In C++, you can take input from the user using the `cin` object along with the insertion operator (`>>`) to store the input in a variable.
Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
Understanding User Input in C++
What is User Input?
User input refers to the data that is provided by the user to a program during its execution. In the context of C++, it plays a critical role in making programs dynamic and responsive to user actions. Applications that rely on user input range from simple command-line tools to complex software with graphical interfaces, where user interaction is essential.
Importance of User Input in C++
User input is crucial for various scenarios in programming. It allows programs to interact with users, making them more than just static output tools. Here are a few situations where user input is particularly important:
- Interactive applications like games where players make decisions.
- Forms where users input data needed for submissions.
- Command-line utilities that require specific parameters to execute actions.
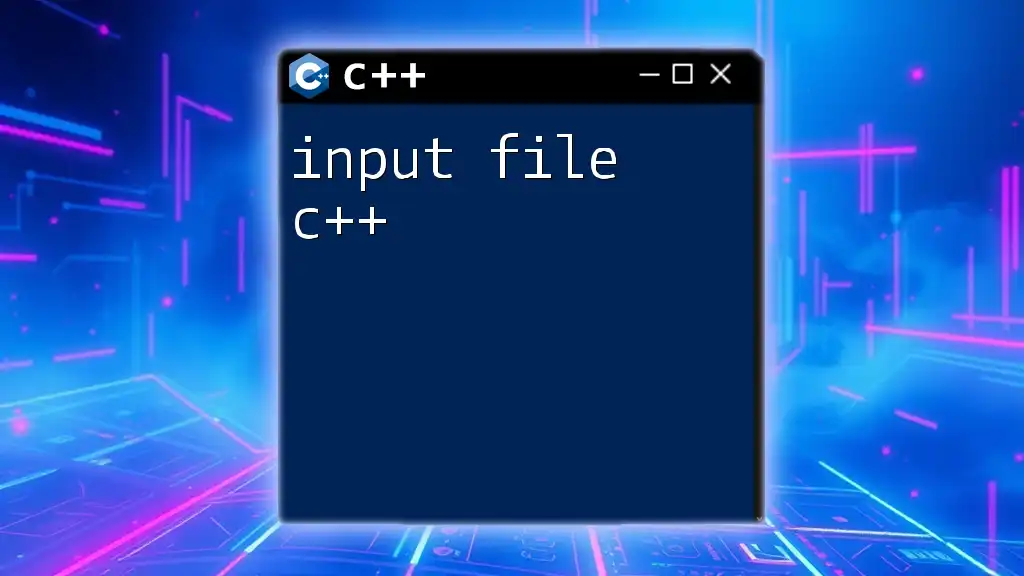
How to Get User Input in C++
Using `cin` for Standard Input
One of the most common methods to accept user input in C++ is through the `cin` stream, which is part of the `<iostream>` library.
Basic Syntax and Example:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You entered: " << age << endl;
return 0;
}
In this example, when the program prompts the user to "Enter your age: ", the user can type a number. The `cin >> age;` statement takes this input and stores it in the variable `age`. The program then confirms the input by echoing it back.
Handling Different Data Types with User Input
C++ allows you to capture various data types from user input seamlessly.
Inputting Primitive Data Types
Here’s how you can accept different primitive types in a straightforward manner:
int age;
float salary;
char grade;
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Enter your salary: ";
cin >> salary;
cout << "Enter your grade: ";
cin >> grade;
Each variable is read using the same `cin` format. It’s essential to note how data types are matched with user input, as an improper data type can lead to errors or undefined behavior.
Inputting Multiple Values
You can also collect multiple inputs in a single line:
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
cout << "You entered: " << a << " and " << b << endl;
This example showcases how multiple variables can be filled in with a single `cin` statement, improving the flow of data collection.
