In C++, input and output operations are primarily handled using the `cin` object for input and the `cout` object for output, allowing for interaction with the user through the console.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: "; // Output
cin >> number; // Input
cout << "You entered: " << number << endl; // Output
return 0;
}
Overview of Input and Output in C++
Understanding the Importance of Input and Output in C++
Input and output operations are critical in programming, particularly in C++. They serve as the primary means through which user interaction occurs, enabling applications to receive data and display results. By mastering input and output in C++, developers can create programs that are not only functional but also user-friendly.
Basic Concepts in C++ I/O
C++ utilizes a concept known as streams for I/O operations, which represent a sequence of data elements that can be processed. Streams can be thought of as a flow of data that can be directed to various sources or targets, such as the console or files. The most common types of streams are:
- Input Streams: Used to read data from sources (like keyboard input).
- Output Streams: Used to send data to targets (like the console or files).
The standard streams provided by C++ include:
- `cin`: Standard input stream for receiving input.
- `cout`: Standard output stream for sending output.
- `cerr`: Standard error stream, usually for error messages.
- `clog`: Standard logging stream for logging information.

The C++ Standard Input and Output Library
Introduction to iostream
To utilize the input and output functionalities in C++, one must include the `<iostream>` header file. This is essential for accessing the stream classes and operations that facilitate I/O operations. The C++ standard library manages various components related to input and output, making it easier for developers to implement these features within their applications.
The Output Stream: cout
Basic Output Operations
The `cout` stream is used for outputting information to the console. The syntax involves using the insertion operator (`<<`). For example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, the message "Hello, World!" is printed to the console. The `std::endl` is often used to insert a newline and flush the output buffer.
Formatting Output
C++ allows for various output formatting options, enhancing how data is presented. For instance, using `std::endl` can be a simple way to end a line, but a newline character (`'\n'`) can be more efficient in specific contexts since it doesn't flush the buffer:
#include <iostream>
int main() {
std::cout << "First Line" << '\n'; // More efficient than std::endl
std::cout << "Second Line" << std::endl; // Flushes the buffer
return 0;
}
Formatting numbers can be accomplished through manipulators like `std::setw()` for setting field width and `std::setprecision()` for controlling decimal places:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl; // Outputs: 3.14
return 0;
}
The Input Stream: cin
Basic Input Operations
The `cin` stream facilitates user input via the keyboard. Through the extraction operator (`>>`), data can be read into variables:
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number; // Input operation
std::cout << "You entered: " << number << std::endl;
return 0;
}
In this example, the program prompts the user to enter a number, which is then stored in the variable `number` and displayed back to the user.
Handling Input Data
Input can sometimes lead to errors, especially if the data type does not match what is expected. Robust programs should include input validation to handle these scenarios:
#include <iostream>
#include <limits>
int main() {
int number;
std::cout << "Enter a number: ";
while (!(std::cin >> number)) {
std::cin.clear(); // Clears the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discards invalid input
std::cout << "Invalid input, please enter a number: ";
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
This code snippet employs a `while` loop to ensure that the user is prompted until valid input is entered.

Advanced C++ Input and Output Techniques
File Input and Output
Introduction to File Streams
File handling in C++ enables the reading from and writing to files, which is crucial for data persistence. To manage file operations, the `<fstream>` library is integral.
Writing to a File
Creating and writing content to a file is straightforward with `ofstream` (output file stream):
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
outFile << "Writing to a file using C++!" << std::endl;
outFile.close(); // Don't forget to close the file!
return 0;
}
This example demonstrates how to write text to a file named `example.txt`. It’s essential to close the file once operations are complete to ensure all buffers are flushed.
Reading from a File
Reading data from a file is similarly easy using `ifstream` (input file stream):
#include <iostream>
#include <fstream>
int main() {
std::ifstream inFile("example.txt");
std::string content;
while (std::getline(inFile, content)) {
std::cout << content << std::endl; // Output each line
}
inFile.close(); // Close the file after reading
return 0;
}
This code reads each line from `example.txt` and outputs it to the console.
Formatting Input and Output
Using Stream Manipulators
C++ offers a range of stream manipulators to format input and output conveniently. Manipulators like `std::left`, `std::right`, `std::fixed`, and `std::scientific` change how data is displayed:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::left << std::setw(10) << "Name" << std::setw(10) << "Age" << std::endl;
std::cout << std::left << std::setw(10) << "Alice" << std::setw(10) << 30 << std::endl;
std::cout << std::left << std::setw(10) << "Bob" << std::setw(10) << 25 << std::endl;
return 0;
}
This example demonstrates how to align text within a specified width, enhancing visual clarity in console output.

