The C++ Rule of Five emphasizes the importance of defining five special member functions to manage resources properly in a class that utilizes dynamic memory, including the destructor, copy constructor, copy assignment operator, move constructor, and move assignment operator.
Here’s a basic example of a class implementing the Rule of Five:
class Resource {
public:
Resource() : data(new int[10]) {} // Constructor
~Resource() { delete[] data; } // Destructor
// Copy Constructor
Resource(const Resource& other) : data(new int[10]) {
std::copy(other.data, other.data + 10, data);
}
// Copy Assignment Operator
Resource& operator=(const Resource& other) {
if (this != &other) {
delete[] data;
data = new int[10];
std::copy(other.data, other.data + 10, data);
}
return *this;
}
// Move Constructor
Resource(Resource&& other) noexcept : data(other.data) {
other.data = nullptr;
}
// Move Assignment Operator
Resource& operator=(Resource&& other) noexcept {
if (this != &other) {
delete[] data;
data = other.data;
other.data = nullptr;
}
return *this;
}
private:
int* data;
};
What is the Rule of 5 in C++?
The c++ rule of 5 refers to a set of five special member functions that are critical for proper resource management in classes that use dynamic memory or other resources. It builds upon earlier principles (notably the Rule of 3) to address modern C++ programming needs. This rule emphasizes the need for developers to be conscious of resource ownership, ensuring that classes manage their resources effectively during object creation, copying, and destruction.
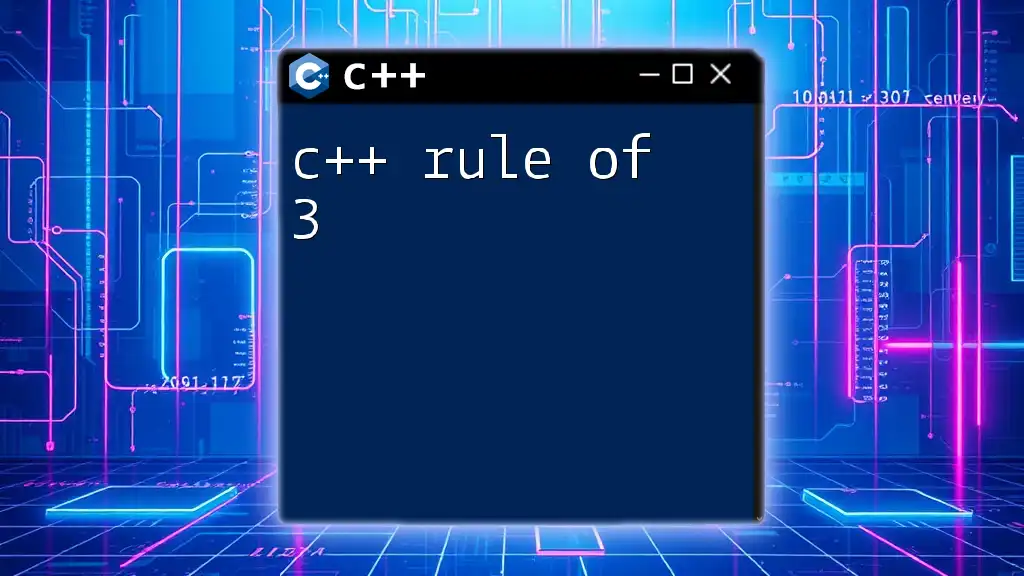
Why the Rule of 5?
The necessity of the Rule of 5 arises from the complexities involved in managing resources manually. In C++, when a class dynamically allocates memory or handles resources such as file descriptors, it must have a defined strategy for releasing those resources when they are no longer needed. A failure to do so can lead to memory leaks, undefined behavior, and other critical issues in applications.
Understanding this rule not only aids in creating more robust and maintainable code but also enhances performance by optimizing how resources are moved rather than copied.