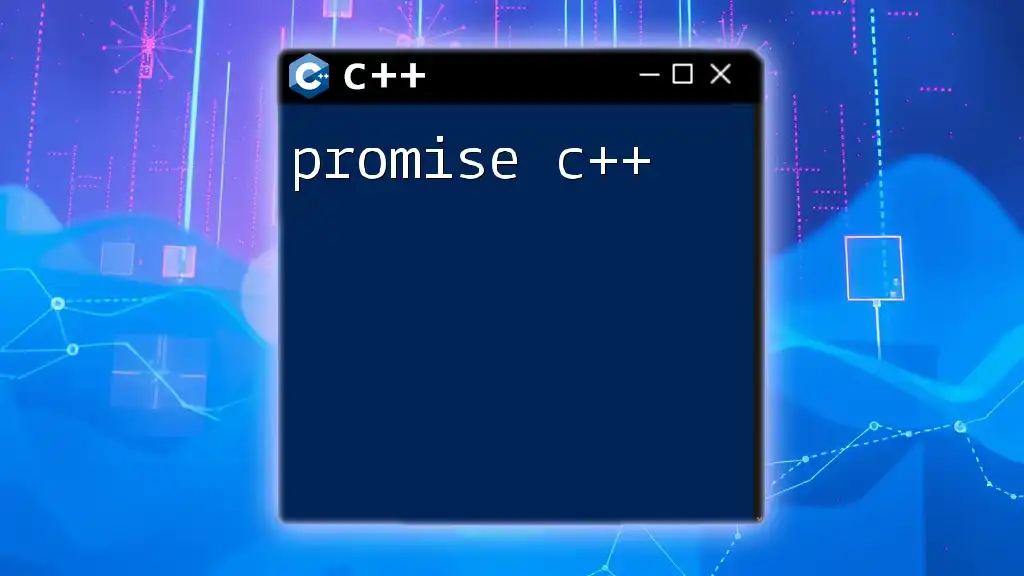
Advanced User Input Techniques
Using `getline` for Strings
While `cin` is versatile, it has limitations, especially when dealing with strings that may contain spaces. Using `getline` allows for more comprehensive input handling.
Example Using `getline`:
string fullName;
cout << "Enter your full name: ";
getline(cin, fullName);
cout << "Hello, " << fullName << endl;
In this case, `getline(cin, fullName);` captures the entire line of input, including spaces, which `cin` would otherwise ignore after the first space.
Input Validation Techniques
Validating Integer Input
Validating user input is crucial to prevent crashes or unexpected behavior in programs. Here's a simple way to validate integer input:
int x;
while (true) {
cout << "Enter an integer: ";
if (cin >> x) {
break; // Valid input, exit loop
} else {
cout << "Invalid input. Please enter an integer." << endl;
cin.clear(); // Clear the error flag
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discard invalid input
}
}
In this example, the program continues to prompt the user until a valid integer is received. The `cin.clear()` function resets the error state, allowing for further attempts, while `cin.ignore()` discards the bad input, ensuring that the next input is taken correctly.
Using Custom Input Functions
Creating a Function for Input
To enhance code readability and reusability, creating custom functions for input can be beneficial. Here’s how you could define such a function:
int getUserInput() {
int input;
cout << "Please enter an integer: ";
cin >> input;
return input;
}
int main() {
int userValue = getUserInput();
cout << "You entered: " << userValue << endl;
return 0;
}
By creating the `getUserInput` function, we encapsulate the input logic, making it reusable throughout the program. This approach not only cleans up the `main` function but also keeps the logic organized.
