we define a buffer to hold the binary data and read it into the buffer. The size of the buffer determines how much data you read in one operation.
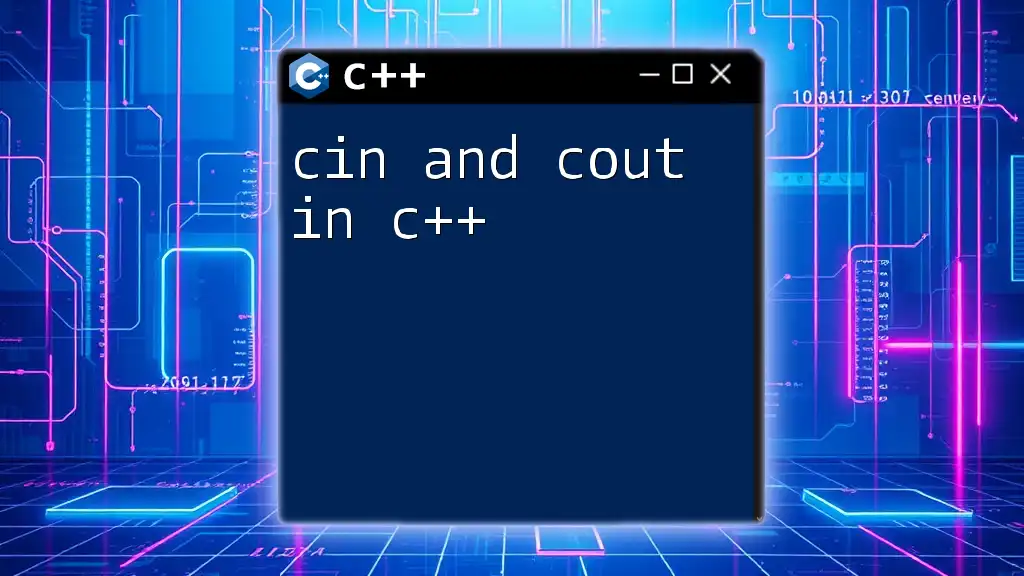
Writing to Files
Writing Text Files
Writing to text files can be accomplished using the `ofstream` class. This allows you to create new files or overwrite existing ones. Here’s a simple example:
std::ofstream outputFile("output.txt");
outputFile << "Hello, World!" << std::endl;
In this case, the message "Hello, World!" is written to `output.txt`. The `std::endl` ensures a newline character is added.
Writing Binary Files
Similarly, writing binary files involves opening the stream in binary mode using `ofstream`. Here is how you might write to a binary file:
std::ofstream binaryOutput("output.bin", std::ios::binary);
binaryOutput.write(buffer, sizeof(buffer));
This example writes the contents of a buffer directly to a binary file, preserving the byte structure of the data.
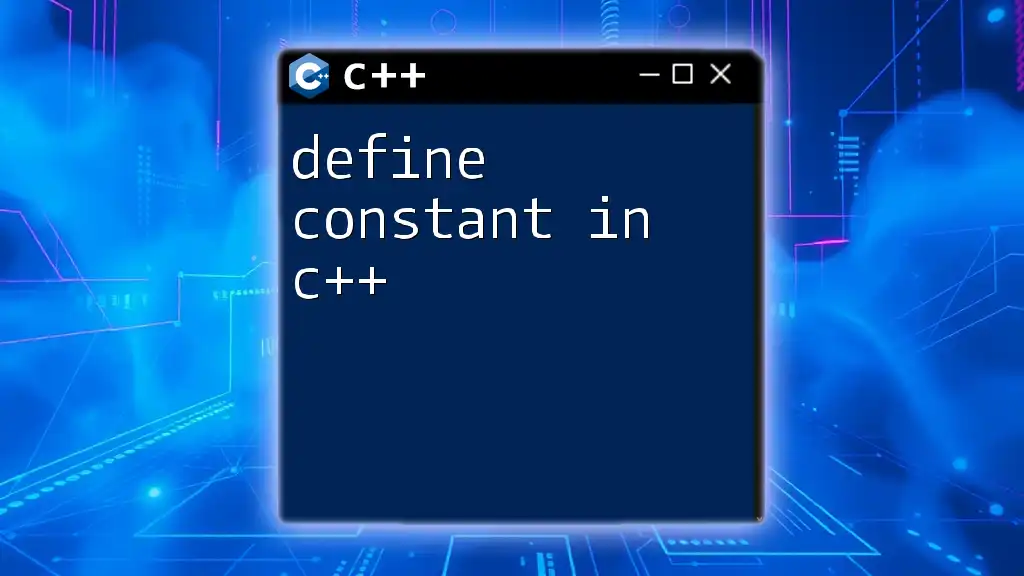
Handling File Errors
Common File I/O Errors
File I/O can sometimes be prone to errors, arising from issues like missing files, permission restrictions, or corrupt data. Common errors include:
- File not found: The specified file path may be incorrect.