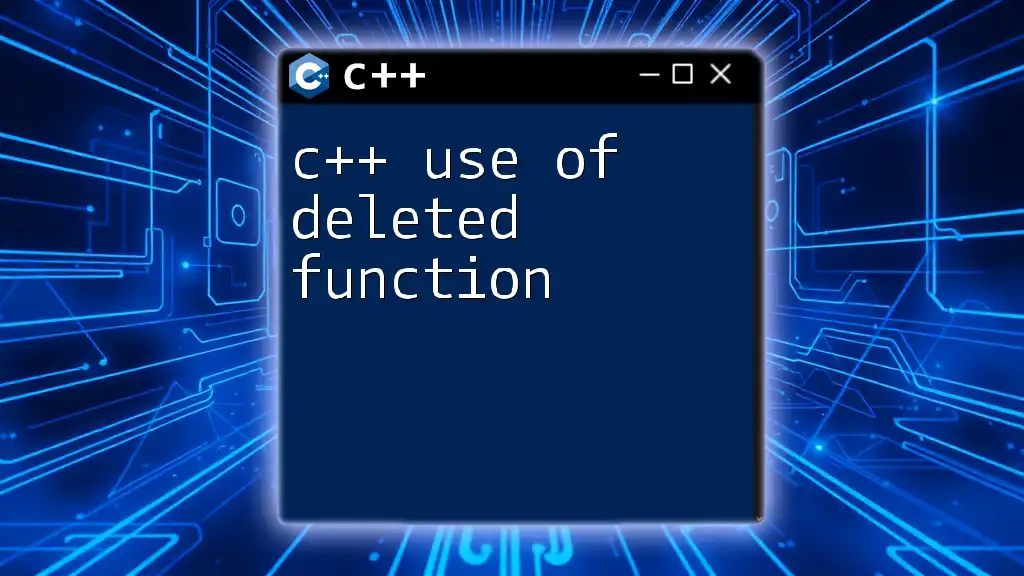
Core Concepts of the Rule of 5
Understanding Resource Management
Resource management is pivotal in C++. It involves allocating and releasing resources throughout the life cycle of an object.
Types of resources include:
- Memory: Using `new` for allocation and forgetting to `delete` can cause leaks.
- File Handles: Opening a file without closing it can lead to file locks or resource exhaustion.
- Network Connections: Improperly managed connections may lead to unexpected disconnections.
When resources are not managed correctly, it's easy to fall into common pitfalls like memory leaks or dangling pointers, which can compromise the integrity of your applications.
The Five Special Member Functions Explained
Default Constructor
The default constructor initializes an object without any parameters. If custom memory management is needed, a user-defined default constructor is essential.
Example:
class Resource {
public:
Resource() {
// Allocate resources.
}
};
The default constructor provides an intuitive way to create instances of a class that require resource initialization. This constructor is necessary in scenarios where default values need to be set or when resources must be allocated.
Copy Constructor
The copy constructor creates a new object as a copy of an existing object. It is vital when your class manages dynamic resources.
Example:
class Resource {
public:
Resource(const Resource& other) {
// Perform a deep copy of resources.
}
};
Understanding the difference between shallow and deep copy is critical here. A shallow copy simply duplicates the pointer, risking double deletion. In contrast, a deep copy replicates the object itself, ensuring that each instance has its own separate copy of the data.
Copy Assignment Operator
The copy assignment operator lets an existing object acquire the values of another existing object.
Example:
class Resource {
public:
Resource& operator=(const Resource& other) {
// Handle self-assignment.
return *this;
}
};
This operator matters because it enables the assignment of already initialized objects, extending the functionality of your class. Proper handling of self-assignment is crucial, where the object is assigned to itself, which could lead to resource loss or corruption without appropriate checks.
Move Constructor
The move constructor enables the transfer of resources from a temporary object, making it a powerful component for efficiency.
Example:
class Resource {
public:
Resource(Resource&& other) noexcept {
// Transfer ownership of resources.
}
};
Thanks to rvalue references, move semantics can significantly improve performance by allowing resources to be transferred rather than copied. This is especially beneficial for handling temporary objects in functions and STL containers.
Move Assignment Operator
The move assignment operator allows an existing object to take ownership of another existing object's resources, enhancing efficiency and avoiding unnecessary copies.
Example:
class Resource {
public:
Resource& operator=(Resource&& other) noexcept {
// Release current resource and take ownership of other's.
return *this;
}
};
In using the move assignment operator, developers must ensure that they release any resources held by the object before taking ownership of the new resources.