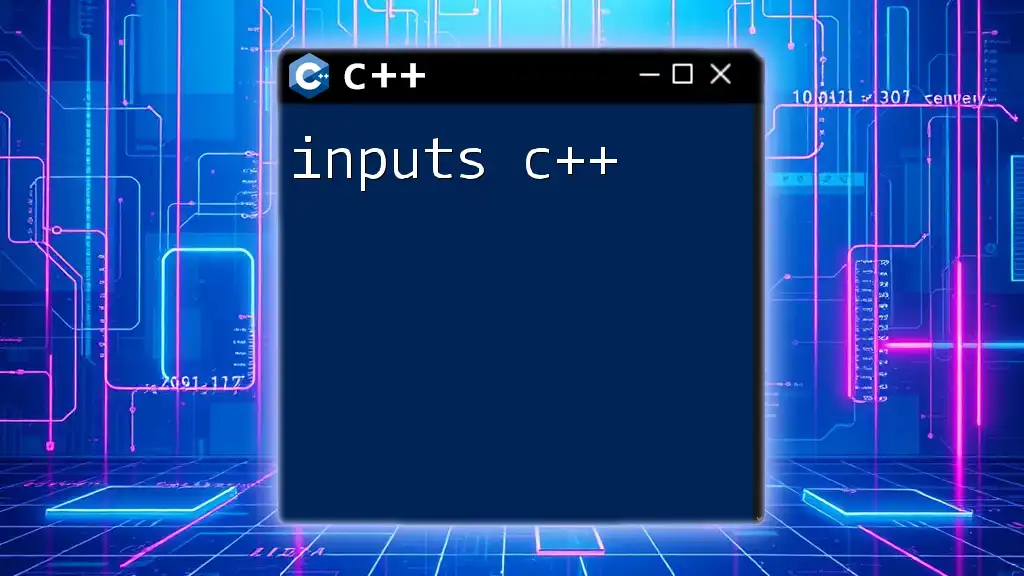
Conclusion
Being proficient in handling input from user c++ is paramount for creating interactive and user-friendly applications. From utilizing `cin` for basic input and `getline` for comprehensive string capture, to implementing validation techniques and custom functions, mastering these skills will significantly enhance your programming capabilities. Encouraging practice in various input scenarios will not only solidify these concepts but also prepare you for more complex programming challenges in the future.
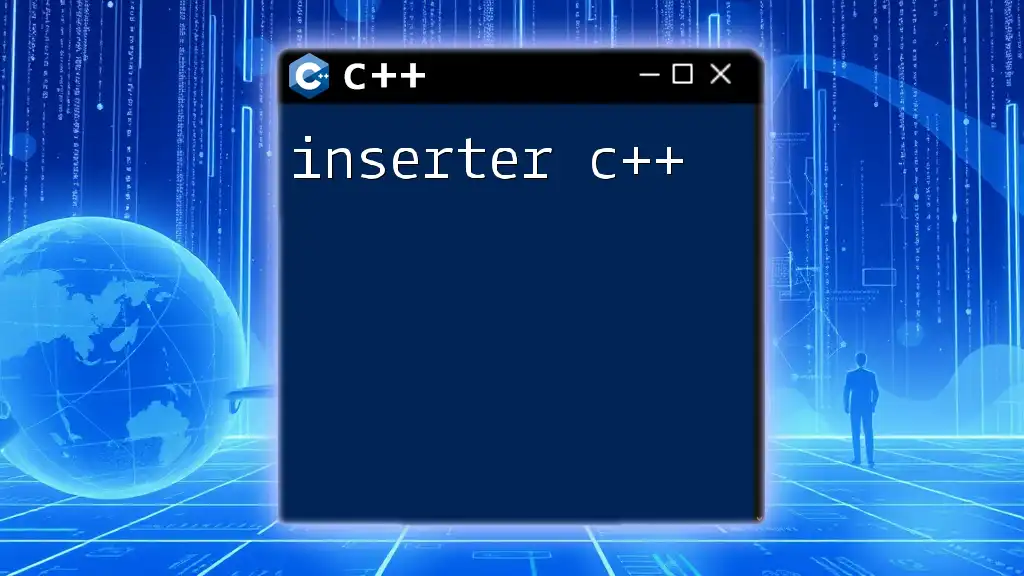
Additional Resources
For further reading and practice, refer to the C++ documentation and seek out exercises that focus specifically on input handling and validation techniques. The more you experiment with user inputs, the more adept you will become at utilizing this fundamental aspect of C++ programming.