- Permission denied: The program might not have the necessary access rights.
- Disk full: The device may have insufficient space to maintain new data.
Error Handling Techniques in C++
C++ provides built-in methods such as `fail()`, `eof()`, and `bad()` to verify the state of a stream. For instance, you might want to check if a read operation failed:
if (inputFile.fail()) {
std::cerr << "Error reading from file." << std::endl;
}
This line checks if the last file operation encountered an error, allowing for cleaner error handling.
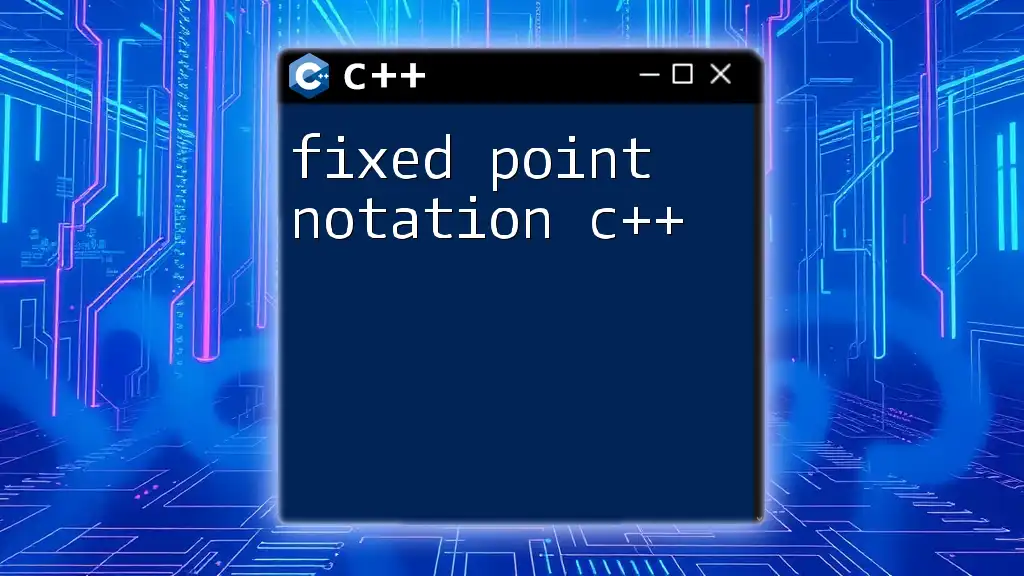
Practical Examples of File I/O
Example: Copying a Text File
A practical implementation of file I/O can be seen in a simple file copy program. Here’s how you can copy contents from one file to another using `rdbuf()`:
std::ifstream source("source.txt");
std::ofstream destination("destination.txt");
destination << source.rdbuf();
This efficiently copies all data from `source.txt` to `destination.txt`.
Example: Storing User Input in a File
A basic use case for file output is storing user input. Here’s how you can prompt users and save their responses to a file:
std::string userInput;
std::ofstream userFile("user_input.txt");
std::cout << "Enter some text: ";
std::getline(std::cin, userInput);
userFile << userInput << std::endl;
In this code snippet, user input is accepted and written to `user_input.txt`.