Implementing the Rule of 5
Step-by-Step Implementation
To highlight the practical utility of the c++ rule of 5, consider creating a simple class to manage a dynamic array of integers.
class IntArray {
private:
int* data;
size_t size;
public:
IntArray(size_t s) : size(s), data(new int[s]()) {}
// Default constructor
IntArray() : size(0), data(nullptr) {}
// Copy constructor
IntArray(const IntArray& other) : size(other.size), data(new int[other.size]) {
std::copy(other.data, other.data + other.size, data);
}
// Copy assignment operator
IntArray& operator=(const IntArray& other) {
if (this == &other) return *this; // self-assignment check
delete[] data; // free existing resource
size = other.size;
data = new int[size];
std::copy(other.data, other.data + size, data);
return *this;
}
// Move constructor
IntArray(IntArray&& other) noexcept : data(other.data), size(other.size) {
other.data = nullptr; // Leave other in a valid state
other.size = 0;
}
// Move assignment operator
IntArray& operator=(IntArray&& other) noexcept {
if (this == &other) return *this; // self-assignment check
delete[] data; // free existing resource
data = other.data; // take ownership
size = other.size;
other.data = nullptr; // Leave other in a valid state
other.size = 0;
return *this;
}
~IntArray() {
delete[] data; // Release resource
}
};
This example illustrates how to implement each of the five special member functions effectively.
Common Mistakes to Avoid
Despite the clear benefits, there are frequent mistakes developers can make when implementing the Rule of 5. For example, forgetting to handle self-assignment could lead to resource loss during assignments. Not leaving moved-from objects in a valid state might also create issues later in the code.
Best practices suggest always validating resource ownership, carefully managing object destruction, and thoroughly testing all functions to ensure correctness.
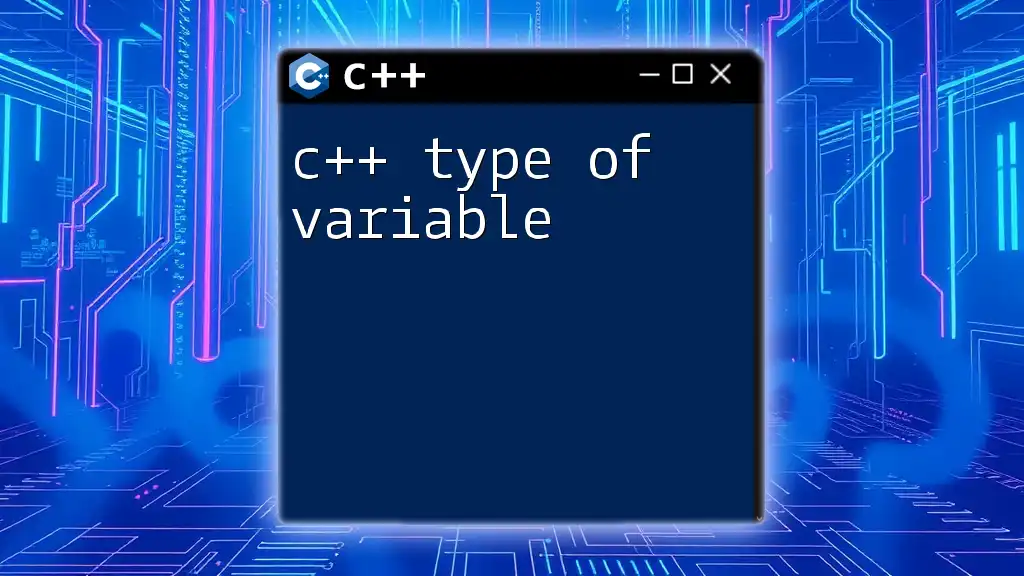
Practical Applications of the Rule of 5
Real-World Scenarios
Over time, the c++ rule of 5 has become essential in various applications requiring resource management. Systems such as databases, image processing libraries, and template containers utilize classes that adhere to this guideline to maintain integrity and performance.
For example, data structures like smart pointers in the C++ Standard Library exemplify how the Rule of 5 is applied for effective memory management, utilizing both move semantics and copy semantics where appropriate.
Testing and Best Practices
A key aspect of implementing the Rule of 5 involves rigorous testing. Each of the five functions should be unit tested to verify memory management practices. Assertions that check for memory leaks following object creation, copying, and moving can ensure robustness.
Utilizing testing frameworks like Google Test can ease this process, allowing developers to create comprehensive tests that validate the performance of the special member functions.