Best Practices for C++ Input and Output
Handling Errors and Exceptions
Implementing error checking is vital for maintaining program stability. Utilizing `try` and `catch` blocks can help manage exceptions that arise during input and output operations:
#include <iostream>
#include <stdexcept>
int main() {
try {
int number;
std::cout << "Enter a number: ";
if (!(std::cin >> number)) {
throw std::runtime_error("Invalid input!");
}
std::cout << "You entered: " << number << std::endl;
} catch (const std::runtime_error& e) {
std::cerr << "Error: " << e.what() << std::endl; // Display error message
}
return 0;
}
This code snippet illustrates how to manage errors gracefully and provide feedback to the user.
Optimizing Performance
To improve the efficiency of input and output operations, consider minimizing delays during I/O. Using `std::ios::sync_with_stdio(false)` can enhance performance by decoupling C++ streams from C streams, which can be beneficial when high-performance console applications are required.
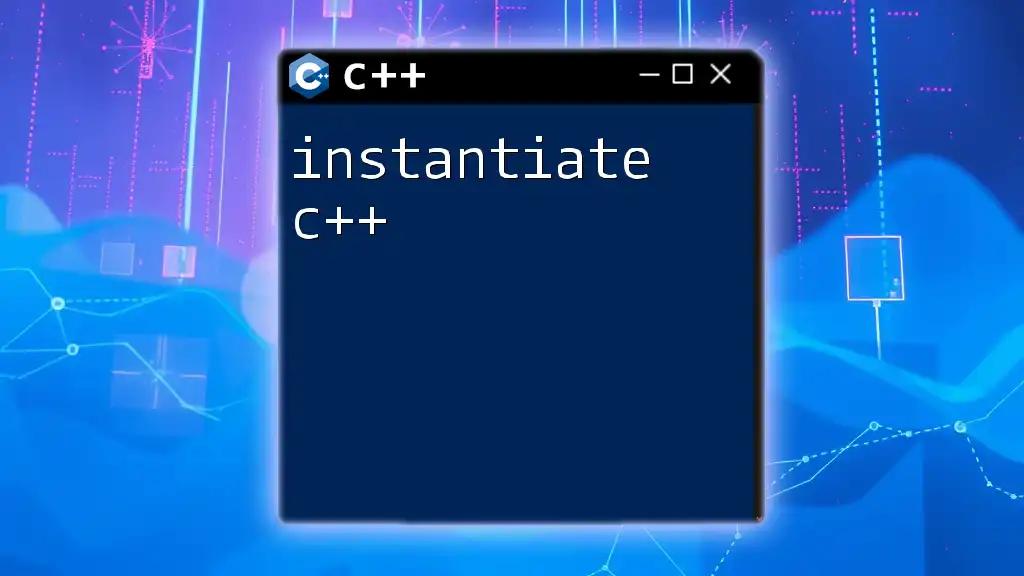
Conclusion
Recap of Key Points
In summary, input and output in C++ are essential components that empower user interaction with applications. Mastering these functions enables programmers to create more reliable and user-friendly software.
Encouraging Further Learning
To deepen your understanding of input and output in C++, explore additional concepts such as manipulator functions, advanced file handling techniques, and performance optimization strategies. Additionally, consulting the official C++ documentation can provide further insights into the rich capabilities of the language.