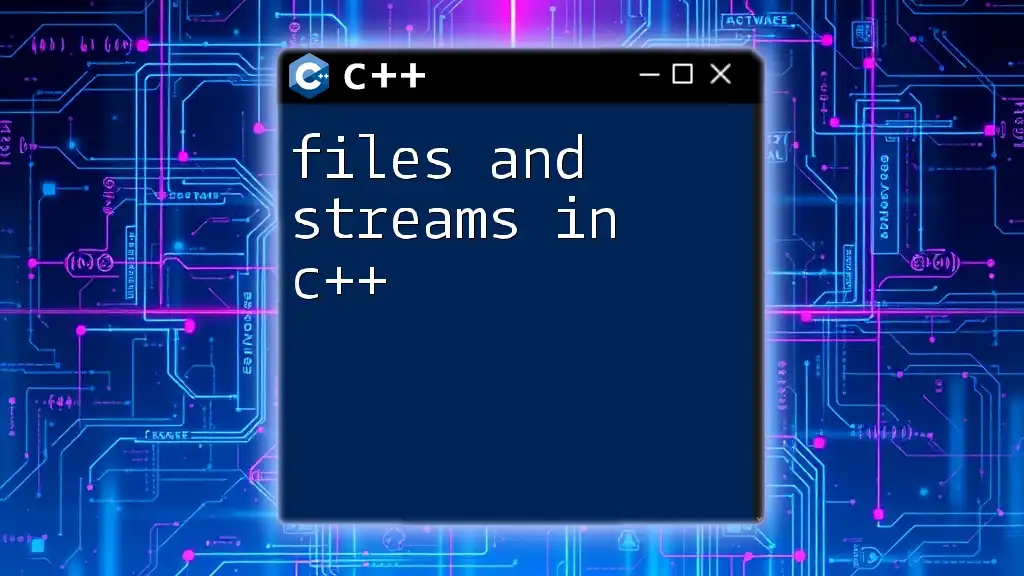
Conclusion
In conclusion, understanding file input and output in C++ is essential for managing data effectively in any programming project. Familiarizing yourself with file streams, reading and writing methods, and proper error handling equips you with the necessary skills to manipulate and manage files smoothly. Practicing these concepts will deepen your understanding and enhance your programming efficiency in C++.
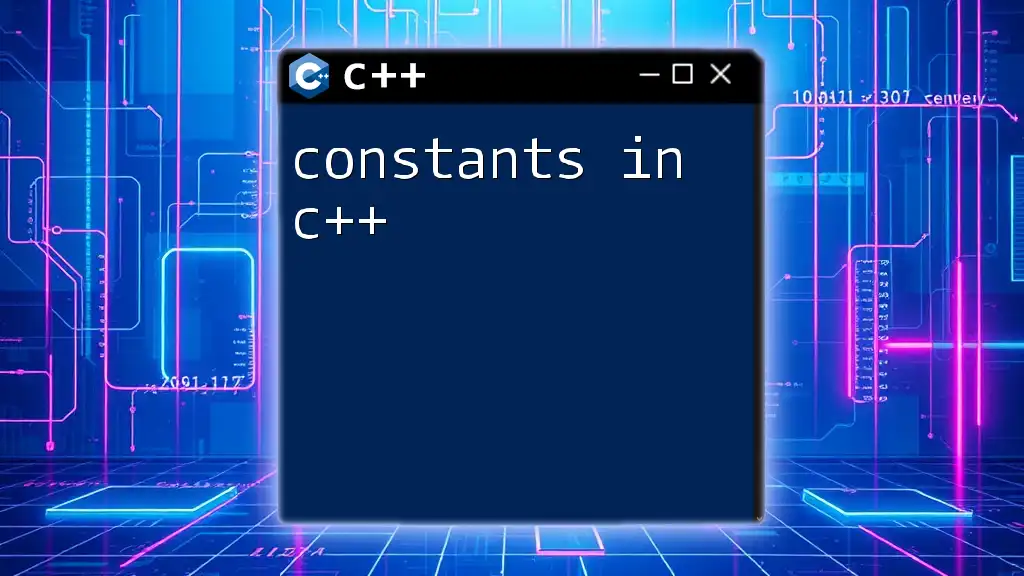
FAQ About File Input and Output in C++
What is the difference between text and binary files?
Text files store data in a human-readable format, while binary files store data in a format that is meant for machine reading. This fundamental difference affects how data is read and written.
How do I check if a file exists in C++?
You can verify the existence of a file by attempting to open it with an ifstream and checking if the open operation was successful.
Can I read and write to the same file in C++?
Yes, you can read and write from the same file by using the `fstream` class, which allows you to perform both operations on the same file stream.