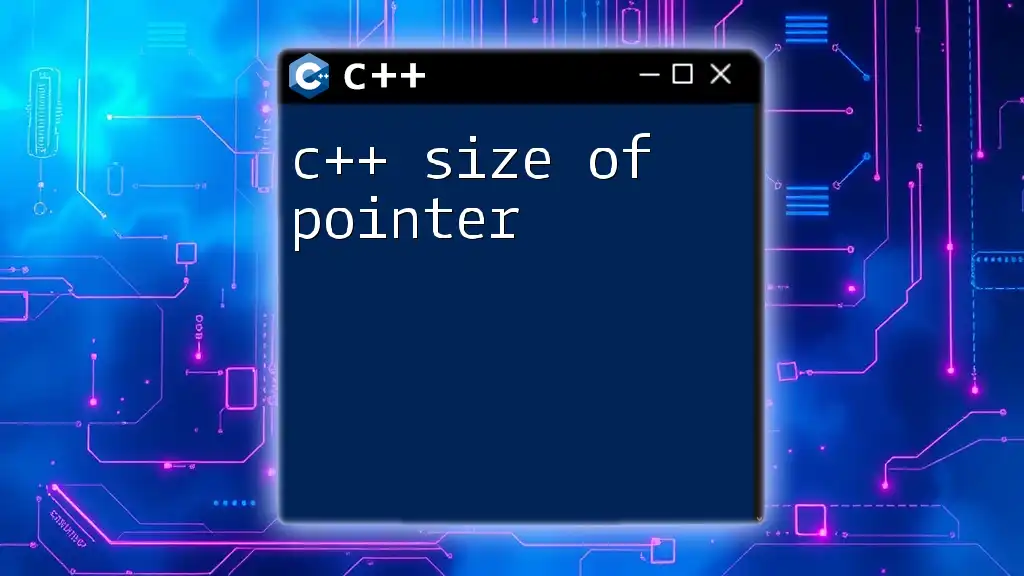
Conclusion
Understanding and applying the c++ rule of 5 is crucial for anyone serious about C++ programming. It encompasses a protected methodology for creating classes that manage resources efficiently and avoids the common pitfalls associated with manual resource management. By mastering the five special member functions, developers can create more reliable and maintainable software systems.
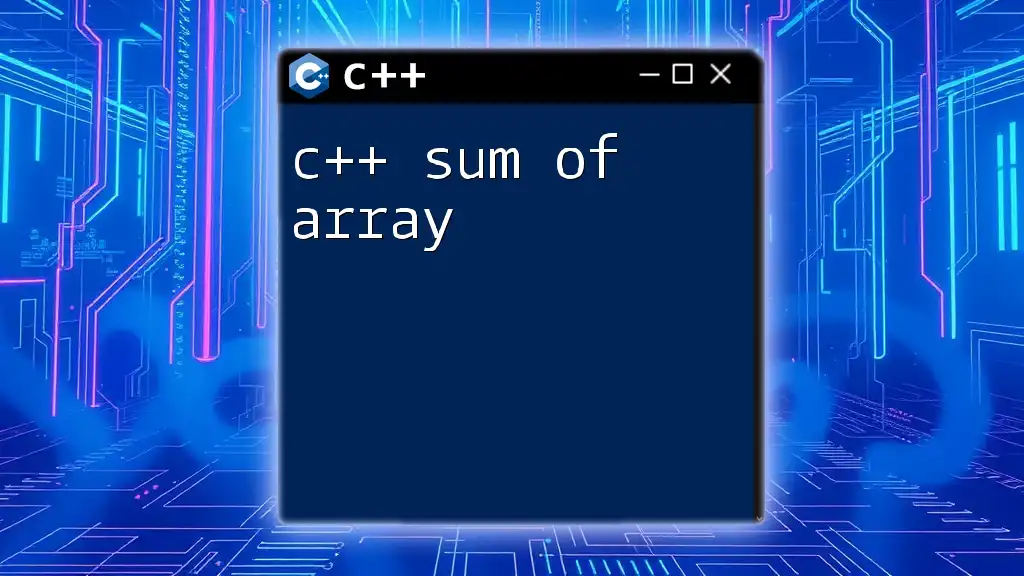
Common Questions and Misconceptions
While the Rule of 5 is well-established, common misconceptions still lurk. One such misunderstanding is the over-application of move semantics—believing that it should always be preferred over copying, regardless of context. In scenarios where copies are more beneficial for readability, the simpler copy operations should still be utilized wisely.
As you grow more familiar with C++, grasping these nuances will become vital for developing high-performance applications that adhere to best practices. By embracing the c++ rule of 5, programmers can craft sophisticated, resource-aware code that thrives in modern programming environments.